//
// CopyRight 2011 //
//
// By 张哲 //
//
// 东南大学--仪器科学与工程学院 //
/
#include<iostream>
#include<fstream>
#include<sstream>
#include<string>
#include<iomanip>
#include<vector>
#include<algorithm>
#include<numeric>
#include<functional>
using namespace std;
class Course{
private:
int no;//序号
string year;//学年学期
int code;//课程代码
string name;//课程名称
float credit;//学分
int score;//成绩
string quality;//成绩性质
string courseQua;//课程性质
float gpa;//绩点,(非绩点和)
public:
//构造与析构 及赋值运算符
Course(){}
Course(int noV,string yearV,int codeV,string nameV,float creditV,int scoreV,string qualityV,string courseQuaV="#")
:no(noV),year(yearV),code(codeV),name(nameV),credit(creditV),score(scoreV),quality(qualityV),courseQua(courseQuaV){}
Course(const Course& temp)
{
no = temp.no;
year = temp.year;
code = temp.code;
name = temp.name;
credit = temp.credit;
score = temp.score;
quality = temp.quality;
courseQua = temp.courseQua;
gpa = temp.gpa;
}
~Course(){}
Course& operator = (const Course& temp)
{
no = temp.no;
year = temp.year;
code = temp.code;
name = temp.name;
credit = temp.credit;
score = temp.score;
quality = temp.quality;
courseQua = temp.courseQua;
gpa = temp.gpa;
return *this;
}
//访问器成员函数
string getYear() const {return year;}
string getName() const {return name;}
float getCredit() const {return credit;}
float getGpa() const {return gpa;}
int getScore() const {return score;}
//mutator 函数
void set(int noV,string yearV,int codeV,string nameV,float creditV,int scoreV,string qualityV,string courseQuaV="#")
{
no=noV;
year=yearV;
code=codeV;
name=nameV;
credit=creditV;
score=scoreV;
quality=qualityV;
courseQua=courseQuaV;
}
void setGpa()
{
if(score<60) gpa = 0;
if(score>=60&&score<=62) gpa = 1.0;
if(score>=63&&score<=65) gpa = 1.5;
if(score>=66&&score<=69) gpa = 1.8;
if(score>=70&&score<=72) gpa = 2.0;
if(score>=73&&score<=75) gpa = 2.5;
if(score>=76&&score<=79) gpa = 2.8;
if(score>=80&&score<=82) gpa = 3.0;
if(score>=83&&score<=85) gpa = 3.5;
if(score>=86&&score<=89) gpa = 3.8;
if(score>=90&&score<=92) gpa = 4.0;
if(score>=93&&score<=95) gpa = 4.5;
if(score>=96&&score<=100) gpa = 4.8;
}
void show()
{
cout<<left<<setw(10)<<year<<setw(36)<<name;
cout<<setw(5)<<credit<<setw(5)<<score;
cout<<setw(6)<<quality<<setw(6)<<gpa<<endl;
}
//运算符重载
bool operator < (const Course& temp) const
{
if(score < temp.score) return true;
else return false;
}
bool operator > (const Course& temp) const
{
if(score > temp.score) return true;
else return false;
}
friend class List;
};
class CourseSigma: public Course
{
private:
float creditPlusGpa;
public:
CourseSigma(const Course& temp):Course(temp),creditPlusGpa(temp.getCredit() * temp.getGpa()){}
bool operator < (const CourseSigma& temp) const
{
return creditPlusGpa < temp.creditPlusGpa? true : false ;
}
bool operator > (const CourseSigma& temp) const
{
return creditPlusGpa > temp.creditPlusGpa? true : false ;
}
void show()
{
cout<<left<<setw(10)<<getYear()<<setw(36)<<getName();
cout<<setw(5)<<getCredit()<<setw(5)<<getScore();
cout<<setw(6)<<getGpa()<<setw(5)<<creditPlusGpa<<endl;
}
};
class List{
public:
vector<Course> data;
public:
List(){}
~List(){}
//
void add(const Course& temp){data.push_back(temp);}//在矢量尾添加一个元素
void sort_des(){sort( data.begin() , data.end() , less<Course>() );}//升序排序
void sort_asc(){sort( data.begin() , data.end() , greater<Course>() );}//降序排序
void clear_list(){ data.clear(); }
int getAmoun
[源代码] [东南大学]学分绩点计算器gpa 1.0.2 升级版
最新推荐文章于 2022-03-18 09:53:14 发布
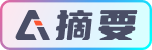