最后
除了简历做到位,面试题也必不可少,整理了些题目,前面有117道汇总的面试到的题目,后面包括了HTML、CSS、JS、ES6、vue、微信小程序、项目类问题、笔试编程类题等专题。
主要代码:
图片选择引入:
html, body {
margin: 0;
padding: 0;
overflow: hidden;
background: #000;
font-family: Montserrat, sans-serif;
background-image: url(img/pexels-photo-219692.jpeg);
background-size: cover;
background-position: center;
}
css样式:
html, body {
margin: 0;
padding: 0;
overflow: hidden;
background: #000;
font-family: Montserrat, sans-serif;
background-image: url(img/pexels-photo-219692.jpeg);
background-size: cover;
background-position: center;
}
canvas {
mix-blend-mode: lighten;
cursor: pointer;
}
#hero {
display: inline;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
mix-blend-mode: color-dodge;
}
#year {
font-size: 30vw;
color: #d0d0d0;
font-weight: bold;
}
#timeleft {
color: #fbfbfb;
font-size: 2.5vw;
text-align: center;
font-family: Lora, serif;
}
Javascirpt:
const canvas = document.createElement('canvas'),
context = canvas.getContext('2d'),
width = canvas.width = window.innerWidth,
height = canvas.height = window.innerHeight,
HalfPI = Math.PI / 2,
gravity = vector.create(0, 0.35),
year = document.getElementById('year'),
timeleft = document.getElementById('timeleft'),
newyear = new Date('01/01/2020');
let objects = [],
startFireworks = false,
newYearAlready = false;
function renderTimeLeft() {
let date = new Date();
let delta = Math.abs(newyear - date) / 1000;
let hours = Math.floor(delta / 3600) % 24;
delta -= hours * 3600;
let minutes = Math.floor(delta / 60) % 60;
delta -= minutes * 60;
let seconds = Math.floor(delta % 60) + 1;
let string = '';
let DaysLeft = Math.floor((newyear - date) / (1000 * 60 * 60 * 24)),
HoursLeft = `${hours} ${hours > 1 ? 'hours' : 'hour'}`,
MinutesLeft = `${minutes} ${minutes > 1 ? 'minutes' : 'minute'}`,
SecondsLeft = `${seconds} ${seconds > 1 ? 'seconds' : 'second'}`;
if (hours > 0) string = `${HoursLeft} & ${MinutesLeft}`;else
if (minutes > 0) string = `${MinutesLeft} & ${SecondsLeft}`;else
string = `${SecondsLeft}`;
if (DaysLeft > 0) string = DaysLeft + ' days, ' + string;
string += ' until 2020';
timeleft.innerHTML = string;
}
renderTimeLeft();
setInterval(function () {
let date = new Date();
if (date >= newyear) {
if (!newYearAlready) {
year.innerHTML = '2022';
startFireworks = true;
