复习题
判断下列表达式是 true还是 false
a.100 > 3 && 'a'>'c'
b.100 > 3 || 'a'>'c'
c.!(100>3)
答: a. false b.ture c.false
2.根据下列描述的条件,分别构造一个表达式:
a.number 等于或大于90 ,但是小于100
b.ch 不是字符q 或k
c.number 在1 ~9 之间(包括1 和9 ),但不是5
d.number 不在1 ~9 之间
答:
//a
number >= 90 && number < 100
//b
ch != 'q' || ch != 'k'
//c
(number >= 1 && number <= 9) && number !=5
//d
number < 1 || number > 9
3.下面的程序关系表达式过于复杂,而且还有些错误,请简化并改正。
#include <stdio.h>
int main(void) /* 1 */
{ /* 2 */
int weight, height; /* weight以磅为单位,height以英寸为单位 */
/* 4 */
scanf("%d , weight, height); /* 5 */
if (weight < 100 && height > 64) /* 6 */
if (height >= 72) /* 7 */
printf("You are very tall for your weight.\n");
else if (height < 72 &&> 64) /* 9 */
printf("You are tall for your weight.\n"); /* 10 */
else if (weight > 300 && !(weight <= 300) /* 11 */
&& height < 48) /* 12 */
if (!(height >= 48)) /* 13 */
printf(" You are quite short for your weight.\n");
else /* 15 */
printf("Your weight is ideal.\n"); /* 16 */
/* 17 */
return 0;
}
答:
第5行 scanf()函数的格式书写错误
第6行 条件判断语句逻辑混乱, weitght 的判断标准为 100磅或300磅;
height的判断标准为 48 、64 或 72 inch,
故得到4种情况对应4个不同printf()
#include <stdio.h>
int main(void)
{
int weight, height; /* weight以磅为单位,height以英寸为单位 */
scanf("%d %d", &weight, &height);
if (weight < 100 && height > 64)
if (height >= 72)
/*weight < 100 且 height 大于等于 72*/
printf("You are very tall for your weight.\n");
else
/*weight < 100 , height 大于64且小于 72*/
printf("You are tall for your weight.\n");
else if (weight > 300 && height < 48)
/*weight 大于300 , height 小于48*/
printf(" You are quite short for your weight.\n");
else
/*weight 大于100且小于 300 , height 大于48小于72*/
printf("Your weight is ideal.\n");
return 0;
}
4.下列各表达式的值是多少?
a.5 > 2
b.3 + 4 > 2 && 3 < 2
c.x >= y || y > x
d.d = 5 + ( 6 > 2 )
e.'X' > 'T' ? 10 : 5
f. x > y ? y > x : x > y
答:
a | b | c | d | e | f |
1 | 0 | 1 | 6 | 10 (顺序为‘X’前, ‘Y’在后) | 0 (均为false) |
5.下面的程序将打印什么?
#include <stdio.h>
int main()
{
int num = 0;
for ( num = 1; num <= 11; num++ )
{
if (num % 3 == 0)
putchar('$');
else
putchar('*');
putchar('#');
putchar('%');
}
putchar('\n');
return 0;
}
答:
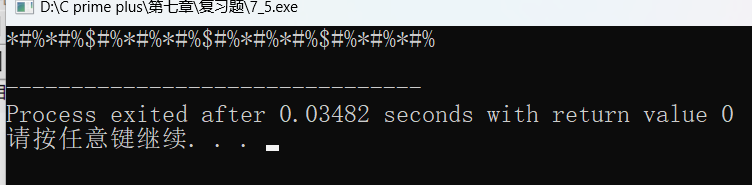
解析: num 为3 倍数时打印'$',否则打印'* '。
完成if语句后继续打印’#‘ 和 '%'
因此实际应用if-else语句要注意 范围,最好用花括号{}
6.下面的程序将打印什么?
#include <stdio.h>
int main(void)
{
int i = 0;
while (i < 3) {
switch (i++) {
case 0: printf("fat ");
case 1: printf("hat ");
case 2: printf("cat ");
default: printf("Oh no!");
}
putchar('\n');
}
return 0;
}
答:

解析: switch语句没有搭配break,
i 从 0开始取值, 执行case的匹配语句之下的所有语句
i++会先用 i的值再递增
7.下面的程序有哪些错误?
