IoTDB连接信息
iotdb_host = “iotdb.example.com”
iotdb_port = 6667
iotdb_username = “your_username”
iotdb_password = “your_password”
设备配置表,用于映射设备字段和IoTDB表
device_config = {
“field1”: “table1”,
“field2”: “table2”,
# 添加更多字段和表的映射关系
}
MQTT消息处理函数
def on_message(client, userdata, msg):
# 解析接收到的消息
data = msg.payload.decode(“utf-8”)
topic = msg.topic
# 根据设备配置表映射字段和表
field = topic.split("/")[-1]
if field in device_config:
table = device_config[field]
# 连接到IoTDB数据库
connection = IoTDBConnection(iotdb_host, iotdb_port, iotdb_username, iotdb_password)
connection.open()
# 插入数据到IoTDB
sql = f"INSERT INTO {table} (timestamp, {field}) VALUES ({msg.timestamp}, {data})"
connection.execute(sql)
# 关闭连接
connection.close()
创建MQTT客户端
client = mqtt.Client()
client.username_pw_set(mqtt_username, mqtt_password)
设置消息处理函数
client.on_message = on_message
连接到MQTT服务器并订阅主题
client.connect(mqtt_broker, mqtt_port)
client.subscribe(mqtt_topic)
循环接收消息
client.loop_forever()
请根据您的实际情况修改代码中的相关参数,包括MQTT连接信息、IoTDB连接信息和设备配置表。确保您已安装所需的依赖库(paho-mqtt和pyiotdb)。
这是一个基本的示例,您可能需要根据您的具体需求进行进一步的修改和优化。希望对您有所帮助!如果您有任何进一步的问题,请随时告诉我。
**自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。**
**深知大多数嵌入式工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!**
**因此收集整理了一份《2024年嵌入式&物联网开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。**
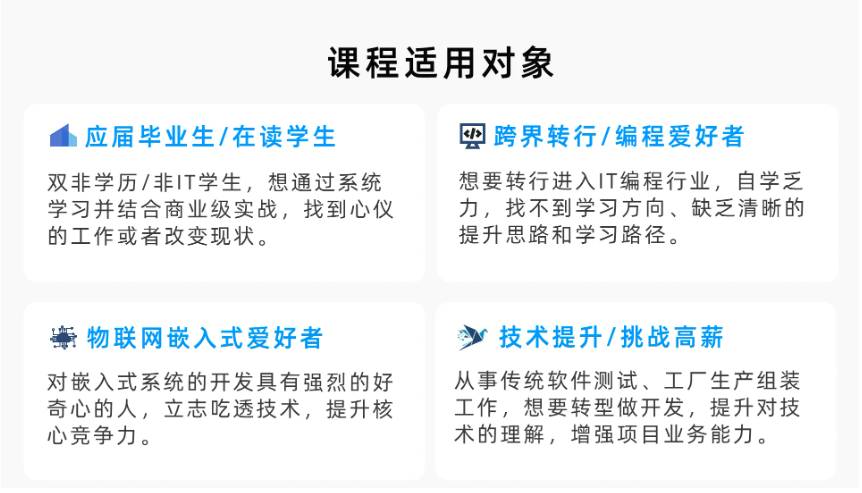
