start-dfs.sh
- 再启动hbase
start-hbase.sh
- jps出现下面这些即可
- 停止 HBase 运行
stop-hbase.sh
- 停止Hadoop的运行
stop-dfs.sh
2.2 伪分布模式配置
2.2.1 配置hbase-site.xml
3. HBase常用的Shell命令
3.1 在HBase中创建表
create 'student','Sname','Ssex','Sage','Sdept','course'
3.2 添加数据
put 'student','95001','Sname','LiYing'
put 'student','95001','Ssex','male'
3.3 查看数据
get 'student','95001'
3.4 删除数据
在 Hbase 中用 delete 以及 deleteall 命令进行删除数据操作
区别:
delete
用于删除一个单元格数据,是 put 的反向操作;
deleteall
用于删除一行数据
delete 'student','95001','Ssex'
3.5 删除表
删除表需要分两步操作
第一步先让该表不可用,第二步删除表。
比如,要删除student表,可以使用如下命令:
disable 'student'
drop 'student'
3.6 查询历史记录
- 先创建一个
teacher
表
create 'teacher',{NAME=>'username',VERSIONS=>5}
- 不断put数据
查询时,默认情况下回显示当前最新版本的数据,如果要查询历史数据,需要指定查询的历史版本数,由于上面设置了保存版本数为5,所以,在查询时制定的历史版本数的有效取值为1到5,具体命令如下:
get 'teacher','91001', {COLUMN=>'username',VERSIONS=>3}
下面是查询版本号为3的
3.7 退出HBase数据库
exit
4. HBase编程实践
- 在IDEA创建项目
- 为项目添加需要用到的JAR包
JAR包位于HBase安装目录下
如位于:/usr/local/hbase/lib目录下,单击界面中的Libraries选项卡,再单击界面右侧的Add External JARs按钮,选中/usr/local/hbase/lib目录下的所有JAR包,点击OK,继续添加JAR包,选中client-facing-thirdparty下的所有JAR文件,点击OK。
- 编写Java应用程序
如果程序里面示例网址“hdfs://localhost:9000/hbase”,运行时出错,可以把” localhost ”改成” master ”。 - 编译运行
4.1 编程题 API文档
4.1.1 第一题
利用4中的程序,创建上表:表scores的概念视图如上图所示
- 用学生的名字name作为行键(rowKey)
- 年级grade是一个只有一个列的列族
- score是一个列族。
- 每一门课程都是score的一个列,如English、math、Chinese等。score 的列可以随时添加。
添加如下数据:
Name | Grade | English | Math | Chinese |
---|---|---|---|---|
Leelei | 6 | 78 | 88 | 90 |
Dandan | 6 | 95 | 100 | 92 |
Sansan | 6 | 67 | 99 | 60 |
Zhanshan | 6 | 66 | 66 | 66 |
- 创建表
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.\*;
import org.apache.hadoop.hbase.client.Admin;
import org.apache.hadoop.hbase.client.Connection;
import org.apache.hadoop.hbase.client.ConnectionFactory;
import org.apache.hadoop.hbase.HBaseConfiguration;
import java.io.IOException;
public class Create {
public static Configuration configuration;
public static Connection connection;
public static Admin admin;
//建立连接
public static void init(){
configuration = HBaseConfiguration.create();
configuration.set("hbase.rootdir","hdfs://localhost:9000/hbase");
try{
connection = ConnectionFactory.createConnection(configuration);
admin = connection.getAdmin();
}catch (IOException e){
e.printStackTrace();
}
}
//关闭连接
public static void close(){
try{
if(admin != null){
admin.close();
}
if(null != connection){
connection.close();
}
}catch (IOException e){
e.printStackTrace();
}
}
public static void CreateTable(String tableName) throws IOException {
if (admin.tableExists(TableName.valueOf(tableName))) {
System.out.println("Table Exists!!!");
}
else{
HTableDescriptor tableDesc = new HTableDescriptor(tableName);
tableDesc.addFamily(new HColumnDescriptor("grade"));
tableDesc.addFamily(new HColumnDescriptor("score"));
tableDesc.addFamily(new HColumnDescriptor("score.english"));
tableDesc.addFamily(new HColumnDescriptor("score.math"));
tableDesc.addFamily(new HColumnDescriptor("score.chinese"));
admin.createTable(tableDesc);
System.out.println("Create Table Successfully .");
}
}
public static void main(String[] args) {
String tableName = "scores\_zqc";
try {
init();
CreateTable(tableName);
close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
- 插入数据
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.\*;
import java.io.IOException;
public class Insert {
public static Configuration configuration;
public static Connection connection;
public static Admin admin;
//建立连接
public static void init(){
configuration = HBaseConfiguration.create();
configuration.set("hbase.rootdir","hdfs://localhost:9000/hbase");
try{
connection = ConnectionFactory.createConnection(configuration);
admin = connection.getAdmin();
}catch (IOException e){
e.printStackTrace();
}
}
//关闭连接
public static void close(){
try{
if(admin != null){
admin.close();
}
if(null != connection){
connection.close();
}
}catch (IOException e){
e.printStackTrace();
}
}
public static void InsertRow(String tableName, String rowKey, String colFamily, String col, String val) throws IOException {
Table table = connection.getTable(TableName.valueOf(tableName));
Put put = new Put(rowKey.getBytes());
