#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int spitString(const char *str, char c, char buf[10][30], int *count)
{
if (str == NULL || count == NULL)
{
return -1;
}
const char *start = str;
char * p = NULL;
int i = 0;
do
{
p = strchr(start, c);
if (p != NULL)
{
int len = p - start;
strncpy(buf[i], start, len);
buf[i][len] = 0;
i++;
start = p + 1;
}
else
{
strcpy(buf[i], start);
i++;
break;
}
} while (*start != 0);
if (i == 0)
{
return -2;
}
*count = i;
return 0;
}
int main01(void)
{
const char *p = "abcdef,acccd,eeee,aaaa,e3eeee,ssss";
char buf[10][30] = { 0 };
int n = 0;
int i = 0;
int ret = 0;
ret = spitString(p, ',', buf, &n);
if (ret != 0)
{
printf("spitString err:%d\n", ret);
return ret;
}
for (i = 0; i < n; i++)
{
printf("%s\n", buf[i]);
}
printf("\n");
return 0;
}
char **getMem(int n)
{
char **buf = NULL;
buf = (char **)malloc(n * sizeof(char *));
if (buf == NULL)
{
return NULL;
}
int i = 0;
for (i = 0; i < n; i++)
{
buf[i] = (char *)malloc(30);
memset(buf[i], 0, 30);
}
return buf;
}
int getMem2(char ***tmp, int n)
{
char **buf = NULL;
buf = (char **)malloc(n * sizeof(char *));
if (buf == NULL)
{
return -1;
}
int i = 0;
for (i = 0; i < n; i++)
{
buf[i] = (char *)malloc(30);
memset(buf[i], 0, 30);
}
*tmp = buf;
return 0;
}
int spitString2(const char *str, char c, char **buf, int *count)
{
if (str == NULL || count == NULL)
{
return -1;
}
const char *start = str;
char * p = NULL;
int i = 0;
do
{
p = strchr(start, c);
if (p != NULL)
{
int len = p - start;
strncpy(buf[i], start, len);
buf[i][len] = 0;
i++;
start = p + 1;
}
else
{
strcpy(buf[i], start);
i++;
break;
}
} while (*start != 0);
if (i == 0)
{
return -2;
}
*count = i;
return 0;
}
void freeBuf3(char **buf, int n)
{
int i = 0;
for (i = 0; i < n; i++)
{
free(buf[i]);
buf[i] = NULL;
}
if (buf != NULL)
{
free(buf);
buf = NULL;
}
}
void freeBuf4(char ***tmp, int n)
{
char **buf = *tmp;
int i = 0;
for (i = 0; i < n; i++)
{
free(buf[i]);
buf[i] = NULL;
}
if (buf != NULL)
{
free(buf);
buf = NULL;
}
*tmp = NULL;
}
int main(void)
{
const char *p = "abcdef,acccd,eeee,aaaa,e3eeee,ssss";
char **buf = NULL;
int n = 0;
int i = 0;
int ret = 0;
#if 0
buf = getMem(6);
if (buf == NULL)
{
return -1;
}
#endif
ret = getMem2(&buf, 6);
if (ret != 0)
{
return ret;
}
ret = spitString2(p, ',', buf, &n);
if (ret != 0)
{
printf("spitString err:%d\n", ret);
return ret;
}
for (i = 0; i < n; i++)
{
printf("%s\n", buf[i]);
}
freeBuf4(&buf, n);
if(buf != NULL)
{
free(buf);
buf = NULL;
}
#if 0
for (i = 0; i < n; i++)
{
free(buf[i]);
buf[i] = NULL;
}
if (buf != NULL)
{
free(buf);
buf = NULL;
}
#endif
return 0;
}
测试结果
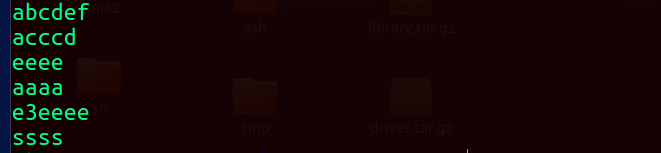
#define _CRT_SECURE_NO_WARNINGS
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
int spitString(const char *buf1, char c, char buf2[10][30], int *count)
{
const char *p=NULL, *pTmp = NULL;
int tmpcount = 0;
if(buf1 == NULL || count == NULL)
