1.面向对象的初步认知
1.1什么是面向对象
java是一门纯面向对象的语言,在面向对象的世界里,一切皆为对象.面向对象是解决问题的一种思想,主要依靠对象之间的交互来完成一件事.
1.2面向对象与面向过程的区别
举个例子:
1.传统洗衣服过程
传统的方式: 注重的是洗衣服的过程,少了一个环节都不行.
而且不同衣服洗的方式,时间长度,拧干方式都不同,处理起来就比较麻烦。如果将来要洗鞋子,那就是另 一种放方式。
总共有四个对象: 人 , 衣服 , 洗衣粉 , 洗衣机
整个洗衣服过程: 人将衣服发放进洗衣机,倒入洗衣粉,启动洗衣机,洗衣机就会完成洗衣服过程并且甩干
整个过程主要是: 人 , 衣服 , 洗衣粉 , 洗衣机四个对象之间交互完成的,人不需要关心洗衣机具体是如何洗衣服的,是如何甩干的
2.类的定义和使用
2.1简单认识类
2.2类的定义格式
//创建类 class Test { field;//成员变量(属性) method;//成员属性或方法 } class为定义类的关键字,ClassName为类的名字,{}中为类的主体。
class WashMachine{ public String brand;//品牌 public String type;//型号 public double weight;//长度 public double width;//宽 public double height;//高 public String color;//颜色 public void washClothes(){ //洗衣服 System.out.println("洗衣功能"); } public void dryClothes(){ //脱水 System.out.println("脱水功能"); } public void setTime(){ //定时 System.out.println("定时功能"); }
3.类的实例化
3.1什么是实例化?
定义了一个类,就相当于在计算机中定义了一种新的类型,与int,double类似,只不过int和double是java语言自带的内置类型,而类是用户自己定义的一个新的类型,比如上述的:WashMachine.有了自定义类型之后,就可以使用这些类来定义对象了.
class WashMachine{ public String brand;//品牌 public String type;//型号 public double weight;//长度 public double width;//宽 public double height;//高 public String color;//颜色 public void washClothes(){ //洗衣服 System.out.println("洗衣功能"); } public void dryClothes(){ //脱水 System.out.println("脱水功能"); } public void setTime(){ //定时 System.out.println("定时功能"); } public static void main(String[] args) { WashMachine washMachine1 = new WashMachine();//通过new实例化对象 washMachine1.brand = "小天鹅"; washMachine1.color = "黑色"; washMachine1.dryClothes(); System.out.println(washMachine1.brand); System.out.println(washMachine1.color); System.out.println("============================="); WashMachine washMachine2 = new WashMachine(); washMachine2.brand = "海尔"; washMachine2.color = "白色"; washMachine2.dryClothes(); System.out.println(washMachine2.brand); System.out.println(washMachine2.color); } } //输出结果: 脱水功能 小天鹅 黑色 ============================= 脱水功能 海尔 白色
3.2 类和对象的说明
4.this引用
4.1为什么要有this引用
class Date { public int year; public int month; public int day; public void setDate(int y, int m,int d) { year = y; month = m; day = d; } public void printDate(){ System.out.println(year + "/" + month + "/" + day); } public static void main(String[] args) { //构造三个日期类型的对象d1 d2 d3 Date d1 = new Date(); Date d2 = new Date(); Date d3 = new Date(); d1.setDate(2024,7,19); d2.setDate(2024,7,20); d3.setDate(2024,7,21); d1.printDate(); d2.printDate(); d3.printDate(); }
