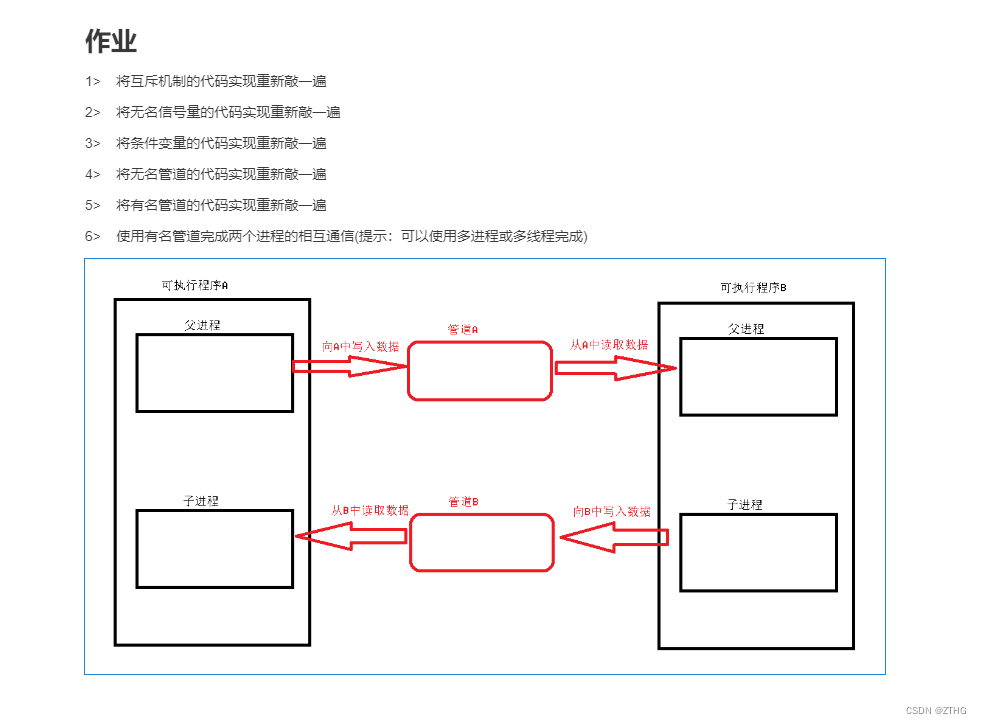
1.互斥机制
代码:
#include<myhead.h>
int num = 256;
pthread_mutex_t mutex;
void* task1(void* arg)
{
printf("111100001111\n");
pthread_mutex_lock(&mutex);
num = 555;
sleep(3);
printf("task1:num = %d\n", num);
pthread_mutex_unlock(&mutex);
}
void* task2(void* arg)
{
printf("222200002222\n");
pthread_mutex_lock(&mutex);
num++;
sleep(1);
printf("task2:num = %d\n", num);
pthread_mutex_unlock(&mutex);
}
int main(int argc, char const *argv[])
{
pthread_mutex_init(&mutex, NULL);
pthread_t thread1, thread2;
if (pthread_create(&thread1, NULL, task1, NULL) != 0 || \
pthread_create(&thread2, NULL, task2, NULL) != 0)
{
perror("thread create error");
return -1;
}
printf("thread1:%#lx, thread2:%#lx\n", thread1, thread2);
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
pthread_mutex_destroy(&mutex);
return 0;
}
结果:
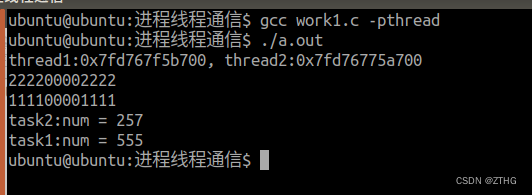
2.无名信号量
代码:
#include <myhead.h>
//定义无名信号量
sem_t sem;
//生产者线程
void *task1(void *arg)
{
int num=5;
while(num--)
{
//申请资源
//sem_wait(&sem);
sleep(1);
printf("生产商品成功\n");
//释放资源
sem_post(&sem);
}
pthread_exit(NULL);
}
//消费者线程
void *task2(void *arg)
{
int num=5;
while(num--)
{
//申请资源
sem_wait(&sem);
printf("消费金钱成功\n");
//释放资源
//sem_post(&sem);
}
pthread_exit(NULL);
}
int main(int argc, const char *argv[])
{
//初始化无名信号量
sem_init(&sem,0,0);
//创建线程(生产者+消费者)
pthread_t tid1,tid2;
if(pthread_create(&tid1,NULL,task1,NULL)!=0)
{
printf("tid1 create error");
return 0;
}
if(pthread_create(&tid2,NULL,task2,NULL)!=0)
{
printf("tid2 create error");
return 0;
}
//回收资源
pthread_join(tid1,NULL);
pthread_join(tid2,NULL);
//释放无名信号量
sem_destroy(&sem);
return 0;
}
结果:
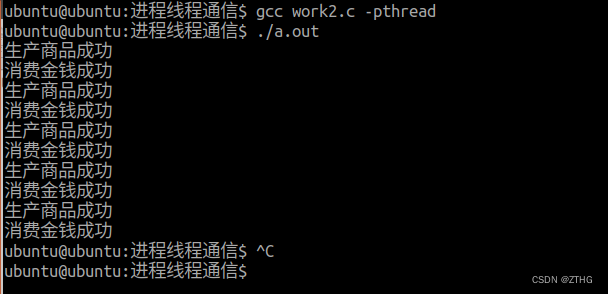
3.条件变量
代码:
#include <myhead.h>
//定义条件变量
pthread_cond_t cond;
//定义互斥锁变量
pthread_mutex_t mutex;
//生产者线程
void *task1(void *arg)
{
