开发背景
常用日历组件可能满足不了我们自定义的多种需求(比如样式),因此通常情况下我们可能需要自己手动开发款日历,先上图
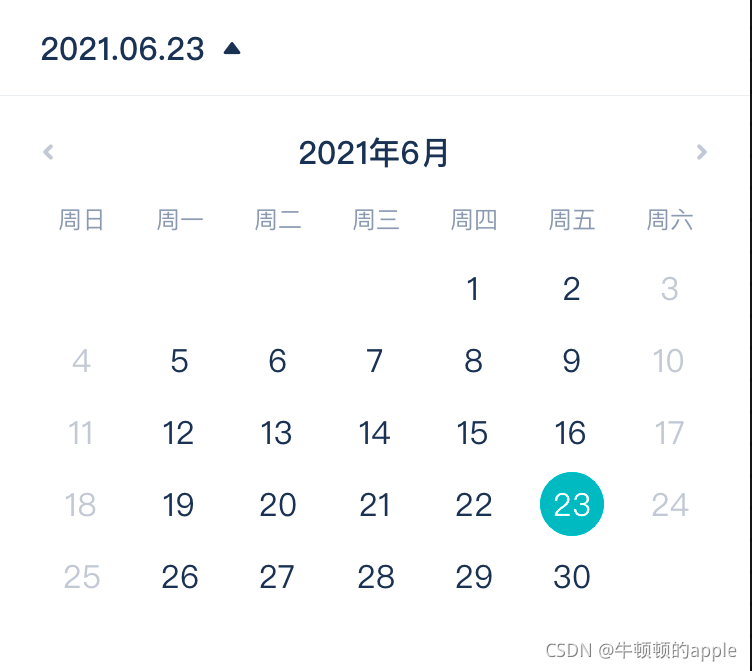
开发流程
1. 根据常用日历样式,我们template部分可以分为三部分(上下月及当前月份展示;周日至周六展示;主体日期展示三部分)
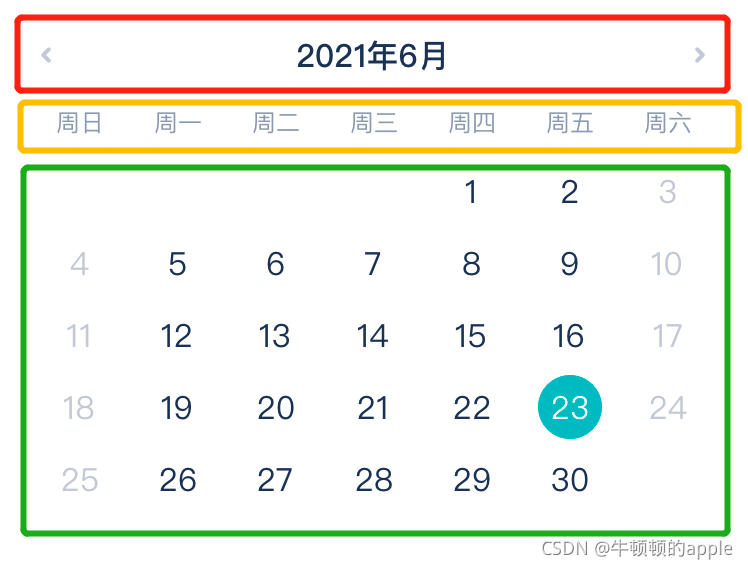
1) template部分代码
<div class="date">
<div class="header">
<span class="pre_month" @click="onPreMonth"></span>
<span v-cloak>{{ date.year }}年{{ date.month }}月</span>
<span class="next_month" @click="onNextMonth"></span>
</div>
<div class="days">
<table v-cloak border="0" cellspacing="0" cellpadding="0">
<tr class="label">
<td>周日</td>
<td>周一</td>
<td>周二</td>
<td>周三</td>
<td>周四</td>
<td>周五</td>
<td>周六</td>
</tr>
<tr class="row" v-for="week in weeks" :key="week[0].date">
<td
class="column"
v-for="day in week"
:key="day.date"
v-bind:day="day.date"
@click="onSelectDay(day)"
>
<span
v-bind:class="[{
checked: selectedDate == day.date,
weekend: day.isWeekend,
}]"
v-if="day.month == date.month">{{ day.v }}</span>
</td>
</tr>
</table>
</div>
</div>
2)js部分代码
// 生成日历函数
initDate(month) {
var weeks = [] // template中用来渲染日历的数组
var firstDay = this.moment(month, 'YYYY-MM') // 当月1号
var week = firstDay.format('d') // 当月1号是周几 (比如周五则week = 5)
var start = firstDay.subtract(week, 'days') // 日历上展示的第一个数(上个月的二十几号之类的,用于补齐日历)
for (var i = 0; i < 6; i++) { // 通常日历为6行7排 42天,因此两个for循环
var days = []
