public String getOrderNumber() {
return orderNumber;
}
public void setOrderNumber(String orderNumber) {
this.orderNumber = orderNumber;
}
public String getCategory() {
return category;
}
public void setCategory(String category) {
this.category = category;
}
}
3.3xm sax解析l的handler如下:
-
package com.uim.microblog.net.handler;
-
import java.util.ArrayList;
-
import java.util.List;
-
import org.xml.sax.Attributes;
-
import org.xml.sax.SAXException;
-
import org.xml.sax.helpers.DefaultHandler;
-
import com.uim.microblog.model.Emotions;
-
import com.uim.microblog.model.ResponseResult;
-
public class BlogEmotionsHandler extends DefaultHandler {
-
private List list;
-
private Emotions emotions;
-
private ResponseResult responseresult;
-
private String tag = null;//正在解析的元素
-
public List getEmotionsList(){
-
return list;
-
}
-
@Override
-
public void characters(char[] ch, int start, int length)
-
throws SAXException {
-
if (tag != null) {
-
String textArea = new String(ch,start,length);
-
/**开始解析表情数据*/
-
if (“phrase”.equals(tag)) {
-
emotions.setPhrase(textArea);
-
} else if (“type”.equals(tag)) {
-
emotions.setType(textArea);
-
} else if (“url”.equals(tag)) {
-
try {
-
emotions.setUrl(textArea);
-
String imageName = textArea.substring(textArea.lastIndexOf(“/”) + 1,textArea.length() - 4);
-
emotions.setImageName(imageName);
-
} catch (Exception e) {
-
e.printStackTrace();
-
}
-
} else if (“is_hot”.equals(tag)) {
-
emotions.setIsHot(textArea);
-
} else if (“is_common”.equals(tag)) {
-
emotions.setIsCommon(textArea);
-
} else if (“order_number”.equals(tag)) {
-
emotions.setOrderNumber(textArea);
-
} else if (“category”.equals(tag)) {
-
emotions.setCategory(textArea);
-
} else if (“retn”.equals(tag)) {
-
responseresult.setRetn(textArea);
-
} else if (“desc”.equals(tag)) {
-
responseresult.setDesc(textArea);
-
}
-
}
-
}
-
@Override
-
public void endDocument() throws SAXException {
-
super.endDocument();
-
}
-
@Override
-
public void endElement(String uri, String localName, String qName)
-
throws SAXException {
-
tag = null;
-
if (“mb”.equals(localName)) {
-
} else if (“emotions”.equals(localName)) {
-
responseresult =null;
-
} else if (“emotion”.equals(localName)) {
-
list.add(emotions);
-
emotions = null;
-
}
-
}
-
@Override
-
public void startDocument() throws SAXException {
-
list = new ArrayList();
-
}
-
@Override
-
public void startElement(String uri, String localName, String qName,
-
Attributes attributes) throws SAXException {
-
if (“mb”.equals(localName)) {
-
responseresult = new ResponseResult();
-
} else if (“emotions”.equals(localName)) {
-
} else if (“emotion”.equals(localName)) {
-
emotions = new Emotions();
-
}
-
tag = localName;
-
}
-
}
package com.uim.microblog.net.handler;
import java.util.ArrayList;
import java.util.List;
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.helpers.DefaultHandler;
import com.uim.microblog.model.Emotions;
import com.uim.microblog.model.ResponseResult;
public class BlogEmotionsHandler extends DefaultHandler {
private List list;
private Emotions emotions;
private ResponseResult responseresult;
private String tag = null;//正在解析的元素
public List getEmotionsList(){
return list;
}
@Override
public void characters(char[] ch, int start, int length)
throws SAXException {
if (tag != null) {
String textArea = new String(ch,start,length);
/*开始解析表情数据/
if (“phrase”.equals(tag)) {
emotions.setPhrase(textArea);
