- 创建Firebase项目
# Firebase控制台:https://console.firebase.google.com
# 创建流程
1. 在 Firebase 控制台中,点击添加项目
2. 如果出现 Firebase 条款提示,请查看并接受。
3. 点击继续。
4. 为您的项目设置 Google Analytics(可选)
5. 点击创建项目(如果使用现有的 Google Cloud 项目,则点击添加 Firebase)
- 创建包含服务帐号凭据的配置文件
1. 在 Firebase 控制台中,打开设置 > 服务帐号。
2. 点击生成新的私钥,然后点击生成密钥进行确认。
3. 妥善存储包含密钥的 JSON 文件.
- 添加SDK
Firebase Admin Python SDK 可通过 pip 获得。
pip3 install firebase-admin
- 初始化SDK
通过服务帐号进行授权时,有两种方式可为您的应用提供凭据。
方式1(推荐)
(1)将环境变量 GOOGLE_APPLICATION_CREDENTIALS 设置为包含服务帐号密钥的 JSON 文件的文件路径
export GOOGLE_APPLICATION_CREDENTIALS="/home/user/Downloads/service-account-file.json"
(2)完成上述步骤后,应用默认凭据 (ADC) 能够隐式确定您的凭据
import firebase_admin
default_app = firebase_admin.initialize_app()
方式2:直接在代码中写死JSON文件路径
import firebase_admin
from firebase_admin import credentials
cred = credentials.Certificate("path/to/service_account.json")
default_app = firebase_admin.initialize_app(credential=cred)
- 向指定设备发送消息
from firebase_admin import messaging
# This registration token comes from the client FCM SDKs.
registration_token = 'YOUR_REGISTRATION_TOKEN'
# See documentation on defining a message payload.
message = messaging.Message(
notification=messaging.Notification(
title='your_titme',
body='your_body',
image='your_img',
),
token=registration_token,
)
# Send a message to the device corresponding to the provided registration token.
response = messaging.send(message)
# Response is a message ID string.
print('Successfully sent message:', response)
- 向主题发送消息
创建主题后,使用服务器 API向主题发送消息,则订阅过该主题的设备都会受到消息。
from firebase_admin import messaging
# The topic name can be optionally prefixed with "/topics/".
topic = 'highScores'
# See documentation on defining a message payload.
message = messaging.Message(
notification=messaging.Notification(
title='your_titme',
body='your_body',
image='your_img',
),
topic=topic,
)
# Send a message to the devices subscribed to the provided topic.
response = messaging.send(message)
# Response is a message ID string.
print('Successfully sent message:', response)
- 批量发送消息
# Create a list containing up to 500 messages.
messages = [
messaging.Message(
notification=messaging.Notification('Price drop', '5% off all electronics'),
token=registration_token,
),
# ...
messaging.Message(
notification=messaging.Notification('Price drop', '2% off all books'),
topic='readers-club',
),
]
response = messaging.send_all(messages)
# See the BatchResponse reference documentation
# for the contents of response.
print('{0} messages were sent successfully'.format(response.success_count))
- 服务端订阅主题
客户端SDK可以帮用户订阅主题,服务端可以通过接口帮用户订阅主题。
您可以为客户端应用实例订阅任何现有主题,也可创建新主题。当您使用 API 为客户端应用订阅新主题(您的 Firebase 项目中尚不存在的主题)时,系统会在 FCM 中创建一个使用该名称的新主题,随后任何客户端都可订阅该主题。
# 您可以将注册令牌列表传递给 Firebase Admin SDK 订阅方法,以便为相应的设备订阅主题
# These registration tokens come from the client FCM SDKs.
registration_tokens = [
'YOUR_REGISTRATION_TOKEN_1',
# ...
'YOUR_REGISTRATION_TOKEN_n',
]
# Subscribe the devices corresponding to the registration tokens to the
# topic.
response = messaging.subscribe_to_topic(registration_tokens, topic)
# See the TopicManagementResponse reference documentation
# for the contents of response.
print(response.success_count, 'tokens were subscribed successfully')
