android:id=“@+id/ll_content”
android:layout_width=“match_parent”
android:layout_height=“match_parent”
android:orientation=“vertical”
android:translationY=“@dimen/content_trans_y”
app:layout_behavior=“com.pengguanming.mimusicbehavior.behavior.ContentBehavior”>
<com.flyco.tablayout.SlidingTabLayout
android:id=“@+id/stl”
…/>
<android.support.v4.view.ViewPager
android:id=“@+id/vp”
… />
</android.support.design.widget.CoordinatorLayout>
ContentBehavior
这个Behavior主要处理Content部分的Measure、嵌套滑动。
绑定需要做效果的View、引入Dimens、测量Content部分的高度
从上面图片能够分析出:
折叠状态时,Content部分高度=满屏高度-TopBar部分的高度
public class ContentBehavior extends CoordinatorLayout.Behavior{
private int topBarHeight;//topBar内容高度
private float contentTransY;//滑动内容初始化TransY
private float downEndY;//下滑时终点值
private View mLlContent;//Content部分
public ContentBehavior(Context context) {
this(context, null);
}
public ContentBehavior(Context context, AttributeSet attrs) {
super(context, attrs);
//引入尺寸值
int resourceId = context.getResources().getIdentifier(“status_bar_height”, “dimen”, “android”);
int statusBarHeight = context.getResources().getDimensionPixelSize(resourceId);
topBarHeight= (int) context.getResources().getDimension(R.dimen.top_bar_height)+statusBarHeight;
contentTransY= (int) context.getResources().getDimension(R.dimen.content_trans_y);
downEndY= (int) context.getResources().getDimension(R.dimen.content_trans_down_end_y);
…
}
@Override
public boolean onMeasureChild(@NonNull CoordinatorLayout parent, View child,
int parentWidthMeasureSpec, int widthUsed,
int parentHeightMeasureSpec,int heightUsed) {
final int childLpHeight = child.getLayoutParams().height;
if (childLpHeight == ViewGroup.LayoutParams.MATCH_PARENT
|| childLpHeight == ViewGroup.LayoutParams.WRAP_CONTENT) {
//先获取CoordinatorLayout的测量规格信息,若不指定具体高度则使用CoordinatorLayout的高度
int availableHeight = View.MeasureSpec.getSize(parentHeightMeasureSpec);
if (availableHeight == 0) {
availableHeight = parent.getHeight();
}
//设置Content部分高度
final int height = availableHeight - topBarHeight;
final int heightMeasureSpec = View.MeasureSpec.makeMeasureSpec(height,
childLpHeight == ViewGroup.LayoutParams.MATCH_PARENT
-
? View.MeasureSpec.EXACTLY
- View.MeasureSpec.AT_MOST);
//执行指定高度的测量,并返回true表示使用Behavior来代理测量子View
parent.onMeasureChild(child, parentWidthMeasureSpec,
widthUsed, heightMeasureSpec, heightUsed);
return true;
}
return false;
}
@Override
public boolean onLayoutChild(@NonNull CoordinatorLayout parent, @NonNull View child, int layoutDirection) {
boolean handleLayout = super.onLayoutChild(parent, child, layoutDirection);
//绑定Content View
mLlContent=child;
return handleLayout;
}
}
实现NestedScrollingParent2接口
onStartNestedScroll()
ContentBehavior只处理Content部分里可滑动View的垂直方向的滑动。
public boolean onStartNestedScroll(@NonNull CoordinatorLayout coordinatorLayout, @NonNull View child,
@NonNull View directTargetChild, @NonNull View target, int axes, int type) {
//只接受内容View的垂直滑动
return directTargetChild.getId() == R.id.ll_content
&&axes== ViewCompat.SCROLL_AXIS_VERTICAL;
}
onNestedPreScroll()
接下来就是处理滑动,上面效果分析提过:
Content部分的:
上滑范围=[topBarHeight,contentTransY]、
下滑范围=[contentTransY,downEndY]即滑动范围为[topBarHeight,downEndY];
