7.1
/*
写一个函数,该函数接受三个整型参数,调用此函数之后,三个参数的值会按照从小到达进行排序,例:
int a = 3;
int b = 9;
int c = 1;
// 调用函数
sort_three(a,b,c);
// 调用之后:
// a = 1, b = 3, c = 9
*/
#include <iostream>
using namespace std;
void swap_if_max(int &a, int &b) {
int tmp=a;
if(a>b)
{
tmp=a;
a=b;
b=tmp;
}
}
void sort_three(int&a ,int &b, int &c) {
swap_if_max(a,b);
swap_if_max(a,c);
swap_if_max(b,c);
}
int main()
{
int a,b,c;
cin>>a>>b>>c;
sort_three(a,b,c);
cout << a << "," << b << "," << c <<endl;
return 0;
}
统计输入的单词个数,利用指针
7.2
#include<iostream>
using namespace std;
int main()
{
/*char str[20]="I have a dream.";
char *p;
int word=0;
for(p=str;*p!='\0';p++)
if(*p==' ')
word++;
cout<<"一共有"<<word+1<<"个单词"<<endl;*/
char str[100];
gets(str);//gets可以接受连续输入,包括空格和制表符
// cin>>str;
char *p;
int word=0;
for(p=str;*p!='\0';p++)
{ if(*p==' ')
word++;
}
cout<<"一共有"<<word+1<<"个单词"<<endl;
return 0;
}
投票系统
7.3
#include<iostream>
#include<string.h>
using namespace std;
struct person
{
char name[20];
int count;
};
int main()
{
person Person[3]={ {"张三",0}, {"刘六",0}, {"苏武",0}};
char name[10];
int i,j;
cout<<"请输入唱票过程:\n";
for(i=0;i<10;i++)//计数
{
cin>>name;
for(j=0;j<3;j++)
{
if(strcmp(Person[j].name,name)==0)
{
Person[j].count++;
}
}
}
for(i=0;i<3;i++)
{
//printf("%s:%d票\n",Person[i].name,Person[i].count);
cout<<Person[i].name<<":"<<Person[i].count<<"票"<<endl;//输出
}
return 0;
}
面包信息
7.4
#include<iostream>
using namespace std;
struct tastes //结构体
{
char taste1[10]; //口味1
char taste2[10]; //口味2
char taste3[10]; //口味3
};
struct Information //面包基本信息结构体
{
char name[30]; //品牌名
int price; //零售价格
int weight; //重量
struct tastes sweet; //口味
}information = { "桃李",5,350,{ "原味","紫薯味","水果味" } }; //为结构变量初始化
//Information information = { "桃李",5,350,{ "原味","紫薯味","水果味" } };
int main()
{
cout<<"基本信息:\n";
cout<<"品牌:"<< information.name<<endl; //输出结构体information成员数据
cout<<"零售价:"<<information.price<<"元"<<endl;
