代码实现:
package com.demo.progressbarwithpercent.view;
import com.demo.progressbarwithpercent.R;
import android.content.Context;
import android.content.res.TypedArray;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.Paint;
import android.graphics.RectF;
import android.os.Bundle;
import android.os.Parcelable;
import android.util.AttributeSet;
import android.view.View;
public class ProgressBarWithPercent extends View {
private int mMaxProgress = 100;
/**
- Current progress, can not exceed the max progress.
*/
private int mCurrentProgress = 0;
/**
- The progress area bar color.
*/
private int mReachedBarColor;
/**
- The bar unreached area color.
*/
private int mUnreachedBarColor;
/**
- The progress text color.
*/
private int mTextColor;
/**
- The progress text size.
*/
private float mTextSize;
/**
- The height of the reached area.
*/
private float mReachedBarHeight;
/**
- The height of the unreached area.
*/
private float mUnreachedBarHeight;
/**
- The suffix of the number.
*/
private String mSuffix = “%”;
/**
- The prefix.
*/
private String mPrefix = “”;
private final int default_text_color = Color.rgb(66, 145, 241);
private final int default_reached_color = Color.rgb(66, 145, 241);
private final int default_unreached_color = Color.rgb(204, 204, 204);
private final float default_progress_text_offset;
private final float default_text_size;
private final float default_reached_bar_height;
private final float default_unreached_bar_height;
/**
- For save and restore instance of progressbar.
*/
private static final String INSTANCE_STATE = “saved_instance”;
private static final String INSTANCE_TEXT_COLOR = “text_color”;
private static final String INSTANCE_TEXT_SIZE = “text_size”;
private static final String INSTANCE_REACHED_BAR_HEIGHT = “reached_bar_height”;
private static final String INSTANCE_REACHED_BAR_COLOR = “reached_bar_color”;
private static final String INSTANCE_UNREACHED_BAR_HEIGHT = “unreached_bar_height”;
private static final String INSTANCE_UNREACHED_BAR_COLOR = “unreached_bar_color”;
private static final String INSTANCE_MAX = “max”;
private static final String INSTANCE_PROGRESS = “progress”;
private static final String INSTANCE_SUFFIX = “suffix”;
private static final String INSTANCE_PREFIX = “prefix”;
private static final String INSTANCE_TEXT_VISIBILITY = “text_visibility”;
private static final int PROGRESS_TEXT_VISIBLE = 0;
/**
- The width of the text that to be drawn.
*/
private float mDrawTextWidth;
/**
- The drawn text start.
*/
private float mDrawTextStart;
/**
- The drawn text end.
*/
private float mDrawTextEnd;
/**
- The text that to be drawn in onDraw().
*/
private String mCurrentDrawText;
/**
- The Paint of the reached area.
*/
private Paint mReachedBarPaint;
/**
- The Paint of the unreached area.
*/
private Paint mUnreachedBarPaint;
/**
- The Paint of the progress text.
*/
private Paint mTextPaint;
/**
- Unreached bar area to draw rect.
*/
private RectF mUnreachedRectF = new RectF(0, 0, 0, 0);
/**
- Reached bar area rect.
*/
private RectF mReachedRectF = new RectF(0, 0, 0, 0);
/**
- The progress text offset.
*/
private float mOffset;
/**
- Determine if need to draw unreached area.
*/
private boolean mDrawUnreachedBar = true;
private boolean mDrawReachedBar = true;
private boolean mIfDrawText = true;
/**
- Listener
*/
private OnProgressBarListener mListener;
public enum ProgressTextVisibility {
Visible, Invisible
}
public ProgressBarWithPercent(Context context) {
this(context, null);
}
public ProgressBarWithPercent(Context context, AttributeSet attrs) {
this(context, attrs, 0);
}
public ProgressBarWithPercent(Context context, AttributeSet attrs,
int defStyleAttr) {
super(context, attrs, defStyleAttr);
default_reached_bar_height = dp2px(1.5f);
default_unreached_bar_height = dp2px(1.0f);
default_text_size = sp2px(10);
default_progress_text_offset = dp2px(3.0f);
// load styled attributes.
