Given N axis-aligned rectangles where N > 0, determine if they all together form an exact cover of a rectangular region.
Each rectangle is represented as a bottom-left point and a top-right point. For example, a unit square is represented as [1,1,2,2]. (coordinate of bottom-left point is (1, 1) and top-right point is (2, 2)).
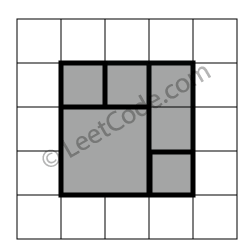
Example 1:
rectangles = [ [1,1,3,3], [3,1,4,2], [3,2,4,4], [1,3,2,4], [2,3,3,4] ] Return true. All 5 rectangles together form an exact cover of a rectangular region.
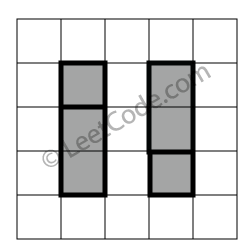
Example 2:
rectangles = [ [1,1,2,3], [1,3,2,4], [3,1,4,2], [3,2,4,4] ] Return false. Because there is a gap between the two rectangular regions.

Example 3:
rectangles = [ [1,1,3,3], [3,1,4,2], [1,3,2,4], [3,2,4,4] ] Return false. Because there is a gap in the top center.

Example 4:
rectangles = [ [1,1,3,3], [3,1,4,2], [1,3,2,4], [2,2,4,4] ] Return false. Because two of the rectangles overlap with each other.
Idea1:面积和相等,矩形两两不相交,O(N^2)
Idea2:面积和相等,所有矩形的四个角(除了最后合并成的大矩形的四个角之外),都应该出现偶数次,O(N)
摘自
https://discuss.leetcode.com/topic/56052/really-easy-understanding-solution-o-n-java
The right answer must satisfy two conditions:
- the large rectangle area should be equal to the sum of small rectangles
- count of all the points should be even, and that of all the four corner points should be one
public class Solution {
public static boolean isRectangleCover(int[][] rectangles)
{
int len=rectangles.length;
if(len<2)
return true;
int left=Integer.MAX_VALUE,buttom=Integer.MAX_VALUE,right=Integer.MIN_VALUE,top=Integer.MIN_VALUE;
int sumArea=0;
HashSet<String> hashset=new HashSet<>();
for(int i=0;i<len;i++)
{
if(rectangles[i][0]<left||rectangles[i][0]==left&&rectangles[i][1]<buttom)
{
left=rectangles[i][0];
buttom=rectangles[i][1];
}
if(rectangles[i][2]>right||rectangles[i][2]==right&&rectangles[i][3]>top)
{
right=rectangles[i][2];
top=rectangles[i][3];
}
sumArea+=(rectangles[i][2]-rectangles[i][0])*(rectangles[i][3]-rectangles[i][1]);
String[] strs={rectangles[i][0]+" "+rectangles[i][1],rectangles[i][0]+" "+rectangles[i][3],rectangles[i][2]+" "+rectangles[i][1],rectangles[i][2]+" "+rectangles[i][3]};
for(String s :strs)
if(hashset.contains(s))
hashset.remove(s);
else {
hashset.add(s);
}
}
if(sumArea!=(right-left)*(top-buttom))
return false;
if(!hashset.contains(left+" "+buttom)||!hashset.contains(left+" "+top)||!hashset.contains(right+" "+buttom)||!hashset.contains(right+" "+top)||hashset.size()!=4)
return false;
return true;
}
}