作者主页:源码空间codegym
简介:Java领域优质创作者、Java项目、学习资料、技术互助
文中获取源码
项目介绍
这是一个基于ssm框架的购物系统
- 该项目中有五个子模块。
- shop-manager模块负责导入该项目所需要的所有依赖包。
- shop-web模块负责存放前端代码以及项目的配置文件。
- shop-controller模块负责存放项目的控制器代码。
- shop-service模块负责存放项目的业务逻辑代码。
- shop-dao模块负责存放项目的mybatis数据库操作代码。
技术选型
运行环境:MySQL5.7+jdk1.8+Idea2020.3+Tomcat9
服务端技术:jsp+servlet+jdbc+jstl+el表达式
前端技术:bootstrap+jQuery+ajax
环境要求
1.运行环境:最好是java jdk1.8,我们在这个平台上运行的。其他版本理论上也可以。
2.IDE环境:IDEA,Eclipse,Myeclipse都可以。推荐IDEA;
3.tomcat环境:Tomcat7.x,8.X,9.x版本均可
4.硬件环境:windows7/8/10 4G内存以上;或者Mac OS;
5.是否Maven项目:是;查看源码目录中是否包含pom.xml;若包含,则为maven项目,否则为非maven.项目
6.数据库:MySql5.7/8.0等版本均可;
技术栈
后台框架:servlet、MyBatis
数据库:MySQL
环境:JDK8、TOMCAT、IDEA
使用说明
1.使用Navicati或者其它工具,在mysql中创建对应sq文件名称的数据库,并导入项目的sql文件;
2.使用IDEA/Eclipse/MyEclipse导入项目,修改配置,运行项目;
3.将项目中config-propertiesi配置文件中的数据库配置改为自己的配置,然后运行;
运行指导
idea导入源码空间站顶目教程说明(Vindows版)-ssm篇:
http://mtw.so/5MHvZq
源码地址:http://codegym.top
运行截图
前端界面
后台界面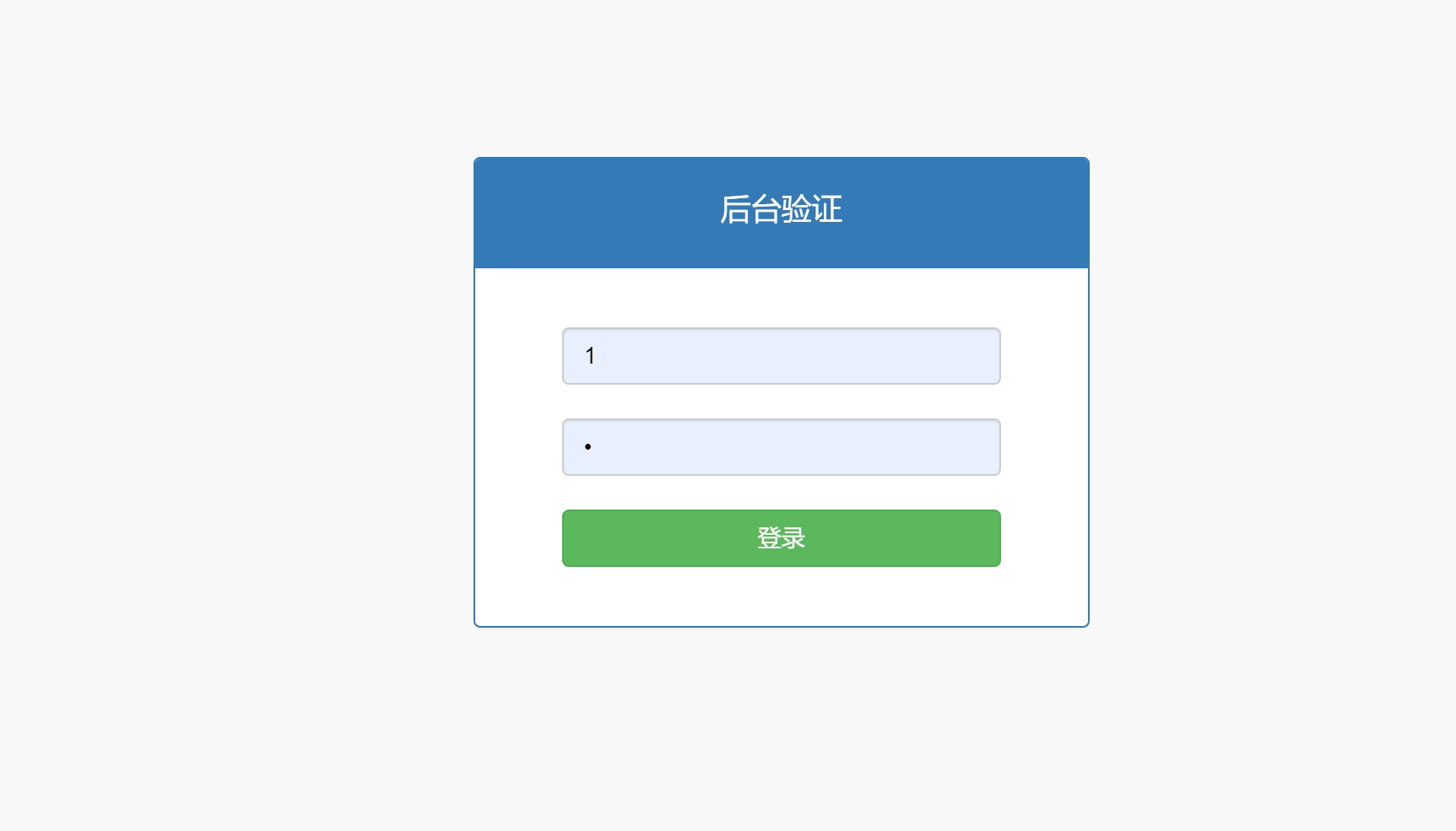
代码
BackstageController
package com.zt.controller;
import com.zt.pojo.cart;
import com.zt.pojo.custom;
import com.zt.pojo.goods;
import com.zt.pojo.order;
import com.zt.service.*;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.servlet.ModelAndView;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
@Controller
public class BackstageController {
@Resource
private AdminService adminService;
@Resource
private GoodsService goodsService;
@Resource
private OrderService orderService;
@Resource
private CustomService customService;
@Resource
private CartService cartService;
// 管理员后台界面跳转
@RequestMapping(value = "/admin")
public ModelAndView toBackstage(){
ModelAndView modelAndView = new ModelAndView();
modelAndView.setViewName("page/alogin.jsp");
return modelAndView;
}
// 处理管理员登录
@RequestMapping(value = "/alogin.action",method = RequestMethod.POST)
public void alogin(@RequestParam(value = "cname") String cname,
@RequestParam(value = "password") String password,
HttpServletResponse response){
PrintWriter out = null;
try{
out = response.getWriter();
String result = adminService.loginService(cname,password);
out.print(result);
out.flush();
}catch (IOException e){
e.printStackTrace();
}finally {}
out.close();
}
// 添加商品信息
@RequestMapping(value = "/addGoods.action",method = RequestMethod.POST)
public void addGoods(@RequestParam(value = "id") String id,
@RequestParam(value = "img") String img,
@RequestParam(value = "title") String title,
@RequestParam(value = "info") String info,
