Object findUserByUsername(String username);
}
com.stiti.model.AppUser和 AppUserRole是Model类。
您可以使用自己的方式获取数据库用户和用户角色表并定义 *findUserByUsername(String username)*函数体。
*findUserByUsername(String username)*返回AppUser类型对象。
package com.stiti.dao.impl;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.HibernateException;
import org.springframework.stereotype.Repository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.transaction.annotation.Transactional;
import com.stiti.dao.LoginDAO;
import com.stiti.model.AppUser;
import com.stiti.model.AppUserRole;
/**
- @author Stiti Samantray
*/
@Repository(“loginDao”)
@Transactional
public class LoginDAOImpl implements LoginDAO {
@Autowired
SessionFactory sessionFactory;
/**
- Finds the AppUser which has a matching username
- @param username
- @return
*/
@Override
public AppUser findUserByUsername( String username )
{
Session session = sessionFactory.getCurrentSession();
List users = new ArrayList();
List userData = new ArrayList();
Set userRoles = new HashSet(0);
try {
String hql = “FROM AppUser U WHERE U.username = :username”;
org.hibernate.Query query = session.createQuery(hql)
.setParameter(“username”, username);
users = query.list();
} catch (HibernateException e) {
System.err.println("ERROR: "+ e.getMessage());
}
AppUser user = null;
if(users.size() > 0) {
user = (AppUser) users.get(0);
// Get the user roles
try {
String hql = “FROM AppUserRole R WHERE R.username = :username”;
org.hibernate.Query query = session.createQuery(hql)
.setParameter(“username”, username);
userRoles = new HashSet(query.list());
} catch (HibernateException e) {
// You can log the error here. Or print to console
}
user.setUserRole(userRoles);
}
return user;
}
}
findUserByUsername(String username) 返回AppUser类型对象。
package com.stiti.service;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.authority.SimpleGrantedAuthority;
import org.springframework.security.core.userdetails.User;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.core.userdetails.UsernameNotFoundException;
import org.springframework.stereotype.Service;
import com.stiti.model.AppUser;
import com.stiti.model.AppUserRole;
import com.stiti.dao.LoginDAO;
/**
- This class gets the appuser information from the database and
- populates the “org.springframework.security.core.userdetails.User” type object for appuser.
- @author Stiti Samantray
*/
@Service(“loginService”)
public class LoginServiceImpl implements UserDetailsService {
@Autowired
private LoginDAO loginDao;
/**
- @see UserDetailsService#loadUserByUsername(String)
*/
@Override
public UserDetails loadUserByUsername( String username ) throws UsernameNotFoundException
{
AppUser user = (AppUser) loginDao.findUserByUsername(username);
List authorities = buildUserAuthority(user.getUserRole());
return buildUserForAuthentication(user, authorities);