final TypedArray attributes = context.getTheme()
.obtainStyledAttributes(attrs,
R.styleable.ProgressBarWithPercent, defStyleAttr, 0);
mReachedBarColor = attributes.getColor(
R.styleable.ProgressBarWithPercent_progress_reached_color,
default_reached_color);
mUnreachedBarColor = attributes.getColor(
R.styleable.ProgressBarWithPercent_progress_unreached_color,
default_unreached_color);
mTextColor = attributes.getColor(
R.styleable.ProgressBarWithPercent_progress_text_color,
default_text_color);
mTextSize = attributes.getDimension(
R.styleable.ProgressBarWithPercent_progress_text_size,
default_text_size);
mReachedBarHeight = attributes.getDimension(
R.styleable.ProgressBarWithPercent_progress_reached_bar_height,
default_reached_bar_height);
mUnreachedBarHeight = attributes
.getDimension(
R.styleable.ProgressBarWithPercent_progress_unreached_bar_height,
default_unreached_bar_height);
mOffset = attributes.getDimension(
R.styleable.ProgressBarWithPercent_progress_text_offset,
default_progress_text_offset);
int textVisible = attributes.getInt(
R.styleable.ProgressBarWithPercent_progress_text_visibility,
PROGRESS_TEXT_VISIBLE);
if (textVisible != PROGRESS_TEXT_VISIBLE) {
mIfDrawText = false;
}
setProgress(attributes.getInt(
R.styleable.ProgressBarWithPercent_progress_current, 0));
setMax(attributes.getInt(
R.styleable.ProgressBarWithPercent_progress_max, 100));
attributes.recycle();
initializePainters();
}
@Override
protected int getSuggestedMinimumWidth() {
return (int) mTextSize;
}
@Override
protected int getSuggestedMinimumHeight() {
return Math.max((int) mTextSize,
Math.max((int) mReachedBarHeight, (int) mUnreachedBarHeight));
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
setMeasuredDimension(measure(widthMeasureSpec, true),
measure(heightMeasureSpec, false));
}
private int measure(int measureSpec, boolean isWidth) {
int result;
int mode = MeasureSpec.getMode(measureSpec);
int size = MeasureSpec.getSize(measureSpec);
-
int padding = isWidth ? getPaddingLeft() + getPaddingRight()
- getPaddingTop() + getPaddingBottom();
if (mode == MeasureSpec.EXACTLY) {
result = size;
} else {
-
result = isWidth ? getSuggestedMinimumWidth()
- getSuggestedMinimumHeight();
result += padding;
if (mode == MeasureSpec.AT_MOST) {
if (isWidth) {
result = Math.max(result, size);
} else {
result = Math.min(result, size);
}
}
}
return result;
}
@Override
protected void onDraw(Canvas canvas) {
if (mIfDrawText) {
calculateDrawRectF();
} else {
calculateDrawRectFWithoutProgressText();
}
if (mDrawReachedBar) {
canvas.drawRect(mReachedRectF, mReachedBarPaint);
}
if (mDrawUnreachedBar) {
canvas.drawRect(mUnreachedRectF, mUnreachedBarPaint);
}
if (mIfDrawText)
canvas.drawText(mCurrentDrawText, mDrawTextStart, mDrawTextEnd,
mTextPaint);
}
private void initializePainters() {
mReachedBarPaint = new Paint(Paint.ANTI_ALIAS_FLAG);
mReachedBarPaint.setColor(mReachedBarColor);
mUnreachedBarPaint = new Paint(Paint.ANTI_ALIAS_FLAG);
mUnreachedBarPaint.setColor(mUnreachedBarColor);
mTextPaint = new Paint(Paint.ANTI_ALIAS_FLAG);
mTextPaint.setColor(mTextColor);
mTextPaint.setTextSize(mTextSize);
}
private void calculateDrawRectFWithoutProgressText() {