put.addColumn(colFamily.getBytes(), col.getBytes(), val.getBytes());
System.out.println("Insert Data Successfully");
table.put(put);
table.close();
}
public static void main(String[] args) {
String tableName = "scores\_zqc";
String[] RowKeys = {
"dandan",
"sansan",
};
String[] Grades = {
"6",
"6",
};
String[] English = {
"95",
"87"
};
String[] Math = {
"100",
"95",
};
String[] Chinese = {
"92",
"98",
};
try {
init();
int i = 0;
while (i < RowKeys.length){
InsertRow(tableName, RowKeys[i], "grade", "", Grades[i]);
InsertRow(tableName, RowKeys[i], "score", "english", English[i]);
InsertRow(tableName, RowKeys[i], "score", "math", Math[i]);
InsertRow(tableName, RowKeys[i], "score", "chinese", Chinese[i]);
i++;
} //031904102 zqc
close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
- hbase shell中查看数据
4.1.2 第二题
创建并插入相关数据后,查看Hbase java api 文档
在ExampleForHBase 类中添加两个个函数分别实现一个rowKey 过滤器(RowFilter)以及一个单列值过滤器(SingleColumValueFilter);
之后通过这两个函数分别做如下查询:
- 插入成功
- 查询Zhangshan 的年级以及相关成绩,打印在控制台中并截图。
- 查询数学成绩低于100的所有人的名字,打印在控制台中并截图。
- 插入数据
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.\*;
import java.io.IOException;
public class InsertTwo {
public static Configuration configuration;
public static Connection connection;
public static Admin admin;
//建立连接
public static void init(){
configuration = HBaseConfiguration.create();
configuration.set("hbase.rootdir","hdfs://localhost:9000/hbase");
try{
connection = ConnectionFactory.createConnection(configuration);
admin = connection.getAdmin();
}catch (IOException e){
e.printStackTrace();
}
}
//关闭连接
public static void close(){
try{
if(admin != null){
admin.close();
}
if(null != connection){
connection.close();
}
}catch (IOException e){
e.printStackTrace();
}
}
public static void InsertRow(String tableName, String rowKey, String colFamily, String col, String val) throws IOException {
Table table = connection.getTable(TableName.valueOf(tableName));
Put put = new Put(rowKey.getBytes());
put.addColumn(colFamily.getBytes(), col.getBytes(), val.getBytes());
System.out.println("Insert Data Successfully");
table.put(put);
table.close();
}
public static void main(String[] args) {
String tableName = "scores\_zqc\_two";
String[] RowKeys = {
"Leelei",
"Dandan",
"Sansan",
"Zhanshan",
};
String[] Grades = {
"6",
"6",
"6",
"6",
};
String[] English = {
"78",
"95",
"67",
"66",
};
String[] Math = {
"78",
"95",
"100",
"66",
};
String[] Chinese = {
"90",
"92",
"60",
"66",
};
try {
init();
int i = 0;
while (i < RowKeys.length){
InsertRow(tableName, RowKeys[i], "grade", "", Grades[i]);
InsertRow(tableName, RowKeys[i], "score", "english", English[i]);
InsertRow(tableName, RowKeys[i], "score", "math", Math[i]);
InsertRow(tableName, RowKeys[i], "score", "chinese", Chinese[i]);
i++;
}
close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
- 查询
import java.io.IOException;
import java.util.List;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.Cell;
import org.apache.hadoop.hbase.CellUtil;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.Admin;
import org.apache.hadoop.hbase.client.Connection;
import org.apache.hadoop.hbase.client.ConnectionFactory;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.client.ResultScanner;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.hadoop.hbase.client.Table;
import org.apache.hadoop.hbase.filter.CompareFilter.CompareOp;
import org.apache.hadoop.hbase.filter.Filter;
import org.apache.hadoop.hbase.filter.RowFilter;
import org.apache.hadoop.hbase.filter.SingleColumnValueFilter;
import org.apache.hadoop.hbase.filter.SubstringComparator;
import org.apache.hadoop.hbase.util.Bytes;
public class FindTwo {
public static Configuration configuration;
public static Connection connection;
public static Admin admin;
public static void init() {
configuration = HBaseConfiguration.create();
configuration.set("hbase.rootdir", "hdfs://192.168.0.108:9000/hbase");
try {
connection = ConnectionFactory.createConnection(configuration);
admin = connection.getAdmin();
} catch (IOException e) {
e.printStackTrace();
}
}
还有兄弟不知道网络安全面试可以提前刷题吗?费时一周整理的160+网络安全面试题,金九银十,做网络安全面试里的显眼包!
