/**
- 构建PopupSelectPriceStar 记得调用PopupSelectPriceStar.show()
*/
public PopupWindowHelper build() {
if (context == null) {
throw new IllegalArgumentException(“Context can’t be null!”);
}
if (anchor == null) {
throw new IllegalArgumentException(“anchor View can’t be null!”);
}
if (showView == null && layoutId == -1) {
throw new IllegalArgumentException(“showView can’t be null!”);
}
if (backgroundDrawable == null) {
backgroundDrawable = new ColorDrawable();
}
return new PopupWindowHelper(this);
}
}
创建封装类PopupWindowHelper
首先私有化构造方法,然后将builder里面的属性设置到PopupWindowHelper中
private PopupWindowHelper(Builder builder) {
this.mContext = builder.context;
this.mAnchor = builder.anchor;
this.mView = builder.showView;
this.mLayoutId = builder.layoutId;
this.mWidth = builder.width;
this.mHeight = builder.height;
this.mStyle = builder.style;
this.mFocusable = builder.focusable;
this.mAlpha = builder.alpha;
this.mBackgroundDrawable = builder.backgroundDrawable;
this.mPopupPosition = builder.popupPosition;
}
现在,有了这些东西,可以显示了,PopupWindowHelper提供给外部一个show()方法,用来显示PopupWindow
/**
- 显示PopupWindow
*/
public PopupWindow show() {
//1, 将弹出窗口需要展示的布局加载进来
//View popupView = View.inflate(mContext, mLayoutId, null);
//2, 创建popupWindow
//参数:view,宽度,高度,是否能获取焦点
if (mView != null) {
mPopupWindow = new PopupWindow(mView, mWidth, mHeight, true);
} else if (mLayoutId != -1) {
mView = View.inflate(mContext, mLayoutId, null);
mPopupWindow = new PopupWindow(mView, mWidth, mHeight, true);
}
//3, 设置显示隐藏的动画
mPopupWindow.setAnimationStyle(mStyle);
//4, 在PopupWindow里面就加上下面代码,让键盘弹出时,不会挡住pop窗口。
mPopupWindow.setInputMethodMode(PopupWindow.INPUT_METHOD_NEEDED);
mPopupWindow.setSoftInputMode(WindowManager.LayoutParams.SOFT_INPUT_ADJUST_RESIZE);
//5, 点击空白处时,隐藏掉pop窗口
mPopupWindow.setFocusable(mFocusable);
//6, 隐藏PopupWindow下面的背景透明度
setBackgroundAlpha(mAlpha);
//7, 设置popupWindow的背景颜色 这里需要设置成透明的 这里设置成透明的
mPopupWindow.setBackgroundDrawable(mBackgroundDrawable);
//8, 添加pop窗口关闭事件
mPopupWindow.setOnDismissListener(this);
//9, 位置
switch (mPopupPosition) {
case LEFT:
mPopupWindow.showAsDropDown(mAnchor, -mPopupWindow.getWidth(), -mPopupWindow
.getHeight()
/ 2 - mAnchor.getHeight() / 2);
break;
case RIGHT:
mPopupWindow.showAsDropDown(mAnchor, mAnchor.getWidth(), (-mPopupWindow
.getHeight()
- (mAnchor.getHeight() >> 1));
break;
case TOP:
mPopupWindow.showAsDropDown(mAnchor,
Math.abs(mAnchor.getWidth() / 2 - mPopupWindow.getWidth() / 2),
-(mPopupWindow.getHeight() + mAnchor.getMeasuredHeight()));
break;
case BOTTOM:
mPopupWindow.showAsDropDown(mAnchor,
