后台管理端包括首页、个人中心、学生管理、班级信息管理、师资力量管理、宿舍信息管理、宿舍安排管理、签到信息管理、在线缴费管理、论坛管理、我的收藏管理、系统管理。
为了更好的去理清本系统整体思路,对该系统以结构图的形式表达出来,设计实现该新生报到系统的功能结构图如下所示:
部分代码展示
/\*\*
\* 报道信息
\* 后端接口
\* @author
\* @email
\* @date 2022-04-26 22:00:28
\*/
@RestController
@RequestMapping("/baodaoxinxi")
public class BaodaoxinxiController {
@Autowired
private BaodaoxinxiService baodaoxinxiService;
/\*\*
\* 后端列表
\*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,BaodaoxinxiEntity baodaoxinxi,
HttpServletRequest request){
String tableName = request.getSession().getAttribute("tableName").toString();
if(tableName.equals("xuesheng")) {
baodaoxinxi.setXuehao((String)request.getSession().getAttribute("username"));
}
EntityWrapper<BaodaoxinxiEntity> ew = new EntityWrapper<BaodaoxinxiEntity>();
PageUtils page = baodaoxinxiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, baodaoxinxi), params), params));
return R.ok().put("data", page);
}
/\*\*
\* 前端列表
\*/
@IgnoreAuth
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,BaodaoxinxiEntity baodaoxinxi,
HttpServletRequest request){
EntityWrapper<BaodaoxinxiEntity> ew = new EntityWrapper<BaodaoxinxiEntity>();
PageUtils page = baodaoxinxiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, baodaoxinxi), params), params));
return R.ok().put("data", page);
}
/\*\*
\* 列表
\*/
@RequestMapping("/lists")
public R list( BaodaoxinxiEntity baodaoxinxi){
EntityWrapper<BaodaoxinxiEntity> ew = new EntityWrapper<BaodaoxinxiEntity>();
ew.allEq(MPUtil.allEQMapPre( baodaoxinxi, "baodaoxinxi"));
return R.ok().put("data", baodaoxinxiService.selectListView(ew));
}
/\*\*
\* 查询
\*/
@RequestMapping("/query")
public R query(BaodaoxinxiEntity baodaoxinxi){
EntityWrapper< BaodaoxinxiEntity> ew = new EntityWrapper< BaodaoxinxiEntity>();
ew.allEq(MPUtil.allEQMapPre( baodaoxinxi, "baodaoxinxi"));
BaodaoxinxiView baodaoxinxiView = baodaoxinxiService.selectView(ew);
return R.ok("查询报道信息成功").put("data", baodaoxinxiView);
}
/\*\*
\* 后端详情
\*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
BaodaoxinxiEntity baodaoxinxi = baodaoxinxiService.selectById(id);
return R.ok().put("data", baodaoxinxi);
}
/\*\*
\* 前端详情
\*/
@IgnoreAuth
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") Long id){
BaodaoxinxiEntity baodaoxinxi = baodaoxinxiService.selectById(id);
return R.ok().put("data", baodaoxinxi);
}
/\*\*
\* 后端保存
\*/
@RequestMapping("/save")
public R save(@RequestBody BaodaoxinxiEntity baodaoxinxi, HttpServletRequest request){
baodaoxinxi.setId(new Date().getTime()+new Double(Math.floor(Math.random()\*1000)).longValue());
//ValidatorUtils.validateEntity(baodaoxinxi);
baodaoxinxiService.insert(baodaoxinxi);
return R.ok();
}
/\*\*
\* 前端保存
\*/
@RequestMapping("/add")
public R add(@RequestBody BaodaoxinxiEntity baodaoxinxi, HttpServletRequest request){
baodaoxinxi.setId(new Date().getTime()+new Double(Math.floor(Math.random()\*1000)).longValue());
//ValidatorUtils.validateEntity(baodaoxinxi);
baodaoxinxiService.insert(baodaoxinxi);
return R.ok();
}
/\*\*
\* 修改
\*/
@RequestMapping("/update")
@Transactional
public R update(@RequestBody BaodaoxinxiEntity baodaoxinxi, HttpServletRequest request){
//ValidatorUtils.validateEntity(baodaoxinxi);
baodaoxinxiService.updateById(baodaoxinxi);//全部更新
return R.ok();
}
/\*\*
\* 删除
\*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
baodaoxinxiService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/\*\*
\* 提醒接口
\*/
@RequestMapping("/remind/{columnName}/{type}")
public R remindCount(@PathVariable("columnName") String columnName, HttpServletRequest request,
@PathVariable("type") String type,@RequestParam Map<String, Object> map) {
map.put("column", columnName);
map.put("type", type);
if(type.equals("2")) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Calendar c = Calendar.getInstance();
Date remindStartDate = null;
Date remindEndDate = null;
if(map.get("remindstart")!=null) {
Integer remindStart = Integer.parseInt(map.get("remindstart").toString());
c.setTime(new Date());
c.add(Calendar.DAY\_OF\_MONTH,remindStart);
remindStartDate = c.getTime();
map.put("remindstart", sdf.format(remindStartDate));
}
if(map.get("remindend")!=null) {
Integer remindEnd = Integer.parseInt(map.get("remindend").toString());
c.setTime(new Date());
c.add(Calendar.DAY\_OF\_MONTH,remindEnd);
remindEndDate = c.getTime();
map.put("remindend", sdf.format(remindEndDate));
}
}
Wrapper<BaodaoxinxiEntity> wrapper = new EntityWrapper<BaodaoxinxiEntity>();
if(map.get("remindstart")!=null) {
wrapper.ge(columnName, map.get("remindstart"));
}
if(map.get("remindend")!=null) {
wrapper.le(columnName, map.get("remindend"));
}
### 最后
不知道你们用的什么环境,我一般都是用的Python3.6环境和pycharm解释器,没有软件,或者没有资料,没人解答问题,都可以免费领取(包括今天的代码),过几天我还会做个视频教程出来,有需要也可以领取~
给大家准备的学习资料包括但不限于:
Python 环境、pycharm编辑器/永久激活/翻译插件
python 零基础视频教程
Python 界面开发实战教程
Python 爬虫实战教程
Python 数据分析实战教程
python 游戏开发实战教程
Python 电子书100本
Python 学习路线规划
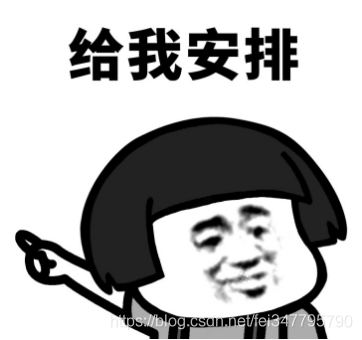
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化学习资料的朋友,可以戳这里无偿获取](https://bbs.csdn.net/topics/618317507)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**