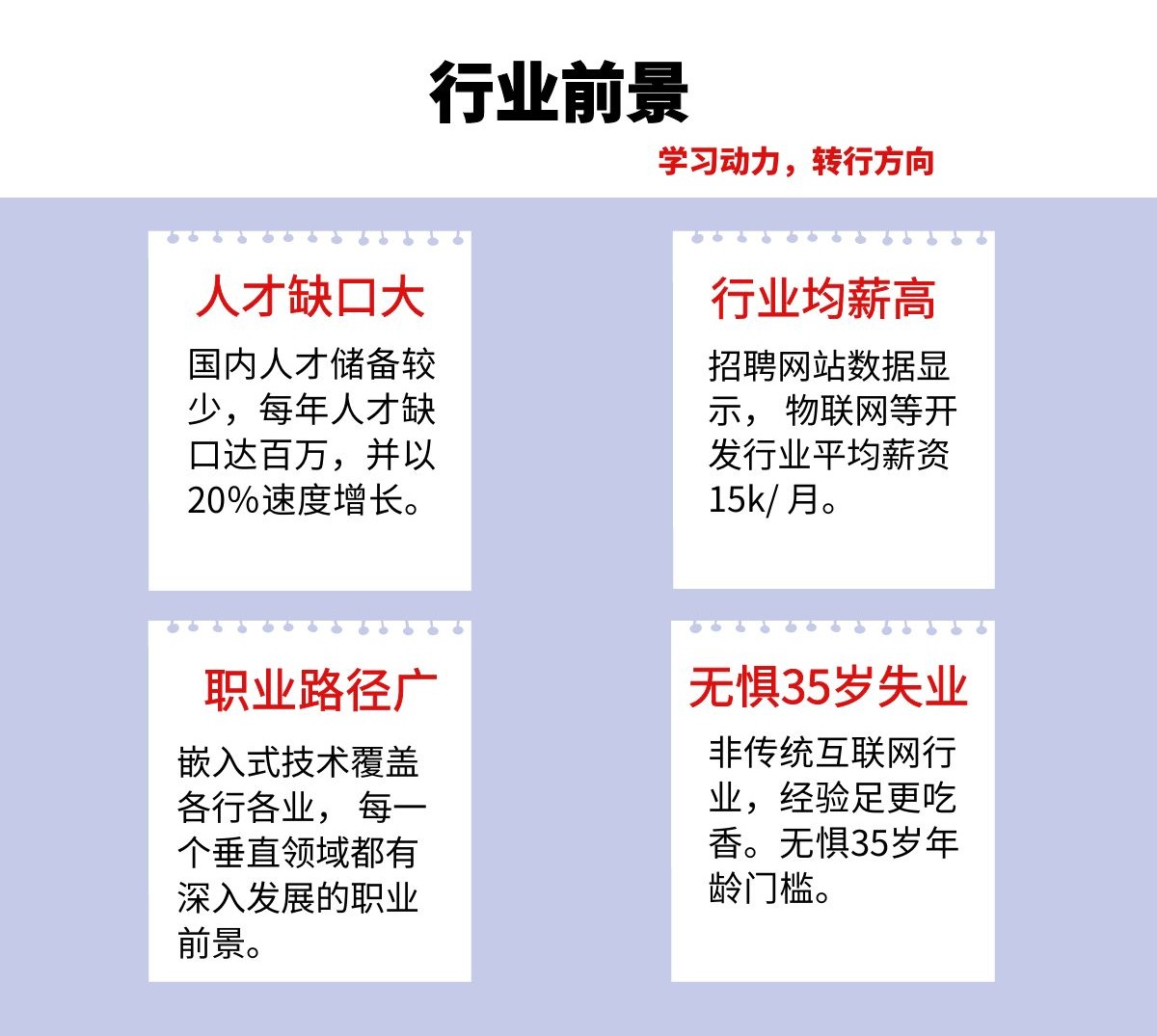
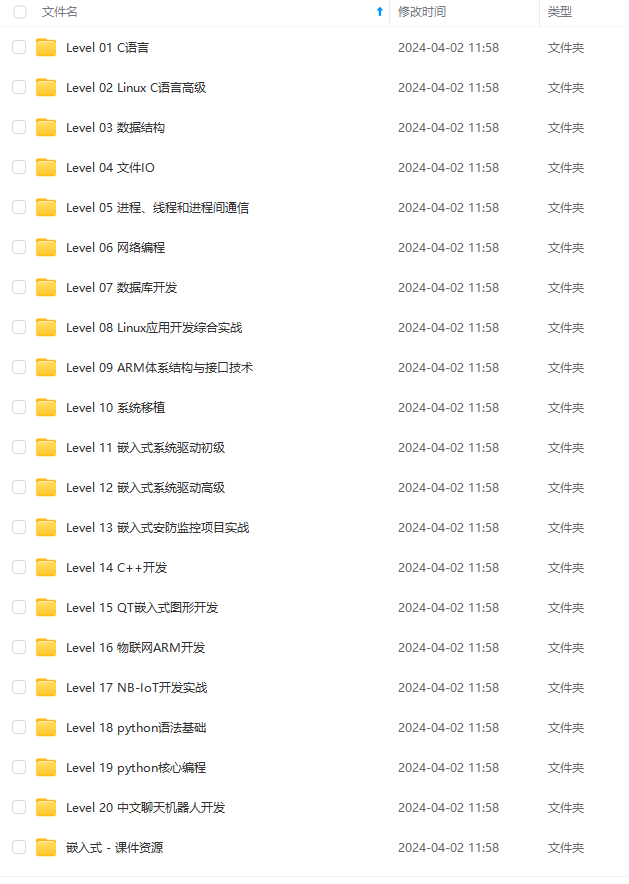
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上嵌入式&物联网开发知识点,真正体系化!**
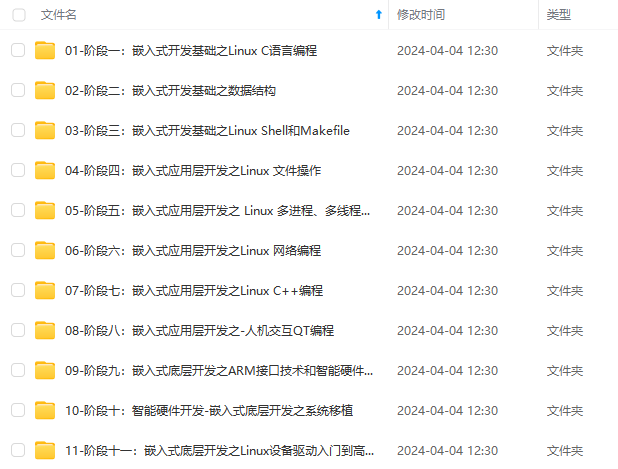
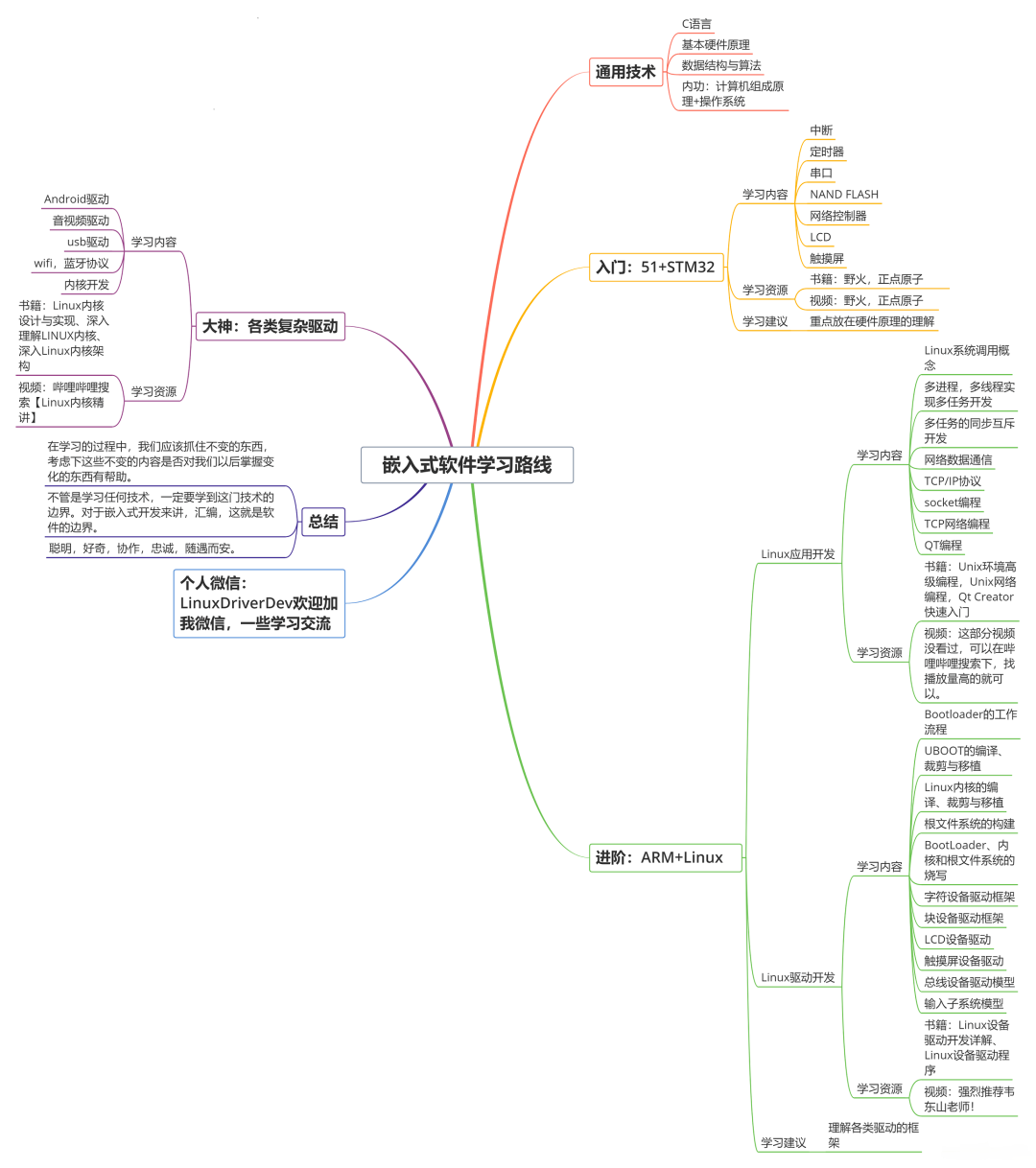
**由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新**
**如果你觉得这些内容对你有帮助,可以+V:Vip1104z获取!!! (备注:嵌入式)**
<img src="https://img-community.csdnimg.cn/images/73bb5de17851459088c6af944156ee24.jpg" alt="img" style="zoom: 67%;" />
# 最后
**资料整理不易,觉得有帮助的朋友可以帮忙点赞分享支持一下小编~**
**你的支持,我的动力;祝各位前程似锦,offer不断,步步高升!!!**
# 最后
**资料整理不易,觉得有帮助的朋友可以帮忙点赞分享支持一下小编~**
**你的支持,我的动力;祝各位前程似锦,offer不断,步步高升!!!**
**[更多资料点击此处获qu!!](https://bbs.csdn.net/topics/618376385)**