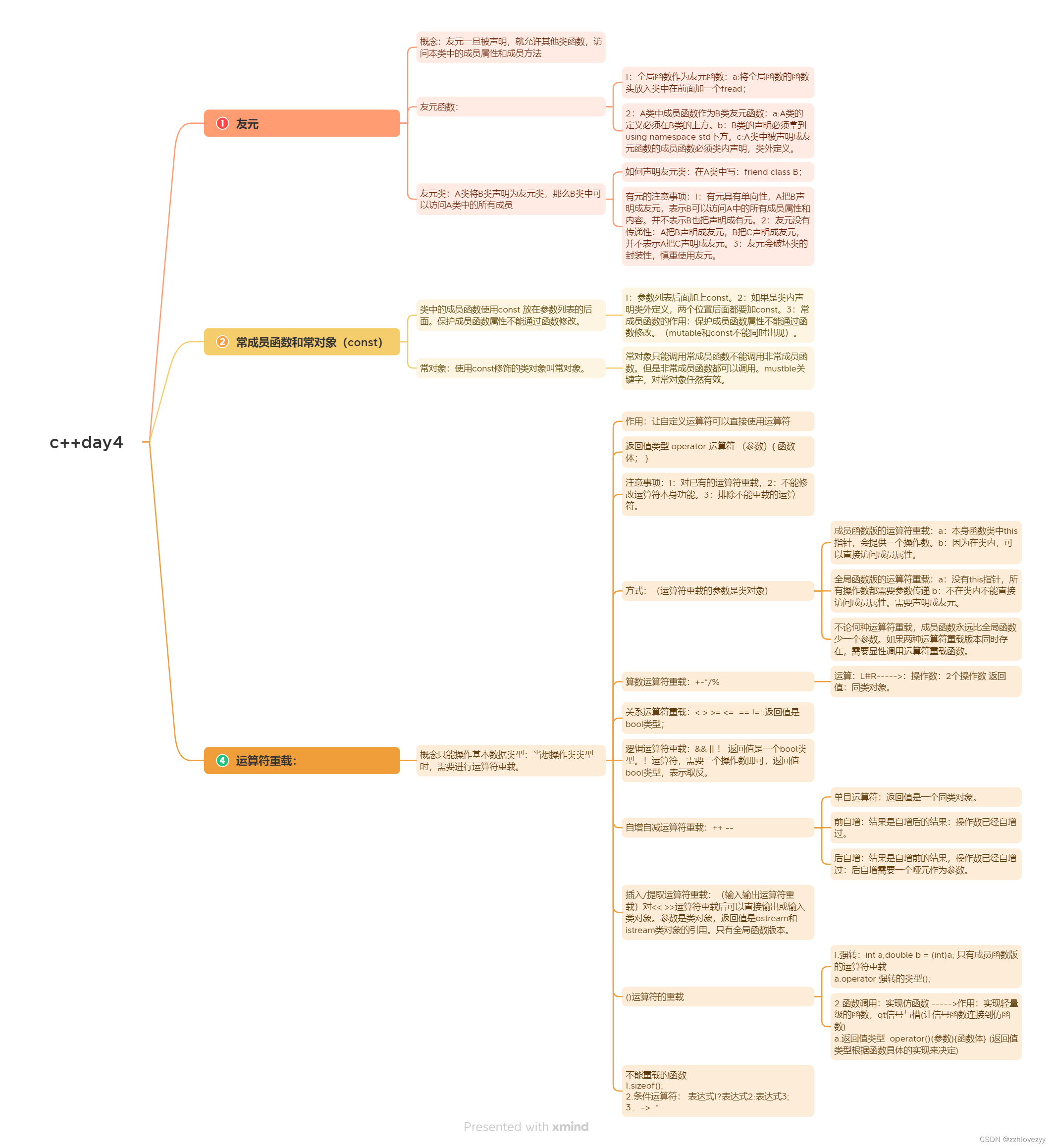
#include <iostream>
#include <cstring>
using namespace std;
char c='\0';
class myString
{
private:
char* str;
int size;
int rel;
int vir;
public:
myString():str(new char),size(0){}
myString(char* p,int size):str(new char[size+1]),size(size)
{
strcpy(str,p);
}
myString(string s1):str(new char[s1.size()+1]),size(s1.length())
{
strcpy(str,s1.c_str());
}
//拷贝构造
myString(const myString &other):str(new char[other.size+1]),size(size)
{
strcpy(str,other.str);
}
//拷贝赋值
myString &operator=(const myString &other)
{
if(&other!=this)
{
delete[]str;
str=new char[other.size+1];
strcpy(str,other.str);
this->size=other.size;
}
return *this;
}
//析构函数
~myString()
{
delete[]str;
}
//判空函数
bool empty()
{
return size==0;
}
//size函数
int size_()
{
return size;
}
//c_str函数
const char* c_str()
{
return str;
}
//at函数
char &at(int pos)
{
if(pos<0||pos>=size)
{
cout <<"位置不合理:" << endl;
return c;
}
return str[pos];
}
void show()
{
cout <<this->rel <<"+" <<this->vir <<endl;
}
myString operator+(const myString m1);
bool operator>(const myString m1);
friend bool operator==(const myString m1,const myString m2);
friend istream &operator>>(istream &in,myString &m1);
friend ostream &operator <<(ostream &out,myString &m1);
};
myString myString::operator+(const myString m1)
{
myString temp;
temp.rel=this->rel+m1.rel;
temp.vir=this->vir+m1.vir;
return temp;
}
bool myString::operator>(const myString m1)
{
return this->rel>m1.rel;
}
bool operator ==(const myString m1,const myString m2)
{
return m1.rel==m2.rel&&m1.vir==m2.vir;
}
istream &operator>>(istream &in,myString &m1)
{
in >> m1.rel >>m1.vir;
return in;
}
ostream &operator <<(ostream &out,myString &m1)
{
out <<m1.rel <<m1.vir;
return out;
}
int main()
{
char str[]="hello";
myString str1(str,5);
cout <<str1.empty() <<endl;
cout <<str1.size_() <<endl;
cout <<str1.c_str() <<endl;
cout <<str1.at(3) <<endl;
str1.at(3)='9';
cout <<str1.at(3) <<endl;
return 0;
}
Complex Complex::operator^(Complex c1)
{
Complex temp;
temp.rel=(this->rel)^c1.rel;
temp.vir=(this->vir)^c1.vir;
return temp;
}
Complex Complex::operator<<(int a)
{
rel=rel<<a;
vir=vir<<a;
return *this;
}
Complex Complex::operator>>(int a)
{
rel=rel>>a;
vir=vir>>a;
return *this;
}
Complex Complex::operator~()
{
rel=~rel;
vir=~vir;
return *this;