Math.abs(mAnchor.getWidth() / 2 - mPopupWindow.getWidth() / 2), 0);
break;
case SCREEN_BOTTOM:
mPopupWindow.showAtLocation(mAnchor, Gravity.BOTTOM, 0, 0);
break;
case SCREEN_TOP:
mPopupWindow.showAtLocation(mAnchor, Gravity.TOP, 0, 0);
break;
default:
mPopupWindow.showAtLocation(mAnchor, Gravity.BOTTOM, 0, 0);
break;
}
return mPopupWindow;
}
完整代码
-
加入注解,代替Java枚举类型
-
提供给外部dismiss()方法,可以控制dismiss()
import android.content.Context;
import android.graphics.drawable.ColorDrawable;
import android.graphics.drawable.Drawable;
import android.support.annotation.IntDef;
import android.support.annotation.NonNull;
import android.support.v7.app.AppCompatActivity;
import android.view.Gravity;
import android.view.View;
import android.view.WindowManager;
import android.widget.PopupWindow;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
/**
-
Created by feiyang on 2017/12/1 12:48
-
Description : 通用的弹出PopupWindow
*/
public class PopupWindowHelper implements PopupWindow.OnDismissListener {
/**
- PopupWindow放在view左边
*/
public static final int LEFT = 1000;
/**
- PopupWindow放在view右边
*/
public static final int RIGHT = 1001;
/**
- PopupWindow放在view上边
*/
public static final int TOP = 1002;
/**
- PopupWindow放在view下边
*/
public static final int BOTTOM = 1003;
/**
- PopupWindow放在屏幕底部
*/
public static final int SCREEN_BOTTOM = 1004;
/**
- PopupWindow放在屏幕顶部
*/
public static final int SCREEN_TOP = 1005;
/**
- popupWindow位置类型 替代Java中的枚举类型
*/
@Retention(RetentionPolicy.SOURCE)
@IntDef({LEFT, RIGHT, TOP, BOTTOM, SCREEN_BOTTOM, SCREEN_TOP})
private @interface PopupPosition {
}
private Context mContext;
private View mAnchor;
private View mView;
private int mLayoutId = -1;
private int mWidth;
private int mHeight;
private int mStyle;
private boolean mFocusable;
private float mAlpha;
private Drawable mBackgroundDrawable;
private int mPopupPosition;
private PopupWindow mPopupWindow;
private PopupWindowHelper(Builder builder) {
this.mContext = builder.context;
this.mAnchor = builder.anchor;
this.mView = builder.showView;
this.mLayoutId = builder.layoutId;
this.mWidth = builder.width;
this.mHeight = builder.height;
this.mStyle = builder.style;
this.mFocusable = builder.focusable;
this.mAlpha = builder.alpha;
this.mBackgroundDrawable = builder.backgroundDrawable;
this.mPopupPosition = builder.popupPosition;
}
/**
- PopupWindow dismiss
*/
public void dismiss() {
if (mPopupWindow != null) {
mPopupWindow.dismiss();
}
}
/**
- 显示PopupWindow
*/
public PopupWindow show() {
//1, 将弹出窗口需要展示的布局加载进来
//View popupView = View.inflate(mContext, mLayoutId, null);