@RequestParam(value = "name") String name,
@RequestParam(value = "price") String price,
@RequestParam(value = "stock") String stock,
@RequestParam(value = "para") String para,
@RequestParam(value = "type") String type,
@RequestParam(value = "weight") String weight,
HttpServletResponse response){
PrintWriter out = null;
try{
out = response.getWriter();
goods goods = new goods(id,img,title,info,name,new Integer(price),new Integer(stock),para,type,new Integer(weight));
goodsService.addGoodsService(goods);
out.print("success");
out.flush();
}catch (IOException e){
e.printStackTrace();
}finally {}
out.close();
}
// 添加订单信息
@RequestMapping(value = "/addOrder.action",method = RequestMethod.POST)
public void addOrder(@RequestParam(value = "rid") String rid,
@RequestParam(value = "cname") String cname,
@RequestParam(value = "ids") String ids,
@RequestParam(value = "names") String names,
@RequestParam(value = "price") String price,
HttpServletResponse response){
PrintWriter out = null;
try{
out = response.getWriter();
order order = new order(rid,cname,ids,names,new Integer(price));
orderService.addOrderService(order);
out.print("success");
out.flush();
}catch (IOException e){
e.printStackTrace();
}finally {}
out.close();
}
// 删除单个字段
@RequestMapping(value = "/deleteItem.action",method = RequestMethod.POST)
public void deleteItem1(@RequestParam("table") String table,
@RequestParam("id") String id,
HttpServletResponse response){
PrintWriter out = null;
try{
out = response.getWriter();
switch (table){
case "custom":{
customService.deleteCustomService(id);
break;
}
case "goods":{
goodsService.deleteGoodsService(id);
break;
}
case "cart":{
String[] s = id.split("_");
cartService.deleteCartService(s[0],s[1]);
break;
}
case "order":{
orderService.deleteOrderService(id);
break;
}
default:break;
}
out.print("success");
out.flush();
}catch (IOException e){
e.printStackTrace();
}finally {}
out.close();
}
// 修改客户信息
@RequestMapping(value = "/updateCustom2.action",method = RequestMethod.POST)
public void updateCustom2(@RequestParam(value = "cname") String cname,
@RequestParam(value = "name") String name,
@RequestParam(value = "phone") String phone,
@RequestParam(value = "address") String address,
@RequestParam(value = "password") String password,
@RequestParam(value = "question") String question,
@RequestParam(value = "answer") String answer,
HttpServletResponse response){
PrintWriter out = null;
try{
