package downloadimg;
import java.awt.image.BufferedImage;
import java.io.BufferedInputStream;
import java.io.BufferedReader;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.URL;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.imageio.ImageIO;
import javax.net.ssl.HttpsURLConnection;
import javax.net.ssl.SSLContext;
import javax.net.ssl.TrustManager;
import javax.net.ssl.X509TrustManager;
/**
* @author Cytosine
* @thanks Wizard's helping with my JAVA learning
* @thanks Breakshadow for the idea of using the following API
* get CAPTCHA Img URL's API:https://passport.baidu.com/v2/?reggetcodestr
* The CAPTCHA Img'url is "https://passport.baidu.com/cgi-bin/genimage?"add verifystr that get by above API.
*/
public class DownloadImgWithTypeRecognize {
enum Type{NULL,ORANGE,LIGHT,DARK,UNKNOW}
public static void main(String[] args){
for(int i=0;i<1000;i++){
BufferedImage bi=httpsGetImg(httpsGetImgURL());
Type type=getType(bi);
String path="";
switch(type){
case ORANGE:path="D:\\TEST\\ORANGE\\"+i+".png";break;
case LIGHT:path="D:\\TEST\\LIGHT\\"+i+".png";break;
case DARK:path="D:\\TEST\\DARK\\"+i+".png";break;
}
//save according to img type
File f=new File(path);
try {
ImageIO.write(bi, "png", f);
} catch (IOException ex) {
Logger.getLogger(DownloadImgWithTypeRecognize.class.getName()).log(Level.SEVERE, null, ex);
}
}
}
private static Type getType(BufferedImage bufferedImg){
Type result=Type.NULL;
int width=bufferedImg.getWidth()-1;
int height=bufferedImg.getHeight()-1;
int minx=bufferedImg.getMinX();
int miny=bufferedImg.getMinY();
int[] RGBPixel=new int[3];
int pixel;
do{
pixel=bufferedImg.getRGB(minx, miny);
RGBPixel[0] = (pixel & 0xff0000) >> 16;
RGBPixel[1] = (pixel & 0xff00) >> 8;
RGBPixel[2] = (pixel & 0xff);
if(RGBPixel[0]!=255){//不是白色
if(RGBPixel[2]>=254&RGBPixel[0]>52&RGBPixel[0]<251&RGBPixel[1]>103&RGBPixel[1]<252){//一定是浅蓝
result=Type.LIGHT;
}else{//区分出深蓝和橙色
if(RGBPixel[0]==200&RGBPixel[1]==80&RGBPixel[2]==80){
result=Type.ORANGE;
}else{
if(RGBPixel[0]>2&RGBPixel[0]<70&RGBPixel[1]>54&RGBPixel[1]<107&RGBPixel[2]>105&RGBPixel[2]<144){
result=Type.DARK;
}
}
}
}
miny++;
if(miny==height){
minx++;
miny=0;
}
if(minx==width){
result=Type.UNKNOW;
}
}while(result==Type.NULL);
return result;
}
private static BufferedImage httpsGetImg(String urlString){
BufferedInputStream bufferedInputStream=null;
ByteArrayOutputStream byteArrayOutputStream=new ByteArrayOutputStream();
try{
SSLContext ssl=SSLContext.getInstance("TLS");
ssl.init(null, new TrustManager[]{cytoX509TrustManager}, null);
URL url=new URL(urlString);
HttpsURLConnection conn=(HttpsURLConnection)url.openConnection();
conn.setSSLSocketFactory(ssl.getSocketFactory());
conn.setRequestMethod("GET");
conn.connect();
bufferedInputStream=new BufferedInputStream(conn.getInputStream());
}catch(Exception ex){
ex.printStackTrace();
}
try{
int b;
while((b=bufferedInputStream.read())!=-1){
byteArrayOutputStream.write(b);
}
bufferedInputStream.close();
}catch(Exception ex){
ex.printStackTrace();
}
byte[] imgData=byteArrayOutputStream.toByteArray();
ByteArrayInputStream in=new ByteArrayInputStream(imgData);
BufferedImage result=null;
try {
result=ImageIO.read(in);
} catch (IOException ex) {
Logger.getLogger(DownloadImgWithTypeRecognize.class.getName()).log(Level.SEVERE, null, ex);
}
return result;
}
private static String httpsGetImgURL(){
StringBuffer requestResult=new StringBuffer();
BufferedReader bufferedReader=null;
try{
SSLContext ssl=SSLContext.getInstance("TLS");
ssl.init(null, new TrustManager[]{cytoX509TrustManager}, null);
URL url=new URL("https://passport.baidu.com/v2/?reggetcodestr");
HttpsURLConnection conn=(HttpsURLConnection)url.openConnection();
conn.setSSLSocketFactory(ssl.getSocketFactory());
conn.setRequestMethod("GET");
conn.connect();
bufferedReader=new BufferedReader(new InputStreamReader(conn.getInputStream()));
}catch(Exception ex){
ex.printStackTrace();
}
try{
String line;
while((line=bufferedReader.readLine())!=null){
requestResult.append(line);
}
bufferedReader.close();
}catch(Exception ex){
ex.printStackTrace();
}
String all=requestResult.toString();
String[] spl=all.split("'");
String verifystr=spl[3];
String result="https://passport.baidu.com/cgi-bin/genimage?"+verifystr;
return result;
}
//https证书
private static X509TrustManager cytoX509TrustManager=new X509TrustManager(){
public X509Certificate[] getAcceptedIssuers(){
return null;
}
@Override
public void checkClientTrusted(X509Certificate[] chain, String authType) throws CertificateException {
}
@Override
public void checkServerTrusted(X509Certificate[] chain, String authType) throws CertificateException {
}
};
}
JAVA区分验证码颜色
最新推荐文章于 2024-08-02 03:59:26 发布
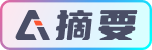