### 3.尾插
void SLPushBack(SL* ps, SLDataType x)
{
assert(ps);
if (ps->size == ps->capacity)
{
int NewCapacity =ps->capacity == 0 ? 4 : 2 * ps->capacity;//判断刚开始是否有空间
SLDataType* tmp = (SLDataType*)realloc(ps->arr,sizeof(SLDataType) * NewCapacity);//开空间
if (tmp == NULL)
{
perror(“realloc”);
exit(-1);
}
ps->arr = tmp;
ps->capacity = NewCapacity;
}
ps->arr[ps->size] = x;
ps->size++;
}
>
> 尾插就是在尾部插入数据,因为刚开始的时候没有给数组分配空间,所以要考虑当没有空间时要去申请空间,`realloc`函数是扩容函数,在指针为空的情况下作用和`malloc`相同,`int NewCapacity =ps->capacity == 0 ? 4 : 2 * ps->capacity`判断当前容量大小并确定要开辟的空间大小。
> `SLDataType* tmp = (SLDataType*)realloc(ps->arr,sizeof(SLDataType) * NewCapacity)`,用来在堆内存中开辟空间,`realloc`之后注意将其强制转化成`SLDataType*`类型。当成功开辟空间之后赋给`ps->arr`,同时注意将`NewCapacity`赋给`ps->capacity`。
>
>
>
### 4.尾删
void SLPopBack(SL* ps)
{
assert(ps);
//暴力的检查
assert(ps->size > 0);//判断ps->size是否大于0,当等于0时再删的话ps->size就会变为-1,越界
//温柔的检查
//if (ps->size == 0)
//{
// return;
//}
ps->size–;
}
>
> 当`ps->size`等于0时直接返回,如果不返回,造成`ps->size`为负数,造成数组越界,当我们再次插入数据的时候可能会出现报错,同时当越界时可能不会报错,但是可能会在`free`时候报错。所以要用`assert(ps->size > 0)`检查`ps->size`大小,等于0时直接退出,并提示。
> 扩展:`free`时候报错可能的错误原因有两种,一是`free`时候指针不正确,可能是野指针,同时释放的时候必须从起始位置释放。二是越界时候`free`会报错。当越界读数组的时候基本不会被检查出来报错,但是改变它的值的时候就可能会报错,是一种抽查行为,是在运行时检查。
>
>
>
### 5.扩容
void SLCheckCapacity(SL* ps)
{
assert(ps);
//检查是否需要扩容
if (ps->size == ps->capacity)
{
int NewCapacity = ps->capacity == 0 ? 4 : 2 * ps->capacity;
SLDataType* tmp = (SLDataType*)realloc(ps->arr, sizeof(SLDataType) * NewCapacity);
if (tmp == NULL)
{
perror(“realloc”);
exit(-1);
}
ps->capacity = NewCapacity;
ps->arr = tmp;
}
}
>
> 扩容函数,用于检查数组空间大小是否适合继续插入,当数组空间不足时进行扩容,定义此函数之后可以对上面尾插函数进行简化,如下:
>
>
>
void SLPushBack(SL* ps, SLDataType x)
{
SLCheckCapacity(ps);
ps->arr[ps->size] = x;
ps->size++;
}
### 6.头插
void SLPushFront(SL* ps, SLDataType x)
{
SLCheckCapacity(ps);
int end = ps->size;
//挪动数据
while (end > 0)
{
ps->arr[end] = ps->arr[end-1];
end–;
}
ps->arr[0] = x;
ps->size++;
}
>
> 利用扩容函数对其检查,注意控制循环结束条件。
>
>
>
### 7.头删
void SLPopFront(SL* ps)
{
assert(ps);
