#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 10
#define OVERFLOW -1
typedef struct {
char* base;
char* top;
int stacksize;
} SqStack;
// 初始化栈
int InitStack(SqStack* S) {
S->base = (char*)malloc(MAXSIZE * sizeof(char));
if (!S->base)
return OVERFLOW;
S->top = S->base;
S->stacksize = MAXSIZE;
return 1;
}
// 入栈
int Push(SqStack* S, int e) {
if (S->top - S->base == S->stacksize) {
printf("栈已满!\n");
return 0;
}
*S->top++ = e;
return 1;
}
// 出栈
int Pop(SqStack* S, int* e) {
if (S->top == S->base) {
printf("栈为空!\n");
return 0;
}
*e = *--S->top;
return 1;
}
// 当ai不为-1时,将ai进栈;当ai=-1时,输出栈顶整数并出栈
void Yes_No(SqStack* S, int a) {
if (a == -1) {
int e;
Pop(S, &e);
printf("%d ", e);
} else {
Push(S, a);
}
}
int main() {
SqStack S;
InitStack(&S);
printf("请输入一整数序列(以*结尾):");
int a;
while (scanf("%d", &a) && a != '*') {
Yes_No(&S, a);
}
printf("\n栈中元素为:");
while (S.top != S.base) {
int e;
Pop(&S, &e);
printf("%d", e);
}
free(S.base); // 释放栈空间
return 0;
}
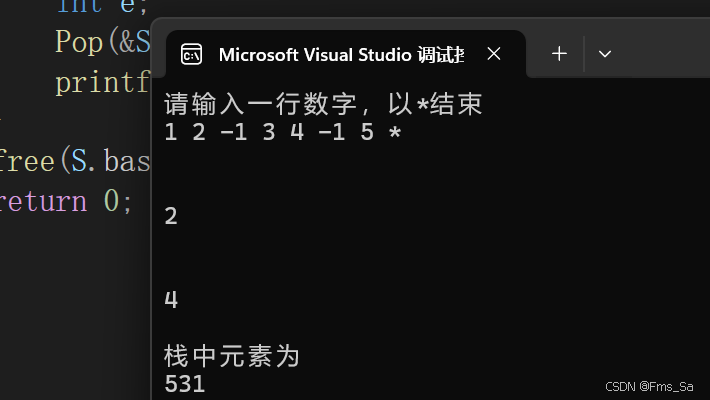