package com.wanshi.single;
//饿汉式单例
public class Hungry {
//会造成资源浪费,占用CPU
private byte[] data1 = new byte[1024*1024];
private byte[] data2 = new byte[1024*1024];
private byte[] data3 = new byte[1024*1024];
private byte[] data4 = new byte[1024*1024];
private Hungry() {
System.out.println("Hungry init...");
}
private static Hungry hungry = new Hungry();
public static Hungry getInstance() {
return hungry;
}
}
class Test {
public static void main(String[] args) {
Hungry hungry = Hungry.getInstance();
Hungry hungry2 = Hungry.getInstance();
System.out.println(hungry);
System.out.println(hungry2);
}
}
package com.wanshi.single;
import java.lang.reflect.Constructor;
// enum 是一个class类
public enum EnumSingle {
INSTANCE;
public static EnumSingle getInstance() {
return INSTANCE;
}
}
class Test {
public static void main(String[] args) throws Exception{
EnumSingle instance1 = EnumSingle.INSTANCE;
Constructor<EnumSingle> declaredConstructor = EnumSingle.class.getDeclaredConstructor(String.class, int.class);
declaredConstructor.setAccessible(true);
EnumSingle instance2 = declaredConstructor.newInstance();
System.out.println(instance1);
System.out.println(instance2);
}
}
在这里自行下载jad编译工具即可
枚举类最后反编译源码
jad工具反编译
jad -sjava EnumSingle.class
// Decompiled by Jad v1.5.8g. Copyright 2001 Pavel Kouznetsov.
// Jad home page: http://www.kpdus.com/jad.html
// Decompiler options: packimports(3)
// Source File Name: EnumSingle.java
package com.wanshi.single;
public final class EnumSingle extends Enum
{
public static EnumSingle[] values()
{
return (EnumSingle[])$VALUES.clone();
}
public static EnumSingle valueOf(String name)
{
return (EnumSingle)Enum.valueOf(com/wanshi/single/EnumSingle, name);
}
private EnumSingle(String s, int i)
{
super(s, i);
}
public static EnumSingle getInstance()
{
return INSTANCE;
}
public static final EnumSingle INSTANCE;
private static final EnumSingle $VALUES[];
static
{
INSTANCE = new EnumSingle("INSTANCE", 0);
$VALUES = (new EnumSingle[] {
INSTANCE
});
}
}
package com.wanshi.single;
public class Lazy {
private static Lazy lazy;
public static Lazy getInterface() {
synchronized (Lazy.class) {
if (lazy == null) {
lazy = new Lazy();
}
}
return lazy;
}
public static void main(String[] args) {
Lazy lazy = Lazy.getInterface();
Lazy lazy2 = Lazy.getInterface();
System.out.println(lazy);
System.out.println(lazy2);
}
}
package com.wanshi.single;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
public class LazyMan {
private static boolean flag = false;
private LazyMan() {
synchronized (this) {
if (!flag) {
flag = true;
} else {
throw new RuntimeException("不要试图通过反射破坏对象");
}
}
}
private volatile static LazyMan lazyMan;
//双重检查锁,懒汉式(DCL懒汉式)
public static LazyMan getInstance() {
if (lazyMan == null) {
synchronized (LazyMan.class) {
if (lazyMan == null) {
//不是原子性操作,1.分配内存空间,2.执行构造方法,3.把对象指向这个空间 指令重排可能会发生 加上volatile关闭指令重排
lazyMan = new LazyMan();
}
}
}
return lazyMan;
}
public static void main(String[] args) throws Exception {
// LazyMan lazyMan1 = LazyMan.getInstance();
Field flag = LazyMan.class.getDeclaredField("flag");
# **最后**
分享一些系统的面试题,大家可以拿去刷一刷,准备面试涨薪。
**这些面试题相对应的技术点:**
* JVM
* MySQL
* Mybatis
* MongoDB
* Redis
* Spring
* Spring boot
* Spring cloud
* Kafka
* RabbitMQ
* Nginx
* ......
