除非有很好的理由选择其他容器,否则应使用vector。
如果你的程序有很多小的元素,且空间的额外开销很重要,则不要使用list或forward_list。
如果程序要求随机访问元素,应使用vector或deque。
如果程序要求在容器的中间插入或删除元素,应使用list或forward_list。
如果程序需要在头尾位置插入或删除元素,但不会在中间位置进行插入或删除操作,则使用deque。
如果程序只有在读取输入时才需要在容器中间位置插入元素,随后需要随机访问元素,则
1) 首先,确定是否真的需要在容器中间位置添加元素。当处理输入数据时,通常可以很容易地向vector追加数据,然后再调用标准库的sort函数来重排容器中的元素,从而避免在中间位置添加元素。
2) 如果必须在中间位置插入元素,考虑在输入阶段使用list,一旦输入完成,将list中的内容拷贝到一个vector中。
#include <string>
#include <iostream>
using namespace std;
int main()
{
string s1;//default
cout << s1 << endl;//空字符串
string s2("hello world");//from c-string
cout << s2 << endl;//hello world
string s3(s2);//copy
//等价于string s3 = s2;
cout << s3 << endl;//hello world
string s4(s3, 0, 5);//substring
cout << s4 << endl;//hello
string s5("hello world", 5);//from buffer
cout << s5 << endl;//hello
string s6(10, '*');//fill
cout << s6 << endl;//**********
string s7(s2.begin() + 6, s2.end());//range
cout << s7 << endl;//world
string s8{ 'h','a','p','p','y' };//initializer list
//等价于string s8 = { 'h','a','p','p','y' };
cout << s8 << endl;//happy
return 0;
}
#include <string>
#include <iostream>
using namespace std;
int main()
{
string s("hello world");
cout << s.size() << endl;//11
cout << s.length() << endl;//11
cout << s.max_size() << endl;//2147483647
cout << s.capacity() << endl;//15
s.resize(15, '!');
cout << s << endl;//hello world!!!!
s.resize(13);
cout << s << endl;//hello world!!
s.reserve(30);
cout << s.capacity() << endl;//31
s.shrink_to_fit();
cout << s.capacity() << endl;//15
s.clear();
if (s.empty())
cout << "s被清空" << endl;
else
cout << "s没被清空" << endl;
//s被清空
return 0;
}
#include <string>
#include <iostream>
using namespace std;
int main()
{
string s1("hello");
string s2("world");
s1 += " ";
s1 += s2;
s1 += '!';
cout << s1 << endl;//hello world!
s2.append(" peace");
s2.append(2, '!');
cout << s2 << endl;//world peace!!
//尾插一个字符的3种写法
s1 += '*';//operator+=最常用
s1.append(1, '~');
s2.push_back('@');
cout << s1 << endl;//hello world!*~
cout << s2 << endl;//world peace!!@
s1.assign("I want to study.");
cout << s1 << endl;//I want to study.
s1.insert(15, " and play");
cout << s1 << endl;//I want to study and play.
s1.erase(10, 10);
cout << s1 << endl;//I want to play.
s1.replace(0, 1, "They");
cout << s1 << endl;//They want to play.
s1.swap(s2);
cout << s1 << endl;//world peace!!@
cout << s2 << endl;//They want to play.
s1.pop_back();
cout << s1 << endl;//world peace!!