ElemeNestedScrollLayout要控制Content部分的TransitionY值要在范围内,具体处理如下:
Content部分里可滑动View往上滑动时:
-
如果Content部分当前TransitionY+View滑动的dy > topBarHegiht,设置Content部分的TransitionY为Content部分当前TransitionY+View滑动的dy达到移动的效果来消费View的dy。
-
如果Content部分当前TransitionY+View滑动的dy = topBarHegiht,同上操作。
-
如果Content部分当前TransitionY+View滑动的dy < topBarHegiht,只消费部分dy(即Content部分当前TransitionY到topBarHeight差值),剩余的dy让View滑动消费。
**Content部分里可滑动View往下滑动并且View已经不能往下滑动
(比如RecyclerView已经到顶部还往下滑)时:**
-
如果Content部分当前TransitionY+View滑动的dy >= topBarHeight 并且 Content部分当前TransitionY+View滑动的dy <= downEndY,设置Content部分的TransitionY为Content部分当前TransitionY+View滑动的dy达到移动的效果来消费View的dy
-
Content部分当前TransitionY+View滑动的dy > downEndY,只消费部分dy(即Content部分当前TransitionY到downEndY差值)并停止NestedScrollingChild2的View滚动。
public void onNestedPreScroll(@NonNull CoordinatorLayout coordinatorLayout, @NonNull View child,
@NonNull View target, int dx, int dy, @NonNull int[] consumed, int type) {
float transY = child.getTranslationY() - dy;
//处理上滑
if (dy > 0) {
if (transY >= topBarHeight) {
translationByConsume(child, transY, consumed, dy);
} else {
translationByConsume(child, topBarHeight, consumed, (child.getTranslationY() - topBarHeight));
}
}
if (dy < 0 && !target.canScrollVertically(-1)) {
//处理下滑
if (transY >= topBarHeight && transY <= downEndY) {
translationByConsume(child, transY, consumed, dy);
} else {
translationByConsume(child, downEndY, consumed, (downEndY-child.getTranslationY()));
stopViewScroll(target);
}
}
}
private void stopViewScroll(View target){
if (target instanceof RecyclerView) {
((RecyclerView) target).stopScroll();
}
if (target instanceof NestedScrollView) {
try {
Class<? extends NestedScrollView> clazz = ((NestedScrollView) target).getClass();
Field mScroller = clazz.getDeclaredField(“mScroller”);
mScroller.setAccessible(true);
OverScroller overScroller = (OverScroller) mScroller.get(target);
overScroller.abortAnimation();
} catch (NoSuchFieldException | IllegalAccessException e) {
e.printStackTrace();
}
}
}
private void translationByConsume(View view, float translationY, int[] consumed, float consumedDy) {
consumed[1] = (int) consumedDy;
view.setTranslationY(translationY);
}
onStopNestedScroll()
在下滑Content部分从初始状态转换到展开状态的过程中松手就会执行收起的动画,这逻辑在onStopNestedScroll()实现,但注意如果动画未执行完毕手指再落下滑动时,应该在onNestedScrollAccepted()取消当前执行中的动画。
private static final long ANIM_DURATION_FRACTION = 200L;
private ValueAnimator restoreAnimator;//收起内容时执行的动画
public ContentBehavior(Context context, AttributeSet attrs) {
…
restoreAnimator = new ValueAnimator();
restoreAnimator.addUpdateListener(new ValueAnimator.AnimatorUpdateListener() {
@Override
public void onAnimationUpdate(ValueAnimator animation) {
translation(mLlContent, (float) animation.getAnimatedValue());
}
});
}
public void onNestedScrollAccepted(@NonNull CoordinatorLayout coordinatorLayout, @NonNull View child,
@NonNull View directTargetChild, @NonNull View target, int axes, int type) {
if (restoreAnimator.isStarted()) {
restoreAnimator.cancel();
}
}
public void onStopNestedScroll(@NonNull CoordinatorLayout coordinatorLayout, @NonNull View child, @NonNull View target, int type) {
//如果是从初始状态转换到展开状态过程触发收起动画
if (child.getTranslationY() > contentTransY) {