**大类就是:**
* Java基础
* 数据结构与算法
* 并发编程
* 数据库
* 设计模式
* 微服务
* 消息中间件
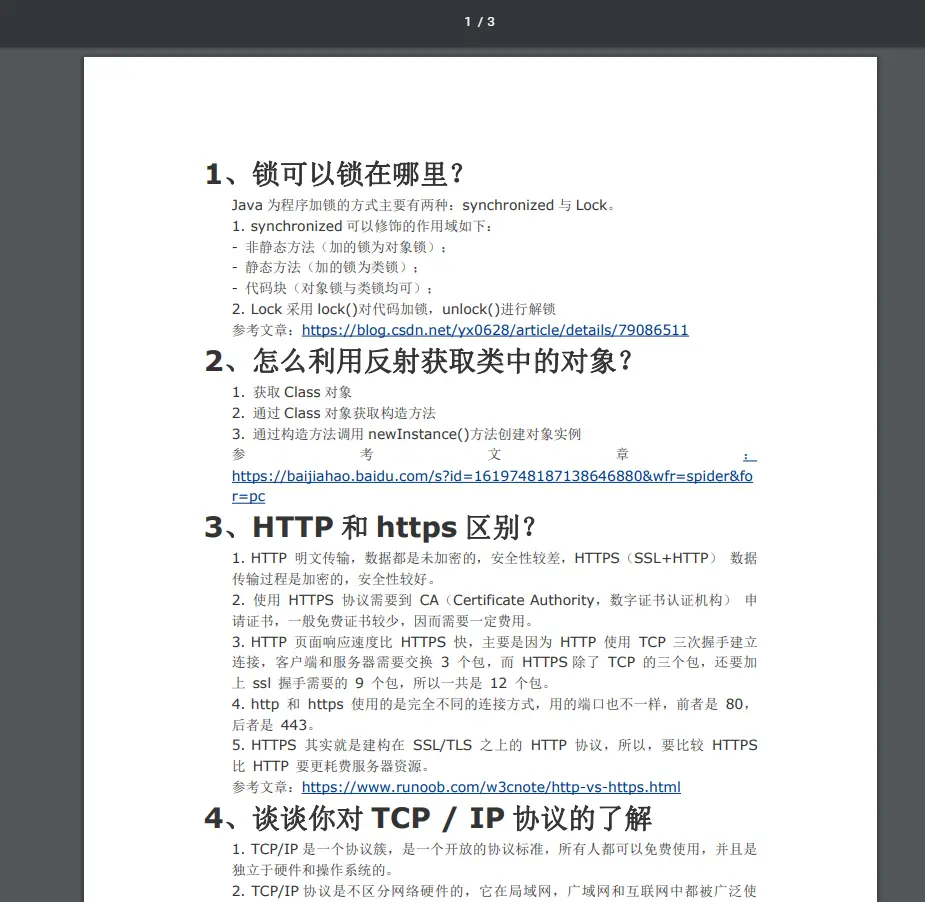
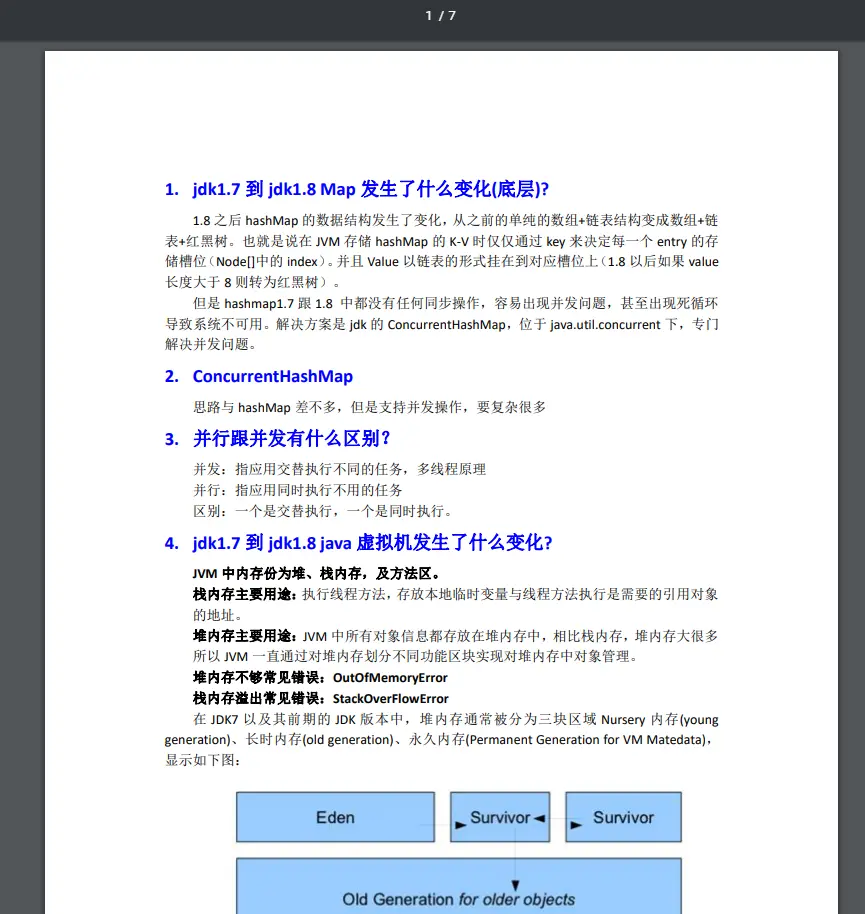