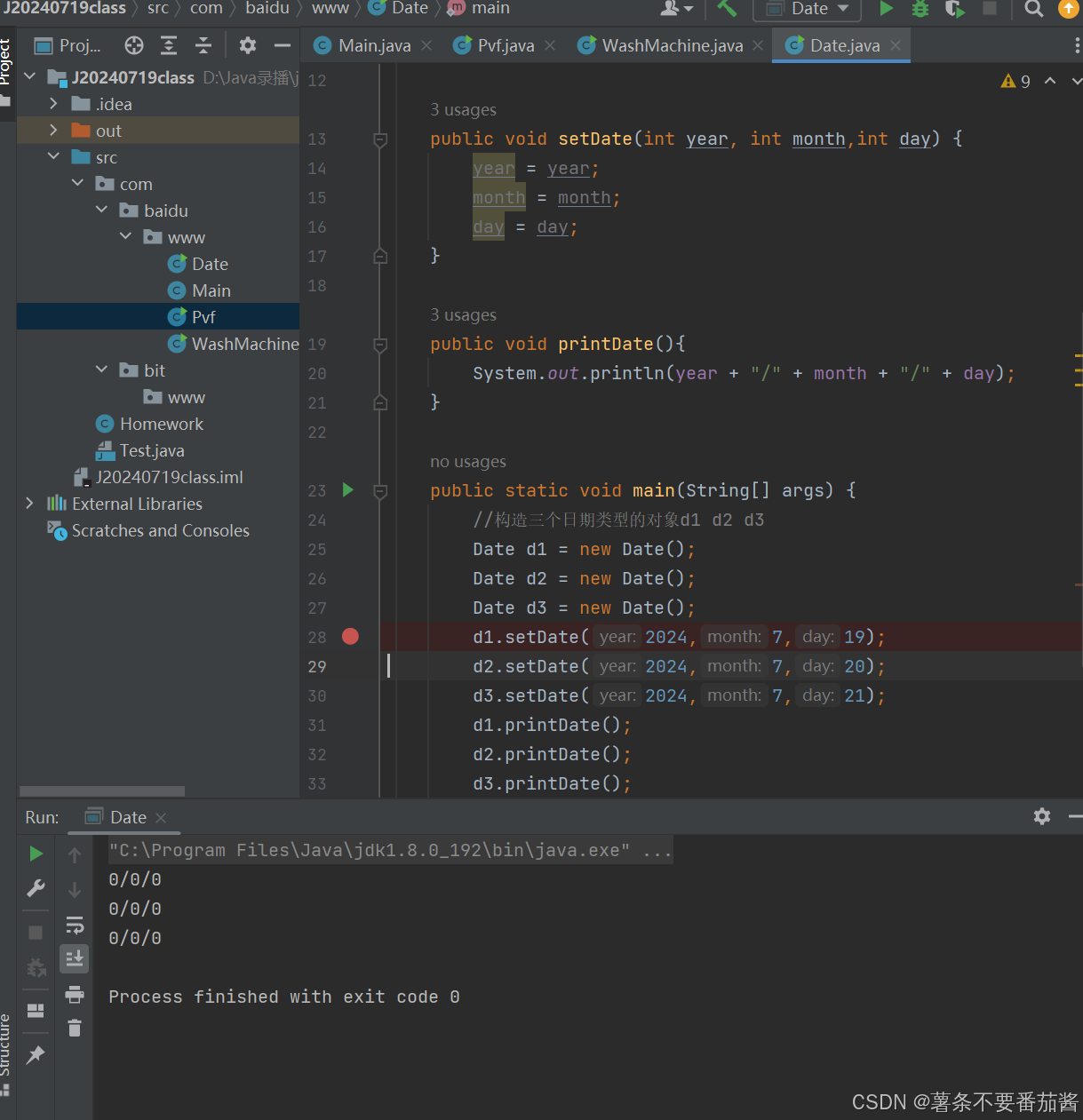
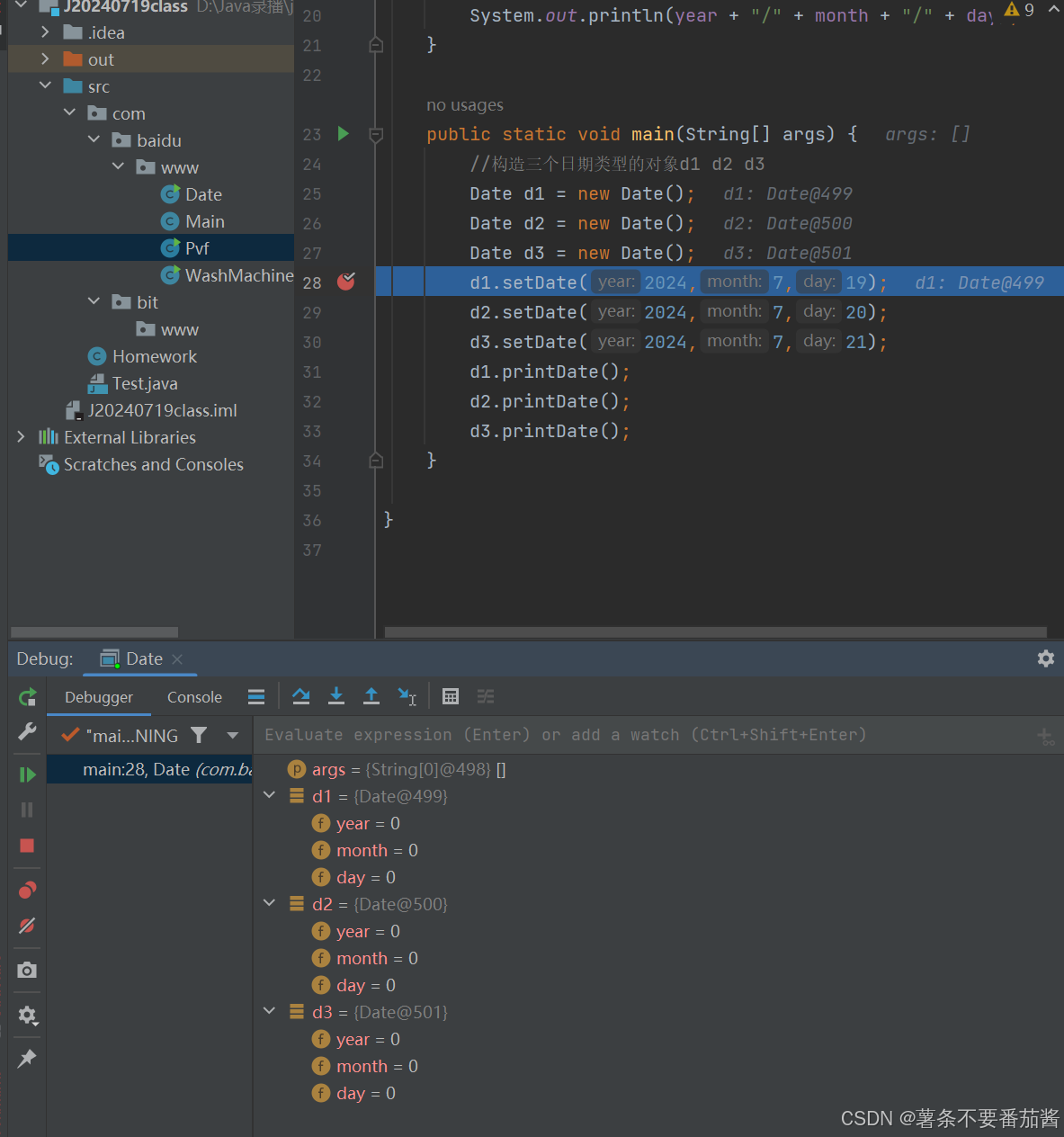
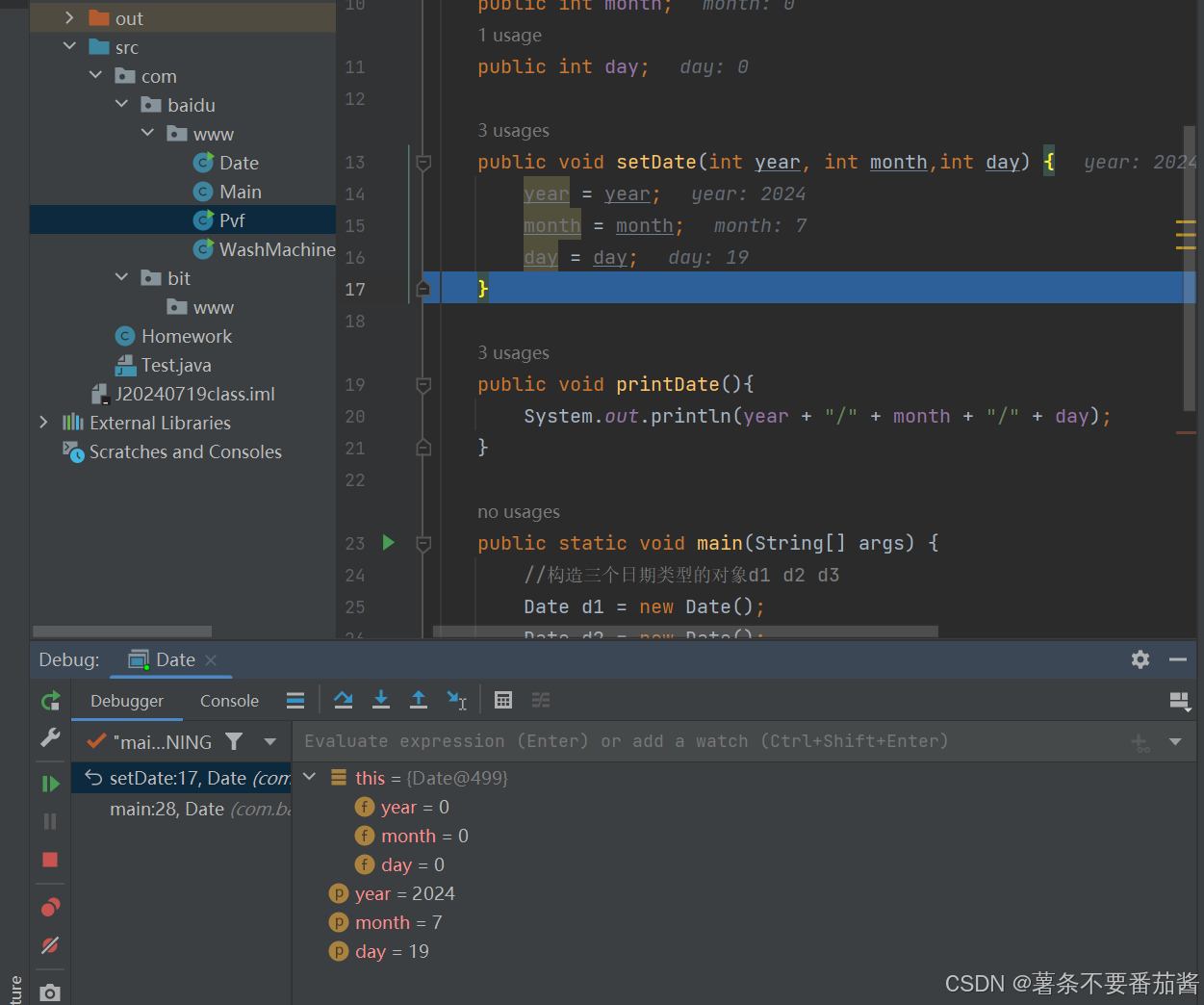
4.2什么是this引用
this引用指向当前对象(成员方法运行时调用该成员方法的对象),在成员方法中所有成员变量的操作,都是通过该引用去访问。只不过所有的操作对用户是透明的,即用户不需要来传递,编译器自动完成。
public class Date { public int year; public int month; public int day; public void setDate(int year, int month,int day) { this.year = year; this.month = month; this.day = day; }
可以发现,利用this引用4.1的问题成功得到解决
4.3this引用的特性
1.this的类型:对应类类型引用,即哪个对象调用就是哪个对象的引用类型
2.this只能在"成员方法"中使用
3.在"成员方法"中,this只能引用当前对象,不能再引用其他对象
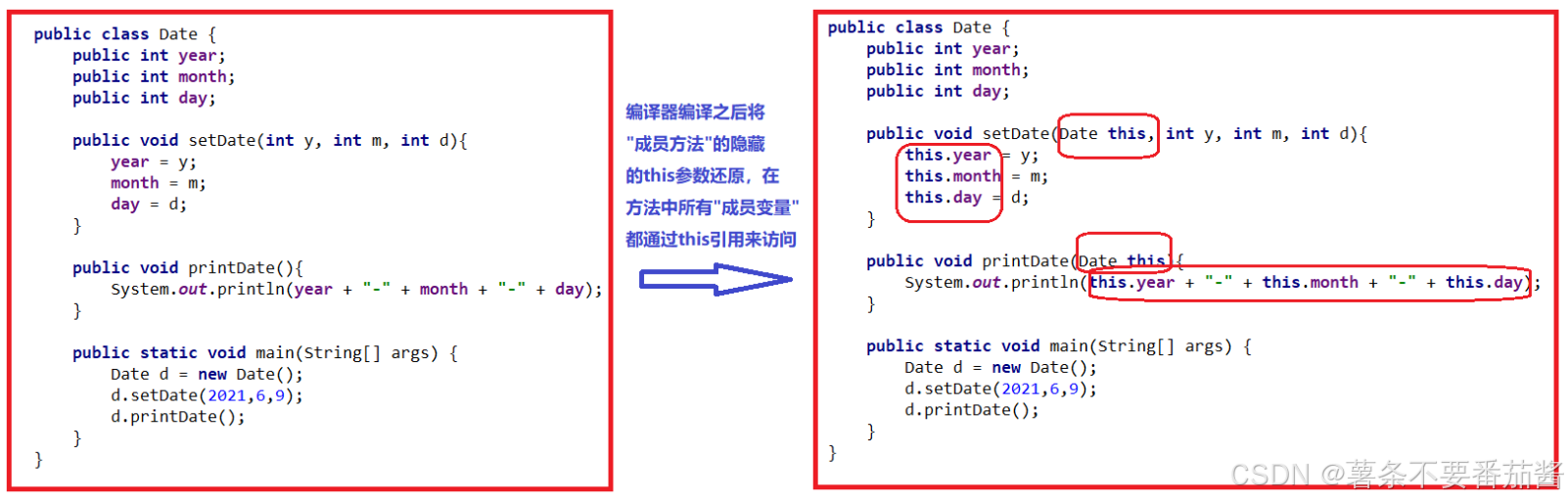
5.对象的构造及初始化
5.1如何初始化对象
public class Main { public static void main(String[] args) { double d; System.out.println(d); } }//编译错误:java: 可能尚未初始化变量d
public static void main(String[] args) { //继上述Date类 Date d1 = new Date(); d1.printDate(); d1.setDate(2024,7,19); d1.printDate(); }//代码可以正常运行
5.2构造方法
5.2.1概念
public class Date { public int year; public int month; public int day; //构造方法 //名字与类名相同,没有返回值类型,设置为void也不行 //一般情况下使用public修饰 //在创建对象时由编译器自动调用,并且在对象的生命周期内只调用一次 public Date(int year, int month,int day) { this.year = year; this.month = month; this.day = day; System.out.println("Date(int,int,int)方法被调用了"); } public void printDate(){ System.out.println(year + "/" + month + "/" + day); } public static void main(String[] args) { //构造三个日期类型的对象d1 d2 d3 Date d1 = new Date(2020,7,19); d1.printDate(); } }
5.2.2特性
public class Date { public int year; public int month; public int day; //构造方法 //名字与类名相同,没有返回值类型,设置为void也不行 //一般情况下使用public修饰 //在创建对象时由编译器自动调用,并且在对象的生命周期内只调用一次 public Date(){ this.year = 2003; this.month = 11; this.day = 25; } public Date(int year, int month,int day) { this.year = year; this.month = month; this.day = day; System.out.println("Date(int,int,int)方法被调用了"); } public void printDate(){ System.out.println(year + "/" + month + "/" + day); } public static void main(String[] args) { //构造三个日期类型的对象d1 d2 d3 Date d1 = new Date();//此时调用的是无参Date() d1.printDate(); } }
public class Date { public int year; public int month; public int day; //构造方法 //名字与类名相同,没有返回值类型,设置为void也不行 //一般情况下使用public修饰 //在创建对象时由编译器自动调用,并且在对象的生命周期内只调用一次 public void printDate(){ System.out.println(year + "/" + month + "/" + day); } public static void main(String[] args) { //构造三个日期类型的对象d1 d2 d3 Date d1 = new Date();//此时调用的是无参Date() d1.printDate(); } }
