Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 35757 | Accepted: 12022 |
Description
A tree is a well-known data structure that is either empty (null, void, nothing) or is a set of one or more nodes connected by directed edges between nodes satisfying the following properties.
There is exactly one node, called the root, to which no directed edges point.
Every node except the root has exactly one edge pointing to it.
There is a unique sequence of directed edges from the root to each node.
For example, consider the illustrations below, in which nodes are represented by circles and edges are represented by lines with arrowheads. The first two of these are trees, but the last is not.
In this problem you will be given several descriptions of collections of nodes connected by directed edges. For each of these you are to determine if the collection satisfies the definition of a tree or not.
There is exactly one node, called the root, to which no directed edges point.
Every node except the root has exactly one edge pointing to it.
There is a unique sequence of directed edges from the root to each node.
For example, consider the illustrations below, in which nodes are represented by circles and edges are represented by lines with arrowheads. The first two of these are trees, but the last is not.
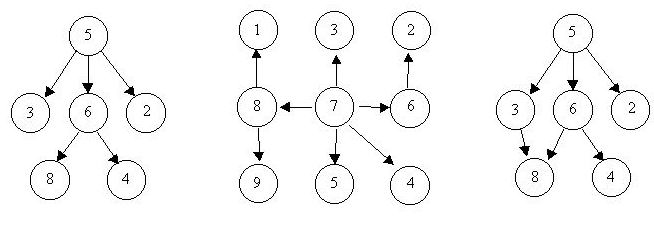
In this problem you will be given several descriptions of collections of nodes connected by directed edges. For each of these you are to determine if the collection satisfies the definition of a tree or not.
Input
The input will consist of a sequence of descriptions (test cases) followed by a pair of negative integers. Each test case will consist of a sequence of edge descriptions followed by a pair of zeroes Each edge description will consist of a pair of integers; the first integer identifies the node from which the edge begins, and the second integer identifies the node to which the edge is directed. Node numbers will always be greater than zero.
Output
For each test case display the line "Case k is a tree." or the line "Case k is not a tree.", where k corresponds to the test case number (they are sequentially numbered starting with 1).
Sample Input
6 8 5 3 5 2 6 4 5 6 0 0 8 1 7 3 6 2 8 9 7 5 7 4 7 8 7 6 0 0 3 8 6 8 6 4 5 3 5 6 5 2 0 0 -1 -1
Sample Output
Case 1 is a tree. Case 2 is a tree. Case 3 is not a tree.
Source
Regionals 1997 >> North America - North Central NA
问题链接:UVALive5461 UVA615 POJ1308 HDU1325 ZOJ1268 Is It A Tree?。
问题简述:
若干组测试用例,最后两个-1(-1 -1)结束。每个测试用例包括若干组边(两个整数组成),最后两个0(0 0)结束。判定每个测试用例是否为一棵树。
问题分析:
判定有向图是否为一棵树的问题。可以用那些边构造一个并查集,构建并查集时,如果有向边的两个结点的根相同则不是一棵树,同时所有的结点指向的根应该是相同的。
注意点:结点虽然用整数表示,然而是随意的,而且范围不定。
程序说明:
程序中,假定最大的结点不超过100000。将所有结点放入集合中,判定结点的根是否相同时,只需要考虑集合中的元素。
代码不够简洁,又写了一个简洁版。
参考链接:HDU1325 Is It A Tree?。
AC的C++语言程序(简洁版)如下:
/* UVALive5461 UVA615 POJ1308 ZOJ1268 Is It A Tree? */
#include <iostream>
#include <stdio.h>
using namespace std;
const int N = 100000;
int f[N + 1], cnt;
bool visited[N + 1];
bool nocircleflag; // 环标记
int edgecount; // 边计数
void UFInit(int n)
{
for(int i = 1; i <=n; i++) {
f[i] = i;
visited[i] = false;
}
cnt = n;
nocircleflag = true;
edgecount = 0;
}
int Find(int a) {
return a == f[a] ? a : f[a] = Find(f[a]);
}
void Union(int a, int b)
{
edgecount++;
visited[a] = true;
visited[b] = true;
a = Find(a);
b = Find(b);
if (a != b) {
f[a] = b;
cnt--;
} else
nocircleflag = false;
}
bool isconnect() {
int cnt = 0;
for( int i=1 ; i<=N ; i++ )
if(visited[i])
cnt++;
return (cnt == edgecount + 1);
}
// 唯一树根判定连通性
bool isconnect() {
int root = -1;
for( int i=1 ; i<=N ; i++ )
if(visited[i]) {
if(root == -1)
root = Find(i);
else
if(Find(i) != root)
return false;
}
return true;
}
int main()
{
int src, dest, caseno=0;
while(scanf("%d%d", &src, &dest) != EOF && (src != -1 || dest != -1)) {
if(src==0 && dest==0) {
//为空树
printf("Case %d is a tree.\n", ++caseno);
} else {
UFInit(N);
Union(src, dest);
for(;;) {
scanf("%d%d", &src, &dest);
if( src==0 && dest==0 )
break;