} else if (“type”.equals(tag)) {
emotions.setType(textArea);
} else if (“url”.equals(tag)) {
try {
emotions.setUrl(textArea);
String imageName = textArea.substring(textArea.lastIndexOf(“/”) + 1,textArea.length() - 4);
emotions.setImageName(imageName);
} catch (Exception e) {
e.printStackTrace();
}
} else if (“is_hot”.equals(tag)) {
emotions.setIsHot(textArea);
} else if (“is_common”.equals(tag)) {
emotions.setIsCommon(textArea);
} else if (“order_number”.equals(tag)) {
emotions.setOrderNumber(textArea);
} else if (“category”.equals(tag)) {
emotions.setCategory(textArea);
} else if (“retn”.equals(tag)) {
responseresult.setRetn(textArea);
} else if (“desc”.equals(tag)) {
responseresult.setDesc(textArea);
}
}
}
@Override
public void endDocument() throws SAXException {
super.endDocument();
}
@Override
public void endElement(String uri, String localName, String qName)
throws SAXException {
tag = null;
if (“mb”.equals(localName)) {
} else if (“emotions”.equals(localName)) {
responseresult =null;
} else if (“emotion”.equals(localName)) {
list.add(emotions);
emotions = null;
}
}
@Override
public void startDocument() throws SAXException {
list = new ArrayList();
}
@Override
public void startElement(String uri, String localName, String qName,
Attributes attributes) throws SAXException {
if (“mb”.equals(localName)) {
responseresult = new ResponseResult();
} else if (“emotions”.equals(localName)) {
} else if (“emotion”.equals(localName)) {
emotions = new Emotions();
}
tag = localName;
}
}
3.4sax解析
-
public List getEmotion(){
-
BlogGetData getdata = new BlogGetData();
-
String result = getdata.blogEmotionsServlet();
-
try {
-
//生成SAX解析对象
-
parser = SAXParserFactory.newInstance().newSAXParser();
-
//生成xml读取器
-
reader = parser.getXMLReader();
-
BlogEmotionsHandler handler = new BlogEmotionsHandler();
-
//设置Handler
-
reader.setContentHandler(handler);
-
//指定文件,进行解析
-
reader.parse(new InputSource(new StringReader(result)));
-
//获取 List
-
emotionList = handler.getEmotionsList();
-
} catch (ParserConfigurationException e) {
-
e.printStackTrace();
-
} catch (SAXException e) {
-
e.printStackTrace();
-
} catch (IOException e) {
-
e.printStackTrace();
-
}
-
return emotionList;
-
}
public List getEmotion(){
BlogGetData getdata = new BlogGetData();
String result = getdata.blogEmotionsServlet();
try {
//生成SAX解析对象
parser = SAXParserFactory.newInstance().newSAXParser();
//生成xml读取器
reader = parser.getXMLReader();
BlogEmotionsHandler handler = new BlogEmotionsHandler();
//设置Handler
reader.setContentHandler(handler);
//指定文件,进行解析
reader.parse(new InputSource(new StringReader(result)));
//获取 List
emotionList = handler.getEmotionsList();
} catch (ParserConfigurationException e) {
e.printStackTrace();
} catch (SAXException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return emotionList;
}
4:
4.1实现表情选择器—GridView
-
<GridView
-
android:id=“@+id/blog_sendmsg_gvemotion”
-
android:layout_width=“fill_parent”
-
android:layout_height=“150sp”
-
android:scrollbars=“vertical”
-
android:numColumns=“auto_fit”
-
android:verticalSpacing=“15dp”
-
android:background=“@color/blog_list_back”
-
android:stretchMode=“columnWidth”
-
android:gravity=“center”
-
android:visibility=“gone”
-
android:columnWidth=“40dp”>
-
</GridView>
<GridView
android:id=“@+id/blog_sendmsg_gvemotion”
android:layout_width=“fill_parent”
android:layout_height=“150sp”
android:scrollbars=“vertical”