//2, 创建popupWindow
//参数:view,宽度,高度,是否能获取焦点
if (mView != null) {
mPopupWindow = new PopupWindow(mView, mWidth, mHeight, true);
} else if (mLayoutId != -1) {
mView = View.inflate(mContext, mLayoutId, null);
mPopupWindow = new PopupWindow(mView, mWidth, mHeight, true);
}
//3, 设置显示隐藏的动画
mPopupWindow.setAnimationStyle(mStyle);
//4, 在PopupWindow里面就加上下面代码,让键盘弹出时,不会挡住pop窗口。
mPopupWindow.setInputMethodMode(PopupWindow.INPUT_METHOD_NEEDED);
mPopupWindow.setSoftInputMode(WindowManager.LayoutParams.SOFT_INPUT_ADJUST_RESIZE);
//5, 点击空白处时,隐藏掉pop窗口
mPopupWindow.setFocusable(mFocusable);
//6, 隐藏PopupWindow下面的背景透明度
setBackgroundAlpha(mAlpha);
//7, 设置popupWindow的背景颜色 这里需要设置成透明的 这里设置成透明的
mPopupWindow.setBackgroundDrawable(mBackgroundDrawable);
//8, 添加pop窗口关闭事件
mPopupWindow.setOnDismissListener(this);
//9, 位置
switch (mPopupPosition) {
case LEFT:
mPopupWindow.showAsDropDown(mAnchor, -mPopupWindow.getWidth(), -mPopupWindow
.getHeight()
/ 2 - mAnchor.getHeight() / 2);
break;
case RIGHT:
mPopupWindow.showAsDropDown(mAnchor, mAnchor.getWidth(), (-mPopupWindow
.getHeight()
- (mAnchor.getHeight() >> 1));
break;
case TOP:
mPopupWindow.showAsDropDown(mAnchor,
Math.abs(mAnchor.getWidth() / 2 - mPopupWindow.getWidth() / 2),
-(mPopupWindow.getHeight() + mAnchor.getMeasuredHeight()));
break;
case BOTTOM:
mPopupWindow.showAsDropDown(mAnchor,
Math.abs(mAnchor.getWidth() / 2 - mPopupWindow.getWidth() / 2), 0);
break;
case SCREEN_BOTTOM:
mPopupWindow.showAtLocation(mAnchor, Gravity.BOTTOM, 0, 0);
break;
case SCREEN_TOP:
mPopupWindow.showAtLocation(mAnchor, Gravity.TOP, 0, 0);
break;
default:
mPopupWindow.showAtLocation(mAnchor, Gravity.BOTTOM, 0, 0);
break;
}
return mPopupWindow;
}
/**
-
设置添加屏幕的背景透明度
-
@param bgAlpha 0.0-1.0
*/
public void setBackgroundAlpha(float bgAlpha) {
if (mContext instanceof AppCompatActivity) {
AppCompatActivity activity = (AppCompatActivity) mContext;
WindowManager.LayoutParams lp = activity.getWindow().getAttributes();
lp.alpha = bgAlpha; //0.0-1.0
activity.getWindow().setAttributes(lp);
}
}
@Override
public void onDismiss() {
//把背景设置回来
setBackgroundAlpha(1f);
}
public static class Builder {
private Context context;
private View anchor;
private int layoutId = -1;
private View showView;
private int width;
private int height;
private int style;
private boolean focusable;
private float alpha;
private Drawable backgroundDrawable;
private int popupPosition;
/**
- Context (必填)
*/
public Builder context(@NonNull Context context) {
this.context = context;
return this;
}
/**
- 锚 (必填)
*/
public Builder anchor(@NonNull View view) {