int num=5;
while(num--)
{
sleep(1);
printf("%#lx:生产商品成功\n",pthread_self());
//唤醒队列第一个
pthread_cond_signal(&cond);
}
pthread_exit(NULL);
}
//消费者线程
void *task2(void *arg)
{
//上锁
pthread_mutex_lock(&mutex);
//进入等待队列
pthread_cond_wait(&cond,&mutex);
printf("%#lx:消费金钱成功\n",pthread_self());
//解锁
pthread_mutex_unlock(&mutex);
//退出线程
pthread_exit(NULL);
}
int main(int argc, const char *argv[])
{
//初始化条件变量
pthread_mutex_init(&mutex,NULL);
//初始化互斥锁
pthread_mutex_init(&mutex,NULL);
//创建线程×5
pthread_t tid1,tid2,tid3,tid4,tid5,tid6;
if(pthread_create(&tid1,NULL,task1,NULL)!=0)
{
printf("tid1 create error");
return 0;
}
if(pthread_create(&tid2,NULL,task2,NULL)!=0)
{
printf("tid2 create error");
return 0;
}
if(pthread_create(&tid3,NULL,task2,NULL)!=0)
{
printf("tid1 create error");
return 0;
}
if(pthread_create(&tid4,NULL,task2,NULL)!=0)
{
printf("tid1 create error");
return 0;
}
if(pthread_create(&tid5,NULL,task2,NULL)!=0)
{
printf("tid1 create error");
return 0;
}
if(pthread_create(&tid6,NULL,task2,NULL)!=0)
{
printf("tid1 create error");
return 0;
}
//回收资源
pthread_join(tid1,NULL);
pthread_join(tid2,NULL);
pthread_join(tid3,NULL);
pthread_join(tid4,NULL);
pthread_join(tid5,NULL);
pthread_join(tid6,NULL);
//销毁条件变量
pthread_cond_destroy(&cond);
//销毁锁资源
pthread_mutex_destroy(&mutex);
return 0;
}
结果:
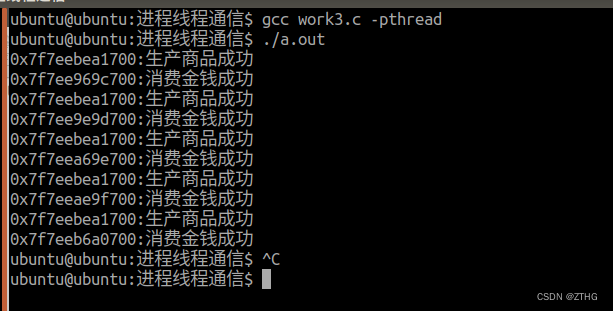
4.无名管道
代码:
#include <myhead.h>
int main(int argc, const char *argv[])
{
//创建管道文件,返回文件描述符
int pipefd[2]={0};
if(pipe(pipefd)==-1)
{
perror("pipe error");
return -1;
}
printf("pipefd[0]=%d,pipefd[1]=%d\n",\
pipefd[0],pipefd[1]);
pid_t pid=fork();
if(pid>0)
{
//父进程
//关闭读端
close(pipefd[0]);
char wbuf[128]="";
while(1)
{
bzero(wbuf,sizeof(wbuf));//清空数组
fgets(wbuf,sizeof(wbuf),stdin);//终端输入
wbuf[strlen(wbuf)-1]=0;
//向管道文件写入数据
write(pipefd[1],wbuf,strlen(wbuf));
if(strcmp(wbuf,"quit")==0)