#include <stdio.h>
int main(void)
{
char ch;
int lc = 0; /* 统计小写字母
int uc = 0; /* 统计大写字母
int oc = 0; /* 统计其他字母
while ((ch = getchar()) != '#')
{
if ('a' <= ch >= 'z')
lc++;
else if (!(ch < 'A') || !(ch > 'Z')
uc++;
oc++;
}
printf(%d lowercase, %d uppercase, %d other, lc, uc, oc);
return 0;
}
答:
三种情况: 1.大写字母 2.小写字母 3.其他字符
原题目逻辑混乱,且 printf()语句语法错误;
注释符号书写错误
判断英文大小写 可以用islower() 和 isupper()函数,
但要加上头文件 #include <ctype.h>
#include <stdio.h>
int main(void)
{
char ch;
int lc = 0; /* 统计小写字母 */
int uc = 0; /* 统计大写字母 */
int oc = 0; /* 统计其他字母 */
while ((ch = getchar()) != '#')
{
if ('a' <= ch && ch <= 'z')
lc++;
else if (ch >= 'A' && ch <= 'Z')
uc++;
else
oc++;
}
printf("%d lowercase, %d uppercase, %d other", lc, uc, oc);
return 0;
}
8.下面的程序将打印什么?
/* retire.c */
#include <stdio.h>
int main(void)
{
int age = 20;
while (age++ <= 65)
{
if ((age % 20) == 0) /* age是否能被20整除? */
printf("You are %d. Here is a raise.\n", age);
if (age = 65)
printf("You are %d. Here is your gold watch.\n", age);
}
return 0;
}
答:会一直打印如下内容
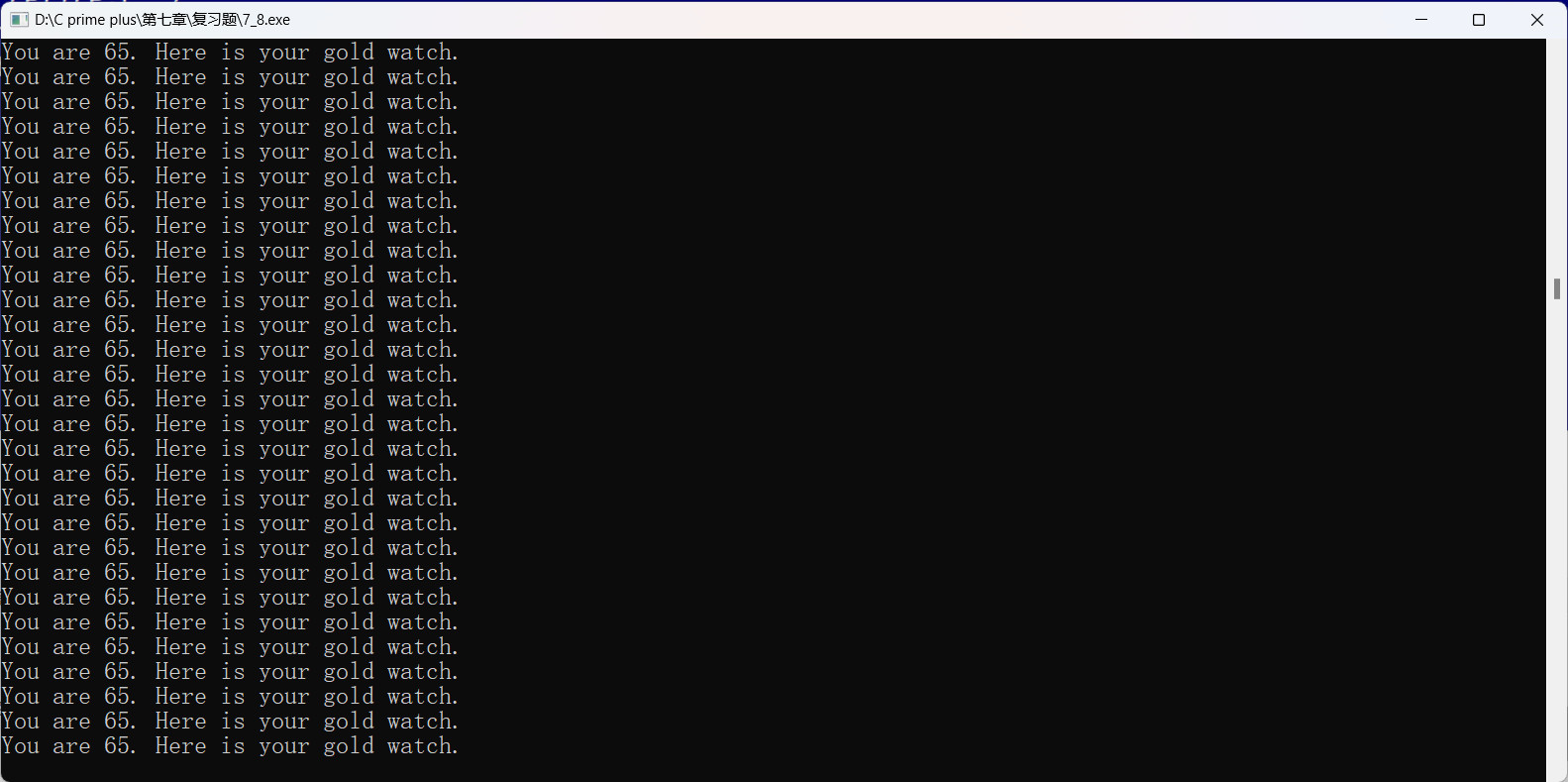
