自行创建controller,mapper,service(impl)等包
controller
@RestController
public class OrderController {
@Autowired
OrderService orderService;
@GetMapping("/test")
public void test(){
Integer test = orderService.test();
System.out.println(test);
}
}
mapper
@Mapper
public interface OrderMapper {
Integer test();
}
service
public interface OrderService {
Integer test ();
}
serviceImpl
@Service
public class OrderServiceImpl implements OrderService {
@Autowired
OrderMapper orderMapper;
@Override
public Integer test() {
return orderMapper.test();
}
}
mappers下的映射文件 记得修改语句喔
<?xml version="1.0" encoding="UTF-8"?> select count(*) from root.ln.wf01.wt01 ```
大致结构如下
接下来运行访问即可得到结果
方案二:使用IotSession
注意:使用此方法就先把mybatis的依赖注释掉不然没有注入数据库会报错
配置文件如下
#iotdb
spring:
iotdb:
ip: 192.168.110.10
port: 6667
user: root
password: root
fetchSize: 10000
maxActive: 10
server:
port: 8080
在config包下创建配置文件
@Configuration
public class IotConfigSession {
@Value("${spring.iotdb.ip}")
private String ip;
@Value("${spring.iotdb.port}")
private int port;
@Value("${spring.iotdb.user}")
private String user;
@Value("${spring.iotdb.password}")
private String password;
@Value("${spring.iotdb.fetchSize}")
private int fetchSize;
@Bean
public Session iotSession(){
Session session = new Session(ip, port, user, password, fetchSize);
try {
session.open();
} catch (IoTDBConnectionException e) {
throw new RuntimeException(e);
}
return session;
}
}
创建controller
小伙伴们也可以自己写一些简单的测试用例 直接用session去 “.” 就行
可以不用在以下方法里使用
Session session = iotConfigSession.iotSession();
可以直接用iotConfigSession去做,我这样做只是因为要实现不同的session连接去测试压力
@RestController
public class TestController {
@Autowired
private IotConfigSession iotConfigSession ;
//批量插入 第一个参数是每次插入多少 第二个是插入总量
@GetMapping("insert/{BATCH_INSERT_SIZE}/{TOTAL_INSERT_ROW_COUNT}")
public String insertTablet(@PathVariable int BATCH_INSERT_SIZE,@PathVariable int TOTAL_INSERT_ROW_COUNT) throws IoTDBConnectionException, StatementExecutionException {