public class Date { public int year; public int month; public int day; // 无参构造方法--内部给各个成员赋初始值,该部分功能与三个参数的构造方法重复 // 此处可以在无参构造方法中通过this调用带有三个参数的构造方法 // 但是this(1900,1,1);必须是构造方法中第一条语句 public Date(){ this(1970,10,25); } //带有三个参数的构造方法 public Date(int year,int month,int day){ this.year = year; this.month = month; this.day = day; } public void printDate(){ System.out.println(year + "/" + month + "/" + day); } }
public Date(){ this(1970,10,25); } //带有三个参数的构造方法 public Date(int year,int month,int day){ this(); }//编译错误:java: 递归构造器调用
5.3默认初始化
在上文中提出的第二个问题:为什么局部变量在使用时必须要初始化,而成员变量可以不用初始化?
public class Date { public int year; public int month; public int day; public Date(int year, int month, int day) { // 成员变量在定义时,并没有给初始值, 为什么就可以使用呢? System.out.println(this.year); System.out.println(this.month); System.out.println(this.day); } public static void main(String[] args) { // 此处a没有初始化,编译时报错: // Error:(24, 28) java: 可能尚未初始化变量a // int a; // System.out.println(a); Date d = new Date(2021,6,9); } }
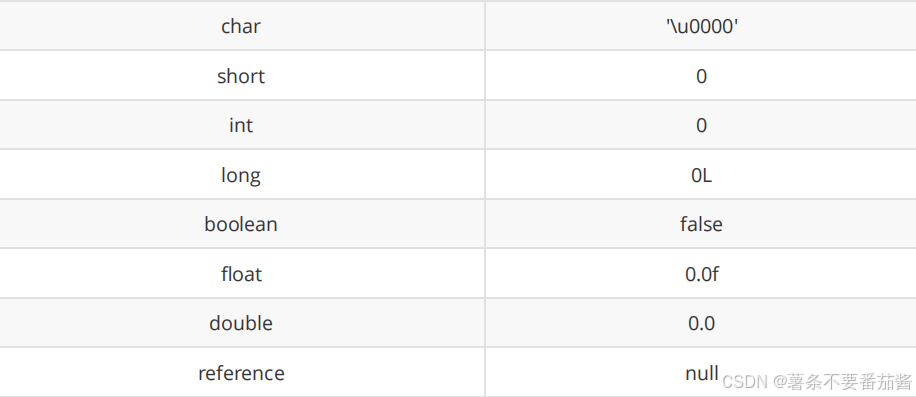
6.封装
6.1概念
面向对象程序的三大特性:封装,继承,多态.而类和对象阶段,主要研究的就是封装特性.那么什么是封装呢?简单来说就是套壳屏蔽细节.