for (var j = 0; j < 7; j++) {
var day = {}
day.num = start.toObject().date // 当前号数 22
day.date = start.format('YYYY-MM-DD') // 返回值为2021-10-22
day.month = start.format('MM') // 当前号数对应的月份,比如日历上个月27号则day.month = 9;这个月1号day.month = 10
day.isWeekend = (start.format('E') === '6' || start.format('E') === '7') ? true : false // 是否是周末,用于UI区分周末和平时的颜色
start.add(1, 'days') // 没循环一次日期加一天
days.push(day)
}
weeks.push(days)
}
this.date.year = this.moment(month).year()
this.date.month = this.moment(month, 'YYYY-MM').add(0, 'month').format('MM')
this.date.preMonth = this.moment(month, 'YYYY-MM').add(-1, 'month').format('YYYY-MM')
this.date.nextMonth = this.moment(month, 'YYYY-MM').add(1, 'month').format('YYYY-MM')
return weeks
}
初始化完成后调用(用于template中渲染)
mounted() {
const currDate = new Date()
this.weeks = this.initDate(this.moment(currDate).format('YYYY-MM'))
}
上个月、下个月处理
// 上一个月
onPreMonth() {
const month = this.date.preMonth
this.weeks = this.getCalendar(this.moment(month).format('YYYY-MM'))
},
// 下一个月
onNextMonth() {
const month = this.date.nextMonth
this.weeks = this.getCalendar(this.moment(month).format('YYYY-MM'))
}
选中某一天
onSelectDay(day) {
if (!this.isSelectDay) return false
if (day.month === this.date.month) {
this.selectedDate = day.date
}
}
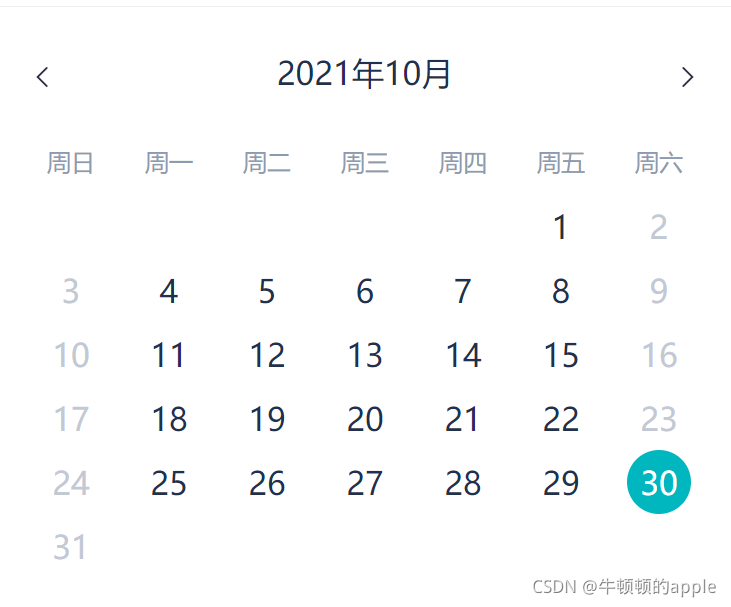
点个赞吧^^