class MinesweeperAI():
def __init__(self, height=8, width=8):
# 设置初始高度和宽度
self.height = height
self.width = width
# 跟踪点击了哪些单元格
self.moves_made = set()
# 跟踪已知安全或地雷的细胞
self.mines = set()
self.safes = set()
# 关于已知为真游戏的句子列表
self.knowledge = []
将一个单元格标记为地雷,并更新所有知识以将该单元格也标记为地雷。
def mark_mine(self, cell):
self.mines.add(cell)
for sentence in self.knowledge:
sentence.mark_mine(cell)
将一个单元格标记为安全,并更新所有知识以将该单元格也标记为安全。
def mark_safe(self, cell):
self.safes.add(cell)
for sentence in self.knowledge:
sentence.mark_safe(cell)
用于获取所有附近的单元格
def nearby_cells(self, cell):
cells = set()
for i in range(cell[0] - 1, cell[0] + 2):
for j in range(cell[1] - 1, cell[1] + 2):
if (i, j) == cell:
continue
if 0 <= i < self.height and 0 <= j < self.width:
cells.add((i, j))
return cells
当扫雷板告诉我们,对于给定的安全单元,有多少相邻单元中有地雷时调用。
这个功能应该:
1)将单元格标记为已进行的移动
2)将单元格标记为安全
3)根据 cell
和 count
的值在 AI 的知识库中添加一个新句子
4)如果可以根据 AI 的知识库得出结论,则将任何其他单元格标记为安全或地雷
5) 如果可以从现有知识中推断出任何新句子,则将其添加到 AI 的知识库中
def add_knowledge(self, cell, count):
self.moves_made.add(cell)
# 标记单元格安全
if cell not in self.safes:
self.mark_safe(cell)
# 获取所有附近的单元格
nearby = self.nearby_cells(cell)
nearby -= self.safes | self.moves_made
new_sentence = Sentence(nearby, count)
self.knowledge.append(new_sentence)
new_safes = set()
new_mines = set()
for sentence in self.knowledge:
if len(sentence.cells) == 0:
self.knowledge.remove(sentence)
else:
tmp_new_safes = sentence.known_safes()
tmp_new_mines = sentence.known_mines()
if type(tmp_new_safes) is set:
new_safes |= tmp_new_safes
if type(tmp_new_mines) is set:
new_mines |= tmp_new_mines
for safe in new_safes:
self.mark_safe(safe)
for mine in new_mines:
self.mark_mine(mine)
prev_sentence = new_sentence
new_inferences = []
for sentence in self.knowledge:
if len(sentence.cells) == 0:
self.knowledge.remove(sentence)
elif prev_sentence == sentence:
break
elif prev_sentence.cells <= sentence.cells:
inf_cells = sentence.cells - prev_sentence.cells
inf_count = sentence.count - prev_sentence.count
new_inferences.append(Sentence(inf_cells, inf_count))
prev_sentence = sentence
self.knowledge += new_inferences
def make_safe_move(self):
**返回一个安全的单元格以在扫雷板上选择。必须知道该移动是安全的,而不是已经做出的移动。
该函数可以使用 self.mines、self.safes 和 self.moves_made 中的知识,但不应修改任何这些值。**
def make_safe_move(self):
safe_moves = self.safes.copy()
safe_moves -= self.moves_made
if len(safe_moves) == 0:
return None
return safe_moves.pop()
def make_random_move(self):
返回在扫雷板上进行的移动。应该在以下单元格中随机选择:
**1) 尚未被选中
- 不知道是地雷**
def make_random_move(self):
if len(self.moves_made) == 56:
return None
random_move = random.randrange(self.height), random.randrange(self.height)
not_safe_moves = self.moves_made | self.mines
while random_move in not_safe_moves:
random_move = random.randrange(self.height), random.randrange(self.height)
return random_move
============================================================================
颜色
BLACK = (0, 0, 0)
GRAY = (180, 180, 180)
WHITE = (255, 255, 255)
创建游戏
pygame.init()
size = width, height = 600, 400
screen = pygame.display.set_mode(size)
字体
字体可以在自己电脑中C:\Windows\Fonts
的位置选择自己喜欢的复制到项目中 assets/fonts目录下即可,我用的是楷体
OPEN_SANS = "assets/fonts/simkai.ttf"
smallFont = pygame.font.Font(OPEN_SANS, 20)
mediumFont = pygame.font.Font(OPEN_SANS, 28)
largeFont = pygame.font.Font(OPEN_SANS, 40)
计算面板尺寸
BOARD_PADDING = 20
board_width = ((2 / 3) * width) - (BOARD_PADDING * 2)
board_height = height - (BOARD_PADDING * 2)
cell_size = int(min(board_width / WIDTH, board_height / HEIGHT))
board_origin = (BOARD_PADDING, BOARD_PADDING)
添加图片
这里我们只用了两张图,一个是地雷,一个是用来标记地雷的旗帜