this.anchor = view;
return this;
}
/**
- 布局id (布局id和view选填1个)
*/
public Builder layout(int layoutId) {
this.layoutId = layoutId;
return this;
}
/**
- 需要显示的View (布局id和view选填1个)
*/
public Builder view(@NonNull View view) {
this.showView = view;
return this;
}
/**
- PopupWindow宽度
*/
public Builder width(int width) {
this.width = width;
return this;
}
/**
- PopupWindow高度
*/
public Builder height(int height) {
this.height = height;
return this;
}
/**
- PopupWindow style
*/
public Builder style(int style) {
this.style = style;
return this;
}
/**
- PopupWindow点击外部是否取消
*/
public Builder focusable(boolean focusable) {
this.focusable = focusable;
return this;
}
/**
- 透明度
*/
public Builder alpha(float alpha) {
this.alpha = alpha;
return this;
}
/**
- 背景
*/
public Builder backgroundDrawable(Drawable backgroundDrawable) {
this.backgroundDrawable = backgroundDrawable;
return this;
}
/**
-
PopupWindow弹出位置
-
@param popupPosition 可选:LEFT, RIGHT, TOP, BOTTOM, SCREEN_BOTTOM, SCREEN_TOP
*/
public Builder position(@PopupPosition int popupPosition) {
this.popupPosition = popupPosition;
return this;
}
/**
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数初中级Android工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则近万的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Android移动开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Android开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
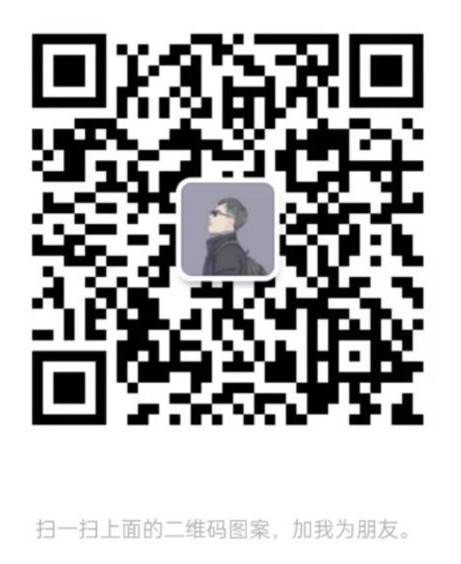
最后
这里附上上述的技术体系图相关的几十套腾讯、头条、阿里、美团等公司2021年的面试题,把技术点整理成了视频和PDF(实际上比预期多花了不少精力),包含知识脉络 + 诸多细节,由于篇幅有限,这里以图片的形式给大家展示一部分。
相信它会给大家带来很多收获:
当程序员容易,当一个优秀的程序员是需要不断学习的,从初级程序员到高级程序员,从初级架构师到资深架构师,或者走向管理,从技术经理到技术总监,每个阶段都需要掌握不同的能力。早早确定自己的职业方向,才能在工作和能力提升中甩开同龄人。
- 无论你现在水平怎么样一定要 持续学习 没有鸡汤,别人看起来的毫不费力,其实费了很大力,这四个字就是我的建议!!!
- 我希望每一个努力生活的IT工程师,都会得到自己想要的,因为我们很辛苦,我们应得的。
当我们在抱怨环境,抱怨怀才不遇的时候,没有别的原因,一定是你做的还不够好!
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
598653297)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Android开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
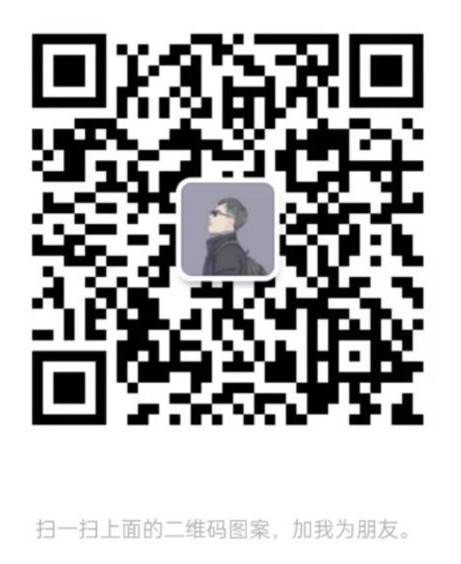
最后
这里附上上述的技术体系图相关的几十套腾讯、头条、阿里、美团等公司2021年的面试题,把技术点整理成了视频和PDF(实际上比预期多花了不少精力),包含知识脉络 + 诸多细节,由于篇幅有限,这里以图片的形式给大家展示一部分。
相信它会给大家带来很多收获:
[外链图片转存中…(img-7AFWnpvq-1713598653298)]
当程序员容易,当一个优秀的程序员是需要不断学习的,从初级程序员到高级程序员,从初级架构师到资深架构师,或者走向管理,从技术经理到技术总监,每个阶段都需要掌握不同的能力。早早确定自己的职业方向,才能在工作和能力提升中甩开同龄人。
- 无论你现在水平怎么样一定要 持续学习 没有鸡汤,别人看起来的毫不费力,其实费了很大力,这四个字就是我的建议!!!
- 我希望每一个努力生活的IT工程师,都会得到自己想要的,因为我们很辛苦,我们应得的。
当我们在抱怨环境,抱怨怀才不遇的时候,没有别的原因,一定是你做的还不够好!
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!