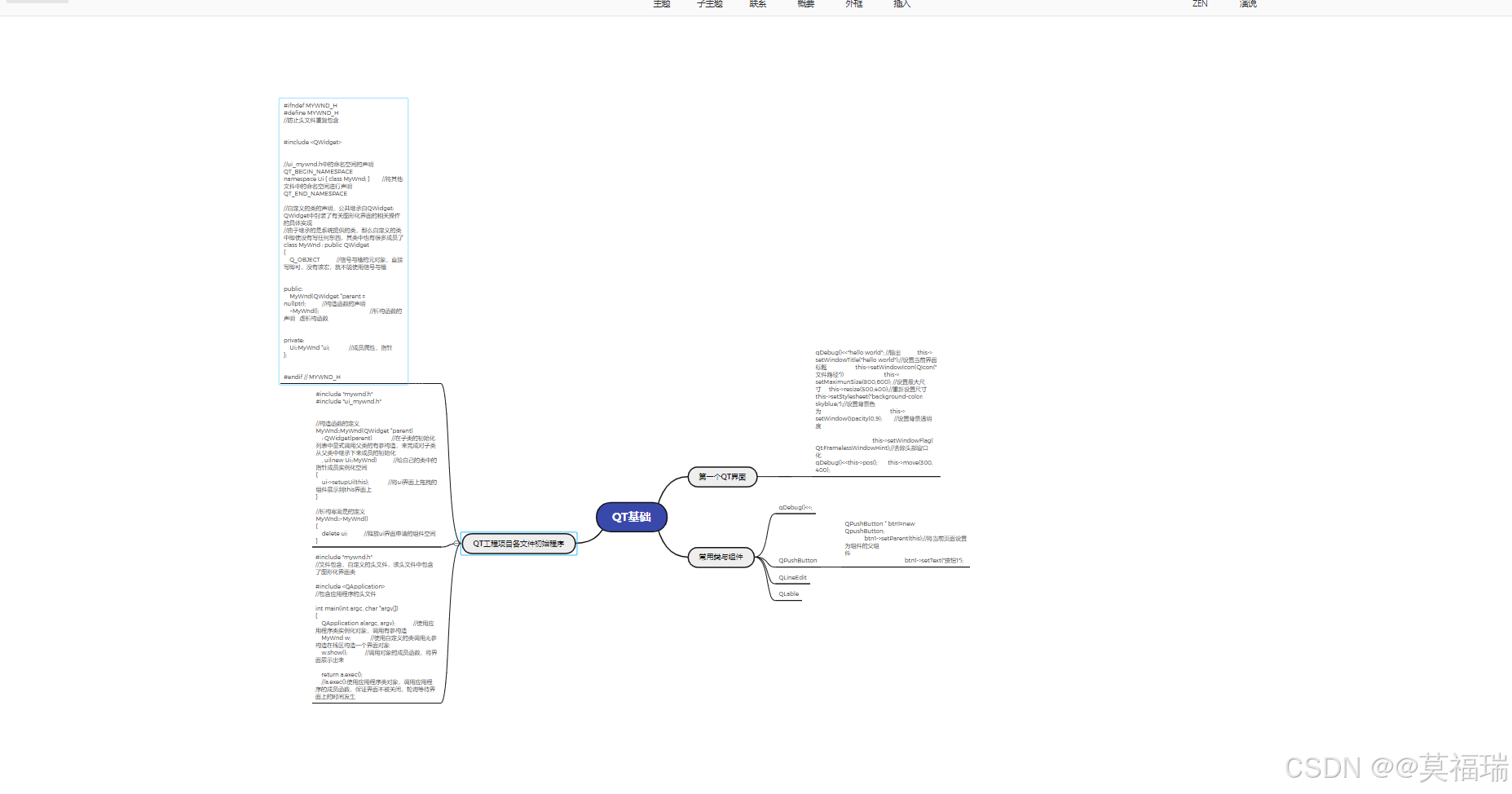
#ifndef WIDGET_H
#define WIDGET_H
//防止头文件重复包含
#include <QWidget>
#include<QIcon>
#include<QDebug>
#include<QPushButton>
#include<QLabel>
#include<QLineEdit>
//ui_mywnd.h中的命名空间的声明
QT_BEGIN_NAMESPACE
namespace Ui { class Widget; } //将其他文件中的命名空间进行声明
QT_END_NAMESPACE
//自定义的类的声明,公共继承自QWidget:QWidget中封装了有关图形化界面的相关操作的具体实现
//由于继承的是系统提供的类,那么自定义的类中即使没有写任何东西,其类中也有很多成员了
class Widget : public QWidget
{
Q_OBJECT //信号与槽的元对象,直接写即可,没有该宏,就不能使用信号与槽
public:
void do_login();
public:
Widget(QWidget *parent = nullptr); //构造函数的声明
~Widget(); //析构函数的声明 虚析构函数
private:
Ui::Widget *ui; //成员属性,指针
QLineEdit *edt1;//用户所对应的编辑器
QLineEdit *edt2;//密码所对应的编辑器
};
#endif // WIDGET_H
#include "widget.h"
#include "ui_widget.h"
//构造函数的定义
Widget::Widget(QWidget *parent) //在子类的初始化列表中显式调用父类的有参构造,来完成对子类从父类中继承下来成员的初始化
: QWidget(parent) //给自己的类中的指针成员实例化空间
, ui(new Ui::Widget)
{
ui->setupUi(this); //将ui界面上拖拽的组件展示到this界面上
this->setFixedSize(543,409);
this->setWindowTitle("华清远见");
this->setWindowIcon(QIcon("C:/QT text/huaqinghuajian.png"));
QLabel *lab1 = new QLabel;
lab1->setParent(this);
lab1->resize(543,200);