restore();
}
}
private void restore(){
if (restoreAnimator.isStarted()) {
restoreAnimator.cancel();
restoreAnimator.removeAllListeners();
}
restoreAnimator.setFloatValues(mLlContent.getTranslationY(), contentTransY);
restoreAnimator.setDuration(ANIM_DURATION_FRACTION);
restoreAnimator.start();
}
private void translation(View view, float translationY) {
view.setTranslationY(translationY);
}
处理惯性滑动
**场景1:**快速往上滑动Content部分的可滑动View产生惯性滑动,这和前面onNestedPreScroll()处理上滑的效果一模一样,因此可以复用逻辑。
**场景2:**从初始化状态快速下滑转为展开状态,这也和和前面onNestedPreScroll()处理上滑的效果一模一样,因此可以复用逻辑。
**场景3:**从折叠状态快速下滑转为初始化状态,这个过程如下图,看起来像是快速下滑停顿的效果。
public void onNestedPreScroll(@NonNull CoordinatorLayout coordinatorLayout, @NonNull View child,
@NonNull View target, int dx, int dy, @NonNull int[] consumed, int type) {
float transY = child.getTranslationY() - dy;
…
if (dy < 0 && !target.canScrollVertically(-1)) {
//下滑时处理Fling,折叠时下滑Recycler(或NestedScrollView) Fling滚动到contentTransY停止Fling
if (type == ViewCompat.TYPE_NON_TOUCH&&transY >= contentTransY&&flingFromCollaps) {
flingFromCollaps=false;
translationByConsume(child, contentTransY, consumed, dy);
stopViewScroll(target);
return;
}
…
}
}
释放资源
在ContentBehavior被移除时候,执行要停止动画、释放监听者的操作。
public void onDetachedFromLayoutParams() {
if (restoreAnimator.isStarted()) {
restoreAnimator.cancel();
restoreAnimator.removeAllUpdateListeners();
restoreAnimator.removeAllListeners();
restoreAnimator = null;
}
super.onDetachedFromLayoutParams();
}
FaceBehavior
这个Behavior主要处理Face部分的ImageView的位移、蒙层的透明度变化,这里因为篇幅原因,只讲解关键方法,具体源码见
public class FaceBehavior extends CoordinatorLayout.Behavior {
private int topBarHeight;//topBar内容高度
private float contentTransY;//滑动内容初始化TransY
private float downEndY;//下滑时终点值
private float faceTransY;//图片往上位移值
public FaceBehavior(Context context, AttributeSet attrs) {
super(context, attrs);
//引入尺寸值
int resourceId = context.getResources().getIdentifier(“status_bar_height”, “dimen”, “android”);
int statusBarHeight = context.getResources().getDimensionPixelSize(resourceId);
topBarHeight= (int) context.getResources().getDimension(R.dimen.top_bar_height)+statusBarHeight;
contentTransY= (int) context.getResources().getDimension(R.dimen.content_trans_y);
downEndY= (int) context.getResources().getDimension(R.dimen.content_trans_down_end_y);
faceTransY= context.getResources().getDimension(R.dimen.face_trans_y);
…
}
public boolean layoutDependsOn(@NonNull CoordinatorLayout parent, @NonNull View child, @NonNull View dependency) {
//依赖Content View
return dependency.getId() == R.id.ll_content;
}
public boolean onDependentViewChanged(@NonNull CoordinatorLayout parent, @NonNull View child, @NonNull View dependency) {
//计算Content的上滑百分比、下滑百分比
float upPro = (contentTransY- MathUtils.clamp(dependency.getTranslationY(), topBarHeight, contentTransY)) / (contentTransY - topBarHeight);
float downPro = (downEndY- MathUtils.clamp(dependency.getTranslationY(), contentTransY, downEndY)) / (downEndY - contentTransY);
ImageView iamgeview = child.findViewById(R.id.iv_face);
View maskView = child.findViewById(R.id.v_mask);
if (dependency.getTranslationY()>=contentTransY){
//根据Content上滑百分比位移图片TransitionY
iamgeview.setTranslationY(downPro*faceTransY);
