运行结果(+-*/)实例: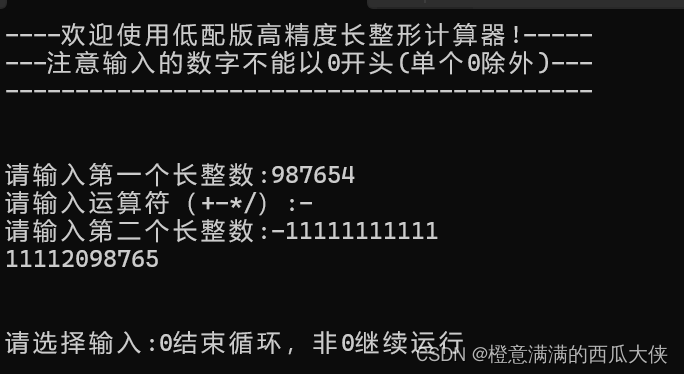
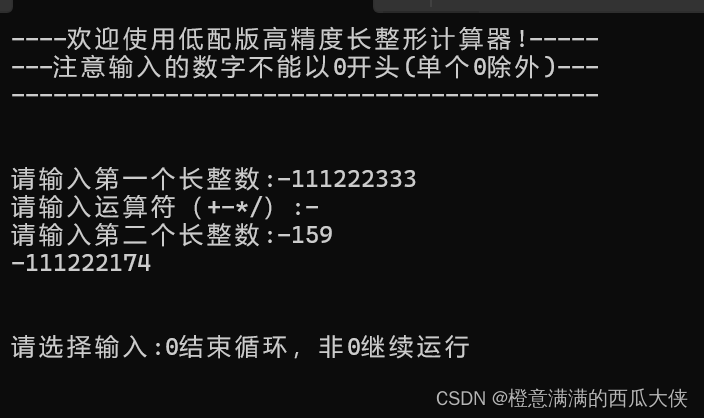
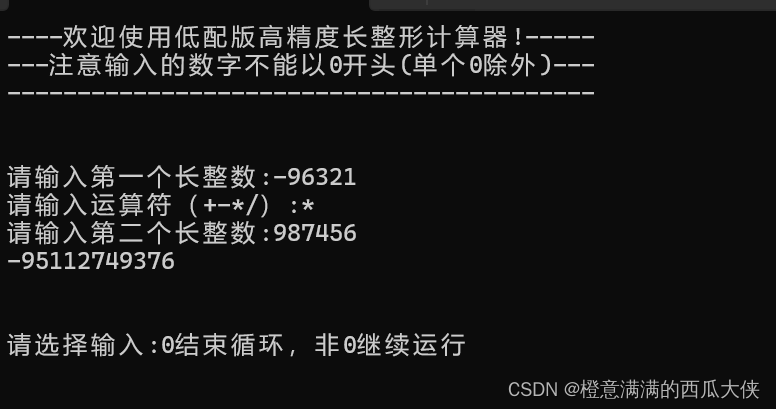
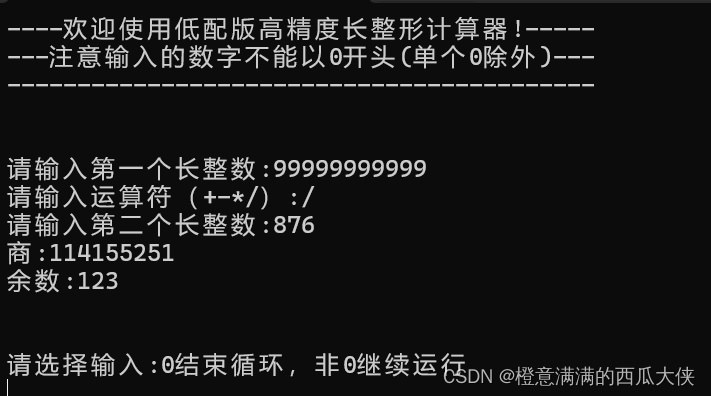
代码如下:
#include<iostream>
#include<cstring>
using namespace std;
//减去符号
void qufuhao(char s[])
{
int len = strlen(s);
for (int i = 1;i < len;i++)
{
s[i - 1] = s[i];
}
s[len - 1] = '\0';
}
void fanzhuan(int a[], int lena)
{
for (int i = 0;i < lena / 2;i++)
{
int t = a[i];
a[i] = a[lena - 1 - i];
a[lena - 1 - i] = t;
}
}
int comp(char s1[], char s2[])
{
int len1 = strlen(s1), len2 = strlen(s2);
if (len1 > len2)
return 1;
else if (len1 < len2)
return -1;
else
{
for (int i = 0;i < len1;i++)
{
if (s1[i] > s2[i])
return 1;
else if (s1[i] < s2[i])
return -1;
}
return 0;
}
}
int comp(int a[], int b[], int lena)//比较等长的数组
{
for (int i = 0;i < lena;i++)
{
if (a[i] > b[i])
return 1;
if (a[i] < b[i])
return -1;
}
return 0;
}
void copy(int a[], int lena, int b[], int& lenb, int d)
{
for (int i = 0;i < lena;i++)
{
b[i + d] = a[i];
}
lenb = lena + d;
}
void subtract(int a[], int lena, int b[], int c[])//大数a减小数b
{
for (int i = 0;i < lena;i++)
{
if (a[i] < b[i])
{
a[i] += 10;//借位
a[i + 1]--;
}
c[i] = a[i] - b[i];
}
}
void subtract(int a[], int b[], int lena)
{
for (int i = lena - 1;i >= 0; i--)
{
if (a[i] < b[i])
{
a[i] += 10;
a[i - 1]--;
}
a[i] -= b[i];
}
}
void multiple(int a[], int lena, int b[], int lenb, int c[])
{
for (int i = 0;i < lena;i++)//大的a
{
for (int j = 0;j < lenb;j++)//小的b
{
c[j + i] += a[i] * b[j];
if (c[j + i] > 9)
{
c[j + i + 1] += c[j + i] / 10;
c[j + i] %= 10;
}
}
}
}
void output(int c[], int lenc, int sig)
{
while (lenc > 0 && c[lenc - 1] == 0)
lenc--;//去除前导零
if (!lenc)
{
cout << '0' << endl;
return;
}
if (sig == -1)
cout << '-';
for (int i = lenc - 1;i >= 0;i--)
cout << c[i];
cout << endl;
}
int main()
{
char s1[260], s2[260];
char k;
int choice = 1;
while (choice)
{
system("cls");
int sig = 1;
cout << "----欢迎使用低配版高精度长整形计算器!-----" << endl;
cout << "---注意输入的数字不能以0开头(单个0除外)---" << endl;
cout << "------------------------------------------" << endl;
cout << endl << endl;
cout << "请输入第一个长整数:";
cin >> s1;
cout << "请输入运算符(+-*/):";
cin >> k;
cout << "请输入第二个长整数:";
cin >> s2;
//化归为两个正数运算的模型
switch (k)
{
case'+':
