-
Interface representing the environment in which the current application is running.
-
Models two key aspects of the application environment: profiles and
-
properties. Methods related to property access are exposed via the
-
{@link PropertyResolver} superinterface.
-
A profile is a named, logical group of bean definitions to be registered
-
with the container only if the given profile is active. Beans may be assigned
-
to a profile whether defined in XML or via annotations; see the spring-beans 3.1 schema
-
or the {@link org.springframework.context.annotation.Profile @Profile} annotation for
-
syntax details. The role of the {@code Environment} object with relation to profiles is
-
in determining which profiles (if any) are currently {@linkplain #getActiveProfiles
-
active}, and which profiles (if any) should be {@linkplain #getDefaultProfiles active
-
by default}.
-
Properties play an important role in almost all applications, and may
-
originate from a variety of sources: properties files, JVM system properties, system
-
environment variables, JNDI, servlet context parameters, ad-hoc Properties objects,
-
Maps, and so on. The role of the environment object with relation to properties is to
-
provide the user with a convenient service interface for configuring property sources
-
and resolving properties from them.
-
Beans managed within an {@code ApplicationContext} may register to be {@link
-
org.springframework.context.EnvironmentAware EnvironmentAware} or {@code @Inject} the
-
{@code Environment} in order to query profile state or resolve properties directly.
-
In most cases, however, application-level beans should not need to interact with the
-
{@code Environment} directly but instead may have to have {@code ${…}} property
-
values replaced by a property placeholder configurer such as
-
{@link org.springframework.context.support.PropertySourcesPlaceholderConfigurer
-
PropertySourcesPlaceholderConfigurer}, which itself is {@code EnvironmentAware} and
-
as of Spring 3.1 is registered by default when using
-
{@code context:property-placeholder/}.
-
Configuration of the environment object must be done through the
-
{@code ConfigurableEnvironment} interface, returned from all
-
{@code AbstractApplicationContext} subclass {@code getEnvironment()} methods. See
-
{@link ConfigurableEnvironment} Javadoc for usage examples demonstrating manipulation
-
of property sources prior to application context {@code refresh()}.
-
@author Chris Beams
-
@since 3.1
-
@see PropertyResolver
-
@see EnvironmentCapable
-
@see ConfigurableEnvironment
-
@see AbstractEnvironment
-
@see StandardEnvironment
-
@see org.springframework.context.EnvironmentAware
-
@see org.springframework.context.ConfigurableApplicationContext#getEnvironment
-
@see org.springframework.context.ConfigurableApplicationContext#setEnvironment
-
@see org.springframework.context.support.AbstractApplicationContext#createEnvironment
*/
public interface Environment extends PropertyResolver {
/**
-
Return the set of profiles explicitly made active for this environment. Profiles
-
are used for creating logical groupings of bean definitions to be registered
-
conditionally, for example based on deployment environment. Profiles can be
-
activated by setting {@linkplain AbstractEnvironment#ACTIVE_PROFILES_PROPERTY_NAME
-
“spring.profiles.active”} as a system property or by calling
-
{@link ConfigurableEnvironment#setActiveProfiles(String…)}.
-
If no profiles have explicitly been specified as active, then any {@linkplain
-
#getDefaultProfiles() default profiles} will automatically be activated.
-
@see #getDefaultProfiles
-
@see ConfigurableEnvironment#setActiveProfiles
-
@see AbstractEnvironment#ACTIVE_PROFILES_PROPERTY_NAME
*/
String[] getActiveProfiles();
/**
-
Return the set of profiles to be active by default when no active profiles have
-
been set explicitly.
-
@see #getActiveProfiles
-
@see ConfigurableEnvironment#setDefaultProfiles
-
@see AbstractEnvironment#DEFAULT_PROFILES_PROPERTY_NAME
*/
String[] getDefaultProfiles();
/**
-
Return whether one or more of the given profiles is active or, in the case of no
-
explicit active profiles, whether one or more of the given profiles is included in
-
the set of default profiles. If a profile begins with ‘!’ the logic is inverted,
-
i.e. the method will return true if the given profile is not active.
