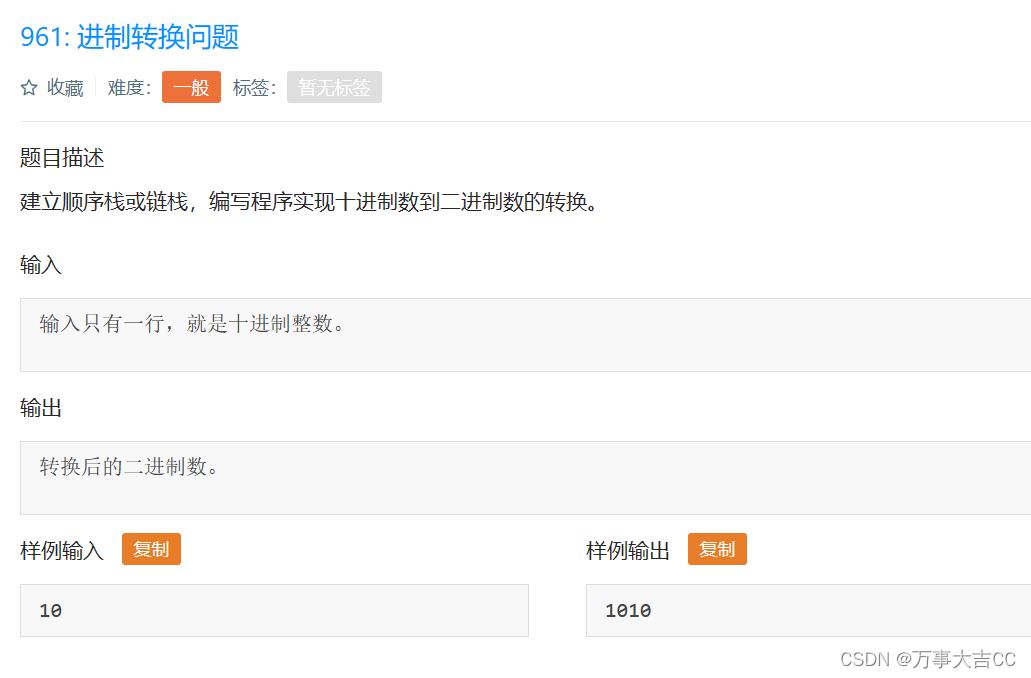
【学习版】
【C语言】
#include<iostream>
struct SeqList {
int top;
int len;
int* s;
};
void initStack(SeqList* stack, int len) {
stack->s = new int[len];
stack->top = -1;
stack->len = len;
}
void push(SeqList* stack, int x) {
stack->s[++stack->top] = x;
}
void pop(SeqList* stack) {
if (stack->top != -1)
stack->top--;
}
void trans(int n, SeqList* stack) {
if (n == 0) {
push(stack, 0);
return;
}
while (n > 0) {
push(stack, n % 2);
n /= 2;
}
while (stack->top != -1) {
std::cout << stack->s[stack->top--];
}
}
int main() {
int n;
std::cin >> n;
SeqList s;
initStack(&s, 1000);
trans(n, &s);
delete[] s.s;
return 0;
}
【C++】
#include<iostream>
#include<vector>
class ArrayStack {
private:
std::vector<int> stack;
public:
bool isEmpty() {
return stack.size() == 0;
}
void push(int num) {
stack.push_back(num);
}
int pop() {
int num = stack.back();
stack.pop_back();
return num;
}
};
void trans(ArrayStack s, int dec) {
if (dec == 0) {
s.push(0);
}
while (dec > 0) {
s.push(dec % 2);
dec /= 2;
}
while (!s.isEmpty()) {
std::cout << s.pop();
}
}
int main() {
int n;
std::cin >> n;
ArrayStack stk;
trans(stk, n);
return 0;
}
【STL】
#include<iostream>
#include<stack>
void trans(std::stack<int> s, int dec) {
if (dec == 0) {
s.push(0);
}
while (dec > 0) {
s.push(dec % 2);
dec /= 2;
}
while (!s.empty()) {
std::cout << s.top();
s.pop();
}
}
int main() {
int n;
std::cin >> n;
std::stack<int> stk;
trans(stk, n);
return 0;
}