android:numColumns=“auto_fit”
android:verticalSpacing=“15dp”
android:background=“@color/blog_list_back”
android:stretchMode=“columnWidth”
android:gravity=“center”
android:visibility=“gone”
android:columnWidth=“40dp”>
4.2 GridView的item-----gridview_emotion_item.xml
- <?xml version\="1.0" encoding\="utf-8"?>
-
<LinearLayout
-
xmlns:android=“http://schemas.android.com/apk/res/android”
-
android:layout_width=“fill_parent”
-
android:layout_height=“fill_parent”>
-
<ImageView
-
android:id=“@+id/blog_sendmsg_emotion”
-
android:layout_width=“wrap_content”
-
android:layout_height=“wrap_content”
-
android:layout_weight=“50”
-
android:layout_gravity=“center”>
-
</ImageView>
-
</LinearLayout>
<LinearLayout
xmlns:android=“http://schemas.android.com/apk/res/android”
android:layout_width=“fill_parent”
android:layout_height=“fill_parent”>
<ImageView
android:id=“@+id/blog_sendmsg_emotion”
android:layout_width=“wrap_content”
android:layout_height=“wrap_content”
android:layout_weight=“50”
android:layout_gravity=“center”>
4.3代码加载表情图片到GridView进行显示
-
public void addexpression(View view){
-
if (expressionGriView.getVisibility() == View.GONE) {
-
expressionGriView.setVisibility(View.VISIBLE);
-
emotionList = BlogHomeActivity.emotions;
-
ArrayList<HashMap<String, Object>> lstImageItem = new ArrayList<HashMap<String, Object>>();
-
for(int i=0;i<70;i++)
-
{
-
emtions = emotionList.get(i);
-
if (emtions != null) {
-
HashMap<String, Object> map = new HashMap<String, Object>();
-
Field f;
-
try {
-
f = (Field)R.drawable.class.getDeclaredField(emtions.getImageName());
-
int j = f.getInt(R.drawable.class);
-
map.put(“ItemImage”, j);//添加图像资源的ID
-
lstImageItem.add(map);
-
} catch (SecurityException e) {
-
e.printStackTrace();
-
} catch (NoSuchFieldException e) {
-
e.printStackTrace();
-
}catch (IllegalArgumentException e) {
-
e.printStackTrace();
-
} catch (IllegalAccessException e) {
-
e.printStackTrace();
-
}
-
}
-
}
-
//生成适配器的ImageItem <====> 动态数组的元素,两者一一对应
-
SimpleAdapter saImageItems = new SimpleAdapter(this,
-
lstImageItem,//数据来源
-
R.layout.blog_emotion_list,
-
//动态数组与ImageItem对应的子项
-
new String[] {“ItemImage”},
-
//ImageItem的XML文件里面的一个ImageView
-
new int[] {R.id.blog_sendmsg_emotion});
-
expressionGriView.setAdapter(saImageItems);
-
} else {
-
expressionGriView.setVisibility(View.GONE);
-
}
-
}
public void addexpression(View view){
if (expressionGriView.getVisibility() == View.GONE) {
expressionGriView.setVisibility(View.VISIBLE);
emotionList = BlogHomeActivity.emotions;
ArrayList<HashMap<String, Object>> lstImageItem = new ArrayList<HashMap<String, Object>>();
for(int i=0;i<70;i++)
{
emtions = emotionList.get(i);
if (emtions != null) {
HashMap<String, Object> map = new HashMap<String, Object>();
Field f;
try {
f = (Field)R.drawable.class.getDeclaredField(emtions.getImageName());
int j = f.getInt(R.drawable.class);
map.put(“ItemImage”, j);//添加图像资源的ID
lstImageItem.add(map);
} catch (SecurityException e) {
e.printStackTrace();
} catch (NoSuchFieldException e) {
e.printStackTrace();
}catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
}
//生成适配器的ImageItem <====> 动态数组的元素,两者一一对应
SimpleAdapter saImageItems = new SimpleAdapter(this,
lstImageItem,//数据来源
R.layout.blog_emotion_list,
//动态数组与ImageItem对应的子项
new String[] {“ItemImage”},
//ImageItem的XML文件里面的一个ImageView
new int[] {R.id.blog_sendmsg_emotion});
expressionGriView.setAdapter(saImageItems);
