最近帮同学写一个二维数组的回形遍历,看了一下,没什么好思路,百度,google了一把,使用递归算法还是比较好理解的,记录已备用
1 什么是螺旋遍历?
2 实现的思路是递归:
Solution: There are several ways to solve this problem, but I am mentioning a method that is intuitive to understand and easy to implement. The idea is to consider the matrix similar to onion which can be peeled layer after layer. We can use the same approach to print the outer layer of the matrix and keep doing it recursively on a smaller matrix (with 1 less row and 1 less column).
图形理解:
3 C语言实现:
1 什么是螺旋遍历?
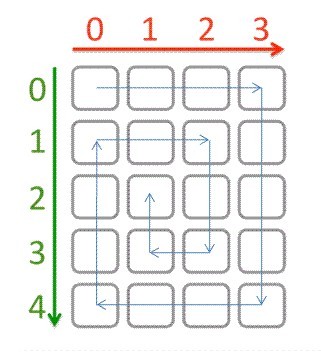
2 实现的思路是递归:
Solution: There are several ways to solve this problem, but I am mentioning a method that is intuitive to understand and easy to implement. The idea is to consider the matrix similar to onion which can be peeled layer after layer. We can use the same approach to print the outer layer of the matrix and keep doing it recursively on a smaller matrix (with 1 less row and 1 less column).
图形理解:
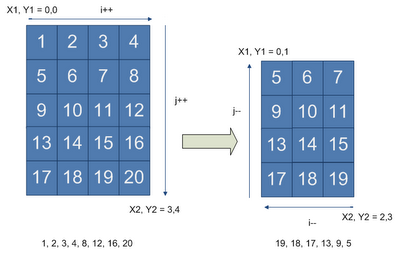
3 C语言实现:
- #include <stdio.h>
- #include <stdlib.h>
- void print_layer_top_right(int a[][4], int col, int row, int collen, int rowlen);
- void print_layer_bottom_left(int a[][4], int col, int row, int collen, int rowlen);
-
- int main(void)
- {
- int a[5][4] = {
- {1,2,3,4},
- {5,6,7,8},
- {9,10,11,12},
- {13,14,15,16},
- {17,18,19,20}
- };
-
- print_layer_top_right(a,0,0,3,4);
- exit(0);
- }
-
- //
- // prints the top and right shells of the matrix
- //
- void print_layer_top_right(int a[][4], int col, int row, int collen, int rowlen)
- {
- int i = 0, j = 0;
-
- // print the row
- for(i = col; i<=collen; i++)
- {
- printf("%d,", a[row][i]);
- }
-
- //print the column
- for(j = row + 1; j <= rowlen; j++)
- {
- printf("%d,", a[j][collen]);
- }
-
- // see if we have more cells left
- if(collen-col > 0)
- {
- // 'recursively' call the function to print the bottom-left layer
- print_layer_bottom_left(a, col, row + 1, collen-1, rowlen);
- }
- }
-
- //
- // prints the bottom and left shells of the matrix
-
- void print_layer_bottom_left(int a[][4], int col, int row, int collen, int rowlen)
- {
- int i = 0, j = 0;
-
- //print the row of the matrix in reverse
- for(i = collen; i>=col; i--)
- {
- printf("%d,", a[rowlen][i]);
- }
-
- // print the last column of the matrix in reverse
- for(j = rowlen - 1; j >= row; j--)
- {
- printf("%d,", a[j][col]);
- }
-
- if(collen-col > 0)
- {
- // 'recursively' call the function to print the top-right layer
- print_layer_top_right(a, col+1, row, collen, rowlen-1);
- }
- }
C Code2:
//打印回形数组
#include<iostream>
using namespace std;
void Traval(int a[][5],int row,int col,int rowlen,int collen)
{
if (row<=rowlen&&col<=collen)
{
//打印上行
for(int j1=col;j1<=collen;j1++)
printf("%d,",a[row][j1]);
//打印右列
for(int i1=row+1;i1<=rowlen;i1++)
printf("%d,",a[i1][collen]);
//打印下行
for(int j2=collen-1;j2>=col;j2--)
printf("%d,",a[rowlen][j2]);
//打印左列
for(int i2=rowlen-1;i2>=row+1;i2--)
printf("%d,",a[i2][col]);
}
else
return;
Traval(a,row+1,col+1,rowlen-1,collen-1);
}
int main()
{
//int aa[4][5]={{1,2,3,4,5},{6,7,8,9,10},{11,12,13,14,15},{16,17,18,19,20}};//4行5列
int aa[5][5]={{1,2,3,4,5},{6,7,8,9,10},{11,12,13,14,15},{16,17,18,19,20},{21,22,23,24,25}};//5行5列
cout<<"回形打印:"<<endl;
Traval(aa,0,0,4,3);//其中,4和3可以随意设置,按要求打印
cout<<endl;
return 0;
}
输出结果:
回形打印:
1,2,3,4,9,14,19,24,23,22,21,16,11,6,7,8,13,18,17,12,
请按任意键继续. . .
4 ref:
http://shashank7s.blogspot.com/2011/04/wap-to-print-2d-array-matrix-in-spiral.html
http://ideone.com/Ik6bFr