王岚嵚工程师面试题(附答案),只能帮兄弟们到这儿了!如果你能答对70%,找一个安全工作,问题不大。
对于有1-3年工作经验,想要跳槽的朋友来说,也是很好的温习资料!
【完整版领取方式在文末!!】
***93道网络安全面试题***
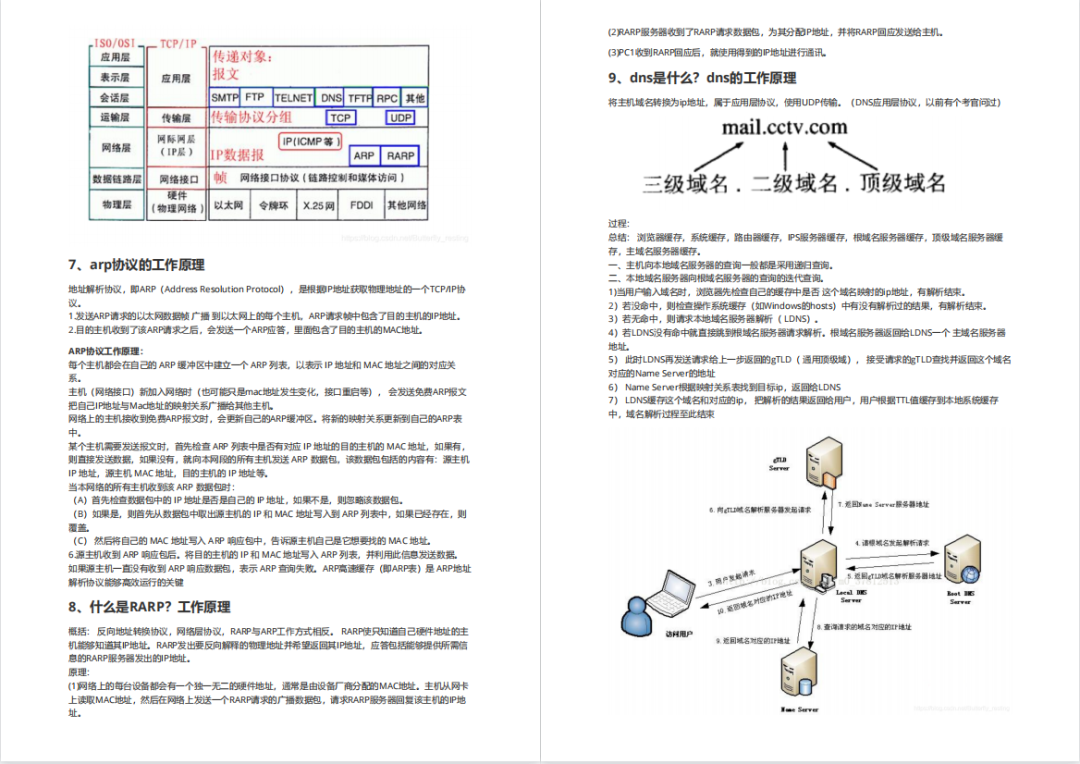