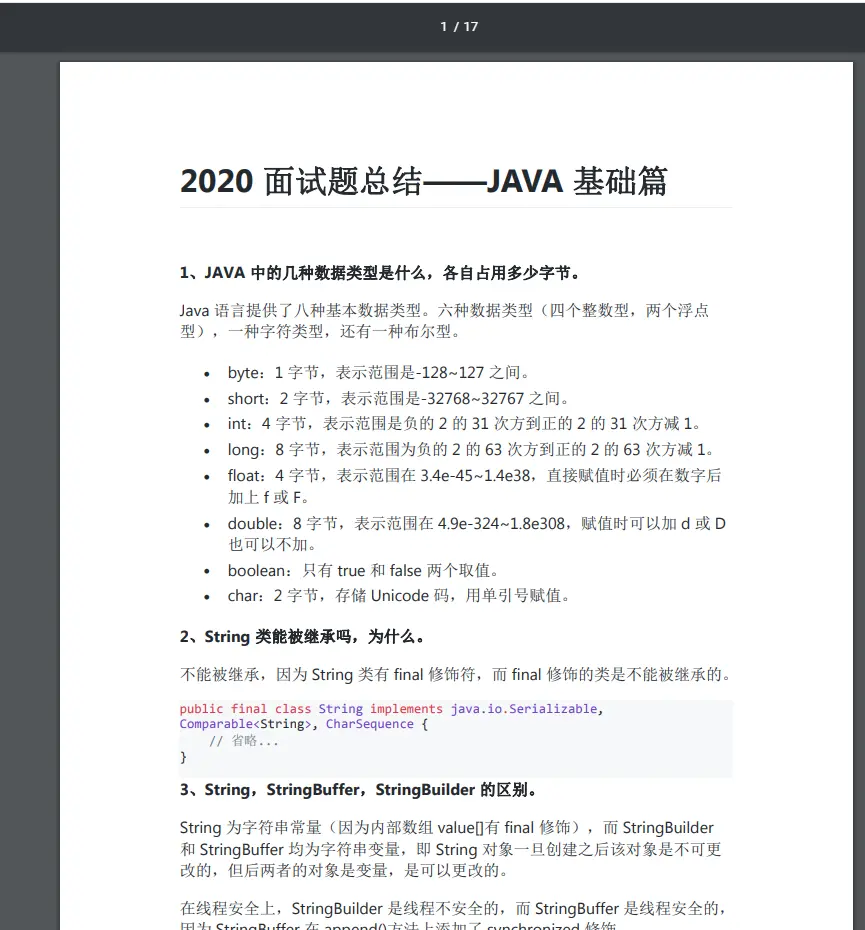
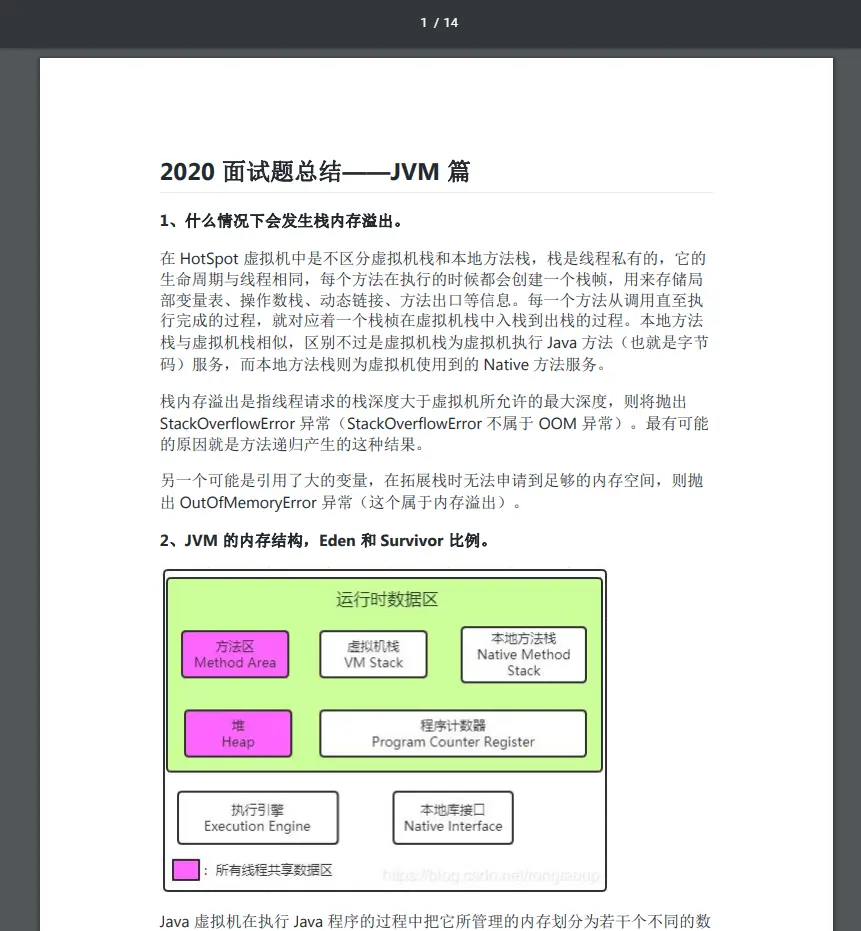
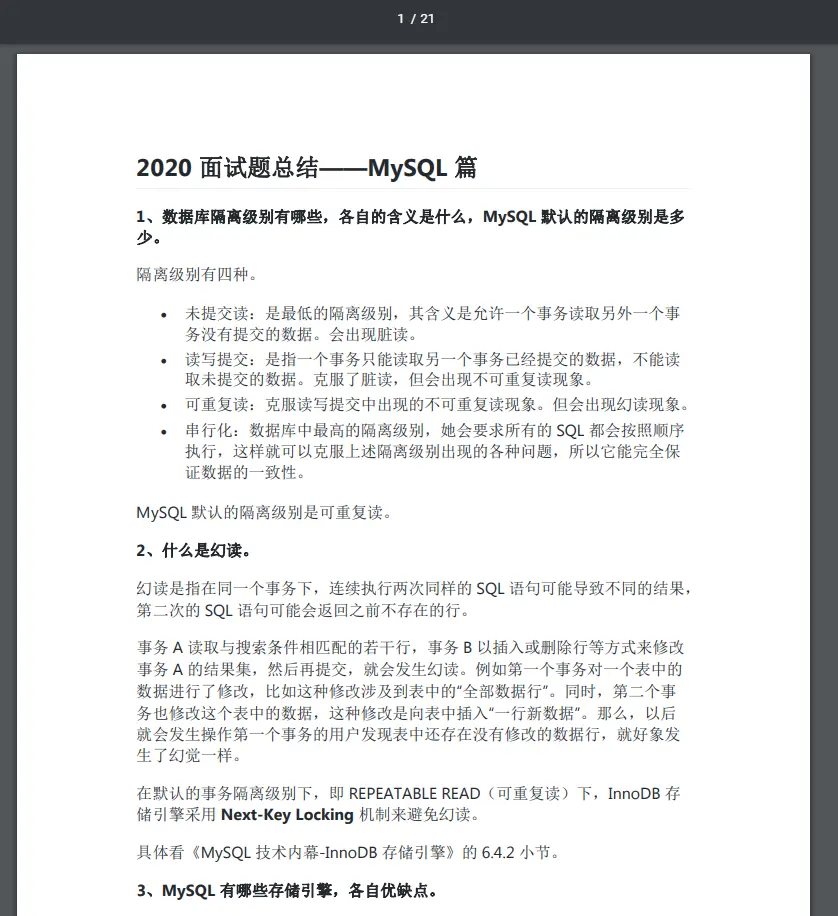
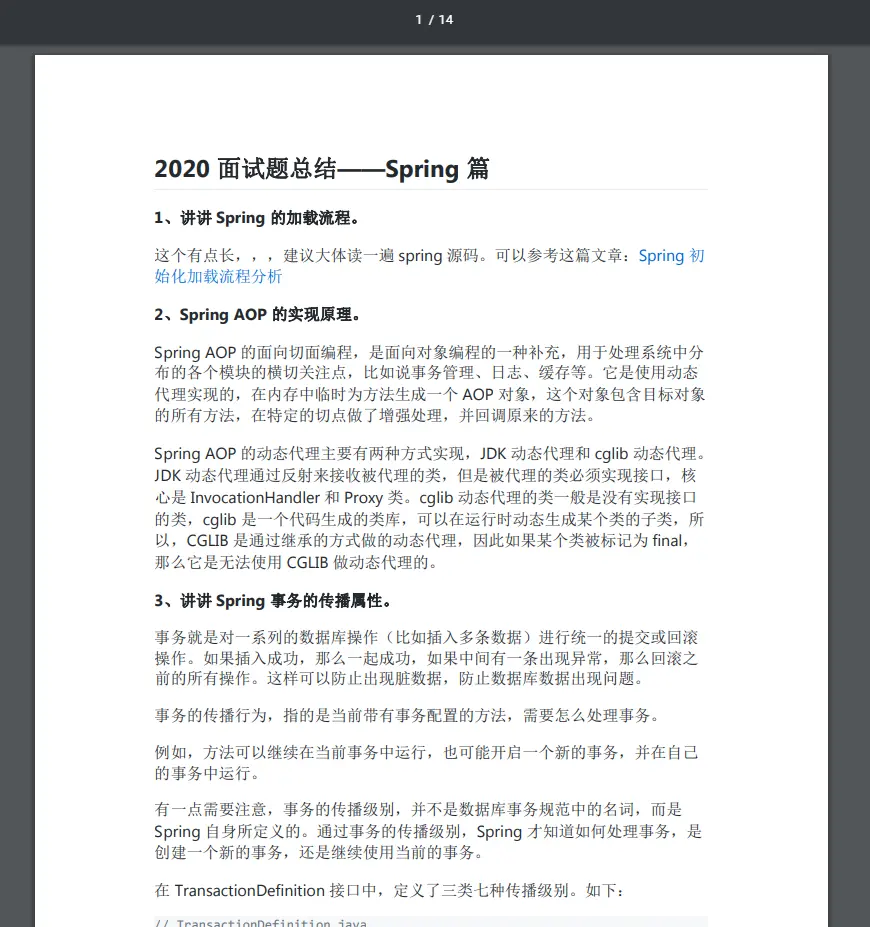
