-
Task task = (Task)msg.obj;
-
// 调用callback对象的loadImage方法,并将图片路径和图片回传给adapter
-
task.callback.loadImage(task.path, task.bitmap);
-
}
-
};
-
private Runnable runnable = new Runnable() {
-
@Override
-
public void run() {
-
while(isRunning){
-
// 当队列中还有未处理的任务时,执行下载任务
-
while(taskQueue.size() > 0){
-
// 获取第一个任务,并将之从任务队列中删除
-
Task task = taskQueue.remove(0);
-
// 将下载的图片添加到缓存
-
task.bitmap = PicUtil.getbitmap(task.path);
-
caches.put(task.path, new SoftReference(task.bitmap));
-
if(handler != null){
-
// 创建消息对象,并将完成的任务添加到消息对象中
-
Message msg = handler.obtainMessage();
-
msg.obj = task;
-
// 发送消息回主线程
-
handler.sendMessage(msg);
-
}
-
}
-
//如果队列为空,则令线程等待
-
synchronized (this) {
-
try {
-
this.wait();
-
} catch (InterruptedException e) {
-
e.printStackTrace();
-
}
-
}
-
}
-
}
-
};
-
//回调接口
-
public interface ImageCallback{
-
void loadImage(String path, Bitmap bitmap);
-
}
-
class Task{
-
// 下载任务的下载路径
-
String path;
-
// 下载的图片
-
Bitmap bitmap;
-
// 回调对象
-
ImageCallback callback;
-
@Override
-
public boolean equals(Object o) {
-
Task task = (Task)o;
-
return task.path.equals(path);
-
}
-
}
-
}
最后附上PicUtil类的代码,之前忘了贴这个类的代码,不好意识了~~
[java] view plain copy
-
public class PicUtil {
-
private static final String TAG = “PicUtil”;
-
/**
-
* 根据一个网络连接(URL)获取bitmapDrawable图像
-
*
-
* @param imageUri
-
* @return
-
*/
-
public static BitmapDrawable getfriendicon(URL imageUri) {
-
BitmapDrawable icon = null;
-
try {
-
HttpURLConnection hp = (HttpURLConnection) imageUri
-
.openConnection();
-
icon = new BitmapDrawable(hp.getInputStream());// 将输入流转换成bitmap
-
hp.disconnect();// 关闭连接
-
} catch (Exception e) {
-
}
-
return icon;
-
}
-
/**
-
* 根据一个网络连接(String)获取bitmapDrawable图像
-
*
-
* @param imageUri
-
* @return
-
*/
-
public static BitmapDrawable getcontentPic(String imageUri) {
-
URL imgUrl = null;
-
try {
-
imgUrl = new URL(imageUri);
-
} catch (MalformedURLException e1) {
-
e1.printStackTrace();
-
}
-
BitmapDrawable icon = null;
-
try {
-
HttpURLConnection hp = (HttpURLConnection) imgUrl.openConnection();
-
icon = new BitmapDrawable(hp.getInputStream());// 将输入流转换成bitmap
-
hp.disconnect();// 关闭连接
-
} catch (Exception e) {
-
}
-
return icon;
-
}
-
/**
-
* 根据一个网络连接(URL)获取bitmap图像
-
*
-
* @param imageUri
-
* @return
-
*/
-
public static Bitmap getusericon(URL imageUri) {
-
// 显示网络上的图片
-
URL myFileUrl = imageUri;
-
Bitmap bitmap = null;
-
try {
-
HttpURLConnection conn = (HttpURLConnection) myFileUrl
-
.openConnection();
-
conn.setDoInput(true);
-
conn.connect();
-
InputStream is = conn.getInputStream();
-
bitmap = BitmapFactory.decodeStream(is);
-
is.close();
-
} catch (IOException e) {
-
e.printStackTrace();
-
}
-
return bitmap;
-
}
-
/**
-
* 根据一个网络连接(String)获取bitmap图像
-
*
-
* @param imageUri
-
* @return
-
* @throws MalformedURLException
-
*/
-
public static Bitmap getbitmap(String imageUri) {
-
// 显示网络上的图片
-
Bitmap bitmap = null;
-
try {
-
URL myFileUrl = new URL(imageUri);
-
HttpURLConnection conn = (HttpURLConnection) myFileUrl
-
.openConnection();
-
conn.setDoInput(true);
-
conn.connect();
-
InputStream is = conn.getInputStream();
-
bitmap = BitmapFactory.decodeStream(is);
-
is.close();
-
Log.i(TAG, “image download finished.” + imageUri);
-
} catch (IOException e) {