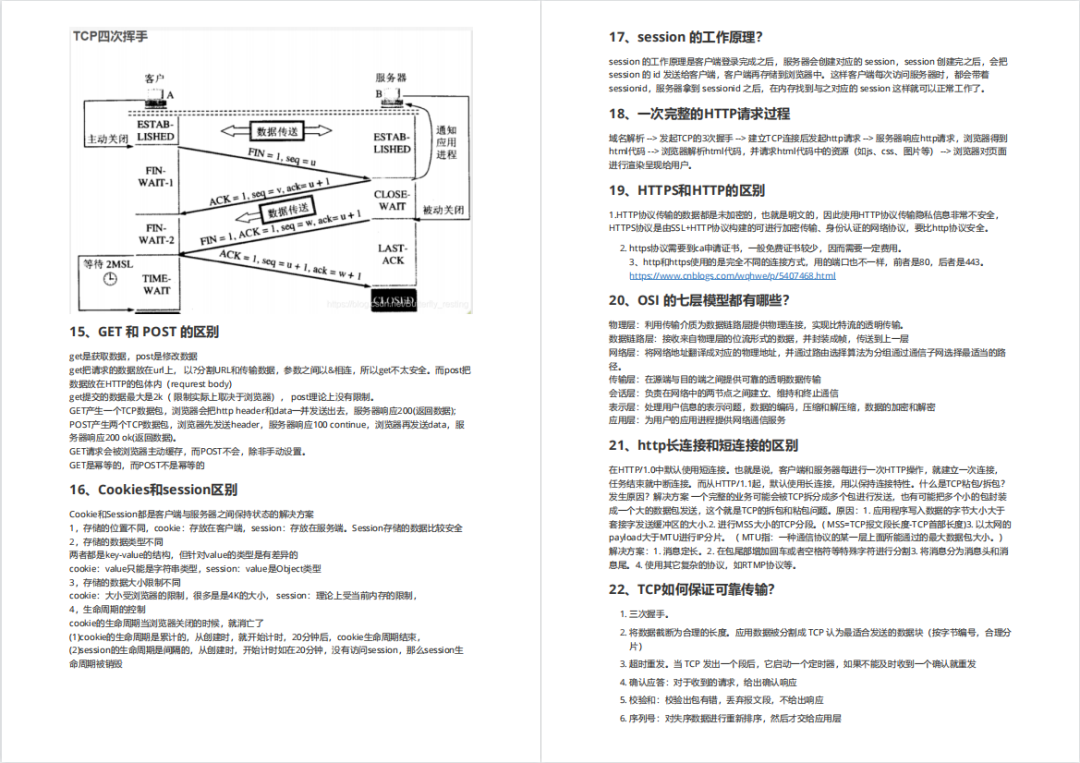
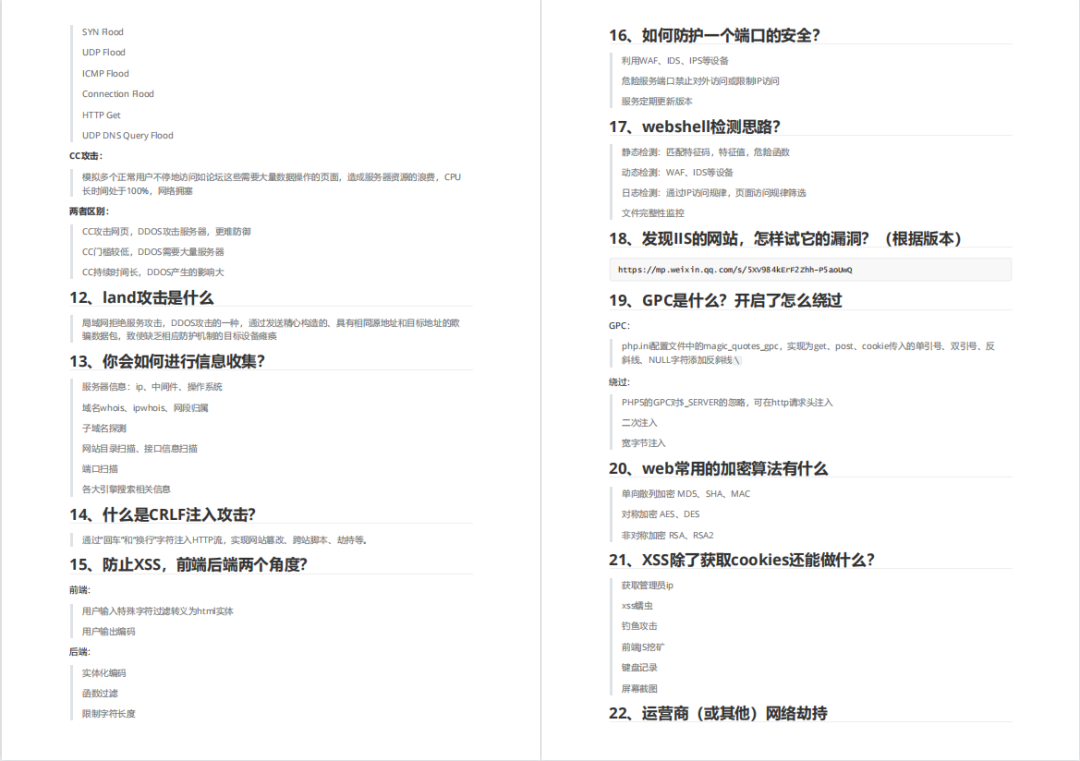
内容实在太多,不一一截图了
### 黑客学习资源推荐
最后给大家分享一份全套的网络安全学习资料,给那些想学习 网络安全的小伙伴们一点帮助!