6.2访问限定符
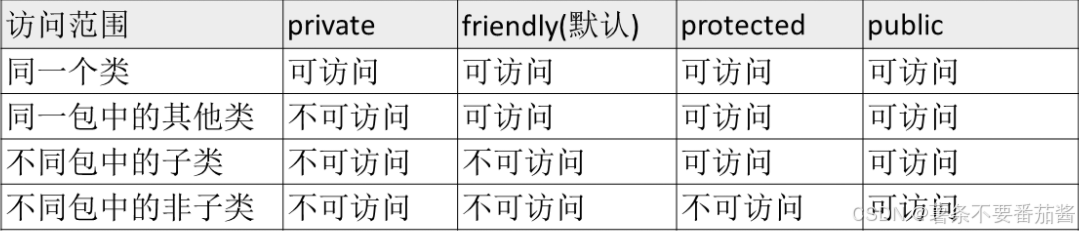
6.3封装扩展之包
6.3.1包的概念
6.3.2导入包中的类
public class Test { public static void main(String[] args) { java.util.Date date = new java.util.Date(); System.out.println(date.getTime()); } }
import java.util.Date; public class Test { public static void main(String[] args) { Date date = new Date(); System.out.println(date.getTime()); } }
import java.util.*; public class Test { public static void main(String[] args) { Date date = new Date(); System.out.println(date.getTime()); } }
import java.util.*; import java.sql.*; public class Test { public static void main(String[] args) { // util 和 sql 中都存在一个 Date 这样的类, 此时就会出现歧义, 编译出错 Date date = new Date(); System.out.println(date.getTime()); } }
import java.util.*; import java.sql.*; public class Test { public static void main(String[] args) { java.util.Date date = new java.util.Date(); System.out.println(date.getTime()); } }
import static java.lang.Math.*; public class Test { public static void main(String[] args) { double x = 30; double y = 40; // 静态导入的方式写起来更方便一些. //double result = Math.sqrt(Math.pow(x, 2) + Math.pow(y, 2)); double result = sqrt(pow(x, 2) + pow(y, 2)); System.out.println(result); } }
6.3.3自定义包
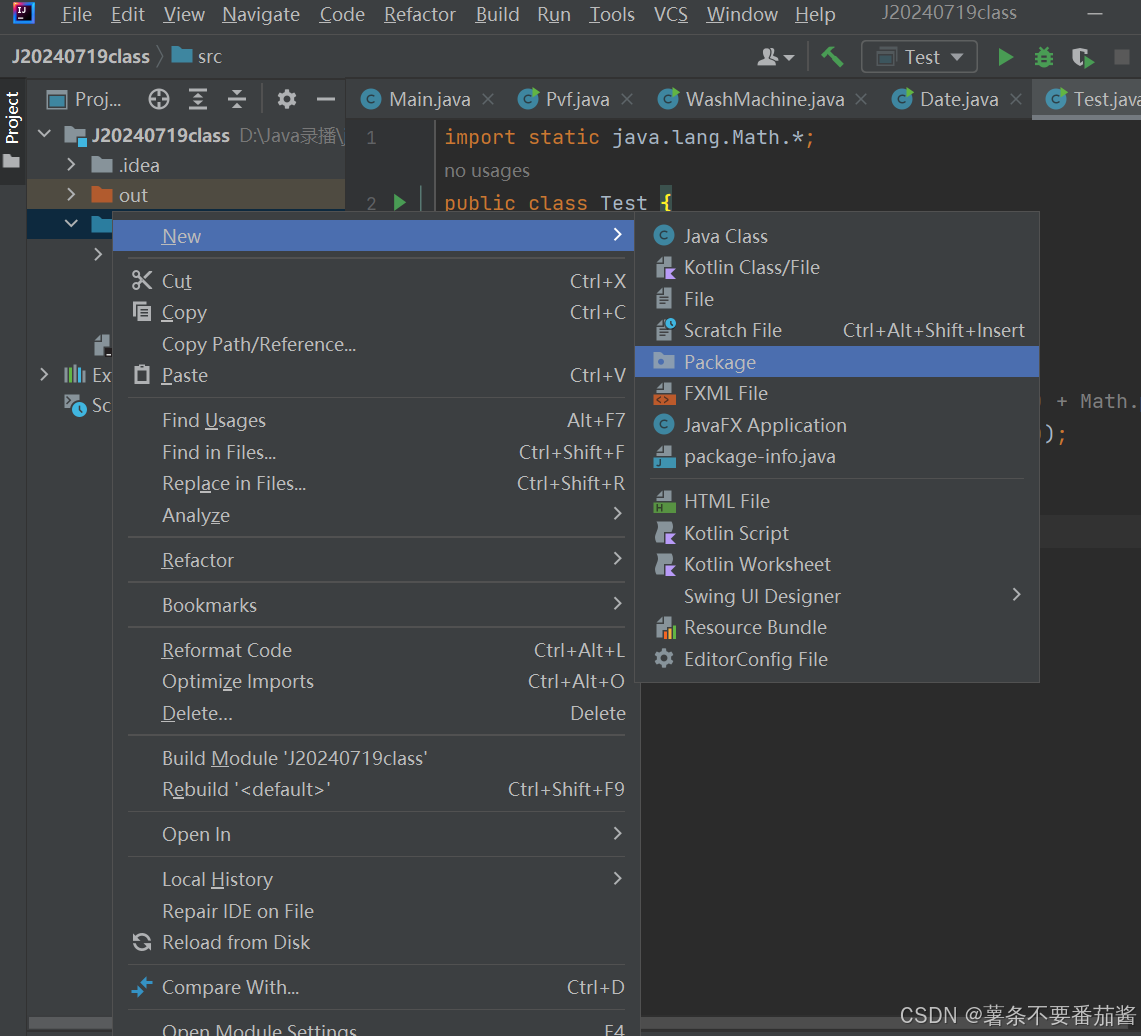
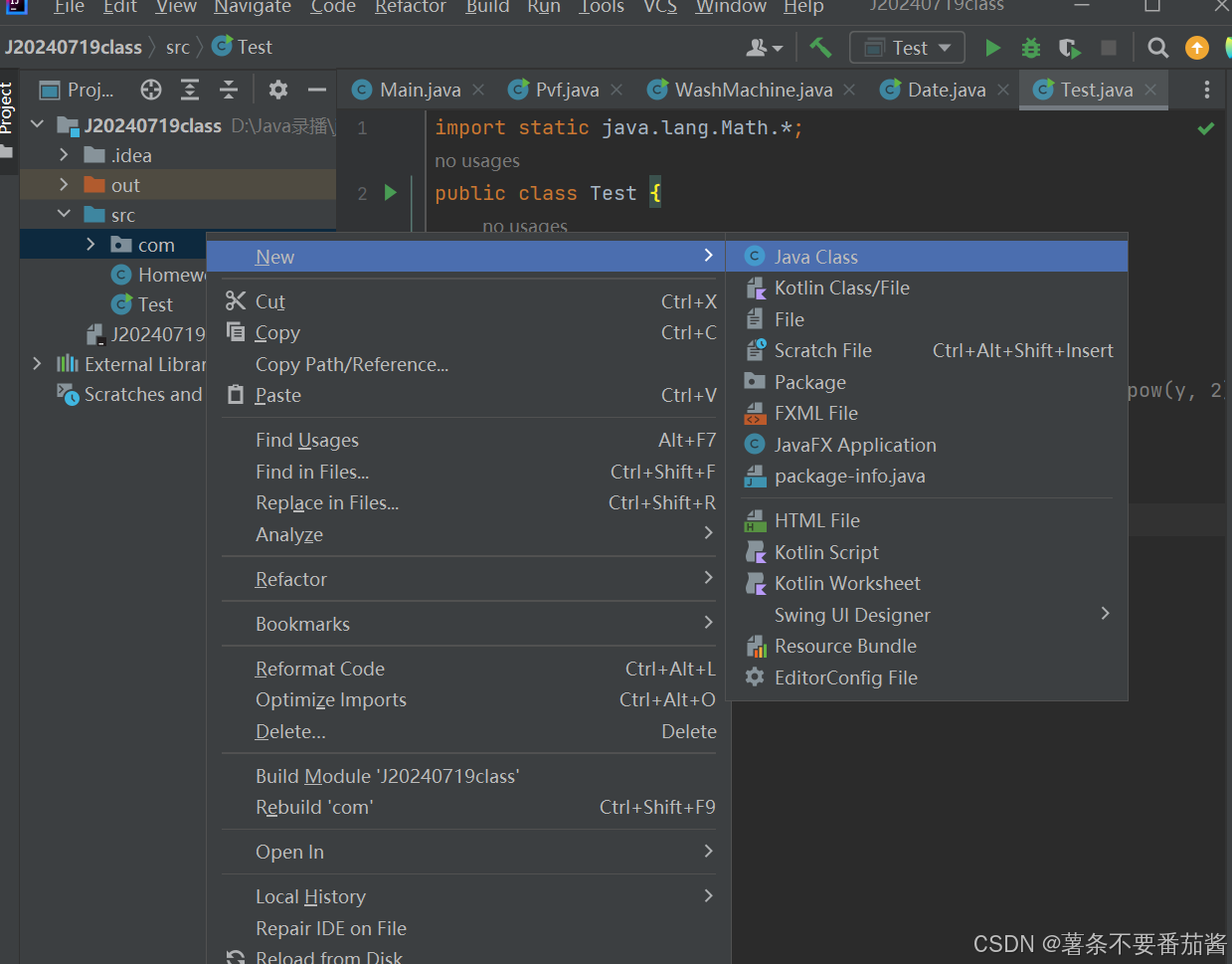