注意:在单次请求中,您最多可以为 1000 台设备订阅或退订主题。
- 服务端推订主题
利用 Admin FCM API,您还可以将注册令牌传递给相应的方法,来为设备退订主题
# These registration tokens come from the client FCM SDKs.
registration_tokens = [
'YOUR_REGISTRATION_TOKEN_1',
# ...
'YOUR_REGISTRATION_TOKEN_n',
]
### 一、Python所有方向的学习路线
Python所有方向路线就是把Python常用的技术点做整理,形成各个领域的知识点汇总,它的用处就在于,你可以按照上面的知识点去找对应的学习资源,保证自己学得较为全面。
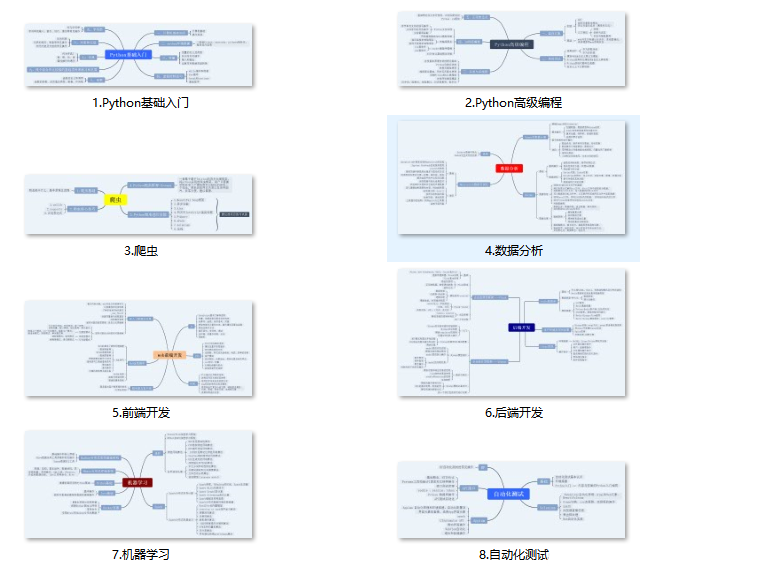
### 二、学习软件
工欲善其事必先利其器。学习Python常用的开发软件都在这里了,给大家节省了很多时间。
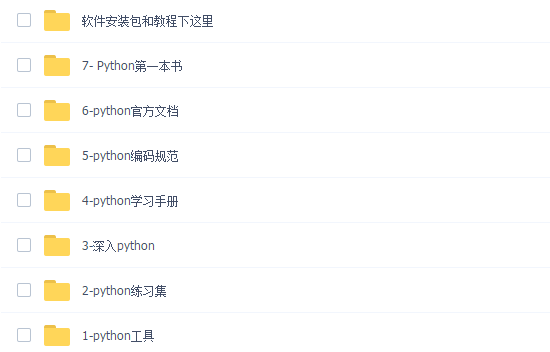
### 三、入门学习视频
我们在看视频学习的时候,不能光动眼动脑不动手,比较科学的学习方法是在理解之后运用它们,这时候练手项目就很适合了。
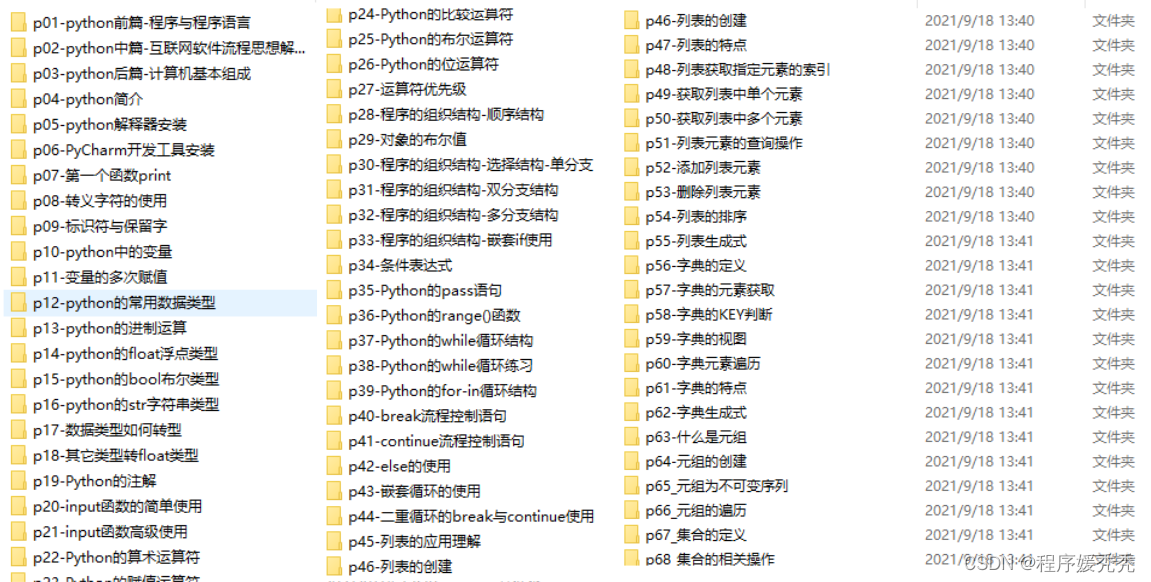