对于从来没有接触过网络安全的同学,我们帮你准备了详细的学习成长路线图。可以说是最科学最系统的学习路线,大家跟着这个大的方向学习准没问题。
😝朋友们如果有需要的话,可以联系领取~
#### 1️⃣零基础入门
##### ① 学习路线
对于从来没有接触过网络安全的同学,我们帮你准备了详细的**学习成长路线图**。可以说是**最科学最系统的学习路线**,大家跟着这个大的方向学习准没问题。
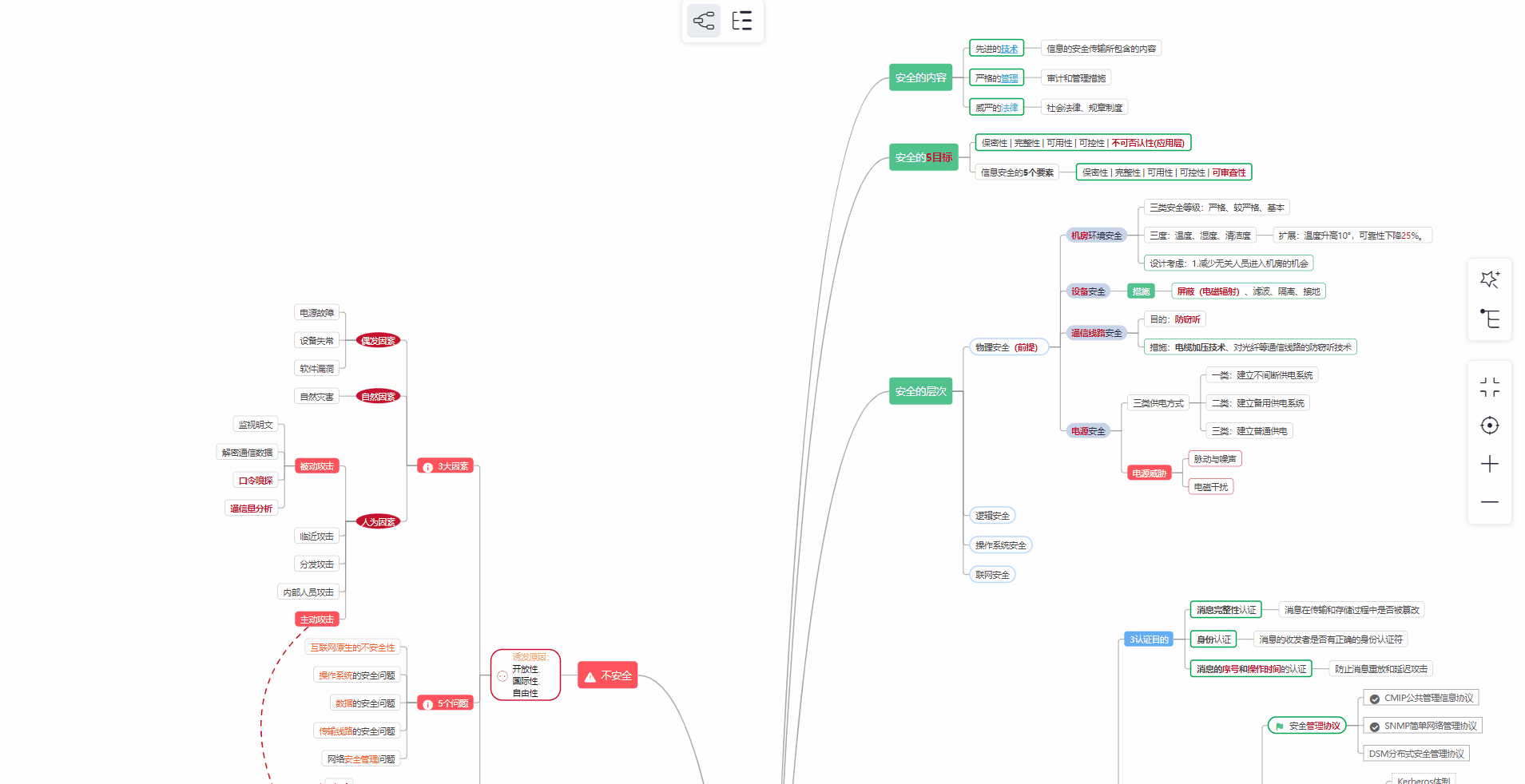
##### ② 路线对应学习视频
同时每个成长路线对应的板块都有配套的视频提供:
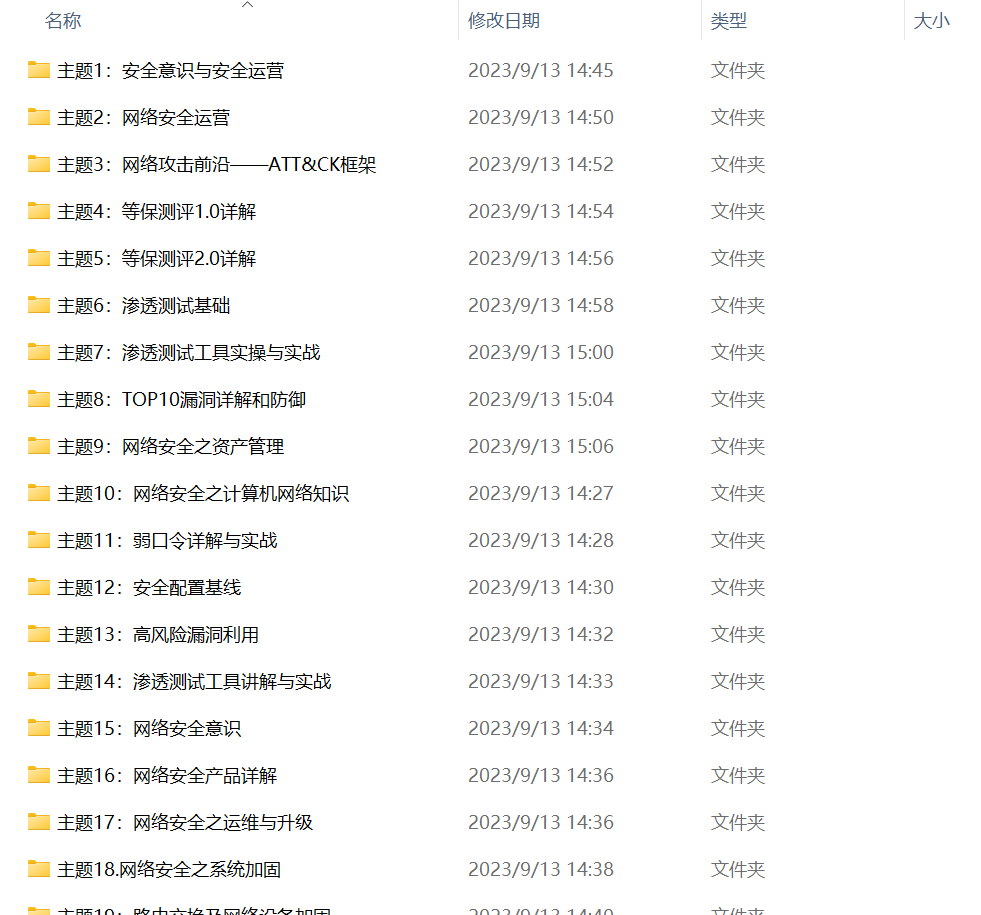
