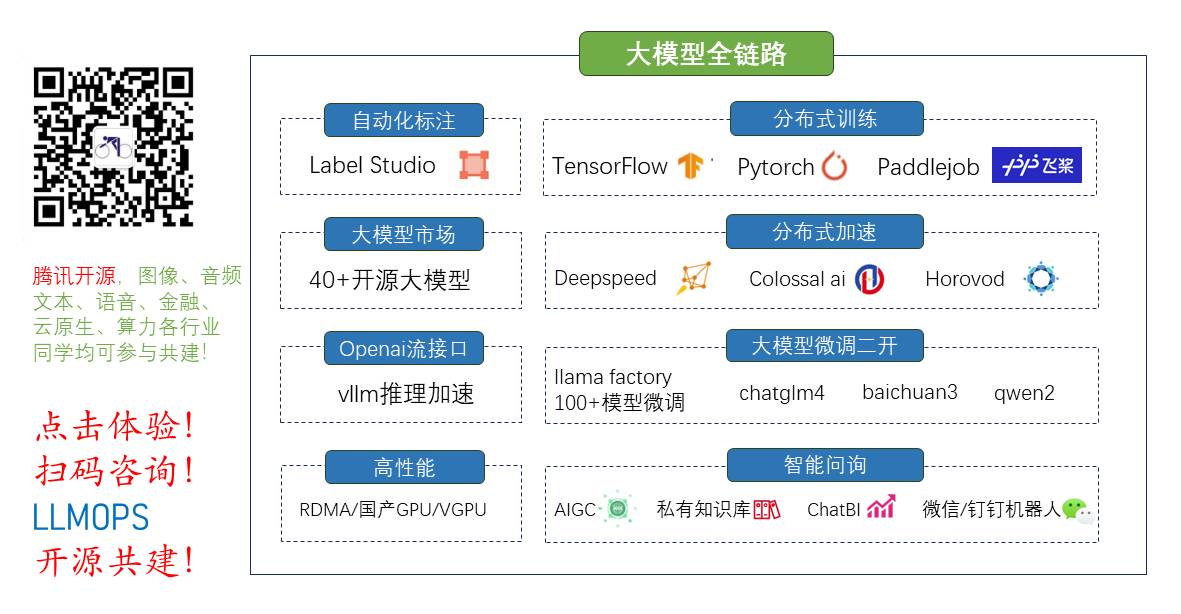
全栈工程师开发手册 (作者:栾鹏)
java教程全解
java使用tar算法压缩解压缩文件、数据流、byte[]字节数组
需要添加org.apache.commons.compress包,下载
测试代码
public static void main(String[] args) {
try {
String inputStr="111111111111111111111111111111111";
//压缩文件测试
byte[] contentOfEntry = inputStr.getBytes();
FileOutputStream fos = new FileOutputStream("test.txt");
fos.write(contentOfEntry);
fos.flush();
fos.close();
//压缩文件
TarUtils.archive("test.txt");
//解压缩文件
TarUtils.dearchive("test.txt" + ".tar");
File file = new File("test.txt");
FileInputStream fis = new FileInputStream(file);
DataInputStream dis = new DataInputStream(fis);
byte[] data = new byte[(int) file.length()];
dis.readFully(data);
fis.close();
String outputStr = new String(data);
System.out.println(outputStr);
//测试压缩目录
String path = "d:/srcdir";
TarUtils.archive(path);
TarUtils.dearchive(path + ".tar", "d:/desdir");
} catch (Exception e) {
e.printStackTrace();
}
}
tar压缩解压缩工具类的实现
package com.lp.app.io;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.DataInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import org.apache.commons.compress.archivers.tar.TarArchiveEntry;
import org.apache.commons.compress.archivers.tar.TarArchiveOutputStream;
import org.apache.commons.compress.archivers.tar.TarArchiveInputStream;
//TAR工具
public class TarUtils {
private static final String BASE_DIR = "";
// 符号"/"用来作为目录标识判断符
private static final String PATH = "/";
//归档
public static void archive(String srcPath, String destPath) throws Exception {
File srcFile = new File(srcPath);
archive(srcFile, destPath);
}
//归档
public static void archive(File srcFile, File destFile) throws Exception {
TarArchiveOutputStream taos = new TarArchiveOutputStream(new FileOutputStream(destFile));
archive(srcFile, taos, BASE_DIR);
taos.flush();
taos.close();
}
//归档
public static void archive(File srcFile) throws Exception {
String name = srcFile.getName();
String basePath = srcFile.getParent();
String destPath = basePath +"\\"+ name + ".tar";
if (basePath==null) {
destPath = name + ".tar";
}
archive(srcFile, destPath);
}
//归档文件
public static void archive(File srcFile, String destPath) throws Exception {
archive(srcFile, new File(destPath));
}
//归档
public static void archive(String srcPath) throws Exception {
File srcFile = new File(srcPath);
archive(srcFile);
}
//归档
private static void archive(File srcFile, TarArchiveOutputStream taos, String basePath) throws Exception {
if (srcFile.isDirectory()) {
archiveDir(srcFile, taos, basePath);
} else {
archiveFile(srcFile, taos, basePath);
}
}
//目录归档
private static void archiveDir(File dir, TarArchiveOutputStream taos, String basePath) throws Exception {