return 0;
}
#include <string>
#include <iostream>
using namespace std;
int main()
{
string s("hello world");
for (auto ch : s)
{
cout << ch << " ";
}
cout << endl;
//h e l l o w o r l d
return 0;
}
#include <string>
#include <iostream>
using namespace std;
int main()
{
string s("hello world i love you");
/*//第1种方法
size_t num = 0;
for (auto ch : s)
{
if (ch == ' ')
++num;//计算有几个空格
}
//提前开空间,避免repalce时扩容
s.reserve(s.size() + 2 * num);
size_t pos = s.find(' ');//第一个空格的位置
while (pos != string::npos)//npos是长度参数,表示直到字符串结束
{
s.replace(pos, 1, "%20");
pos = s.find(' ', pos + 3);
}
cout << s << endl;//hello%20world%20i%20love%20you*/
//第2种方法
string newStr;
size_t num = 0;
for (auto ch : s)
{
if (ch == ' ')
++num;//计算有几个空格
}
//提前开空间
newStr.reserve(s.size() + 2 * num);
for (auto ch : s)
{
if (ch != ' ')
newStr += ch;
else
newStr += "%20";
}
cout << newStr << endl;//hello%20world%20i%20love%20you
return 0;
}
#include <string>
#include <iostream>
using namespace std;
int main()
{
string s("hello world i love you");
/*//第1种方法
size_t num = 0;
for (auto ch : s)
{
if (ch == ' ')
++num;//计算有几个空格
}
//提前开空间,避免repalce时扩容
s.reserve(s.size() + 2 * num);
size_t pos = s.find(' ');//第一个空格的位置
while (pos != s.npos)//npos是长度参数,表示直到字符串结束
{
s.replace(pos, 1, "%20");
pos = s.find(' ', pos + 3);
}
cout << s << endl;//hello%20world%20i%20love%20you*/
//第2种方法
string newStr;
size_t num = 0;
for (auto ch : s)
{
if (ch == ' ')
++num;//计算有几个空格
}
//提前开空间
newStr.reserve(s.size() + 2 * num);
for (auto ch : s)
{
if (ch != ' ')
newStr += ch;
else
newStr += "%20";
}
cout << newStr << endl;//hello%20world%20i%20love%20you
return 0;
}
拿oj一题来练练
以下给出三种解决办法
一
#include<iostream>
#include<string>
using namespace std;
int main() {
int n; cin >> n;
while (n--) {
string s, d;
int m;
char c;
cin >> s >> m >> c >> d;
int j = d.length();
size_t pos = s.find(c);
while (pos != s.npos && m>0)
{
s.replace(pos, 1, d);
pos = s.find(c, pos +j );
m--;
}
cout << s << endl;
}
return 0;
}
二
#include<iostream>
#include<string>
using namespace std;
int main() {
int n; cin >> n;
while (n--) {
string s, d;
int m;
char c;