6.3.5常见的包
7.static
7.1 static修饰成员变量
public class Student { public String name; public String gender; public int age; public double score; public static String classRoom = "306"; public Student(String name, String gender, int age, double score) { this.name = name; this.gender = gender; this.age = age; this.score = score; } public static void main(String[] args) { //静态成员变量可以直接通过类名访问 System.out.println(Student.classRoom); Student s1 = new Student("chen","男",34,4.3); Student s2 = new Student("wu","男",19,4.29); Student s3 = new Student("shi","女",19,3.0); //也可以通过对象访问: 但classRoom是三个对象共享的 System.out.println(s1.classRoom); System.out.println(s2.classRoom); System.out.println(s3.classRoom); } }
7.2static修饰成员方法
public class Student { private String name; private String gender; private int age; private double score; private static String classRoom = "306"; public Student(String name, String gender, int age, double score) { this.name = name; this.gender = gender; this.age = age; this.score = score; } } public class TestStudent { public static void main(String[] args) { System.out.println(Student.classRoom); } }//编译错误:java: classRoom 在 com.baidu.Student 中是 private 访问控制
public class Student { private String name; private String gender; private int age; private double score; private static String classRoom = "306"; public static String getClassRoom(){ return classRoom; } }
public class TestStudent { public static void main(String[] args) { System.out.println(Student.getClassRoom()); } }
public static String getClassRoom(){
System.out.println(this);
return classRoom;
}//编译错误:java: 无法从静态上下文中引用非静态 变量 this
public static String getClassRoom(){
age += 1;
return classRoom;
}//编译错误:java: 无法从静态上下文中引用非静态 变量 age
4. 静态方法中不能调用任何非静态方法,因为非静态方法有this参数,在静态方法中调用时候无法传递this引用
7.3static成员变量初始化
静态成员变量的初始化分为两种:就地初始化 和 静态代码块初始化。
1.就地初始化
private static String classRoom = "306";
2.静态代码块初始化
继续往下看↓↓
8.代码块
8.1概念及分类
8.2普通代码块
public class Main { public static void main(String[] args) { { //直接使用{}定义,普通方法块 int x = 10 ; System.out.println("x1 = " +x); } int x = 100 ; System.out.println("x2 = " +x); } }//输出x1 = 10 x2 = 100 //这种用法较少见
8.3构造代码块
public class Student{ //实例成员变量 private String name; private String gender; private int age; private double score; public Student() { System.out.println("I am Student init()!"); } //实例代码块 { this.name = "bit"; this.age = 12; this.gender = "man"; System.out.println("I am instance init()!"); } public void show(){ System.out.println("name: "+name+" age: "+age+" sex: "+gender); } } // 运行结果 /*I am instance init()! I am Student init()! name: bit age: 12 sex: man*/