cout<<"重量:"<<information.weight<<"g"<<endl;
cout<<"口味种类:\n";
cout<<"口味1:"<<information.sweet.taste1<<endl;//输出结构体tastes成员数据
cout<<"口味2:"<<information.sweet.taste2<<endl;
cout<<"口味3:"<<information.sweet.taste3<<endl;
return 0;
}
统计数字出现的次数
7.5
/**
统计各数字出现的次数
*/
#include<iostream> /*包含头文件*/
using namespace std;
int main() /*主函数main*/
{
int i, a[10], b[10] = { 0 }; /*定义数组,数组a中放数据,b统计次数*/
cout<<"请输入10个0~9的数组元素:\n";
for (i = 0; i < 10; i++) /*逐个输入数组元素*/
{
cin>>a[i];
}
cout<<"----------------------\n";
for (i = 0; i < 10; i++) /*循环数组中的每个值*/
b[a[i]]++; /*统计出现的次数*/
for (i = 0; i < 10; i++) /*从0-9依次循环*/
cout<<i<<"出现的次数"<<b[i]<<endl; /*显示输出次数结果*/
cout<<"----------------------\n";
return 0; /*程序结束*/
}
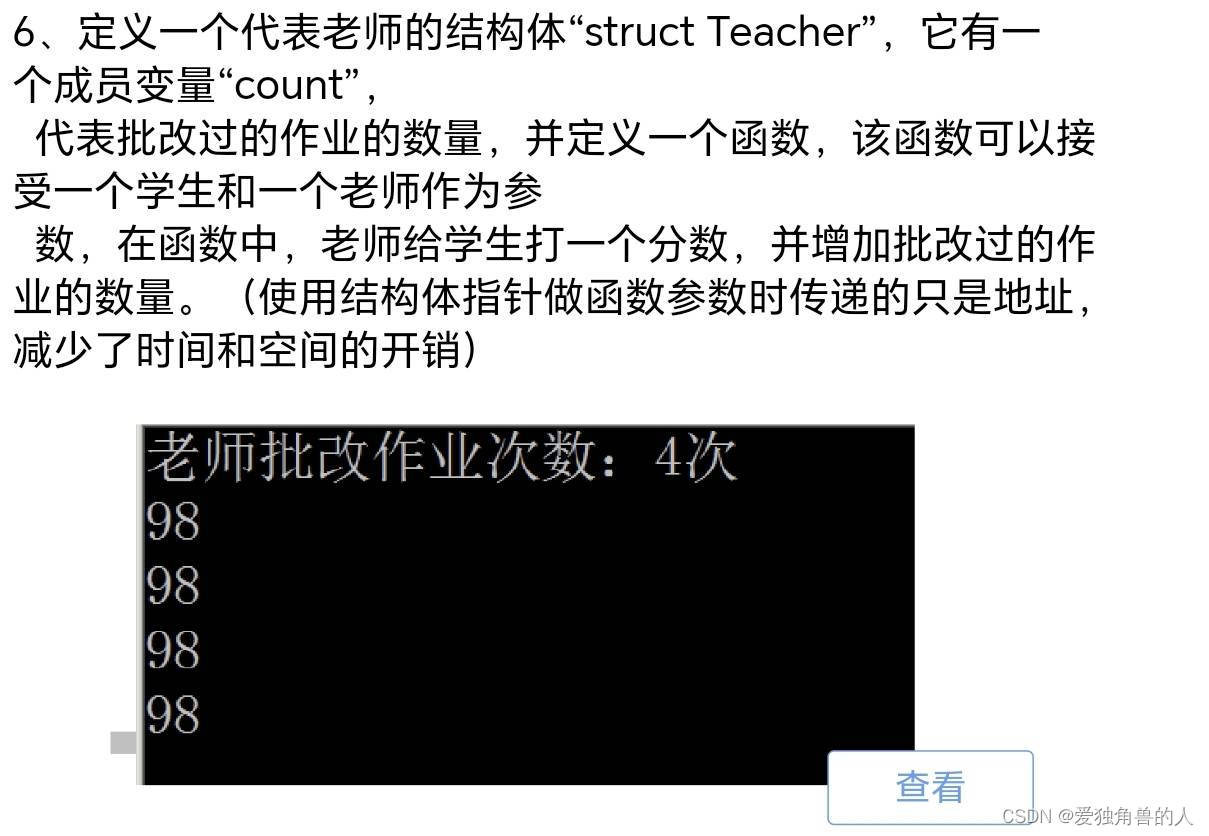
7.6
/*
定义一个代表老师的结构体“struct Teacher”,它有一个成圆变量“count”,
代表批改过的作业的数量,并定义一个函数,该函数可以接受一个学生和一个老师作为参
数,在函数中,老师给学生打一个分数,并增加批改过的作的数量。
*/
#include <iostream>
using namespace std;
struct Teacher {//定义结构体
int count;
};
struct Student {//定义结构体
int score;
};
void Check(Teacher *pTeacher, Student *pStudent) {
int n;
cin>>n;
pStudent->score = n;
pTeacher->count ++;
}
using namespace std;
int main()
{
Teacher t = {0};
Student s1,s2,s3,s4;
Check(&t, &s1);
Check(&t, &s2);
Check(&t, &s3);
Check(&t, &s4);
cout <<"老师批改作业次数:"<< t.count<<"次" << "\n";
cout << s1.score << endl;
cout << s2.score << endl;
cout << s3.score << endl;
cout << s4.score << endl;
return 0;
}
7.7
#include<iostream>
#include<string>
#include "7.h"
using namespace std;
int main(){
student s1;
s1.set();
s1.display();
return 0;
}
"77.h"
#include<bits/stdc++.h>
using namespace std;
class Student{
private:
string no;
string name;
string sex;
int age;
public:
void set();
void display();
};
void Student::set()
{
cin>>no;
cin>>name;
cin>>sex;
cin>>age;
}
void Student::display()
{
cout<<no<<endl;
cout<<name<<endl;
cout<<sex<<endl;
cout<<age;
}