-
e.printStackTrace();
-
return null;
-
}
-
return bitmap;
-
}
-
/**
-
* 下载图片 同时写道本地缓存文件中
-
*
-
* @param context
-
* @param imageUri
-
* @return
-
* @throws MalformedURLException
-
*/
-
public static Bitmap getbitmapAndwrite(String imageUri) {
-
Bitmap bitmap = null;
-
try {
-
// 显示网络上的图片
-
URL myFileUrl = new URL(imageUri);
-
HttpURLConnection conn = (HttpURLConnection) myFileUrl
-
.openConnection();
-
conn.setDoInput(true);
-
conn.connect();
-
InputStream is = conn.getInputStream();
-
File cacheFile = FileUtil.getCacheFile(imageUri);
-
BufferedOutputStream bos = null;
-
bos = new BufferedOutputStream(new FileOutputStream(cacheFile));
-
Log.i(TAG, "write file to " + cacheFile.getCanonicalPath());
-
byte[] buf = new byte[1024];
-
int len = 0;
-
// 将网络上的图片存储到本地
-
while ((len = is.read(buf)) > 0) {
-
bos.write(buf, 0, len);
-
}
-
is.close();
-
bos.close();
-
// 从本地加载图片
-
bitmap = BitmapFactory.decodeFile(cacheFile.getCanonicalPath());
-
String name = MD5Util.MD5(imageUri);
-
} catch (IOException e) {
-
e.printStackTrace();
-
}
-
return bitmap;
-
}
-
public static boolean downpic(String picName, Bitmap bitmap) {
-
boolean nowbol = false;
-
try {
-
File saveFile = new File(“/mnt/sdcard/download/weibopic/” + picName
-
+ “.png”);
-
if (!saveFile.exists()) {
-
saveFile.createNewFile();
-
}
-
FileOutputStream saveFileOutputStream;
-
saveFileOutputStream = new FileOutputStream(saveFile);
-
nowbol = bitmap.compress(Bitmap.CompressFormat.PNG, 100,
-
saveFileOutputStream);
-
saveFileOutputStream.close();
-
} catch (FileNotFoundException e) {
-
e.printStackTrace();
-
} catch (IOException e) {
-
e.printStackTrace();
-
} catch (Exception e) {
-
e.printStackTrace();
-
}
-
return nowbol;
-
}
-
public static void writeTofiles(Context context, Bitmap bitmap,
-
String filename) {
-
BufferedOutputStream outputStream = null;
-
try {
-
outputStream = new BufferedOutputStream(context.openFileOutput(
-
filename, Context.MODE_PRIVATE));
-
bitmap.compress(Bitmap.CompressFormat.PNG, 100, outputStream);
-
} catch (FileNotFoundException e) {
-
e.printStackTrace();
-
}
-
}
-
/**
-
* 将文件写入缓存系统中
-
*
-
* @param filename
-
* @param is
-
* @return
-
*/
-
public static String writefile(Context context, String filename,
-
InputStream is) {
-
BufferedInputStream inputStream = null;
-
BufferedOutputStream outputStream = null;
-
try {
-
inputStream = new BufferedInputStream(is);
-
outputStream = new BufferedOutputStream(context.openFileOutput(
-
filename, Context.MODE_PRIVATE));
-
byte[] buffer = new byte[1024];
-
int length;
-
while ((length = inputStream.read(buffer)) != -1) {
-
outputStream.write(buffer, 0, length);
-
}
-
} catch (Exception e) {
-
} finally {
-
if (inputStream != null) {
-
try {
-
inputStream.close();
-
} catch (IOException e) {
-
e.printStackTrace();
-
}
-
}
-
if (outputStream != null) {
-
try {
-
outputStream.flush();
-
outputStream.close();
-
} catch (IOException e) {
-
e.printStackTrace();
-
}
-
}
-
}
-
return context.getFilesDir() + “/” + filename + “.jpg”;
-
}
-
// 放大缩小图片
-
public static Bitmap zoomBitmap(Bitmap bitmap, int w, int h) {
-
int width = bitmap.getWidth();
-
int height = bitmap.getHeight();
-
Matrix matrix = new Matrix();
-