{
break;
}
}
close(pipefd[1]);
wait(NULL);
}
else if(pid==0)
{
//子进程
//
close(pipefd[1]);
char rbuf[128]="";
while(1)
{
//清空rbuf
bzero(rbuf,sizeof(rbuf));
//从管道文件读取文件
read(pipefd[0],rbuf,sizeof(rbuf));
//输出rbuf数据
printf("父进程数据为:%s\n",rbuf);
//判断读取数据
if(strcmp(rbuf,"quit")==0)
{
break;
}
}
close(pipefd[0]);
exit(EXIT_SUCCESS);
}
else
{
perror("fprk error");
return -1;
}
return 0;
}
结果:
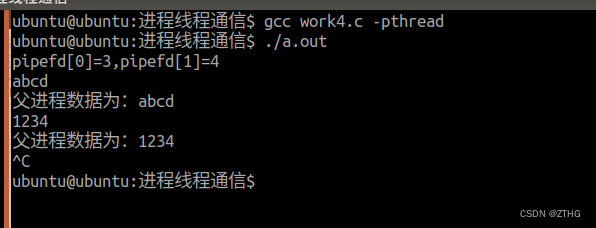
5.有名管道
代码:
create.c
#include<myhead.h>
int main(int argc, const char *argv[])
{
//创建一个管道文件
if(mkfifo("./myfifo",0664)==-1)
{
perror("mkfifo error");
return -1;
}
getchar();//阻塞
system("rm myfifo");
return 0;
}
snd.c
#include<myhead.h>
int main(int argc, const char *argv[])
{
//打开管道文件
int wfd = -1;
//以只写的形式打开文件
if((wfd = open("./myfifo", O_WRONLY)) == -1)
{
perror("open error");
return -1;
}
//定义容器
char wbuf[128] = "";
while(1)
{
printf("请输入>>>");
fgets(wbuf, sizeof(wbuf), stdin);
wbuf[strlen(wbuf)-1] = 0;
//将数据写入管道
write(wfd, wbuf, strlen(wbuf));
//判断结果
if(strcmp(wbuf,"quit") == 0)
{
break;
}
}
//关闭文件
close(wfd);
return 0;
}
rec.c
#include<myhead.h>
int main(int argc, const char *argv[])
{
//打开管道文件
int rfd = -1;
//以只写读的形式打开文件
if((rfd = open("./myfifo", O_RDONLY)) == -1)
{
perror("open error");
return -1;
}
//定义容器
char rbuf[128] = "";
while(1)
{
//清空数组
bzero(rbuf, sizeof(rbuf));
//读取管道中的数据
read(rfd, rbuf, sizeof(rbuf));
//输出结果
printf("收到的数据为:%s\n", rbuf);
//判断结果
if(strcmp(rbuf,"quit") == 0)
{
break;
}
}
//关闭文件
close(rfd);
return 0;
}
结果:
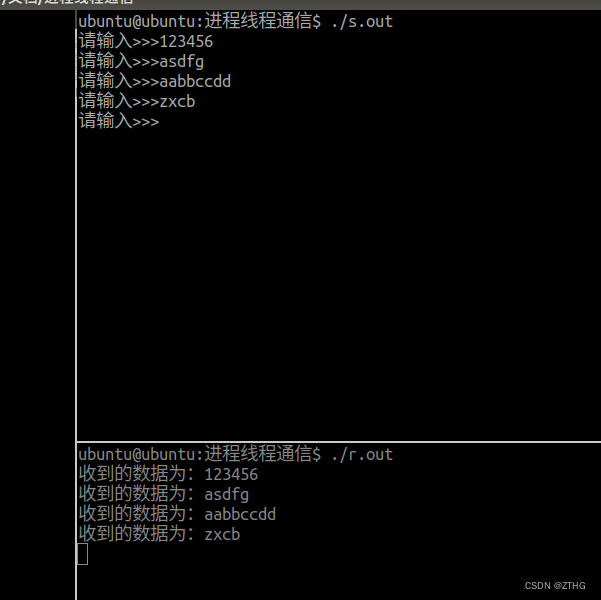
6.使用有名管道完成两个进程的相互通信
代码:
create1.c
#include<myhead.h>
int main(int argc, const char *argv[])
{
//创建有名管道文件1
if(mkfifo("./myfifo1",0664) !=0 )
{
perror("myfifo1 error");
return -1;
}
//创建有名管道文件2
if(mkfifo("./myfifo2",0664) !=0 )
{
perror("myfifo2 error");
return -1;
}
printf("文件创建成功\n");