-
For example,
env.acceptsProfiles(“p1”, “!p2”)
will -
return {@code true} if profile ‘p1’ is active or ‘p2’ is not active.
-
@throws IllegalArgumentException if called with zero arguments
-
or if any profile is {@code null}, empty or whitespace-only
-
@see #getActiveProfiles
-
@see #getDefaultProfiles
*/
boolean acceptsProfiles(String… profiles);
}
鉴于注释太多,抽出其中核心代码:
package org.springframework.core.env;
public interface Environment extends PropertyResolver {
String[] getActiveProfiles();
String[] getDefaultProfiles();
boolean acceptsProfiles(String… profiles);
}
可以看到Environment 又extends PropertyResolver
/*
-
Copyright 2002-2013 the original author or authors.
-
Licensed under the Apache License, Version 2.0 (the “License”);
-
you may not use this file except in compliance with the License.
-
You may obtain a copy of the License at
-
http://www.apache.org/licenses/LICENSE-2.0
-
Unless required by applicable law or agreed to in writing, software
-
distributed under the License is distributed on an “AS IS” BASIS,
-
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-
See the License for the specific language governing permissions and
-
limitations under the License.
*/
package org.springframework.core.env;
/**
-
Interface for resolving properties against any underlying source.
-
@author Chris Beams
-
@since 3.1
-
@see Environment
-
@see PropertySourcesPropertyResolver
*/
public interface PropertyResolver {
/**
-
Return whether the given property key is available for resolution, i.e.,
-
the value for the given key is not {@code null}.
*/
boolean containsProperty(String key);
/**
-
Return the property value associated with the given key, or {@code null}
-
if the key cannot be resolved.
-
@param key the property name to resolve
-
@see #getProperty(String, String)
-
@see #getProperty(String, Class)
-
@see #getRequiredProperty(String)
*/
String getProperty(String key);
/**
-
Return the property value associated with the given key, or
-
{@code defaultValue} if the key cannot be resolved.
-
@param key the property name to resolve
-
@param defaultValue the default value to return if no value is found
-
@see #getRequiredProperty(String)
-
@see #getProperty(String, Class)
*/
String getProperty(String key, String defaultValue);
/**
-
Return the property value associated with the given key, or {@code null}
-
if the key cannot be resolved.
-
@param key the property name to resolve
-
@param targetType the expected type of the property value
-
@see #getRequiredProperty(String, Class)
*/
T getProperty(String key, Class targetType);
/**
-
Return the property value associated with the given key, or
-
{@code defaultValue} if the key cannot be resolved.
-
@param key the property name to resolve
-
@param targetType the expected type of the property value
-
@param defaultValue the default value to return if no value is found
-
@see #getRequiredProperty(String, Class)
*/
T getProperty(String key, Class targetType, T defaultValue);
/**
-
Convert the property value associated with the given key to a {@code Class}
-
of type {@code T} or {@code null} if the key cannot be resolved.
-
@throws org.springframework.core.convert.ConversionException if class specified
-
by property value cannot be found or loaded or if targetType is not assignable
-
from class specified by property value
-
@see #getProperty(String, Class)
*/
Class getPropertyAsClass(String key, Class targetType);
/**
-
Return the property value associated with the given key (never {@code null}).