assert(ps->size > 0);
int begin = 0;
while (begin < ps->size-1)
{
ps->arr[begin] = ps->arr[begin + 1];
begin++;
}
ps->size–;
}
>
> 注意判断当`ps->size`等于0时不能再进行`ps->size--`,要加上一条判断语句`assert(ps->size > 0)`。
>
>
>
### 8.任意位置插入
void SLInsert(SL* ps, int pos, SLDataType x)//任意位置插入
{
assert(ps);
assert(pos >= 0);
assert(pos <= ps->size);
SLCheckCapacity(ps);
int end = ps->size;
while (end > pos)
{
ps->arr[end] = ps->arr[end - 1];
end–;
}
ps->arr[pos] = x;
ps->size++;
}
>
> `pos`是数组下标,同时注意判断`pos`的范围。
>
>
>
### 9.任意位置删除
void SLErase(SL* ps, int pos)//任意位置删除
{
assert(ps);
assert(pos >= 0);
assert(pos < ps->size);
while (pos < ps->size-1)
{
ps->arr[pos] = ps->arr[pos + 1];
pos++;
}
ps->size–;
}
>
> `pos`表示的是数组下标,有`assert(pos >= 0)`和`assert(pos <= ps->size)`两个判断条件之后不用再加`assert(ps->size>0)`,因为当`ps->size`等于0时,`assert(pos <= ps->size)`会报错。
>
>
>
### 10.查找某个数的位置
int SLFind(SL* ps, SLDataType x)//返回类型为int,是数组下标
{
assert(ps);
int i = 0;
for (i = 0; i < ps->size; i++)
{
if (ps->arr[i] == x)
return i;
}
return -1;
}
>
> 返回值是数组下标。
>
>
>
>
> 思考:当我们想删除某个值时应该怎么做?当被删除的值有多个时又要怎么做呢?
> 我们可以通过下面的代码实现:
>
>
>
int SLNFind(SL* ps, SLDataType x, int begin)//begin是开始查找的位置
{
assert(ps);
int i = 0;
for (i = begin; i < ps->size; i++)
{
if (ps->arr[i] == x)
return i;
}
return -1;
}
## 三、顺序表演示及代码(含源码)
### 1.演示效果
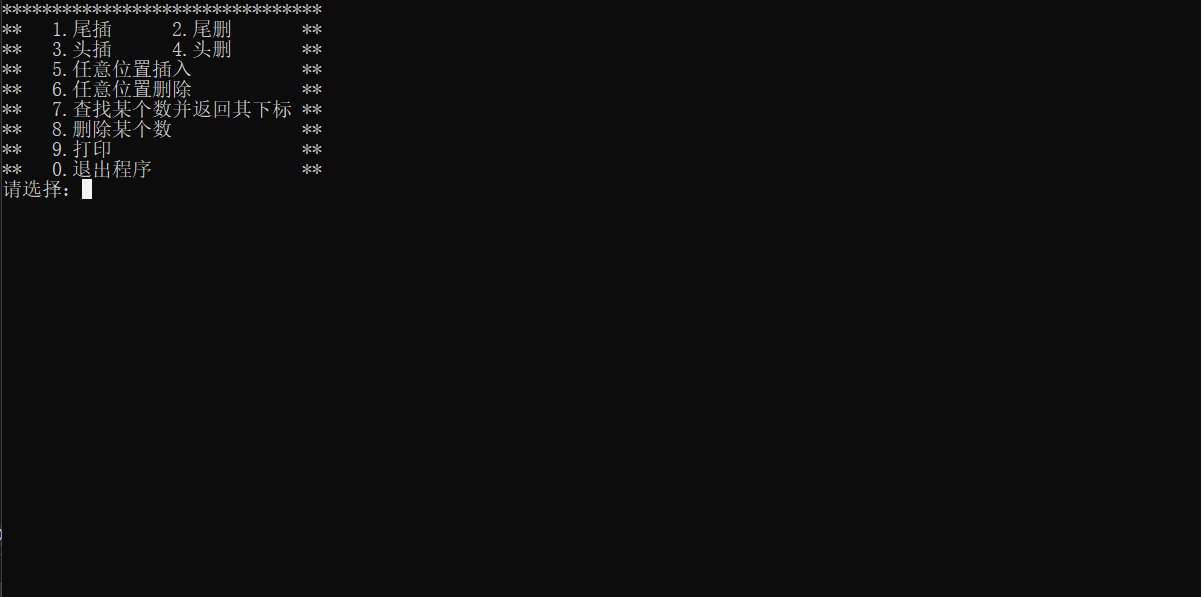
### 2.完整源代码
#include <stdio.h>
#include <assert.h>
#include <stdlib.h>
//动态顺序表
typedef int SLDataType;//当存储数据类型改变时,方便修改
typedef struct SeqList
{
SLDataType* arr;//指向动态开辟的数组
int size;//用来记录数组中存储有效数据元素的个数
int capacity;//用来记录容量大小
}SL;
void SLInit(SL* ps)//初始化顺序表
{
assert(ps);
ps->arr = NULL;
ps->size = ps->capacity = 0;
}
void SLCheckCapacity(SL* ps)//检查是否需要扩容
{
assert(ps);
//检查是否需要扩容
if (ps->size == ps->capacity)
{
int NewCapacity = ps->capacity == 0 ? 4 : 2 * ps->capacity;
SLDataType* tmp = (SLDataType*)realloc(ps->arr, sizeof(SLDataType) * NewCapacity);
if (tmp == NULL)
{
perror(“realloc”);
exit(-1);
}
ps->capacity = NewCapacity;
ps->arr = tmp;
}
}
void SLPushBack(SL* ps, SLDataType x)//尾插