原因是: 第10行,if()语句的判断条件为 age =65, age会不断被赋值为65。
因为程序无法跳出while循环,而一直进行下去。
修改 为 age == 65便可以 正确打印如下
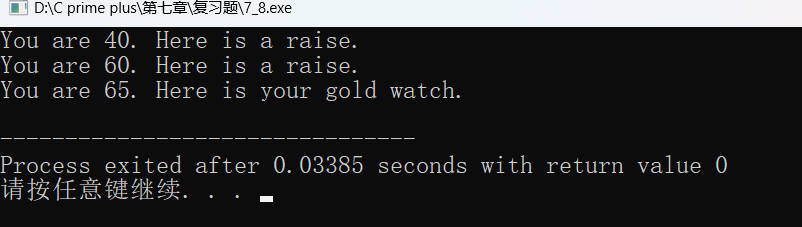
9.给定下面的输入时,以下程序将打印什么?
/*
q
c
h
b
*/
#include <stdio.h>
int main(void)
{
char ch;
while ((ch = getchar()) != '#')
{
if (ch == '\n')
continue;
printf("Step 1\n");
if (ch == 'c')
continue;
else if (ch == 'b')
break;
else if (ch == 'h')
goto laststep;
printf("Step 2\n");
laststep: printf("Step 3\n");
}
printf("Done\n");
return 0;
}
答:
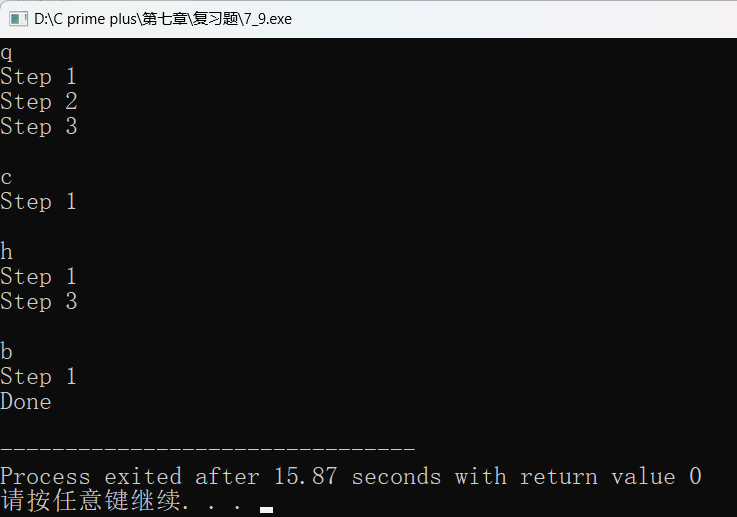
结合答案的解析 :
ch不是#进入 while循环
ch不为换行符\n, 则打印step 1;
ch 为c,结束本轮循环,进入下一轮;ch为b 跳出循环
ch为h,直接打印step 3;
其余情况为先打印step2 再打印step3
10.重写复习题9,但这次不能使用continue 和goto 语句。
答:
抓住 :
\n
‘b’
'c'
'h'
这几种情况;适当考虑其反面可不用到continue和goto;
注意 if()语句的范围,用括号{}提高可读性
// 输出依次为 q c h b
//不能使用 continue 和 goto
#include <stdio.h>
int main(void)
{
char ch;
while ((ch = getchar()) != '#' )
{
if (ch != '\n'){
printf("Step 1\n");
if (ch == 'b')
break;
if (ch != 'c'){
if ( ch == 'h'){
printf("step 3\n"); }
else{
printf("Step 2\n");
printf("Step 3\n");}
}
}
}
printf("Done\n");
return 0;