flag = pygame.image.load("assets/images/flag.png")
flag = pygame.transform.scale(flag, (cell_size, cell_size))
mine = pygame.image.load("assets/images/mine.png")
mine = pygame.transform.scale(mine, (cell_size, cell_size))
创建游戏和 AI 代理
game = Minesweeper(height=HEIGHT, width=WIDTH, mines=MINES)
ai = MinesweeperAI(height=HEIGHT, width=WIDTH)
跟踪显示的单元格、标记的单元格以及是否被地雷击中
revealed = set()
flags = set()
lost = False
最初显示游戏说明
instructions = True
while True:
# 检查游戏是否退出
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
screen.fill(BLACK)
# 显示游戏说明
if instructions:
# 标题
title = largeFont.render("海拥 | 扫雷", True, WHITE)
titleRect = title.get_rect()
titleRect.center = ((width / 2), 50)
screen.blit(title, titleRect)
# Rules
rules = [
"单击一个单元格以显示它",
"右键单击一个单元格以将其标记为地雷",
"成功标记所有地雷以获胜!"
]
for i, rule in enumerate(rules):
line = smallFont.render(rule, True, WHITE)
lineRect = line.get_rect()
lineRect.center = ((width / 2), 150 + 30 * i)
screen.blit(line, lineRect)
# 开始游戏按钮
buttonRect = pygame.Rect((width / 4), (3 / 4) * height, width / 2, 50)
buttonText = mediumFont.render("开始游戏", True, BLACK)
buttonTextRect = buttonText.get_rect()
buttonTextRect.center = buttonRect.center
pygame.draw.rect(screen, WHITE, buttonRect)
screen.blit(buttonText, buttonTextRect)
# 检查是否点击播放按钮
click, _, _ = pygame.mouse.get_pressed()
if click == 1:
mouse = pygame.mouse.get_pos()
if buttonRect.collidepoint(mouse):
instructions = False
time.sleep(0.3)
pygame.display.flip()
continue
画板
cells = []
for i in range(HEIGHT):
row = []
for j in range(WIDTH):
# 为单元格绘制矩形
rect = pygame.Rect(
board_origin[0] + j * cell_size,
board_origin[1] + i * cell_size,
cell_size, cell_size
)
pygame.draw.rect(screen, GRAY, rect)
pygame.draw.rect(screen, WHITE, rect, 3)
# 如果需要,添加地雷、旗帜或数字
if game.is_mine((i, j)) and lost:
screen.blit(mine, rect)
elif (i, j) in flags:
screen.blit(flag, rect)
elif (i, j) in revealed:
neighbors = smallFont.render(
str(game.nearby_mines((i, j))),
True, BLACK
)
neighborsTextRect = neighbors.get_rect()
neighborsTextRect.center = rect.center
screen.blit(neighbors, neighborsTextRect)
row.append(rect)
cells.append(row)
AI 移动按钮
aiButton = pygame.Rect(
(2 / 3) * width + BOARD_PADDING, (1 / 3) * height - 50,
(width / 3) - BOARD_PADDING * 2, 50
)
buttonText = mediumFont.render("AI 移动", True, BLACK)
buttonRect = buttonText.get_rect()
buttonRect.center = aiButton.center
pygame.draw.rect(screen, WHITE, aiButton)
screen.blit(buttonText, buttonRect)
重置按钮
resetButton = pygame.Rect(
(2 / 3) * width + BOARD_PADDING, (1 / 3) * height + 20,
(width / 3) - BOARD_PADDING * 2, 50
)
buttonText = mediumFont.render("重置", True, BLACK)
buttonRect = buttonText.get_rect()
buttonRect.center = resetButton.center
pygame.draw.rect(screen, WHITE, resetButton)
screen.blit(buttonText, buttonRect)
显示文字
text = "失败" if lost else "获胜" if game.mines == flags else ""
text = mediumFont.render(text, True, WHITE)
textRect = text.get_rect()
textRect.center = ((5 / 6) * width, (2 / 3) * height)
screen.blit(text, textRect)
move = None
left, _, right = pygame.mouse.get_pressed()
检查右键单击以切换标记
if right == 1 and not lost:
mouse = pygame.mouse.get_pos()
for i in range(HEIGHT):
for j in range(WIDTH):
if cells[i][j].collidepoint(mouse) and (i, j) not in revealed:
if (i, j) in flags:
flags.remove((i, j))
else:
flags.add((i, j))
time.sleep(0.2)
elif left == 1:
mouse = pygame.mouse.get_pos()
如果单击 AI 按钮,则进行 AI 移动
if aiButton.collidepoint(mouse) and not lost:
move = ai.make_safe_move()
if move is None:
move = ai.make_random_move()
if move is None:
flags = ai.mines.copy()
print("No moves left to make.")