// 设置设备名字,设备下面的传感器名字,各个传感器的类型
Session session = iotConfigSession.iotSession();
List<MeasurementSchema> schemaList = new ArrayList<>();
//指定物理量(字段也叫属性) 传感器并 设置类型
schemaList.add(new MeasurementSchema("status", TSDataType.BOOLEAN));
schemaList.add(new MeasurementSchema("temperature", TSDataType.DOUBLE));
schemaList.add(new MeasurementSchema("speed", TSDataType.INT64));
/* 默认前两级是storage group maxRowNumber 单次插入多少条*/
Tablet tablet = new Tablet("root.ln.wf01.wt01", schemaList, BATCH_INSERT_SIZE);
// 以当前时间戳作为插入的起始时间戳
long timestamp = System.currentTimeMillis();
long beginTime = System.currentTimeMillis();
for (long row = 0; row < TOTAL_INSERT_ROW_COUNT; row++) {
int rowIndex = tablet.rowSize++;
tablet.addTimestamp(rowIndex, timestamp);//批量插入的时间戳是数组 rowIndex为下标
// 随机生成数据 物理量对应的值
tablet.addValue("status", rowIndex, (row & 1) == 0);
tablet.addValue("temperature", rowIndex, (double) row);
tablet.addValue("speed", rowIndex, row);
if (tablet.rowSize == tablet.getMaxRowNumber()) {//拼接到BATCH_INSERT_SIZE 后执行一次