}
private List buildUserAuthority(Set appUserRole) {
Set setAuths = new HashSet();
// Build user’s authorities
for (AppUserRole userRole : appUserRole) {
System.out.println(“****” + userRole.getUserRole());
setAuths.add(new SimpleGrantedAuthority(userRole.getUserRole()));
}
List Result = new ArrayList(setAuths);
return Result;
}
private User buildUserForAuthentication(AppUser user, List authorities) {
return new User(user.getUsername(), user.getPassword(), true, true, true, true, authorities);
}
}
##编写Spring MVC应用程序的Controller
现在我们需要编写Spring MVC应用程序的Controller。
我们需要在Controller类中为应用程序的主路径定义一个RequestMapping方法,该路径在我的示例“/”中。当用户打开应用程序URL例如“http://www.example.com/”时,执行为该请求映射定义的以下方法“loadHomePage()”。在此方法中,它首先获得对此URL的用户身份验证和授权。
Spring-security将首先检查spring配置中的<security:intercept_url>,以查找允许访问此url路径的角色。在此示例中,它查找允许具有角色“USER”的用户访问此URL路径。如果用户有一个角色= USER,那么它将加载主页。
否则,如果它是匿名用户,则spring安全校验会将它重定向到登录页面。
package com.stiti.controller;
import java.util.HashSet;
import java.util.Set;
import org.springframework.security.authentication.AnonymousAuthenticationToken;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.SessionAttributes;
@Controller
@SessionAttributes(value={“accountname”}, types={String.class})
public class HomeController {
@SuppressWarnings(“unchecked”)
@RequestMapping(value=“/”, method = RequestMethod.GET)
public String executeSecurityAndLoadHomePage(ModelMap model) {
String name = null;
Set role = new HashSet();
//check if user is login
Authentication auth = SecurityContextHolder.getContext().getAuthentication();
if (!(auth instanceof AnonymousAuthenticationToken)) {
// Get the user details
UserDetails userDetail = (UserDetails) auth.getPrincipal();
name = userDetail.getUsername();
role = (Set) userDetail.getAuthorities();
}
// set the model attributes
model.addAttribute(“accountname”, name);
model.addAttribute(“userRole”, role);
// go to Home page
return “Home”;
}
}
在我们的Controller类中定义RequestMapping方法以加载自定义“登录页面”,前提是您在Spring <security:http> <security:form-login …>标记中提到了自定义登录页面URL。否则无需为Login页面定义任何控制器方法,spring会自动将用户带到spring-security的默认登录表单页面,这是一个由spring-security本身编写的简单JSP页面。
// Show Login Form
@RequestMapping(value=“/login”, method = RequestMethod.GET)
public String login(ModelMap model,
@RequestParam(value=“error”,defaultValue=“”) String error) {
// If fails to login
if (!error.isEmpty()){
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
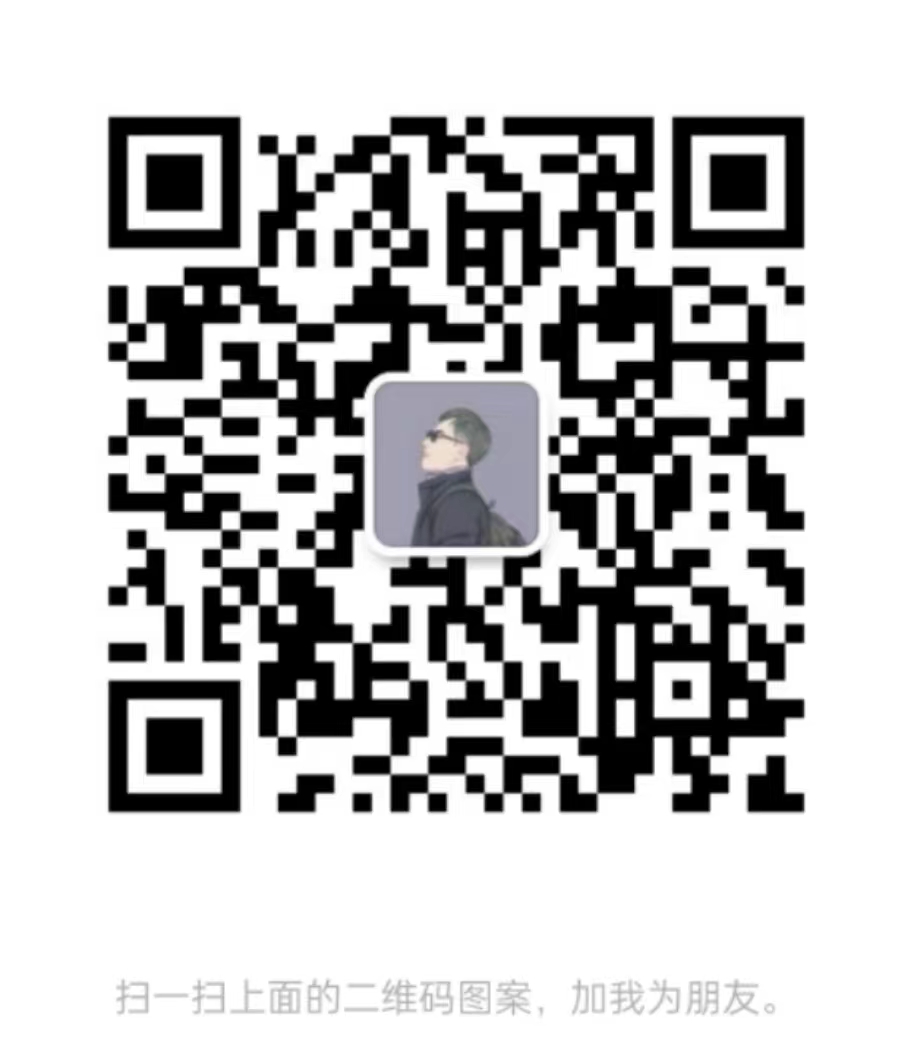
最后
光给面试题不给答案不是我的风格。这里面的面试题也只是凤毛麟角,还有答案的话会极大的增加文章的篇幅,减少文章的可读性
Java面试宝典2021版
最常见Java面试题解析(2021最新版)
2021企业Java面试题精选
《一线大厂Java面试题解析+核心总结学习笔记+最新讲解视频+实战项目源码》,点击传送门即可获取!
2543907)]
[外链图片转存中…(img-xQ93ukrR-1711982543908)]
2021企业Java面试题精选
[外链图片转存中…(img-g9ZAWvRu-1711982543908)]
[外链图片转存中…(img-65F6Y5Hw-1711982543909)]
《一线大厂Java面试题解析+核心总结学习笔记+最新讲解视频+实战项目源码》,点击传送门即可获取!