通讯录是基于顺序表的基础上实现的。顺序表包括内容定义,初始化,插入或删除数据,查找数据。相同的就可以转移到通讯录中的添加联系人,删除联系人,查找联系人,修改联系人。代码细节有注释讲解。
运行效果:
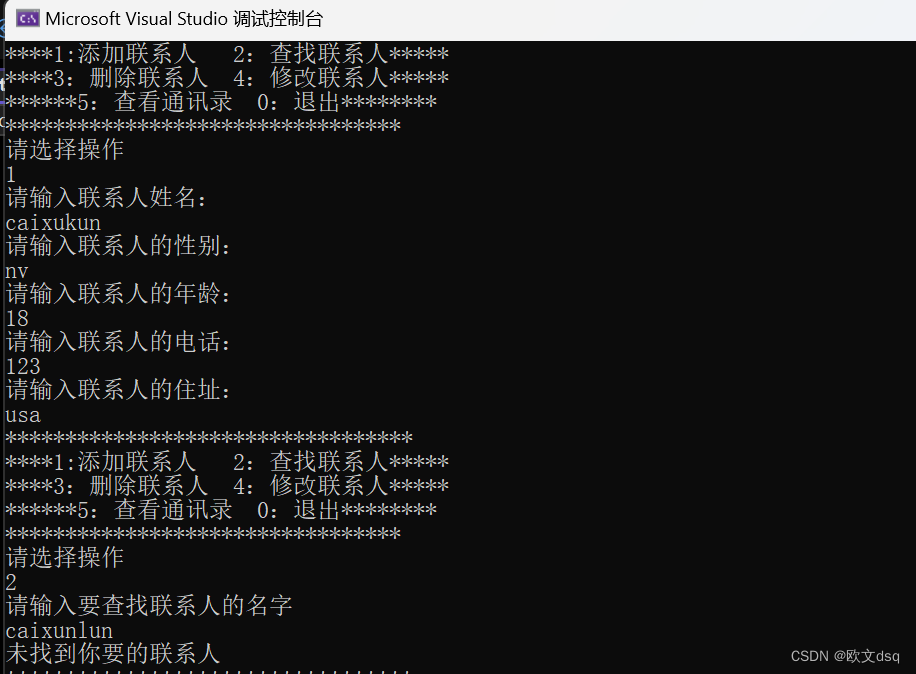
顺序表基础头文件
#pragma once
#include"Contact.h"
#include<stdio.h>
#include<stdlib.h>
#include<assert.h>
#include<stdbool.h>
typedef struct ContactInfo SLDataType;
typedef struct SeqList
{
SLDataType* a;
int size;
int capacity;
}SL;
void SLInit(SL* ps);
void SLDestroy(SL* ps);
void SLPushBack(SL* ps, SLDataType x);
void SLPushFront(SL* ps, SLDataType x);
void SLPopBack(SL* ps);
void SLPopFront(SL* ps);
void SLPrint(SL* ps);
bool SLIsEmpty(SL* ps);
void SLInsert(SL* ps, int pos, SLDataType x);
void SLErase(SL* ps, int pos);
bool SLFind(SL* ps, SLDataType x);
顺序表内容文件
#include"SeqList.h"
void SLInit(SL* ps) {
ps->a = NULL;
ps->size = ps->capacity = 0;
}
void SLDestroy(SL* ps) {
if (ps->a)
free(ps->a);
ps->a = NULL;
ps->size = ps->capacity = 0;
}
void SLCheckCapacity(SL* ps) {
if (ps->size == ps->capacity) {
int newCapcity = ps->capacity == 0 ? 4 : 2 * ps->capacity;
SLDataType* tmp = (SLDataType*)realloc(ps->a, newCapcity * sizeof(SLDataType));
if (tmp == NULL) {
perror("realloc fail!\n");
return 1;
}
ps->a = tmp;
ps->capacity = newCapcity;
}
}
void SLPushBack(SL* ps, SLDataType x) {
assert(ps);
SLCheckCapacity(ps);
ps->a[ps->size++] = x;
}
void SLPushFront(SL* ps, SLDataType x) {
assert(ps);
SLCheckCapacity(ps);
for (size_t i = ps->size; i > 0; i--)
{
ps->a[i] = ps->a[i - 1];
}
ps->a[0] = x;
ps->size++;
}
void SLPopBack(SL* ps) {
assert(ps);
assert(!SLIsEmpty(ps));
ps->size--;
}
void SLPopFront(SL* ps) {
assert(ps);
assert(!SLIsEmpty(ps));
for (size_t i = 0; i < ps->size - 1; i++)
{
ps->a[i] = ps->a[i + 1];
}
ps->size--;
}
void SLPrint(SL* ps) {
for (size_t i = 0; i < ps->size; i++)
{
printf("%d ", ps->a[i]);
}
printf("\n");
}
bool SLIsEmpty(SL* ps) {
assert(ps);
return ps->size == 0;
}
void SLInsert(SL* ps, int pos, SLDataType x) {
assert(ps);
assert(pos >= 0 && pos <= ps->size);
SLCheckCapacity(ps);
for (int i = ps->size - 1; i > pos - 1; i--)
{
ps->a[i + 1] = ps->a[i];
}
ps->a[pos] = x;
ps->size++;
}
void SLErase(SL* ps, int pos) {
assert(ps);
assert(!SLIsEmpty(ps));
assert(pos >= 0 && pos < ps->size);
for (int i = pos; i < ps->size - 1; i++)
{
ps->a[i] = ps->a[i + 1];
}
ps->size--;
}
通讯录头文件
#pragma once
#pragma once
#define _CRT_SECURE_NO_WARNINGS 1
#define NAME_MAX 100
#define SEX_MAX 10
#define TEL_MAX 15
#define ADDR_MAX 100
struct ContactInfo
{
char name[NAME_MAX];
char sex[SEX_MAX];
int age;
char tel[TEL_MAX];
char addr[ADDR_MAX];
};
typedef struct ContactInfo CInfo;
typedef struct SeqList contact;
void ContactInit(contact* pcon);
void ContactDestroy(contact* pcon);
void ContactAdd(contact* pcon);
void ContactDel(contact* pcon);