Union(src, dest);
}
if(nocircleflag && isconnect())
printf("Case %d is a tree.\n", ++caseno);
else
printf("Case %d is not a tree.\n", ++caseno);
}
}
return 0;
}
AC的C++语言程序如下:
/* UVALive5461 UVA615 POJ1308 ZOJ1268 Is It A Tree? */
#include <iostream>
#include <cstdio>
using namespace std;
const int MAXN = 100000;
// 并查集
class UF {
private:
int v[MAXN+1];
bool visited[MAXN+1];
int length;
bool nocircleflag; // 环标记
int edgecount; // 边计数
public:
UF(int n) {
length = n;
}
// 压缩
int Find(int x) {
if(x == v[x])
return x;
else
return v[x] = Find(v[x]);
}
bool Union(int x, int y) {
edgecount++;
visited[x] = true;
visited[y] = true;
x = Find(x);
y = Find(y);
if(x == y) {
nocircleflag = false;
return false;
} else {
v[x] = y;
return true;
}
}
bool isconnect() {
int rootcount = 0;
for( int i=0 ; i<=MAXN ; i++ )
if(visited[i])
rootcount++;
return (rootcount == edgecount + 1);
}
inline bool nocircle() {
return nocircleflag;
}
void init() {
nocircleflag = true;
edgecount = 0;
for(int i=0; i<=length; i++)
v[i] = i, visited[i] = false;
}
};
int main()
{
int src, dest, caseno=0;
UF uf(MAXN);
while(scanf("%d%d", &src, &dest) != EOF) {
if(src == -1 && dest == -1)
break;
uf.init();
if(src==0 && dest==0) {
//为空树
printf("Case %d is a tree.\n", ++caseno);
} else {
uf.Union(src, dest);
for(;;) {
scanf("%d%d", &src, &dest);
if( src==0 && dest==0 )
break;
uf.Union(src, dest);
}
if(uf.nocircle() && uf.isconnect())
printf("Case %d is a tree.\n", ++caseno);
else
printf("Case %d is not a tree.\n", ++caseno);
}
}
return 0;
}
AC的C++语言程序如下(HDU1325未AC,其他三个都AC,错误信息是ACCESS_VIOLATION,有点不明白):
/* UVALive5461 UVA615 POJ1308 HDU1325 Is It A Tree? */
#include <iostream>
#include <vector>
#include <set>
using namespace std;
const int MAXN = 100000;
// 并查集类
class UF {
private:
vector<int> v;
public:
UF(int n) {
for(int i=0; i<=n; i++)
v.push_back(i);
}
int Find(int x) {
for(;;) {
if(v[x] != x)
x = v[x];
else
return x;
}
}
bool Union(int x, int y) {
x = Find(x);
y = Find(y);
if(x == y)
return false;
else {
v[x] = y;
return true;
}
}
};
int main()
{
int src, dest, casecount=0, root;
bool ans;
for(;;) {
UF uf(MAXN);
set<int> is;
ans = true;
while(cin >> src >> dest) {
if(src == -1 && dest == -1)
return 0;
if(src == 0 && dest == 0) {
if(ans) {
root = -1;
for(set<int>::iterator iter=is.begin(); iter!=is.end(); iter++) {
if(root == -1)
root = uf.Find(*iter);
else if(uf.Find(*iter) != root) {
ans = false;
break;
}
}
}
cout << "Case " << ++casecount << (ans ? " is a tree." : " is not a tree.") << endl;
break;
} else if(ans) {
if(uf.Union(src, dest)) {
is.insert(src);
is.insert(dest);
} else
ans = false;
}
}
}
return 0;
}
改进后AC的C++语言程序如下(HDU1325出现Time Limit Exceeded,可能与集合类set有关,其他三个都AC):
/* UVALive5461 UVA615 POJ1308 HDU1325 Is It A Tree? */
#include <iostream>
#include <vector>
#include <set>
#include <cstdio>
using namespace std;
const int MAXN = 100000;
// 并查集
int v[MAXN+1];
class UF {
private:
int length;
public:
UF(int n) {
length = n;
for(int i=0; i<=n; i++)
v[i] = i;
}
// 压缩
int Find(int x) {
if(x == v[x])
return x;
else
return v[x] = Find(v[x]);
}
bool Union(int x, int y) {
x = Find(x);
y = Find(y);
if(x == y)
return false;
else {
v[x] = y;
return true;
}
}
void reset() {
for(int i=0; i<=length; i++)
v[i] = i;
}
};
int main()
{
int src, dest, casecount=0, root;
bool ans;
UF uf(MAXN);
set<int> is;
for(;;) {
ans = true;
// while(cin >> src >> dest) {
while(scanf("%d%d", &src, &dest) != EOF) {
if(src == -1 && dest == -1)
return 0;
if(src == 0 && dest == 0) {
if(ans) {
root = -1;
for(set<int>::iterator iter=is.begin(); iter!=is.end(); iter++) {
if(root == -1)
root = uf.Find(*iter);
else if(uf.Find(*iter) != root) {
ans = false;
break;
}
}
}
// cout << "Case " << ++casecount << (ans ? " is a tree." : " is not a tree.") << endl;
if(ans)
printf("Case %d is a tree.\n", ++casecount);
else
printf("Case %d is not a tree.\n", ++casecount);
break;
} else if(ans) {
if(uf.Union(src, dest)) {
is.insert(src);
is.insert(dest);
} else
ans = false;
}
}
is.clear();
uf.reset();
}
return 0;
}