mReachedRectF.left = getPaddingLeft();
mReachedRectF.top = getHeight() / 2.0f - mReachedBarHeight / 2.0f;
mReachedRectF.right = (getWidth() - getPaddingLeft() - getPaddingRight())
/ (getMax() * 1.0f) * getProgress() + getPaddingLeft();
mReachedRectF.bottom = getHeight() / 2.0f + mReachedBarHeight / 2.0f;
mUnreachedRectF.left = mReachedRectF.right;
mUnreachedRectF.right = getWidth() - getPaddingRight();
mUnreachedRectF.top = getHeight() / 2.0f + -mUnreachedBarHeight / 2.0f;
mUnreachedRectF.bottom = getHeight() / 2.0f + mUnreachedBarHeight
/ 2.0f;
}
private void calculateDrawRectF() {
mCurrentDrawText = String.format(“%d”, getProgress() * 100 / getMax());
mCurrentDrawText = mPrefix + mCurrentDrawText + mSuffix;
mDrawTextWidth = mTextPaint.measureText(mCurrentDrawText);
if (getProgress() == 0) {
mDrawReachedBar = false;
mDrawTextStart = getPaddingLeft();
} else {
mDrawReachedBar = true;
mReachedRectF.left = getPaddingLeft();
mReachedRectF.top = getHeight() / 2.0f - mReachedBarHeight / 2.0f;
mReachedRectF.right = (getWidth() - getPaddingLeft() - getPaddingRight())
/ (getMax() * 1.0f)
- getProgress()
- mOffset
- getPaddingLeft();
mReachedRectF.bottom = getHeight() / 2.0f + mReachedBarHeight
/ 2.0f;
mDrawTextStart = (mReachedRectF.right + mOffset);
}
mDrawTextEnd = (int) ((getHeight() / 2.0f) - ((mTextPaint.descent() + mTextPaint
.ascent()) / 2.0f));
if ((mDrawTextStart + mDrawTextWidth) >= getWidth() - getPaddingRight()) {
mDrawTextStart = getWidth() - getPaddingRight() - mDrawTextWidth;
mReachedRectF.right = mDrawTextStart - mOffset;
}
float unreachedBarStart = mDrawTextStart + mDrawTextWidth + mOffset;
if (unreachedBarStart >= getWidth() - getPaddingRight()) {
mDrawUnreachedBar = false;
} else {
mDrawUnreachedBar = true;
mUnreachedRectF.left = unreachedBarStart;
mUnreachedRectF.right = getWidth() - getPaddingRight();
mUnreachedRectF.top = getHeight() / 2.0f + -mUnreachedBarHeight
/ 2.0f;
mUnreachedRectF.bottom = getHeight() / 2.0f + mUnreachedBarHeight
/ 2.0f;
}
}
/**
-
Get progress text color.
-
@return progress text color.
*/
public int getTextColor() {
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数初中级Android工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则近万的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Android移动开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Android开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
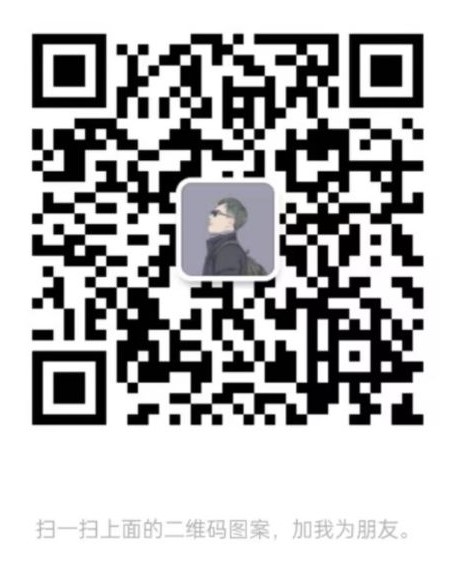
最后是今天给大家分享的一些独家干货:
【Android开发核心知识点笔记】
【Android思维脑图(技能树)】
【Android核心高级技术PDF文档,BAT大厂面试真题解析】
【Android高级架构视频学习资源】
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
6989)]
[外链图片转存中…(img-crW479ph-1712359256989)]
[外链图片转存中…(img-WC2fSIOX-1712359256990)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Android开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
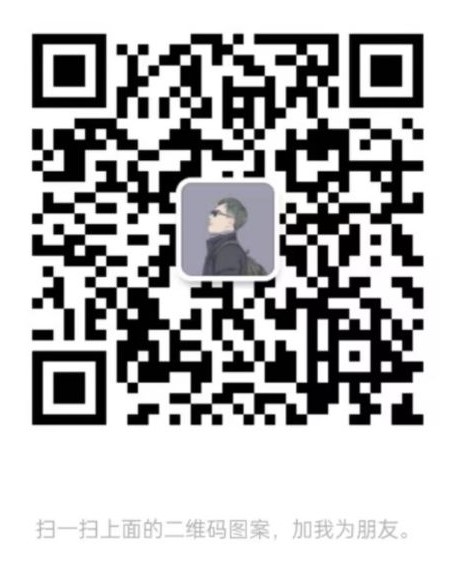
最后是今天给大家分享的一些独家干货:
【Android开发核心知识点笔记】
[外链图片转存中…(img-4K3E9PFi-1712359256990)]
【Android思维脑图(技能树)】
[外链图片转存中…(img-El7HGBla-1712359256990)]
【Android核心高级技术PDF文档,BAT大厂面试真题解析】
[外链图片转存中…(img-KytXpSs8-1712359256990)]
【Android高级架构视频学习资源】
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!