cin >> s >> m >> c >> d;
int j = d.length()-1;
for (int i = 0; i < s.length(); ++i)
{
if (s[i] == c && m > 0)
{
s.replace(i, 1, d);
m--;
i += j;
}
}
cout << s << endl;
}
return 0;
}
三
#include <iostream>
#include <string>
using namespace std;
int main()
{
int n;cin>>n;
while(n--)
{
string s,d; int m; char c; cin>>s>>m>>c>>d;
for (int i = 0; i < s.length(); ++i)
{
if(m>0&&s[i]==c)
{
cout<<d;
m--;
}
else cout<<s[i];
}
cout<<endl;
}
}
#include <iostream>
#include <string>
using namespace std;
int main()
{
int n;cin>>n;
while(n--)
{
string s,d; int m; char c; cin>>s>>m>>c>>d;
for (int i = 0; i < s.length(); ++i)
{
if(m>0&&s[i]==c)
{
cout<<d;
m--;
}
else cout<<s[i];
}
cout<<endl;
}
}
计算空格+提前开空间
size_t num = 0;
for (auto ch : s)
{
if (ch == ' ')
++num;//计算有几个空格
}
//提前开空间,避免repalce时扩容
s.reserve(s.size() + 2 * num);
size_t pos = s.find(' ');//第一个空格的位置
同oj的第三种办法
string newStr;
size_t num = 0;
for (auto ch : s)
{
if (ch == ' ')
++num;//计算有几个空格
}
//提前开空间
newStr.reserve(s.size() + 2 * num);
for (auto ch : s)
{
if (ch != ' ')
newStr += ch;
else
newStr += "%20";
}
cout << newStr << endl;
//hello%20world%20i%20love%20you
wocccccccccccccc
oj那题又写出来一种方法——第四种
#include <iostream>
#include <string>
using namespace std;
int main()
{
int n; cin >> n;
while (n--){
string s, d, newStr; int m; char c; cin >> s >> m >> c >> d;
for (auto ch : s){
if (ch != c) newStr += ch;
else if (ch == c && m > 0)
{
newStr += d;
m--;
}
else if (ch == c && m == 0)newStr += c;
}
cout << newStr << endl;
}
}
使用遍历解决
#include <string>
#include <iostream>
using namespace std;
int main()
{
string s("hello");
cout << s.c_str() << endl;//hello
cout << (void*)s.c_str() << endl;//地址
cout << s.data() << endl;//hello
char buffer[10] = { '\0' };
s.copy(buffer, 3, 1);
for (auto ch : buffer)
{
cout << ch;
}
cout << endl;
//ell
size_t pos = s.find('l');
while (pos != string::npos)//npos是长度参数,表示直到字符串结束
{
s.replace(pos, 1, "L");
pos = s.find('l', pos + 1);
}
cout << s << endl;
//heLLo
pos = s.rfind('L');
while (pos != string::npos)
{
s.replace(pos, 1, "l");
pos = s.rfind('L', pos - 1);
}
cout << s << endl;
//hello