timeleft.innerHTML = 'Happy New Year!';
}
newYearAlready = true;
} else renderTimeLeft();
}, 500);
document.body.appendChild(canvas);
function random255() {
return Math.floor(Math.random() * 100 + 155);
}
function randomColor() {
let r = random255(),
g = random255(),
b = random255();
return `rgb(${r}, ${g}, ${b})`;
}
class PhysicsBody {
constructor() {
objects.push(this);
}
PhysicsUpdate() {
this.lastPosition = this.position.duplicate();
this.position.addTo(this.velocity);
this.velocity.addTo(gravity);
}
deleteObject() {
objects[objects.indexOf(this)] = undefined;
}}
class firework extends PhysicsBody {
constructor() {
super();
this.position = vector.create(Math.random() * width, height);
let Velocity = vector.create(0, 0);
Velocity.setLength(Math.random() * 10 + 15);
Velocity.setAngle(Math.PI * 1.35 + Math.random() * Math.PI * 0.30);
this.velocity = Velocity;
this.trail = Math.floor(Math.random() * 4) != 1;
this.trailColor = this.trail ? randomColor() : undefined;
this.trailWidth = 2;
this.TimeCreated = new Date().getTime();
this.TimeExpired = this.TimeCreated + (Math.random() * 5 + 7) * 100;
this.BlastParticleCount = Math.floor(Math.random() * 50) + 25;
this.funky = Math.floor(Math.random() * 5) == 1;
this.exposionColor = randomColor();
}
draw() {
context.strokeStyle = this.trailColor;
context.lineWidth = this.trailWidth;
let p = this.position,
lp = this.lastPosition;
context.beginPath();
context.moveTo(lp.getX(), lp.getY());
context.lineTo(p.getX(), p.getY());
context.stroke();
}
funkyfire() {
var funky = this.funky;
for (var i = 0; i < Math.floor(Math.random() * 10); i++) {
new BlastParticle({ firework: this, funky });
}
}
explode() {
var funky = this.funky;
for (var i = 0; i < this.BlastParticleCount; i++) {
new BlastParticle({ firework: this, funky });
}
this.deleteObject();
}
checkExpire() {
let now = new Date().getTime();
if (now >= this.TimeExpired) this.explode();
}
#### 最后
-------------------------------------------------------------
**[开源分享:【大厂前端面试题解析+核心总结学习笔记+真实项目实战+最新讲解视频】](https://bbs.csdn.net/topics/618166371)**
大厂面试问深度,小厂面试问广度,如果有同学想进大厂深造一定要有一个方向精通的惊艳到面试官,还要平时遇到问题后思考一下问题的本质,找方法解决是一个方面,看到问题本质是另一个方面。还有大家一定要有目标,我在很久之前就想着以后一定要去大厂,然后默默努力,每天看一些大佬们的文章,总是觉得只有再学深入一点才有机会,所以才有恒心一直学下去。
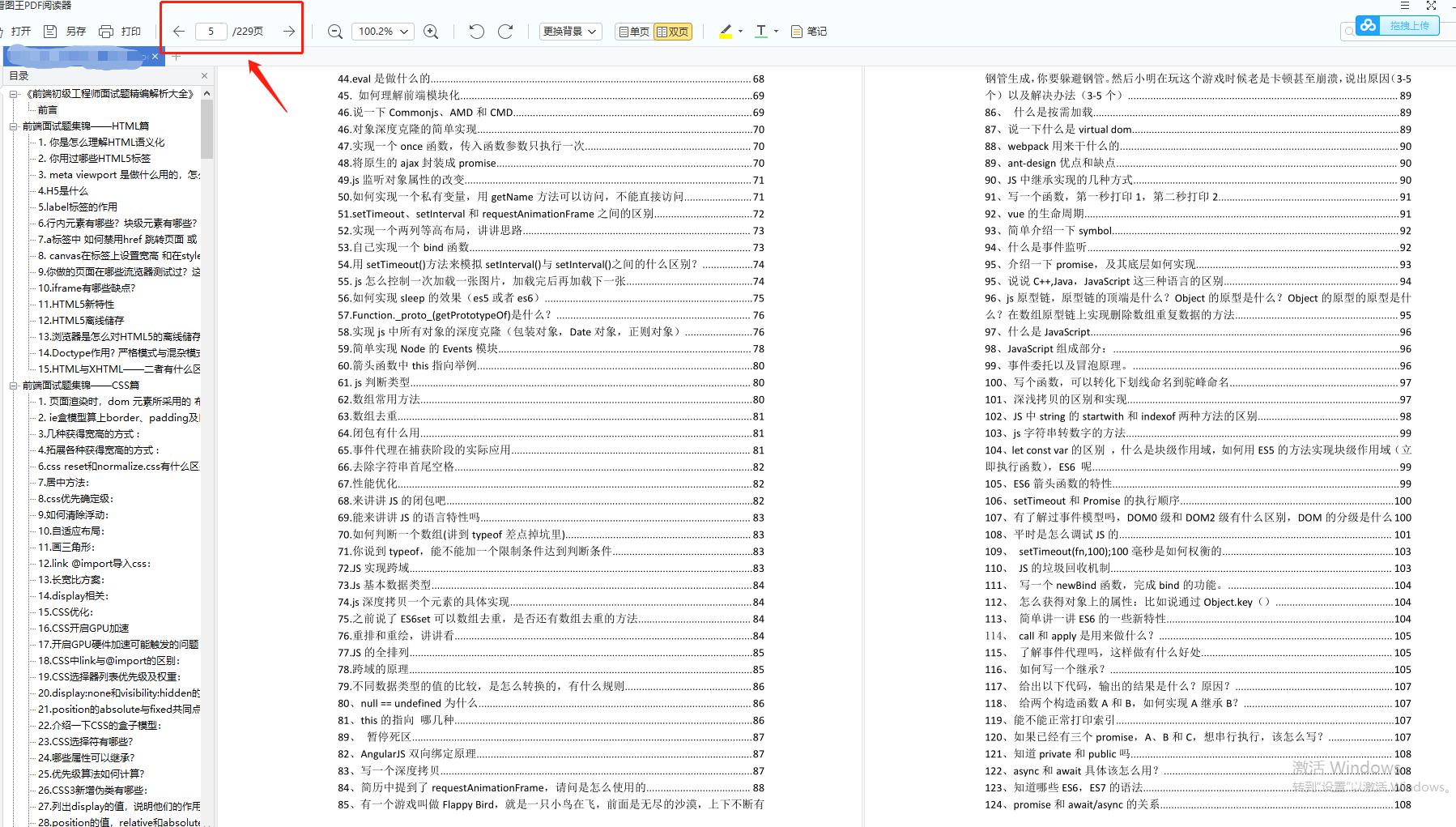