{
SLCheckCapacity(ps);//检查是否需要扩容
//assert(ps);
//if (ps->size == ps->capacity)
//{
// int NewCapacity =ps->capacity == 0 ? 4 : 2 * ps->capacity;//判断刚开始是否有空间
// SLDataType* tmp = (SLDataType*)realloc(ps->arr,sizeof(SLDataType) * NewCapacity);
// if (tmp == NULL)
// {
// perror(“realloc”);
// exit(-1);
// }
// ps->arr = tmp;
// ps->capacity = NewCapacity;
//}
ps->arr[ps->size] = x;
ps->size++;
}
void SLPrint(SL* ps)//打印
{
int i = 0;
for (i = 0; i < ps->size; i++)
{
printf(“%d “, ps->arr[i]);
}
printf(”\n”);
}
void SLDestroy(SL* ps)//销毁
{
assert(ps);
if (ps->arr != NULL)
{
free(ps->arr);
ps->arr = NULL;
}
ps->size = ps->capacity = 0;
}
void SLPopBack(SL* ps)//尾删
{
assert(ps);
//暴力的检查
assert(ps->size > 0);//判断ps->size是否大于0,当等于0时再删的话ps->size就会变为-1,越界
//温柔的检查
if (ps->size == 0)
{
return;
}
ps->size–;
}
void SLPushFront(SL* ps, SLDataType x)//头插
{
SLCheckCapacity(ps);
int end = ps->size;
//挪动数据
while (end > 0)
{
ps->arr[end] = ps->arr[end - 1];
end–;
}
ps->arr[0] = x;
ps->size++;
}
void SLPopFront(SL* ps)//头删
{
assert(ps);
assert(ps->size > 0);
int begin = 0;
while (begin < ps->size - 1)
{
ps->arr[begin] = ps->arr[begin + 1];
begin++;
}
ps->size–;
}
void SLInsert(SL* ps, int pos, SLDataType x)//任意位置插入
{
assert(ps);
assert(pos >= 0);
assert(pos <= ps->size);
SLCheckCapacity(ps);
int end = ps->size;
while (end > pos)
{
ps->arr[end] = ps->arr[end - 1];
end–;
}
ps->arr[pos] = x;
ps->size++;
}
void SLErase(SL* ps, int pos)//任意位置删除
{
assert(ps);
assert(pos >= 0);
assert(pos < ps->size);
while (pos < ps->size - 1)
{
ps->arr[pos] = ps->arr[pos + 1];
pos++;
}
ps->size–;
}
int SLFind(SL* ps, SLDataType x)//查找某个数并返回下标
{
assert(ps);
int i = 0;
for (i = 0; i < ps->size; i++)
{
if (ps->arr[i] == x)
网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
SLDataType x)//查找某个数并返回下标
{
assert(ps);
int i = 0;
for (i = 0; i < ps->size; i++)
{
if (ps->arr[i] == x)
[外链图片转存中…(img-wzTCxIA8-1714274011722)]
[外链图片转存中…(img-iYg5tb16-1714274011723)]
网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!