lab1->setPixmap(QPixmap("C:/QT text/huaqinghuajian.png"));
lab1->setScaledContents(true);//设置组件内容自适应
//账户密码
QLabel *lab2=new QLabel("账户",this);
lab2->move(188,200);
qDebug()<<lab2->size();
lab2->resize(100,40);
QLabel *lab3=new QLabel("密码",this);
lab3->setParent(this);
lab3->move(188,250);
qDebug()<<lab2->size();
lab2->resize(100,40);
edt1=new QLineEdit;
edt1->setParent(this);
edt1->move(230,200);
edt1->resize(200,30);
edt2 = new QLineEdit;//密码所对应的编辑器
edt2->setParent(this);
edt2->move(230,240);
edt2->resize(200,30);
edt2->setEchoMode(QLineEdit::Password);
QPushButton *btn1 = new QPushButton("登录",this);
btn1->move(300,300);
QPushButton *btn2 = new QPushButton("取消",this);
btn2->move(400,300);
connect(btn1,&QPushButton::clicked,this,&Widget::do_login);
//connect(btn2,&QPushButton::clicked,this,this->close());
connect(btn2,&QPushButton::clicked,this,[&](){
this->close();
});
}
Widget::~Widget()
{
delete ui; //释放ui界面申请的组件空间
}
void Widget::do_login()
{
if(edt1->text() == "admine" && edt2->text() == "123456")
{
qDebug() << "登录成功";
}
else
{
qDebug() << "账户密码不匹配";
}
}
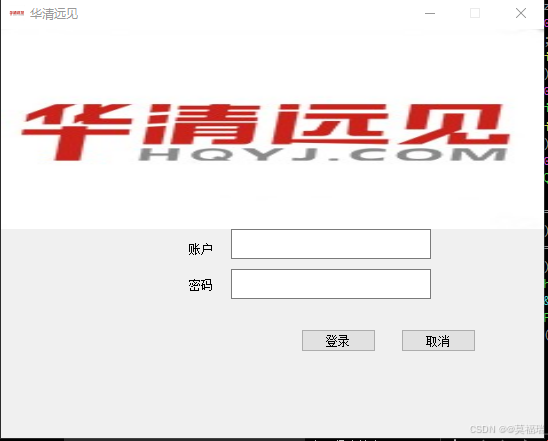