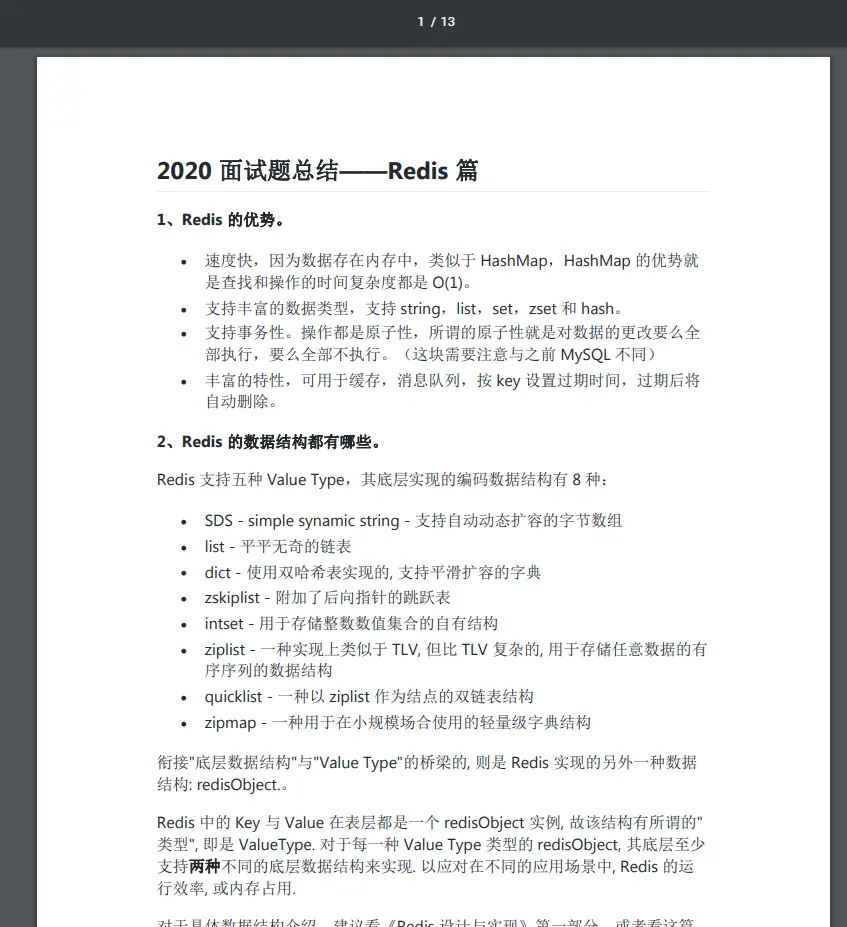
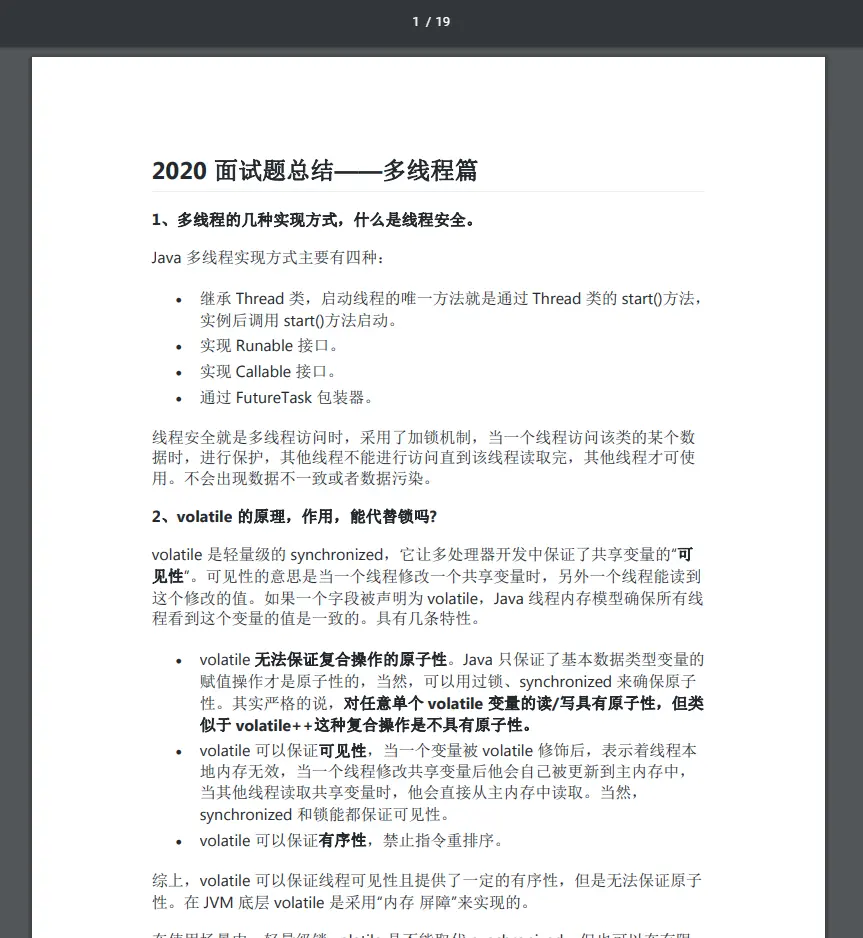
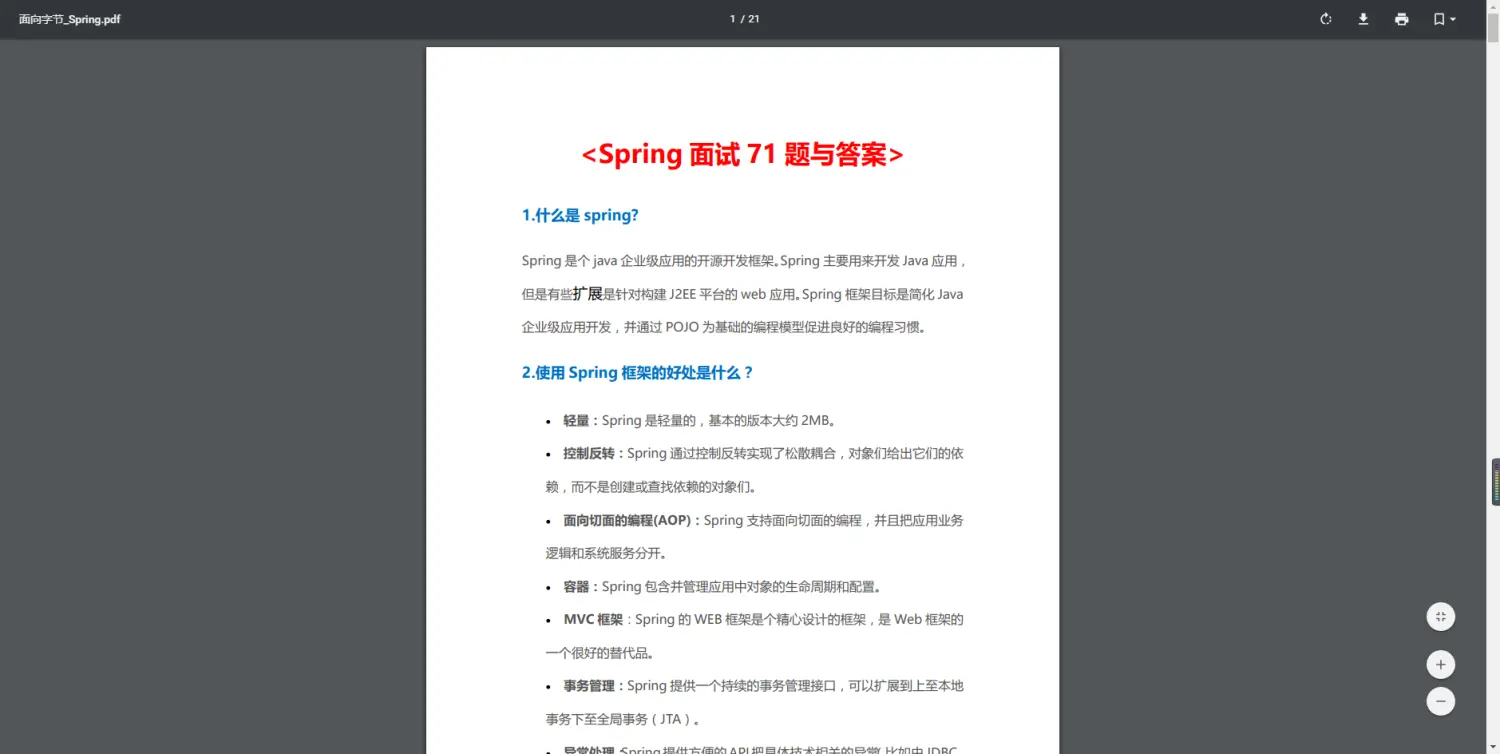
* 数据库
* 设计模式
* 微服务
* 消息中间件
[外链图片转存中...(img-KZOsq5UM-1714537787699)]
[外链图片转存中...(img-b7ufcJWw-1714537787700)]
[外链图片转存中...(img-pnN0TSP2-1714537787700)]
[外链图片转存中...(img-tl7lJlUV-1714537787700)]
[外链图片转存中...(img-6j4vhuTa-1714537787700)]
[外链图片转存中...(img-dqFlk6Jj-1714537787701)]
[外链图片转存中...(img-TKVku3F7-1714537787701)]
[外链图片转存中...(img-nChGc2oD-1714537787701)]
[外链图片转存中...(img-E40f1cmL-1714537787701)]
> **本文已被[CODING开源项目:【一线大厂Java面试题解析+核心总结学习笔记+最新讲解视频+实战项目源码】](https://bbs.csdn.net/topics/618154847)收录**