long bg = System.currentTimeMillis();
session.insertTablet(tablet);
tablet.reset();
System.out.println("已经插入了:" + (row + 1) + "行数据 "+ "耗时:"+(System.currentTimeMillis()-bg));
}
timestamp++;
}
// 插入剩余不足 BATCH_INSERT_SIZE的数据再次插入一次 例如单次允许插入20行 有25条记录 就插入2次(20+5)
if (tablet.rowSize != 0) {
session.insertTablet(tablet);
System.out.println("最后插入"+tablet.rowSize);
tablet.reset();
}
long endTime =System.currentTimeMillis();
System.out.println("插入"+TOTAL_INSERT_ROW_COUNT+"条记录,每次插入"+BATCH_INSERT_SIZE+"总耗时"+(endTime-beginTime)+"ms");
// session.close();
return "插入"+TOTAL_INSERT_ROW_COUNT+"条记录,每次插入"+BATCH_INSERT_SIZE+"总耗时"+(endTime-beginTime)+"ms"+session;
}
//删除存储组
@GetMapping("deleteStorageGroup")
public String deleteStorageGroup() throws IoTDBConnectionException, StatementExecutionException {
Session session = iotConfigSession.iotSession();
session.deleteStorageGroup("root.ln");
// session.close();
return "删除存储组root.ln";
}
//批量插入 num是数量