float scaleWidht = ((float) w / width);
-
float scaleHeight = ((float) h / height);
-
matrix.postScale(scaleWidht, scaleHeight);
-
Bitmap newbmp = Bitmap.createBitmap(bitmap, 0, 0, width, height,
-
matrix, true);
-
return newbmp;
-
}
-
// 将Drawable转化为Bitmap
-
public static Bitmap drawableToBitmap(Drawable drawable) {
-
int width = drawable.getIntrinsicWidth();
-
int height = drawable.getIntrinsicHeight();
-
Bitmap bitmap = Bitmap.createBitmap(width, height, drawable
-
.getOpacity() != PixelFormat.OPAQUE ? Bitmap.Config.ARGB_8888
-
: Bitmap.Config.RGB_565);
-
Canvas canvas = new Canvas(bitmap);
-
drawable.setBounds(0, 0, width, height);
-
drawable.draw(canvas);
-
return bitmap;
-
}
-
// 获得圆角图片的方法
-
public static Bitmap getRoundedCornerBitmap(Bitmap bitmap, float roundPx) {
-
if(bitmap == null){
-
return null;
-
}
-
Bitmap output = Bitmap.createBitmap(bitmap.getWidth(),
-
bitmap.getHeight(), Config.ARGB_8888);
-
Canvas canvas = new Canvas(output);
-
final int color = 0xff424242;
-
final Paint paint = new Paint();
-
final Rect rect = new Rect(0, 0, bitmap.getWidth(), bitmap.getHeight());
-
final RectF rectF = new RectF(rect);
-
paint.setAntiAlias(true);
-
canvas.drawARGB(0, 0, 0, 0);
-
paint.setColor(color);
-
canvas.drawRoundRect(rectF, roundPx, roundPx, paint);
-
paint.setXfermode(new PorterDuffXfermode(Mode.SRC_IN));
-
canvas.drawBitmap(bitmap, rect, rect, paint);
-
return output;
-
}
-
// 获得带倒影的图片方法
-
public static Bitmap createReflectionImageWithOrigin(Bitmap bitmap) {
-
final int reflectionGap = 4;
-
int width = bitmap.getWidth();
-
int height = bitmap.getHeight();
-
Matrix matrix = new Matrix();
-
matrix.preScale(1, -1);
-
Bitmap reflectionImage = Bitmap.createBitmap(bitmap, 0, height / 2,
-
width, height / 2, matrix, false);
-
Bitmap bitmapWithReflection = Bitmap.createBitmap(width,
-
(height + height / 2), Config.ARGB_8888);
-
Canvas canvas = new Canvas(bitmapWithReflection);
-
canvas.drawBitmap(bitmap, 0, 0, null);
-
Paint deafalutPaint = new Paint();
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数初中级Android工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则近万的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Android移动开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Android开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
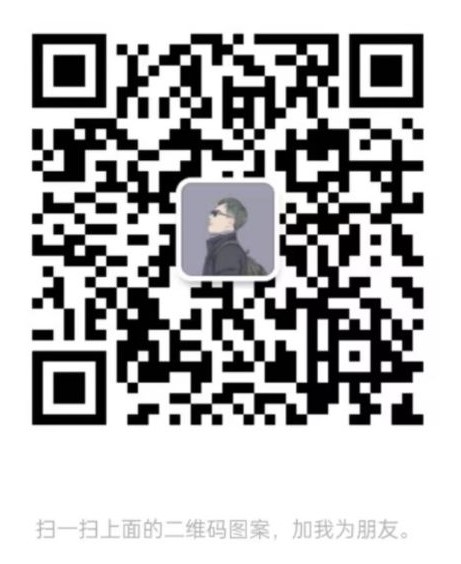
总结
最后对于程序员来说,要学习的知识内容、技术有太多太多,要想不被环境淘汰就只有不断提升自己,从来都是我们去适应环境,而不是环境来适应我们!
这里附上上述的技术体系图相关的几十套腾讯、头条、阿里、美团等公司20年的面试题,把技术点整理成了视频和PDF(实际上比预期多花了不少精力),包含知识脉络 + 诸多细节,由于篇幅有限,这里以图片的形式给大家展示一部分。
相信它会给大家带来很多收获:
当程序员容易,当一个优秀的程序员是需要不断学习的,从初级程序员到高级程序员,从初级架构师到资深架构师,或者走向管理,从技术经理到技术总监,每个阶段都需要掌握不同的能力。早早确定自己的职业方向,才能在工作和能力提升中甩开同龄人。
《Android学习笔记总结+移动架构视频+大厂面试真题+项目实战源码》,点击传送门即可获取!
以上Android开发知识点,真正体系化!**
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
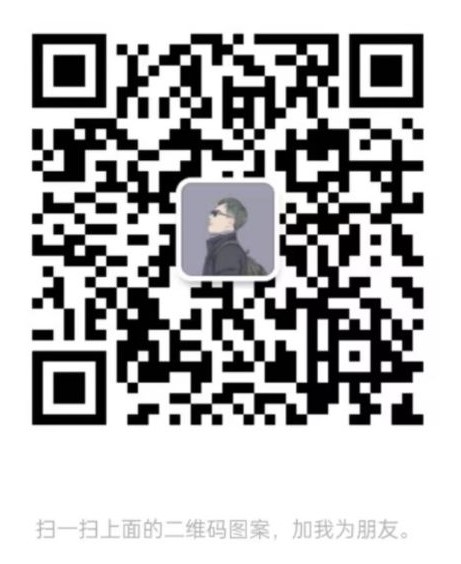
总结
最后对于程序员来说,要学习的知识内容、技术有太多太多,要想不被环境淘汰就只有不断提升自己,从来都是我们去适应环境,而不是环境来适应我们!
这里附上上述的技术体系图相关的几十套腾讯、头条、阿里、美团等公司20年的面试题,把技术点整理成了视频和PDF(实际上比预期多花了不少精力),包含知识脉络 + 诸多细节,由于篇幅有限,这里以图片的形式给大家展示一部分。
相信它会给大家带来很多收获:
[外链图片转存中…(img-CKgrlr9m-1712228623819)]
[外链图片转存中…(img-mVh9HWTv-1712228623820)]
当程序员容易,当一个优秀的程序员是需要不断学习的,从初级程序员到高级程序员,从初级架构师到资深架构师,或者走向管理,从技术经理到技术总监,每个阶段都需要掌握不同的能力。早早确定自己的职业方向,才能在工作和能力提升中甩开同龄人。