cout << s.find_first_of("jklmn") << endl;//2
cout << s.find_last_of("jklmn") << endl;//3
cout << s.find_first_not_of("heL") << endl;//2
cout << s.find_last_not_of("Lo") << endl;//3
cout << s.substr(1, 3) << endl;//ell
cout << s.compare("hello world") << endl;//-1
return 0;
}
任意字符
size_t pos = s.find('l');
while (pos != string::npos)//npos是长度参数,表示直到字符串结束
{
s.replace(pos, 1, "L");
pos = s.find('l', pos + 1);
}
cout << s << endl;
//heLLo
pos = s.rfind('L');
while (pos != string::npos)
{
s.replace(pos, 1, "l");
pos = s.rfind('L', pos - 1);
}
cout << s << endl;
//hello
cout << s.find_first_of("jklmn") << endl;//2
cout << s.find_last_of("jklmn") << endl;//3
cout << s.find_first_not_of("heL") << endl;//2
cout << s.find_last_not_of("Lo") << endl;//3
cout << s.substr(1, 3) << endl;//ell
cout << s.compare("hello world") << endl;//-1
大写变小写小写变大写——
size_t pos = s.find('l');
while (pos != string::npos)//npos是长度参数,表示直到字符串结束
{
s.replace(pos, 1, "L");
pos = s.find('l', pos + 1);
}
cout << s << endl;
//heLLo
pos = s.rfind('L');
while (pos != string::npos)
{
s.replace(pos, 1, "l");
pos = s.rfind('L', pos - 1);
}
cout << s << endl;
也可以用前面文章里提到的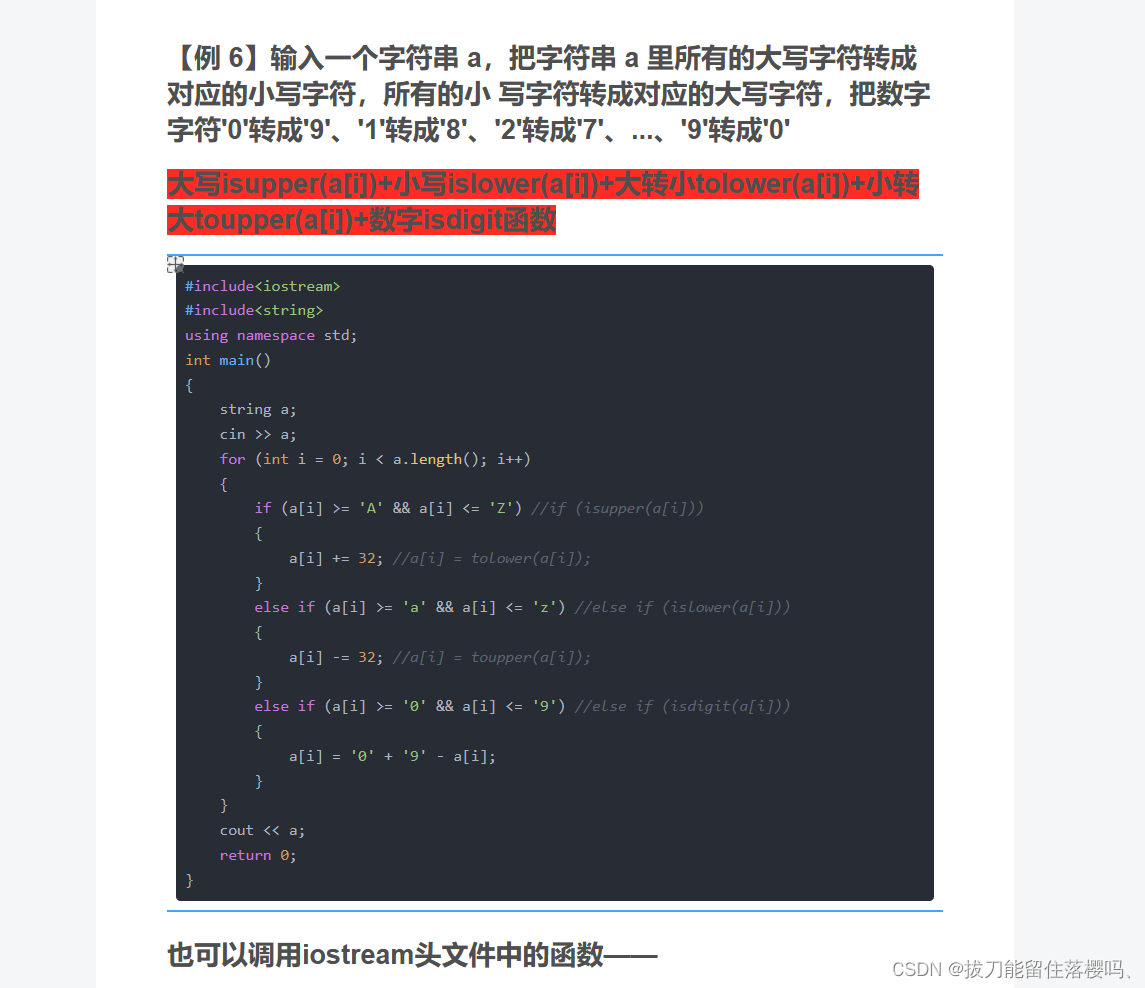
【例 6】输入一个字符串 a,把字符串 a 里所有的大写字符转成对应的小写字符,所有的小 写字符转成对应的大写字符,把数字字符'0'转成'9'、'1'转成'8'、'2'转成'7'、...、'9'转成'0'
大写isupper(a[i])+小写islower(a[i])+大转小tolower(a[i])+小转大toupper(a[i])+数字isdigit函数
#include<iostream>
#include<string>
using namespace std;
int main()
{
string a;
cin >> a;
for (int i = 0; i < a.length(); i++)
{
if (a[i] >= 'A' && a[i] <= 'Z') //if (isupper(a[i]))
{
a[i] += 32; //a[i] = tolower(a[i]);
}
else if (a[i] >= 'a' && a[i] <= 'z') //else if (islower(a[i]))
{
a[i] -= 32; //a[i] = toupper(a[i]);
}
else if (a[i] >= '0' && a[i] <= '9') //else if (isdigit(a[i]))
{
a[i] = '0' + '9' - a[i];
}
}
cout << a;
return 0;
}
#include<iostream>
#include<string>
using namespace std;
int main()
{
string a;cin >> a;
for (int i = 0; i < a.length(); i++)
{
if (isupper(a[i]))a[i] = tolower(a[i]);
else if (islower(a[i]))a[i] = toupper(a[i]);
else if (isdigit(a[i]))a[i] = '0' + '9' - a[i];
}
cout << a;
return 0;
}