@GetMapping("insertRecords/{num}")
## 最后
**自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。**
**深知大多数Java工程师,想要提升技能,往往是自己摸索成长,自己不成体系的自学效果低效漫长且无助。**
**因此收集整理了一份《2024年嵌入式&物联网开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。**
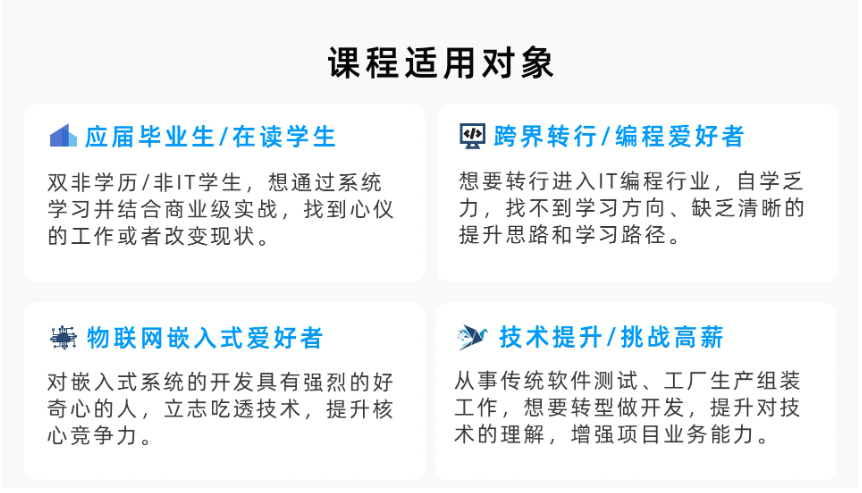
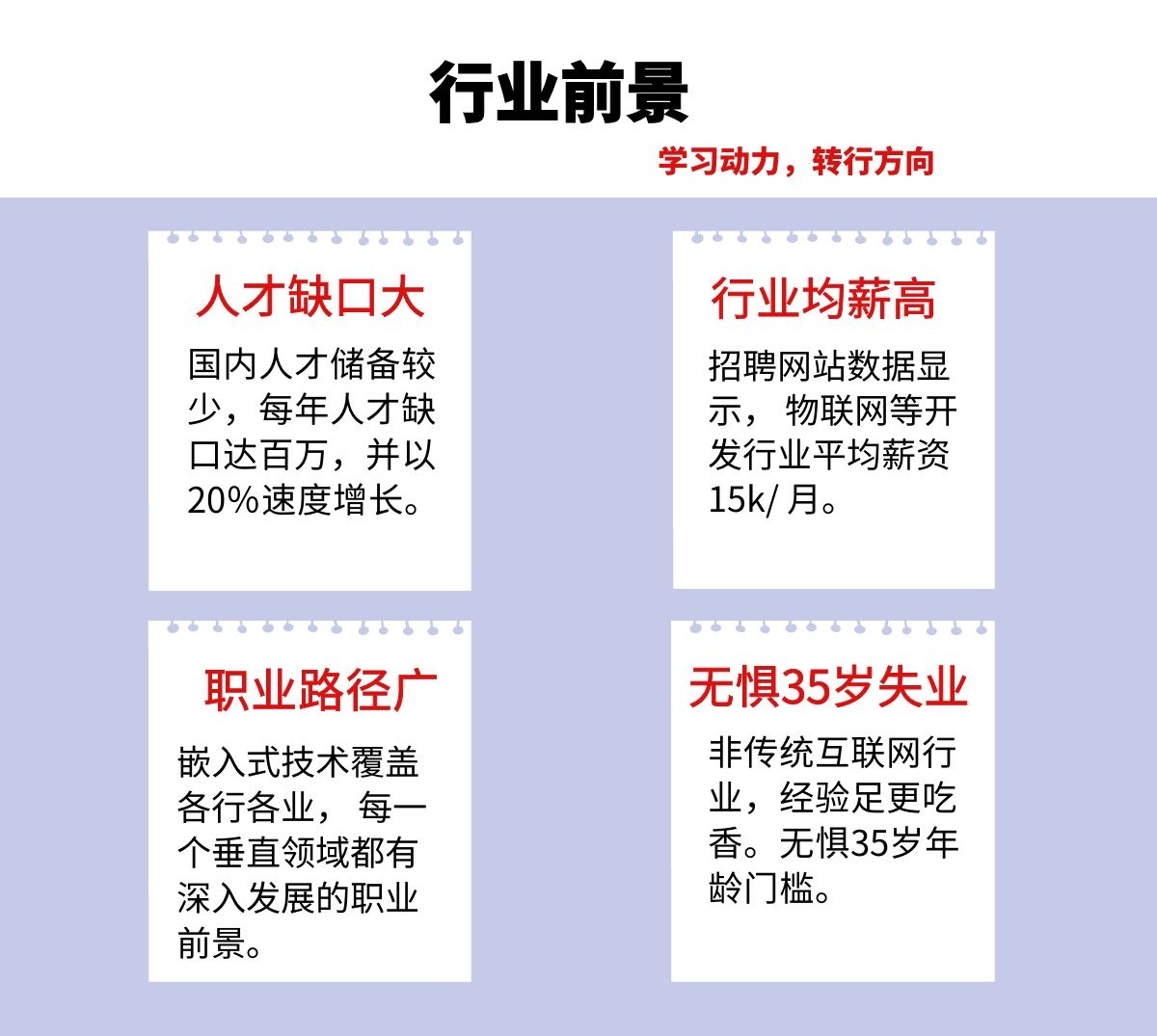
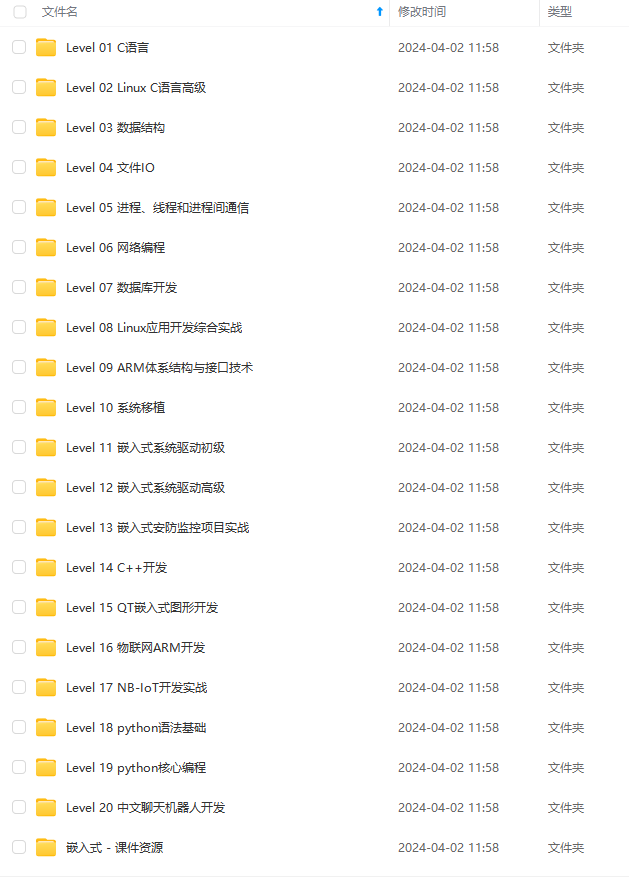
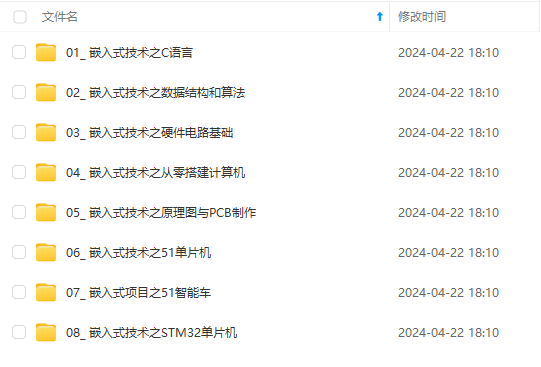
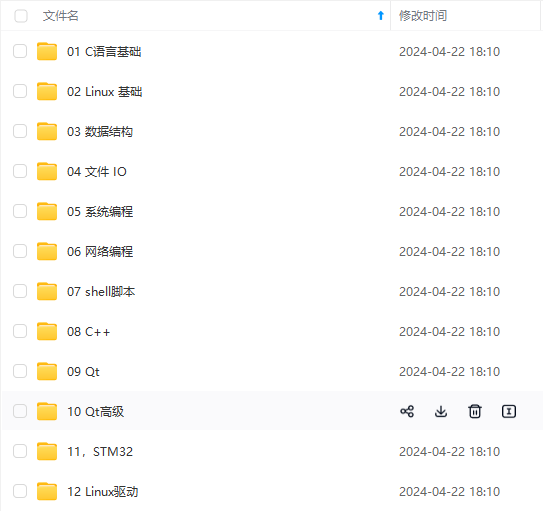
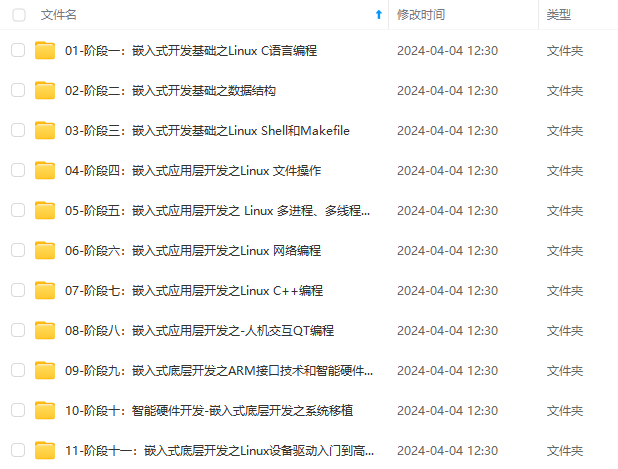
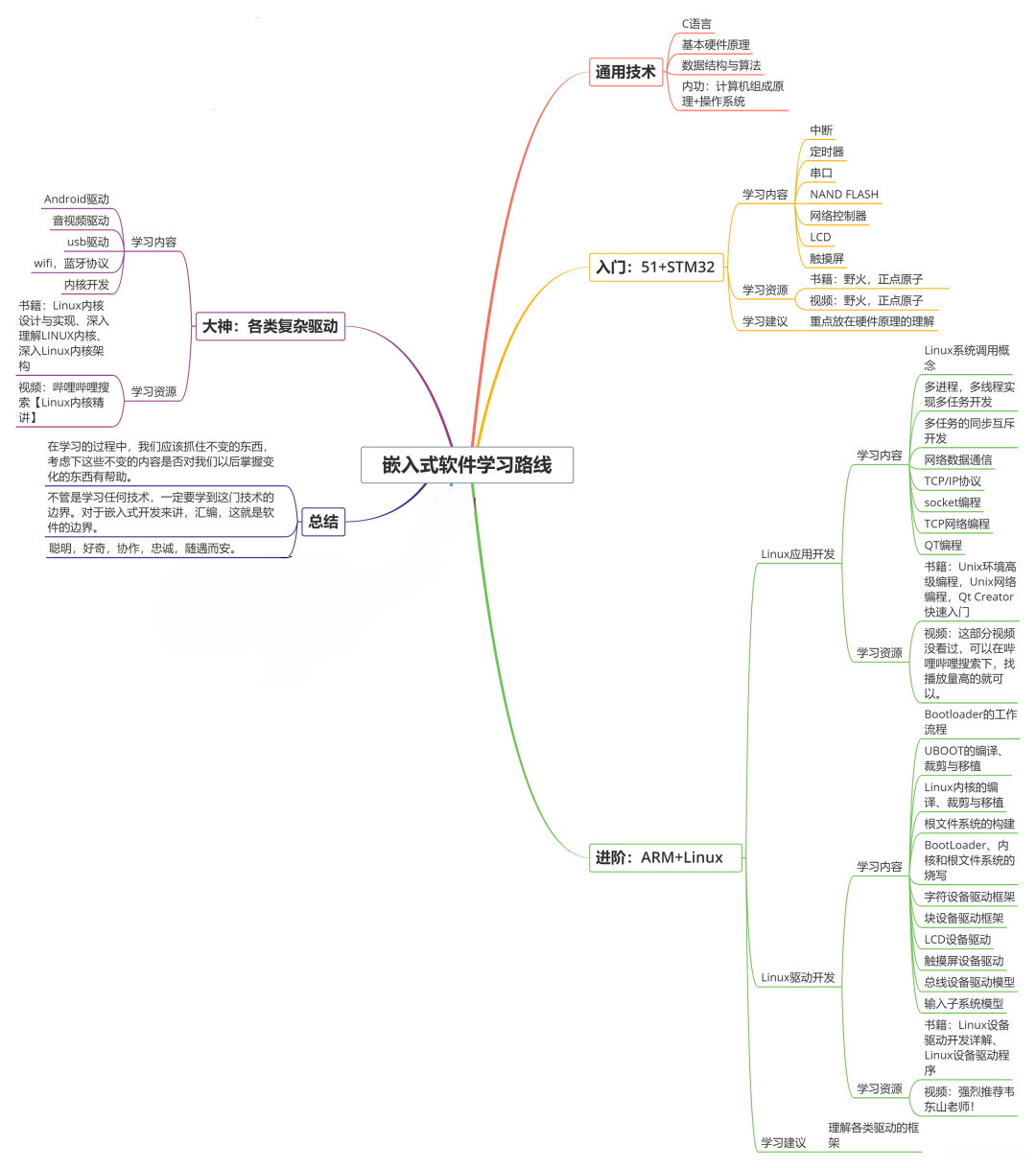
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上嵌入式&物联网开发知识点,真正体系化!**
[**如果你觉得这些内容对你有帮助,需要这份全套学习资料的朋友可以戳我获取!!**](https://bbs.csdn.net/topics/618654289)
**由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新**!!
8253749)]
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上嵌入式&物联网开发知识点,真正体系化!**
[**如果你觉得这些内容对你有帮助,需要这份全套学习资料的朋友可以戳我获取!!**](https://bbs.csdn.net/topics/618654289)
**由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新**!!