return -1;
p = buf1;
pTmp = buf1;
do
{
p = strchr(p, c);
if (p != NULL)
{
if (p-pTmp > 0)
{
strncpy(buf2[tmpcount], pTmp, p-pTmp);
buf2[tmpcount][p-pTmp] = '\0';
tmpcount ++;
pTmp = p = p + 1;
}
}
else
{
break;
}
} while (*p!='\0');
*count = tmpcount;
return 0;
}
int main(int argc, const char * argv[])
{
int ret = 0, i = 0;
char *p1 = "abcdef,acccd,eeee,aaaa,e3eeeee,sssss,";
char cTem= ',';
int nCount;
char myArray[10][30];
memset(myArray, 0, sizeof(myArray));
ret = spitString(p1, cTem, myArray, &nCount);
if (ret != 0)
{
printf("fucn spitString() err: %d \n", ret);
return ret;
}
for (i=0; i<nCount; i++ )
{
printf("%s \n", myArray[i]);
}
return 0;
}
测试结果
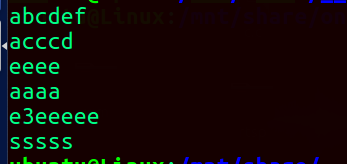
#define _CRT_SECURE_NO_WARNINGS
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
int spitString(const char *buf1, char c, char buf2[][30], int *count)
{
const char *p=NULL, *pTmp = NULL;
int tmpcount = 0;
p = buf1;
pTmp = buf1;
do
{
p = strchr(p, c);
if (p != NULL)
{
if (p-pTmp > 0)
{
strncpy(buf2[tmpcount], pTmp, p-pTmp);
buf2[tmpcount][p-pTmp] = '\0';
tmpcount ++;
pTmp = p = p + 1;
}
}
else
{
break;
}
} while (*p!='\0');
*count = tmpcount;
return 0;
}
int main(int argc, const char * argv[])
{
int ret = 0, i = 0;
char *p1 = "abcdef,acccd,eeee,aaaa,e3eeeee,sssss,";
char cTem= ',';
int nCount;
char myArray[10][30];
ret = spitString(p1, cTem, myArray, &nCount);
if (ret != 0)
{
printf("fucn spitString() err: %d \n", ret);
return ret;
}
for (i=0; i<nCount; i++ )
{
printf("%s \n", myArray[i]);
}
return 0;
}
测试结果
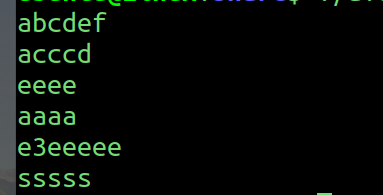
#define _CRT_SECURE_NO_WARNINGS
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
int spitString(const char *buf1, char c, char **myp , int *count)
{
const char *p=NULL, *pTmp = NULL;
int tmpcount = 0;
p = buf1;
pTmp = buf1;
do
{
p = strchr(p, c);
if (p != NULL)
{
if (p-pTmp > 0)
{
strncpy(myp[tmpcount], pTmp, p-pTmp);
myp[tmpcount][p-pTmp] = '\0';
tmpcount ++;
pTmp = p = p + 1;
}
}
else
{
break;
}
} while (*p!='\0');
*count = tmpcount;
return 0;
}
int main(int argc, const char * argv[])
{
int ret = 0, i = 0;
char *p1 = "abcdef,acccd,eeee,aaaa,e3eeeee,sssss,";
char cTem= ',';
int nCount;
char **p = NULL;
p = (char **)malloc(10 * sizeof(char *));
if (p == NULL)
{
return -1;
}
for (i=0; i<10; i++)
{
p[i] = (char *)malloc(30 * sizeof(char));
}
ret = spitString(p1, cTem, p, &nCount);
if (ret != 0)
{
printf("fucn spitString() err: %d \n", ret);
return ret;
}
for (i=0; i<nCount; i++ )
{
printf("%s \n", p[i]);
}
for (i=0; i<10; i++)
{
free(p[i]);
p[i] = NULL;
}
free(p);
p = NULL;
return 0;
}
测试结果
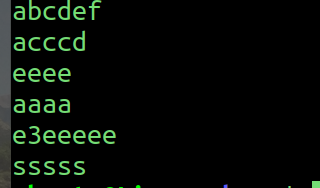
#define _CRT_SECURE_NO_WARNINGS
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
char ** spitString(const char *buf1, char c, int *count)
{
const char *p=NULL, *pTmp = NULL;
int tmpcount = 0;
char **myp = NULL;
p = buf1;
pTmp = buf1;
do
{
p = strchr(p, c);