else:
print("No known safe moves, AI making random move.")
else:
print("AI making safe move.")
time.sleep(0.2)
重置游戏状态
elif resetButton.collidepoint(mouse):
game = Minesweeper(height=HEIGHT, width=WIDTH, mines=MINES)
ai = MinesweeperAI(height=HEIGHT, width=WIDTH)
revealed = set()
flags = set()
lost = False
**自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。**
**深知大多数Python工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!**
**因此收集整理了一份《2024年Python开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。**
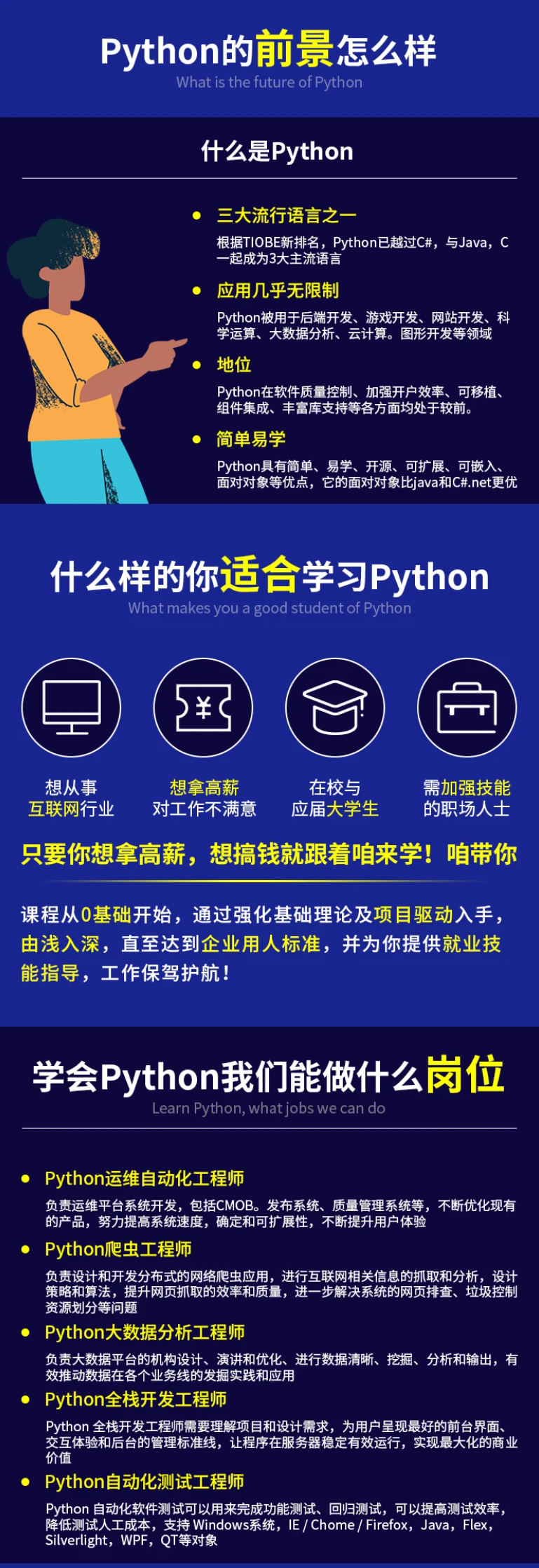
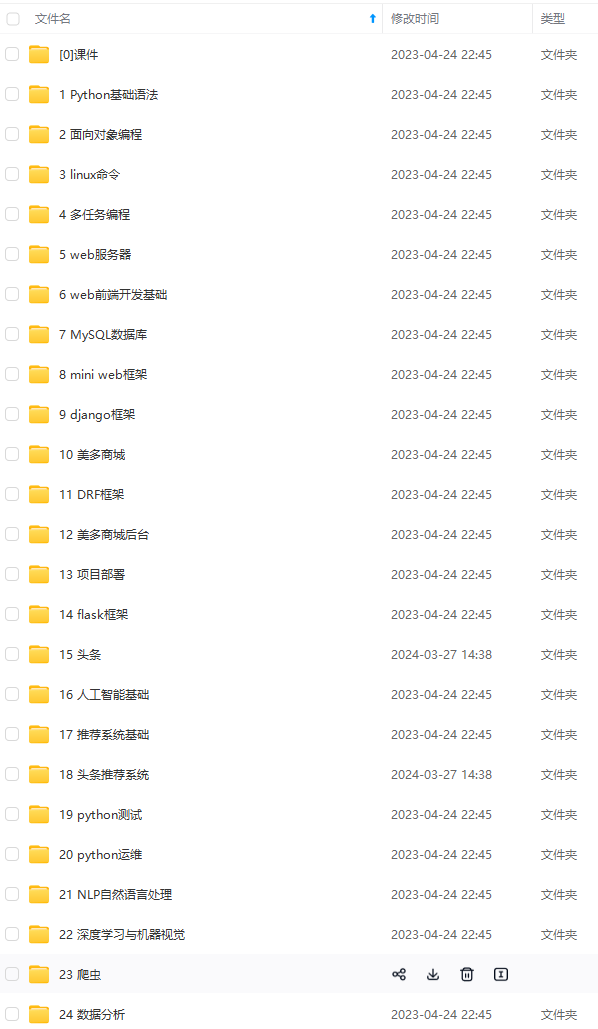
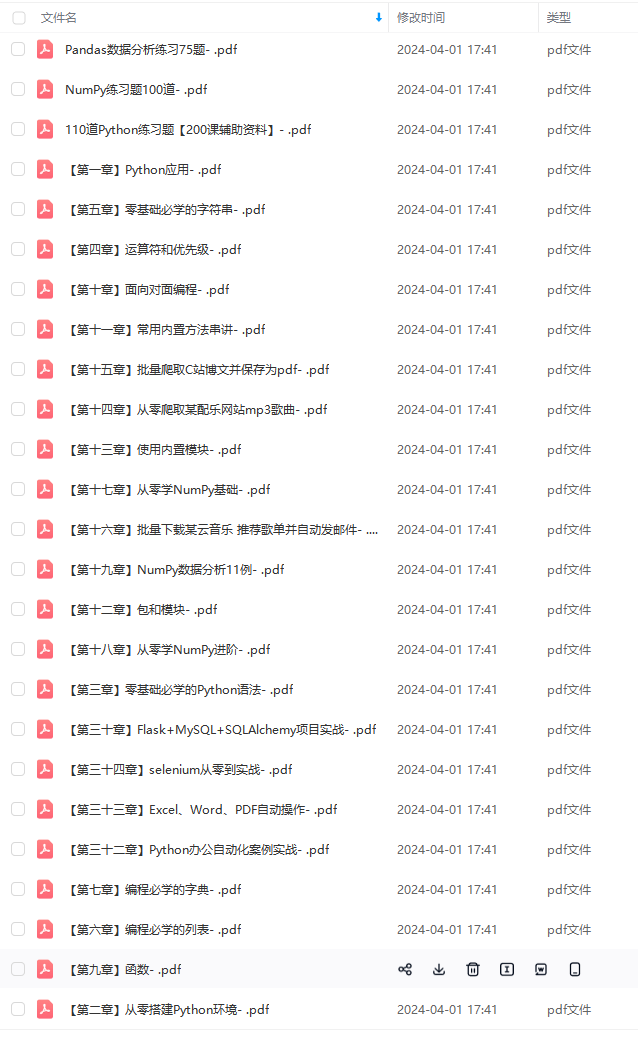
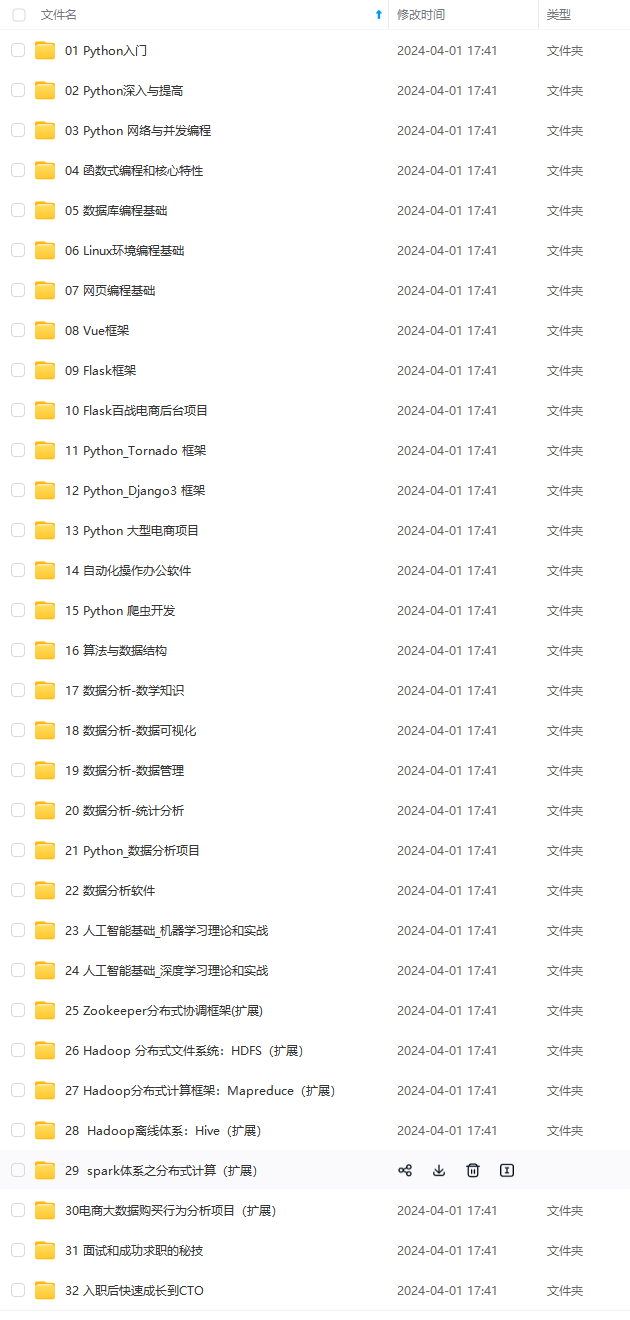
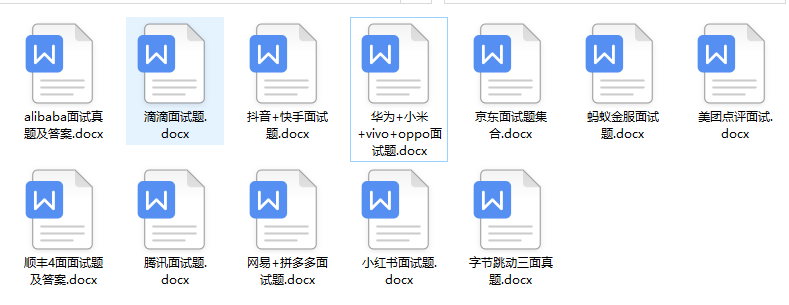
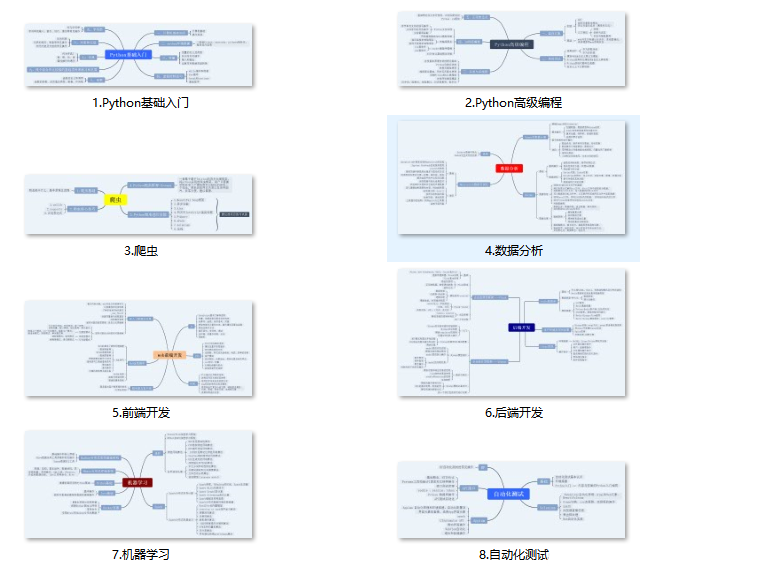
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上前端开发知识点,真正体系化!**