getchar();//阻塞
system("rm myfifo1 myfifo2");
return 0;
}
snd1.c
#include<myhead.h>
int main(int argc, const char *argv[])
{
pid_t pid;
pid=fork();
if(pid>0)
{
//以只读的形式打开管道文件1
int rfd = -1;
if((rfd = open("./myfifo1", O_RDONLY)) == -1)
{
perror("open error");
return -1;
}
//向管道文件1中循环写入数据
char rbuf[128]="";
while(1)
{
//清空内容
bzero(rbuf, sizeof(rbuf));
//从管道1中读取数据
read(rfd, rbuf, sizeof(rbuf));
printf("收到消息:%s\n",rbuf);
//判断结束
if(strcmp(rbuf,"quit") == 0)
break;
}
}
else if(pid==0)
{
//以只写的形式打开管道文件2
int wfd = -1;
if((wfd = open("./myfifo2", O_WRONLY)) == -1)
{
perror("open error");
return -1;
}
//向管道文件2中循环写入数据
char wbuf[128]="";
while(1)
{
//清空内容
bzero(wbuf, sizeof(wbuf));
//从终端输入数据
printf("请输入:\n");
//刷新缓存区
fflush(stdout);
//从终端读取数据
read(0, wbuf, sizeof(wbuf));
wbuf[strlen(wbuf)-1] = '\0';
write(wfd, wbuf, sizeof(wbuf));
if(strcmp(wbuf,"quit") == 0)
break;
}
exit(EXIT_SUCCESS);
}
wait(NULL);
return 0;
}
rec1.c
#include<myhead.h>
int main(int argc, const char *argv[])
{
pid_t pid;
pid=fork();
if(pid>0)
{
//以只写的形式打开管道文件1
int wfd = -1;
if((wfd = open("./myfifo1", O_WRONLY)) == -1)
{
perror("open error");
return -1;
}
//向管道文件1中循环写入数据
char wbuf[128]="";
while(1)
{
//清空内容
bzero(wbuf, sizeof(wbuf));
//从终端输入数据
printf("请输入:\n");
//刷新缓存区
fflush(stdout);
//从终端读取数据
read(0, wbuf, sizeof(wbuf));
wbuf[strlen(wbuf)-1] = '\0';
write(wfd, wbuf, sizeof(wbuf));
if(strcmp(wbuf,"quit") == 0)
break;
}
}
else if(pid==0)
{
//以只读的形式打开管道文件2
int rfd = -1;
if((rfd = open("./myfifo2", O_RDONLY)) == -1)
{
perror("open error");
return -1;
}
//向管道文件2中循环写入数据
char rbuf[128]="";
while(1)
{
//清空内容
bzero(rbuf, sizeof(rbuf));
//从管道2中读取数据
read(rfd, rbuf, sizeof(rbuf));
printf("收到消息:%s\n",rbuf);
//判断结束
if(strcmp(rbuf,"quit") == 0)
break;
}
exit(EXIT_SUCCESS);
}
wait(NULL);
return 0;
}
结果:
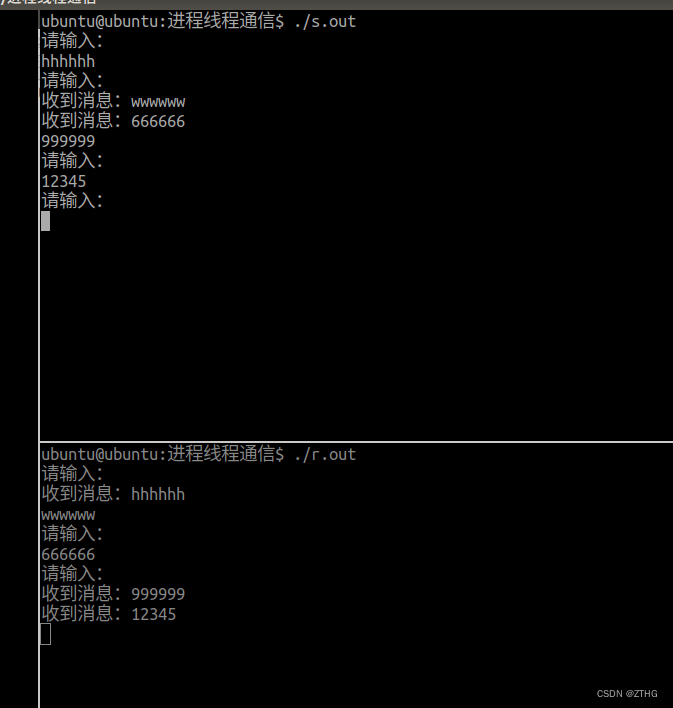