-
@throws IllegalStateException if the key cannot be resolved
-
@see #getRequiredProperty(String, Class)
*/
String getRequiredProperty(String key) throws IllegalStateException;
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
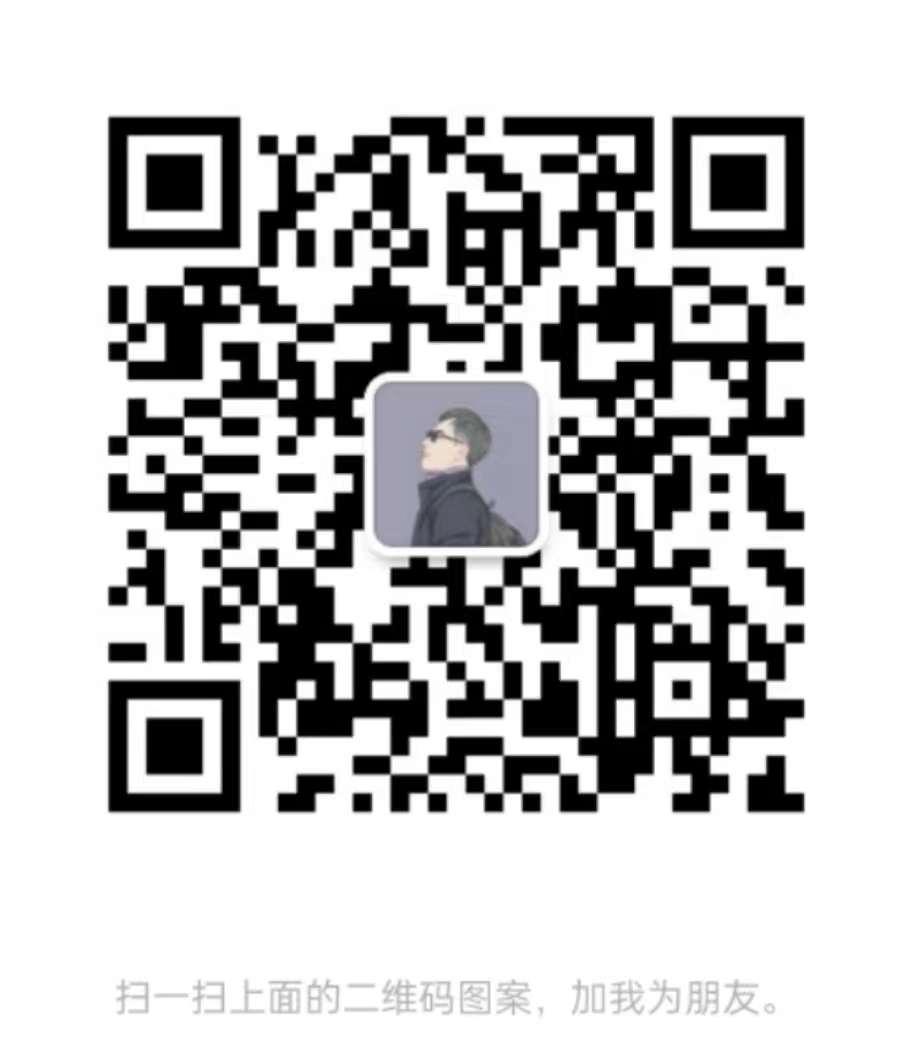
文末
我将这三次阿里面试的题目全部分专题整理出来,并附带上详细的答案解析,生成了一份PDF文档
- 第一个要分享给大家的就是算法和数据结构
- 第二个就是数据库的高频知识点与性能优化
- 第三个则是并发编程(72个知识点学习)
- 最后一个是各大JAVA架构专题的面试点+解析+我的一些学习的书籍资料
还有更多的Redis、MySQL、JVM、Kafka、微服务、Spring全家桶等学习笔记这里就不一一列举出来
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
cgaDqEn-1713293877976)]
- 第二个就是数据库的高频知识点与性能优化
[外链图片转存中…(img-3jUf1bLw-1713293877976)]
- 第三个则是并发编程(72个知识点学习)
[外链图片转存中…(img-5hWJZdvD-1713293877976)]
- 最后一个是各大JAVA架构专题的面试点+解析+我的一些学习的书籍资料
[外链图片转存中…(img-L3Gk7bOm-1713293877977)]
还有更多的Redis、MySQL、JVM、Kafka、微服务、Spring全家桶等学习笔记这里就不一一列举出来
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!