**由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新**
**如果你觉得这些内容对你有帮助,可以扫码获取!!!(备注:Python)**
TH)
revealed = set()
flags = set()
lost = False
**自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。**
**深知大多数Python工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!**
**因此收集整理了一份《2024年Python开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。**
[外链图片转存中...(img-5m2veHDI-1713755597538)]
[外链图片转存中...(img-FVQ7u77K-1713755597539)]
[外链图片转存中...(img-kGmjhulL-1713755597540)]
[外链图片转存中...(img-ANWbjkNg-1713755597540)]
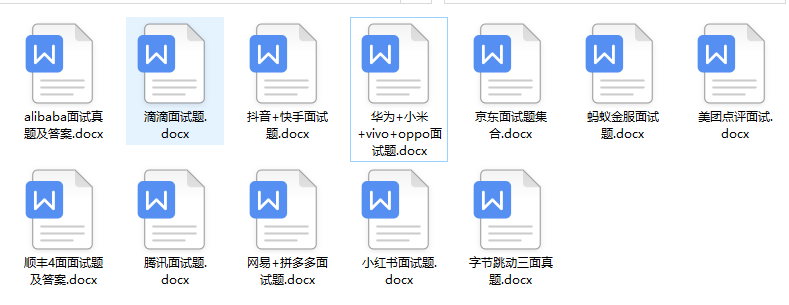
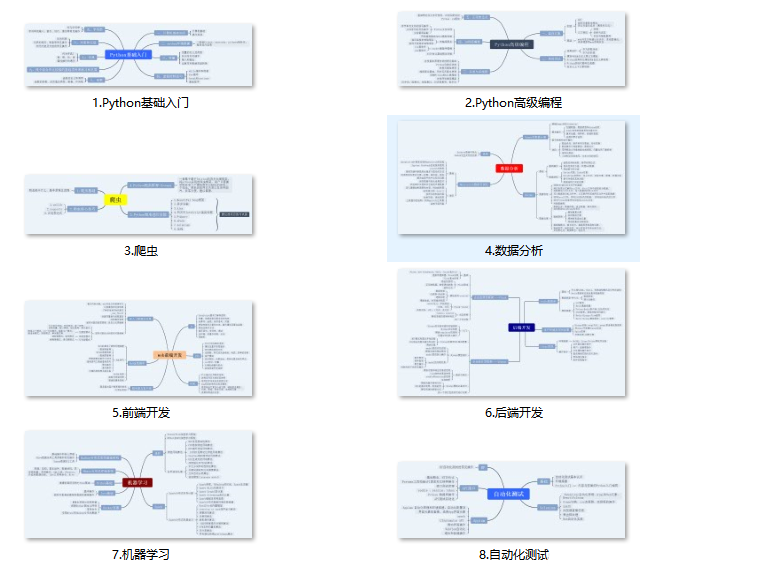
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上前端开发知识点,真正体系化!**
**由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新**
**如果你觉得这些内容对你有帮助,可以扫码获取!!!(备注:Python)**
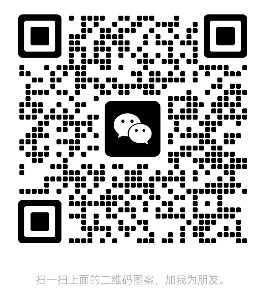