#### 2️⃣视频配套工具&国内外网安书籍、文档
##### ① 工具
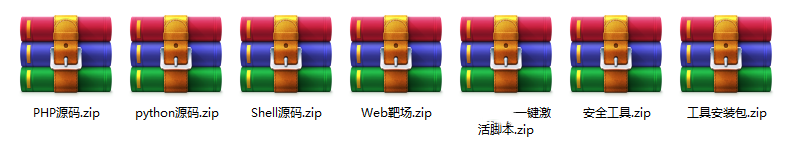
##### ② 视频
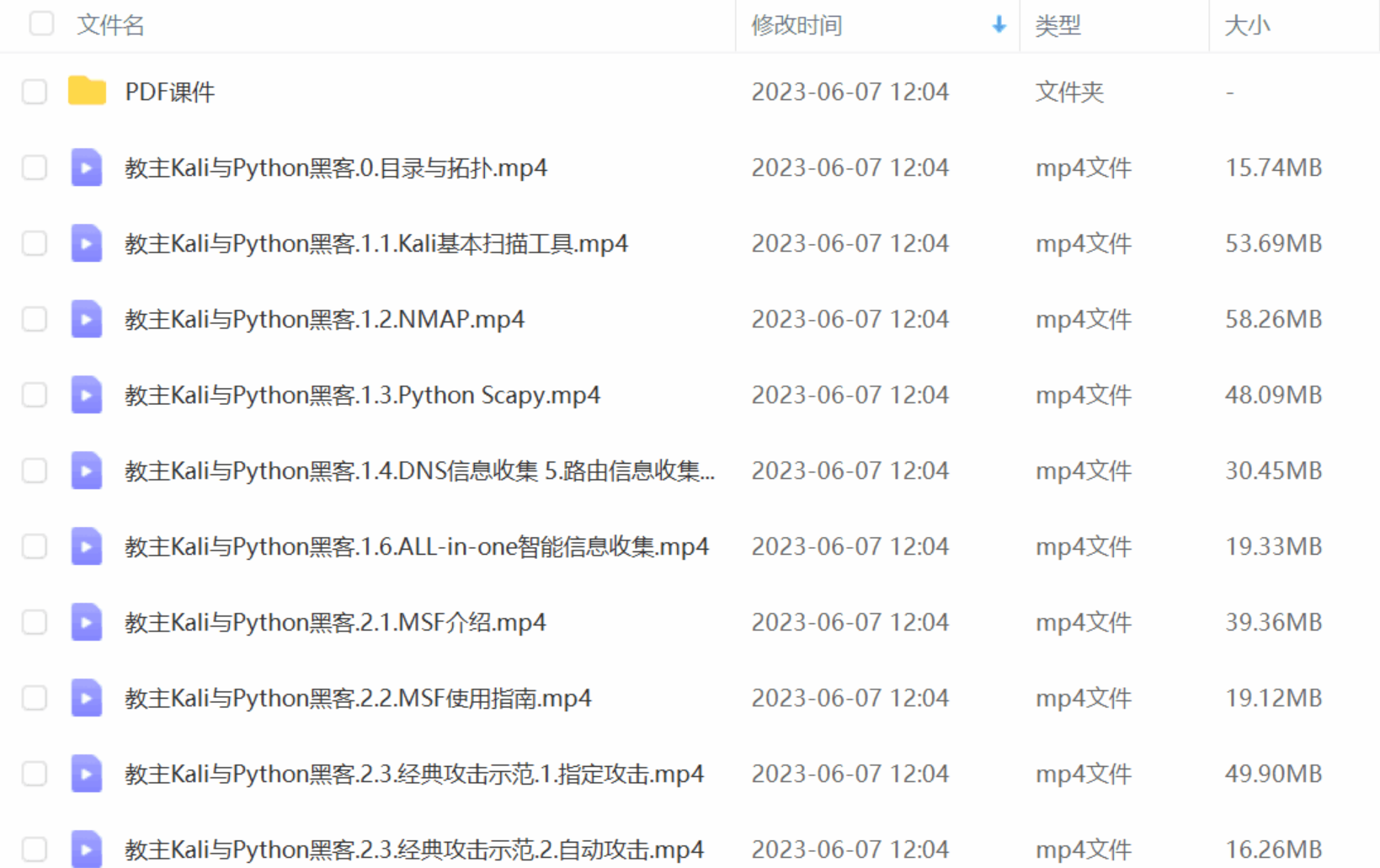