}else {
//根据Content下滑百分比位移图片TransitionY
iamgeview.setTranslationY(faceTransY+4upProfaceTransY);
}
//根据Content上滑百分比设置图片和蒙层的透明度
iamgeview.setAlpha(1-upPro);
maskView.setAlpha(upPro);
//因为改变了child的位置,所以返回true
return true;
}
}
其实从上面代码也可以看出逻辑非常简单,在layoutDependsOn()依赖Content,在onDependentViewChanged()里计算Content的上、下滑动百分比来处理图片和蒙层的位移、透明变化。
TopBarBehavior
这个Behavior主要处理TopBar部分的两个子View的透明度变化,
因为逻辑跟FaceBehavior十分类似就不细说了。
public class TopBarBehavior extends CoordinatorLayout.Behavior {
private float contentTransY;//滑动内容初始化TransY
private int topBarHeight;//topBar内容高度
…
public TopBarBehavior(Context context, AttributeSet attrs) {
super(context, attrs);
//引入尺寸值
contentTransY= (int) context.getResources().getDimension(R.dimen.content_trans_y);
int resourceId = context.getResources().getIdentifier(“status_bar_height”, “dimen”, “android”);
int statusBarHeight = context.getResources().getDimensionPixelSize(resourceId);
topBarHeight= (int) context.getResources().getDimension(R.dimen.top_bar_height)+statusBarHeight;
}
public boolean layoutDependsOn(@NonNull CoordinatorLayout parent, @NonNull View child, @NonNull View dependency) {
//依赖Content
return dependency.getId() == R.id.ll_content;
}
public boolean onDependentViewChanged(@NonNull CoordinatorLayout parent, @NonNull View child, @NonNull View dependency) {
//计算Content上滑的百分比,设置子view的透明度
float upPro = (contentTransY- MathUtils.clamp(dependency.getTranslationY(), topBarHeight, contentTransY)) / (contentTransY - topBarHeight);
View tvName=child.findViewById(R.id.tv_top_bar_name);
View tvColl=child.findViewById(R.id.tv_top_bar_coll);
tvName.setAlpha(upPro);
tvColl.setAlpha(upPro);
return true;
}
}
TitleBarBehavior
这个Behavior主要处理TitleBar部分在布局位置紧贴Content顶部和关联的View的透明度变化。
public class TitleBarBehavior extends CoordinatorLayout.Behavior {
private float contentTransY;//滑动内容初始化TransY
private int topBarHeight;//topBar内容高度
public TitleBarBehavior(Context context, AttributeSet attrs) {
super(context, attrs);
//引入尺寸值
contentTransY= (int) context.getResources().getDimension(R.dimen.content_trans_y);
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数初中级Android工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则近万的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Android移动开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Android开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
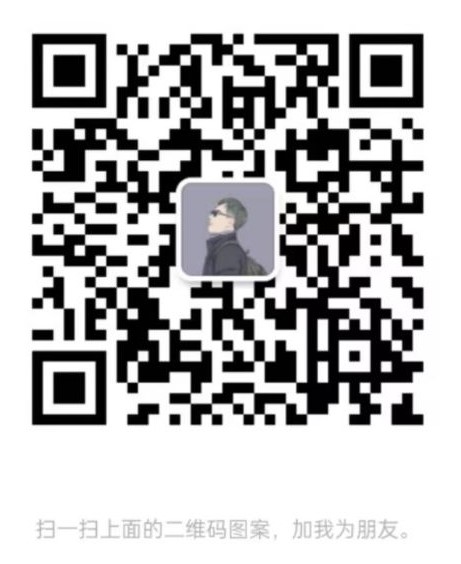
总结
作为一名从事Android的开发者,很多人最近都在和我吐槽Android是不是快要凉了?而在我看来这正是市场成熟的表现,所有的市场都是温水煮青蛙,永远会淘汰掉不愿意学习改变,安于现状的那批人,希望所有的人能在大浪淘沙中留下来,因为对于市场的逐渐成熟,平凡并不是我们唯一的答案!
《Android学习笔记总结+移动架构视频+大厂面试真题+项目实战源码》,点击传送门即可获取!
img-mKhOwoja-1712219266202)]
[外链图片转存中…(img-gcRLbrAq-1712219266203)]
[外链图片转存中…(img-XcoZFVkC-1712219266203)]
[外链图片转存中…(img-v0zImJZd-1712219266203)]
[外链图片转存中…(img-VpPnPRfg-1712219266204)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Android开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
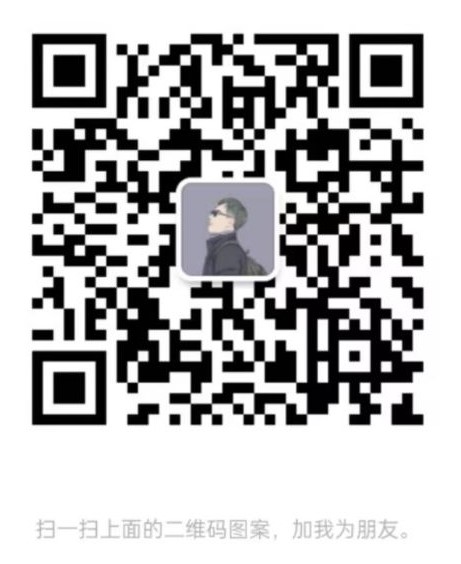
总结
作为一名从事Android的开发者,很多人最近都在和我吐槽Android是不是快要凉了?而在我看来这正是市场成熟的表现,所有的市场都是温水煮青蛙,永远会淘汰掉不愿意学习改变,安于现状的那批人,希望所有的人能在大浪淘沙中留下来,因为对于市场的逐渐成熟,平凡并不是我们唯一的答案!
[外链图片转存中…(img-XzFtgRoq-1712219266204)]
[外链图片转存中…(img-tz3Oih8L-1712219266204)]