} else {
expressionGriView.setVisibility(View.GONE);
}
}
5:实现点击GridView的每一个item,处理根据item的index查找对应的表情code,然后再把code利用正则把code转换为相对应的表情图片,最后表情插入EditText进行发送
5.1:code转换为图片:
-
public SpannableString txtToImg(String content){
-
SpannableString ss = new SpannableString(content);
-
int starts = 0;
-
int end = 0;
-
if(content.indexOf(“[”, starts) != -1 && content.indexOf(“]”, end) != -1){
-
starts = content.indexOf(“[”, starts);
-
end = content.indexOf(“]”, end);
-
String phrase = content.substring(starts,end + 1);
-
String imageName = “”;
-
List list = BlogHomeActivity.emotions;
-
for (Emotions emotions : list) {
-
if (emotions.getPhrase().equals(phrase)) {
-
imageName = emotions.getImageName();
-
}
-
}
-
try {
-
Field f = (Field)R.drawable.class.getDeclaredField(imageName);
-
int i= f.getInt(R.drawable.class);
-
Drawable drawable = BlogSendMsgActivity.this.getResources().getDrawable(i);
-
if (drawable != null) {
-
drawable.setBounds(0, 0, drawable.getIntrinsicWidth(), drawable.getIntrinsicHeight());
-
ImageSpan span = new ImageSpan(drawable, ImageSpan.ALIGN_BASELINE);
-
ss.setSpan(span, starts,end + 1, Spannable.SPAN_EXCLUSIVE_EXCLUSIVE);
-
}
-
} catch (SecurityException e) {
-
e.printStackTrace();
-
} catch (NoSuchFieldException e) {
-
e.printStackTrace();
-
} catch (IllegalArgumentException e) {
-
e.printStackTrace();
-
} catch (IllegalAccessException e) {
-
}
-
}
-
return ss;
-
}
public SpannableString txtToImg(String content){
SpannableString ss = new SpannableString(content);
int starts = 0;
int end = 0;
if(content.indexOf(“[”, starts) != -1 && content.indexOf(“]”, end) != -1){
starts = content.indexOf(“[”, starts);
end = content.indexOf(“]”, end);
String phrase = content.substring(starts,end + 1);
String imageName = “”;
List list = BlogHomeActivity.emotions;
for (Emotions emotions : list) {
if (emotions.getPhrase().equals(phrase)) {
imageName = emotions.getImageName();
}
}
try {
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数初中级Android工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则近万的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Android移动开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Android开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
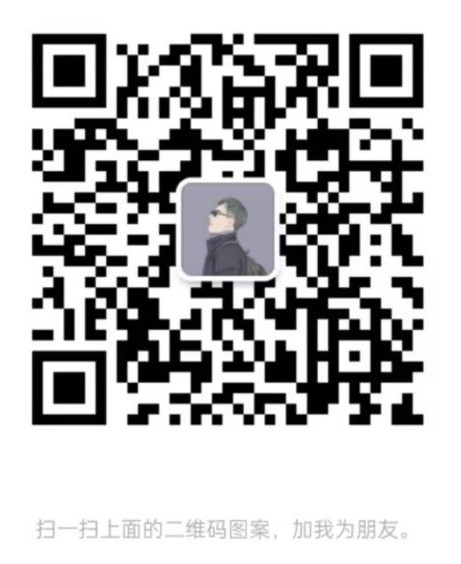
总结:
各行各样都会淘汰一些能力差的,不仅仅是IT这个行业,所以,不要被程序猿是吃青春饭等等这类话题所吓倒,也不要觉得,找到一份工作,就享受安逸的生活,你在安逸的同时,别人正在奋力的向前跑,这样与别人的差距也就会越来越遥远,加油,希望,我们每一个人,成为更好的自己。
-
BAT大厂面试题、独家面试工具包,
-
资料包括 数据结构、Kotlin、计算机网络、Framework源码、数据结构与算法、小程序、NDK、Flutter
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。**
深知大多数初中级Android工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则近万的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Android移动开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
[外链图片转存中…(img-URivxxWY-1712356171499)]
[外链图片转存中…(img-b4DHgrU2-1712356171499)]
[外链图片转存中…(img-YnrTXxT0-1712356171500)]
[外链图片转存中…(img-aRfOe29X-1712356171500)]
[外链图片转存中…(img-bNII6mTi-1712356171500)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Android开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
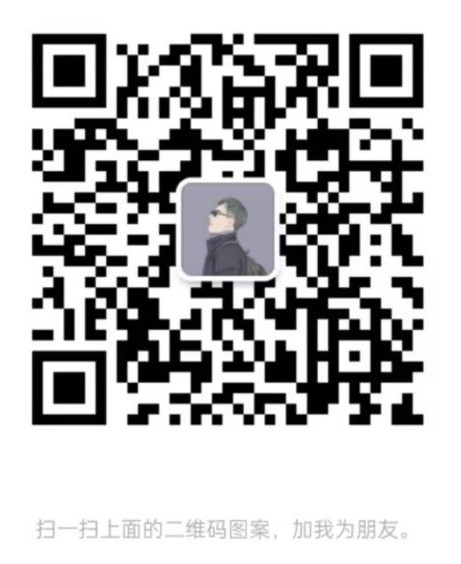
总结:
各行各样都会淘汰一些能力差的,不仅仅是IT这个行业,所以,不要被程序猿是吃青春饭等等这类话题所吓倒,也不要觉得,找到一份工作,就享受安逸的生活,你在安逸的同时,别人正在奋力的向前跑,这样与别人的差距也就会越来越遥远,加油,希望,我们每一个人,成为更好的自己。
-
BAT大厂面试题、独家面试工具包,
-
资料包括 数据结构、Kotlin、计算机网络、Framework源码、数据结构与算法、小程序、NDK、Flutter
[外链图片转存中…(img-5UxpTLmo-1712356171500)]
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!