out = response.getWriter();
custom c = new custom(cname,password,name,phone,address,question,answer);
customService.updateCustomService(c);
out.print("success");
out.flush();
}catch (IOException e){
e.printStackTrace();
}finally {}
out.close();
}
// 修改商品信息
@RequestMapping(value = "/updateGoods.action",method = RequestMethod.POST)
public void updateGoods(@RequestParam(value = "id") String id,
@RequestParam(value = "img") String img,
@RequestParam(value = "title") String title,
@RequestParam(value = "info") String info,
@RequestParam(value = "name") String name,
@RequestParam(value = "price") String price,
@RequestParam(value = "stock") String stock,
@RequestParam(value = "para") String para,
@RequestParam(value = "type") String type,
@RequestParam(value = "weight") String weight,
HttpServletResponse response){
PrintWriter out = null;
try{
out = response.getWriter();
goods goods = new goods(id,img,title,info,name,new Integer(price),new Integer(stock),para,type,new Integer(weight));
goodsService.updateGoodsService(goods);
out.print("success");
out.flush();
}catch (IOException e){
e.printStackTrace();
}finally {}
out.close();
}
// 修改购物车信息
@RequestMapping(value = "/updateCart.action",method = RequestMethod.POST)
public void updateCart(@RequestParam(value = "cname") String cname,
@RequestParam(value = "id") String id,
@RequestParam(value = "img") String img,
@RequestParam(value = "name") String name,
@RequestParam(value = "price") String price,
@RequestParam(value = "number") String number,
HttpServletResponse response){
PrintWriter out = null;
try{
out = response.getWriter();
cart cart = new cart(cname,id,img,name,new Integer(price),new Integer(number));
cartService.updateCartService(cart);
out.print("success");
out.flush();
}catch (IOException e){
e.printStackTrace();
}finally {}
out.close();
}
// 修改订单信息
@RequestMapping(value = "/updateOrder.action",method = RequestMethod.POST)
public void updateCart(@RequestParam(value = "rid") String rid,
@RequestParam(value = "cname") String cname,
@RequestParam(value = "ids") String ids,
@RequestParam(value = "names") String names,
@RequestParam(value = "price") String price,
HttpServletResponse response){
PrintWriter out = null;
try{
out = response.getWriter();
order order = new order(rid,cname,ids,names,new Integer(price));
orderService.updateOrderService(order);
out.print("success");
out.flush();
}catch (IOException e){
e.printStackTrace();
}finally {}
out.close();
}
}