if (p != NULL)
{
if (p-pTmp > 0)
{
tmpcount ++;
pTmp = p = p + 1;
}
}
else
{
break;
}
} while (*p!='\0');
*count = tmpcount;
myp = (char **)malloc(tmpcount * sizeof(char *) );
if (myp == NULL)
{
return NULL;
}
tmpcount = 0;
p = buf1;
pTmp = buf1;
do
{
p = strchr(p, c);
if (p != NULL)
{
if (p-pTmp > 0)
{
int len = p - pTmp + 1;
myp[tmpcount] = (char *)malloc(len * sizeof(char));
if (myp[tmpcount] == NULL)
{
return NULL;
}
strncpy(myp[tmpcount], pTmp, p-pTmp);
myp[tmpcount][p-pTmp] = '\0';
tmpcount ++;
pTmp = p = p + 1;
}
}
else
{
break;
}
} while (*p!='\0');
return myp;
}
void FreeMem2(char **myp, int count)
{
int i =0;
if (myp == NULL)
{
return;
}
for (i=0; i<count; i++)
{
if (myp[i] != NULL)
{
free(myp[i]);
}
}
if (myp != NULL)
{
free(myp);
}
}
void FreeMem3(char ***p, int count)
{
int i =0;
char **myp = NULL;
if (p == NULL)
{
return;
}
myp = *p;
if (myp == NULL)
{
return ;
}
for (i=0; i<count; i++)
{
if (myp[i] != NULL)
{
free(myp[i]);
}
}
if (myp != NULL)
{
free(myp);
}
*p = NULL;
}
int spitString4(const char *buf1, char c, char ***myp3, int *count)
{
int ret = 0;
const char *p=NULL, *pTmp = NULL;
int tmpcount = 0;
char **myp = NULL;
pTmp = buf1;
p = buf1;
do
{
p = strchr(p, c);
if (p != NULL)
{
if (p-pTmp > 0)
{
tmpcount ++;
pTmp = p = p + 1;
}
}
else
{
break;
}
} while (*p!='\0');
*count = tmpcount;
myp = (char **)malloc(tmpcount * sizeof(char *) );
if (myp == NULL)
{
ret = -1;
printf("func spitString4() err:%d (tmpcount * sizeof(char *) )", ret);
goto END;
}
memset(myp, 0, tmpcount * sizeof(char *));
tmpcount = 0;
p = buf1;
pTmp = buf1;
do
{
p = strchr(p, c);
if (p != NULL)
{
if (p-pTmp > 0)
{
int len = p - pTmp + 1;
myp[tmpcount] = (char *)malloc(len * sizeof(char));
if (myp[tmpcount] == NULL)
{
ret = -2;
printf("func spitString4() err:%d malloc(len * sizeof(char) )", ret);
goto END;
}
strncpy(myp[tmpcount], pTmp, p-pTmp);
myp[tmpcount][p-pTmp] = '\0';
tmpcount ++;
pTmp = p = p + 1;
}
}
else
{
break;
}
} while (*p!='\0');
END:
if (ret != 0)
{
FreeMem3(&myp, *count);
}
else
{
*myp3 = myp;
}
return ret;
}
#if 0
int main(int argc, const char * argv[])
{
int ret = 0, i = 0;
char *p1 = "abcdef,acccd,";
char cTem= ',';
int nCount;
char **p = NULL;
p= spitString(p1, cTem, &nCount);
if (p == NULL)
{
printf("fucn spitString() err: %d \n", ret);
return ret;
}
for (i=0; i<nCount; i++ )
{
printf("%s \n", p[i]);
}
for (i=0; i<nCount; i++)
{
free(p[i]);
p[i] = NULL;
}
free(p);
p[i] = NULL;
return 0;
}
#else
int main(int argc, const char * argv[])
{
int ret = 0, i = 0;
char *p1 = "abcdef,111222,444444,";
char cTem= ',';
int nCount;
char **p = NULL;
ret = spitString4(p1, cTem, &p, &nCount);
if (ret != 0)
{
printf("fucn spitString() err: %d \n", ret);
return ret;
}
for (i=0; i<nCount; i++ )
{
printf("%s \n", p[i]);
}
for (i=0; i<nCount; i++)
{
free(p[i]);
}
free(p);
return 0;
}
#endif
测试结果
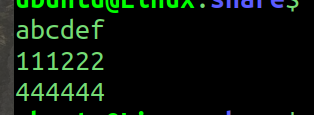