void ContactModify(contact* pcon);
void ContactShow(contact* pcon);
void ContactFind(contact* pcon);
通讯录内容文件
#include"Contact.h"
#include"SeqList.h"
void ContactInit(contact* pcon) {
SLInit(pcon);
}
void ContactDestroy(contact* pcon) {
SLDestroy(pcon);
}
void ContactAdd(contact* pcon) {
CInfo info;
printf("请输入联系人姓名:\n");
scanf("%s", info.name);
printf("请输入联系人的性别:\n");
scanf("%s", info.sex);
printf("请输入联系人的年龄:\n");
scanf("%d", &info.age);
printf("请输入联系人的电话:\n");
scanf("%s", info.tel);
printf("请输入联系人的住址:\n");
scanf("%s", info.addr);
SLPushBack(pcon, info);
}
int FindByName(contact* pcon, char name[]) {
for (int i = 0; i < pcon->size; i++)
{
if (strcmp(pcon->a[i].name, name) == 0) {
return i;
}
}
return -1;
}
void ContactDel(contact* pcon) {
printf("请输入要删除的用户名称:\n");
char name[NAME_MAX];
scanf("%s", name);
int findidex = FindByName(pcon, name);
if (findidex < 0) {
printf("要删除的联系人不存在!\n");
return;
}
SLErase(pcon, findidex);
}
void ContactModify(contact* pcon)
{
char name[NAME_MAX];
scanf("%s", name);
int find = FindByName(pcon, name);
if (find < 0)
{
printf("查找的联系人不存在");
return;
}
printf("请输入新用户的名字\n");
scanf("%s", pcon->a[find].name);
printf("请输入新用户的性别\n");
scanf("%s", pcon->a[find].sex);
printf("请输入新用户的年龄\n");
scanf("%d", &pcon->a[find].age);
printf("请输入新用户的号码\n");
scanf("%s", pcon->a[find].tel);
printf("请输入新用户的地址\n");
scanf("%s", pcon->a[find].addr);
printf("修改成功\n");
}
void ContactShow(contact* pcon) {
printf("%s %s %s %s %s\n", "姓名", "性别", "年龄", "电话", "住址");
for (int i = 0; i < pcon->size; i++)
{
printf("%-4s %-4s %-4d %-4s %-4s\n",
pcon->a[i].name,
pcon->a[i].sex,
pcon->a[i].age,
pcon->a[i].tel,
pcon->a[i].addr
);
}
}
void ContactFind(contact* pcon)
{
char name[NAME_MAX];
printf("请输入要查找联系人的名字\n");
scanf("%s", name);
int find = FindByName(pcon, name);
if (find < 0)
{
printf("未找到你要的联系人\n");
return;
}
else
{
printf("%s %s %s %s %s\n", "姓名", "性别", "年龄", "电话", "住址");
printf("%-4s %-4s %-4d %-4s %-4s\n",
pcon->a[find].name,
pcon->a[find].sex,
pcon->a[find].age,
pcon->a[find].tel,
pcon->a[find].addr
);
}
}
测试文件
#include"SeqList.h"
#include"Contact.h"
void mnue()
{
printf("**********************************\n");
printf("****1:添加联系人 2:查找联系人*****\n");
printf("****3:删除联系人 4:修改联系人*****\n");
printf("******5:查看通讯录 0:退出********\n");
printf("*********************************\n");
}
int main() {
int op = -1;
contact con;
ContactInit(&con);
do
{
mnue();
printf("请选择操作\n");
scanf("%d", &op);
switch (op)
{
case 1:
ContactAdd(&con);
break;
case 2:
ContactFind(&con);
break;
case 3:
ContactDel(&con);
break;
case 4:
ContactModify(&con);
break;
case 5:
ContactShow(&con);
break;
case 0:
printf("goodbye~\n");
break;
default:
printf("输入错误\n");
break;
}
} while (op);
ContactDestroy(&con);
return 0;
}