{if (s1[0] == '-' && s2[0] != '-')
{
qufuhao(s1);
k = '-';sig = -1;
}
if (s1[0] != '-' && s2[0] == '-')
{
qufuhao(s2);
k = '-';
}
if (s1[0] == '-' && s2[0] == '-')
{
qufuhao(s1);qufuhao(s2);
sig = -1;
}}
break;
case'-':
{if (s1[0] == '-' && s2[0] != '-')
{
qufuhao(s1);
k = '+';sig = -1;
}
if (s1[0] != '-' && s2[0] == '-')
{
qufuhao(s2);
k = '+';
}
if (s1[0] == '-' && s2[0] == '-')
{
qufuhao(s1);qufuhao(s2);
sig = -1;
}}
break;
case'*':case'/':
{if (s1[0] == '-' && s2[0] != '-')
{
qufuhao(s1);
sig = -1;
}
if (s1[0] != '-' && s2[0] == '-')
{
qufuhao(s2);
sig = -1;
}
if (s1[0] == '-' && s2[0] == '-')
{
qufuhao(s1);
qufuhao(s2);
}}
break;
}
//存储
int a[260] = { 0 }, b[260] = { 0 }, c[260] = { 0 };
int lena = strlen(s1), lenb = strlen(s2), lenc;
for (int i = 0;i < lena;i++)
{
a[i] = s1[lena - 1 - i] - '0';
}
for (int i = 0;i < lenb;i++)
{
b[i] = s2[lenb - 1 - i] - '0';
}
//选择运算
switch (k)
{
case'+'://加法
{
lenc = lena > lenb ? lena : lenb;
for (int i = 0;i < lenc;i++)
{
c[i] += a[i] + b[i];
if (c[i] > 9)
{
c[i + 1] = 1;
c[i] %= 10;
}
}
if (c[lenc])
lenc++;
output(c, lenc, sig);
}break;
case'-'://减法
{
int t = comp(s1, s2);
if (t == 1)
{
subtract(a, lena, b, c);
lenc = lena;
output(c, lenc, sig);
}
else if (t == -1)
{
subtract(b, lenb, a, c);
lenc = lenb;
sig = -sig;
output(c, lenc, sig);
}
else
cout << 0 << endl;
}break;
case'*'://乘法
{
int t = comp(s1, s2);
if (t == 1)
{
multiple(a, lena, b, lenb, c);
}
else
{
multiple(b, lenb, a, lena, c);
}
lenc = lena + lenb;
output(c, lenc, sig);
}break;
case'/'://除法
{ int t = 0;
for (int i = lenb - 1;i >= 0;i--)//判断被除数是否为零
{
if (b[i])
{
t = 1;break;
}
}
if (t == 0)
{
cout << "Error!被除数不能为0!\n";
break;
}
int tempb[260] = { 0 };int lentb;
fanzhuan(a, lena);
fanzhuan(b, lenb);
lenc = lena - lenb + 1;
int s = 0;
if (lena < lenb || comp(a, b, lena) < 0)//当a比b小时
s = 1;
for (int i = 0;i < lenc;i++)
{
memset(tempb, 0, sizeof(tempb));
copy(b, lenb, tempb, lentb, i);
lena = lentb;
while (comp(a, tempb, lena) >= 0)
{
subtract(a, tempb, lena);
c[i]++;
}
}
fanzhuan(c, lenc);
cout << "商:";
if (s)
cout << '0' << endl;
else
{
output(c, lenc, sig);
}
cout << "余数:";
fanzhuan(a, lena);
output(a, lena, sig);
}break;
}
cout << endl << endl;
cout << "请选择输入:0结束循环,非0继续运行 \n";
cin >> choice;
}
return 0;
}