File[] files = dir.listFiles();
if (files.length < 1) {
TarArchiveEntry entry = new TarArchiveEntry(basePath+ dir.getName() + PATH);
taos.putArchiveEntry(entry);
taos.closeArchiveEntry();
}
for (File file : files) {
// 递归归档
archive(file, taos, basePath + dir.getName() + PATH);
}
}
//数据归档
private static void archiveFile(File file, TarArchiveOutputStream taos, String dir) throws Exception {
/**
* 归档内文件名定义
*
* <pre>
* 如果有多级目录,那么这里就需要给出包含目录的文件名
* 如果用WinRAR打开归档包,中文名将显示为乱码
* </pre>
*/
TarArchiveEntry entry = new TarArchiveEntry(dir + file.getName());
entry.setSize(file.length());
taos.putArchiveEntry(entry);
BufferedInputStream bis = new BufferedInputStream(new FileInputStream(file));
int count;
byte data[] = new byte[1024];
while ((count = bis.read(data, 0, 1024)) != -1) {
taos.write(data, 0, count);
}
bis.close();
taos.closeArchiveEntry();
}
//解归档
public static void dearchive(File srcFile) throws Exception {
String basePath = srcFile.getParent();
if (basePath==null) {
basePath="";
}
dearchive(srcFile, basePath);
}
//解归档
public static void dearchive(File srcFile, File destFile) throws Exception {
TarArchiveInputStream tais = new TarArchiveInputStream(new FileInputStream(srcFile));
dearchive(destFile, tais);
tais.close();
}
//解归档
public static void dearchive(File srcFile, String destPath)throws Exception {
dearchive(srcFile, new File(destPath));
}
//文件 解归档
private static void dearchive(File destFile, TarArchiveInputStream tais) throws Exception {
TarArchiveEntry entry = null;
while ((entry = tais.getNextTarEntry()) != null) {
// 文件
String dir = destFile.getPath() + File.separator + entry.getName();
File dirFile = new File(dir);
// 文件检查
fileProber(dirFile);
if (entry.isDirectory()) {
dirFile.mkdirs();
} else {
dearchiveFile(dirFile, tais);
}
}
}
//文件 解归档
public static void dearchive(String srcPath) throws Exception {
File srcFile = new File(srcPath);
dearchive(srcFile);
}
//文件 解归档
public static void dearchive(String srcPath, String destPath) throws Exception {
File srcFile = new File(srcPath);
dearchive(srcFile, destPath);
}
//文件解归档
private static void dearchiveFile(File destFile, TarArchiveInputStream tais) throws Exception {
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(destFile));
int count;
byte data[] = new byte[1024];
while ((count = tais.read(data, 0, 1024)) != -1) {
bos.write(data, 0, count);
}
bos.close();
}
//文件探针 ,当父目录不存在时,创建目录!
private static void fileProber(File dirFile) {
File parentFile = dirFile.getParentFile();
if (!parentFile.exists()) {
// 递归寻找上级目录
fileProber(parentFile);
parentFile.mkdir();
}
}
}