##### ③ 书籍
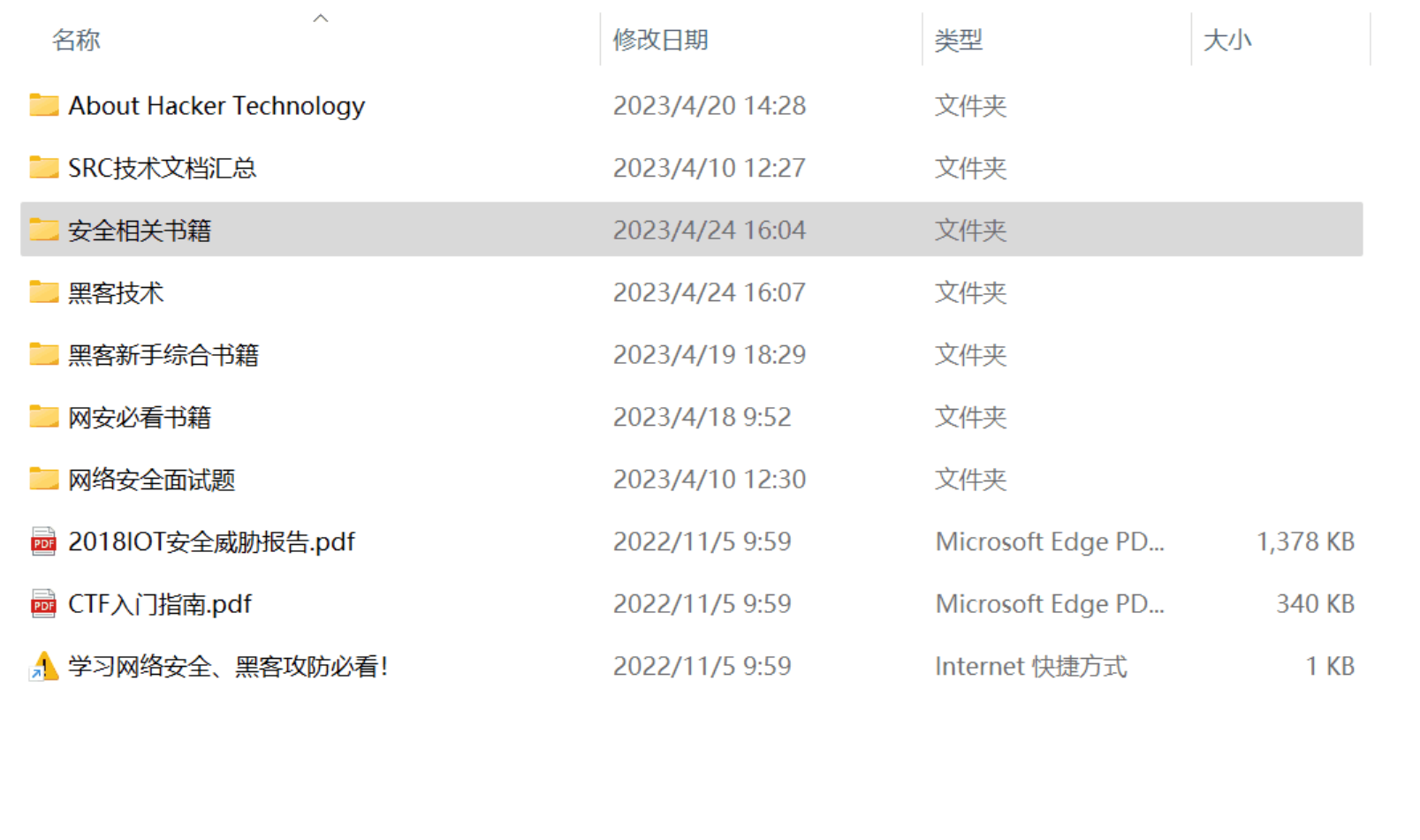
资源较为敏感,未展示全面,需要的最下面获取
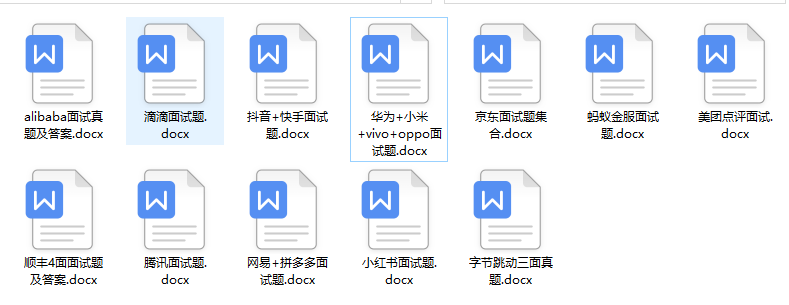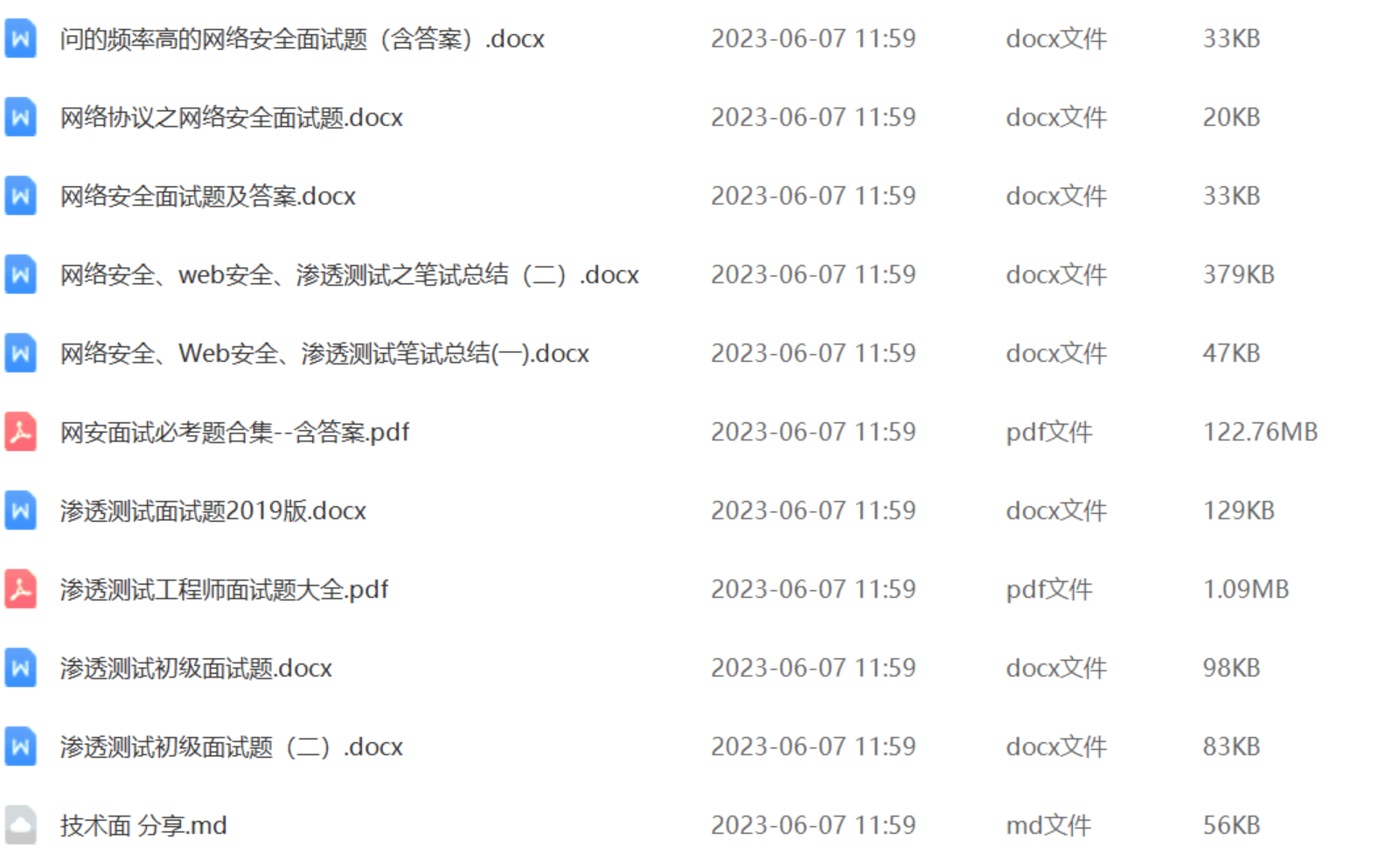
##### ② 简历模板
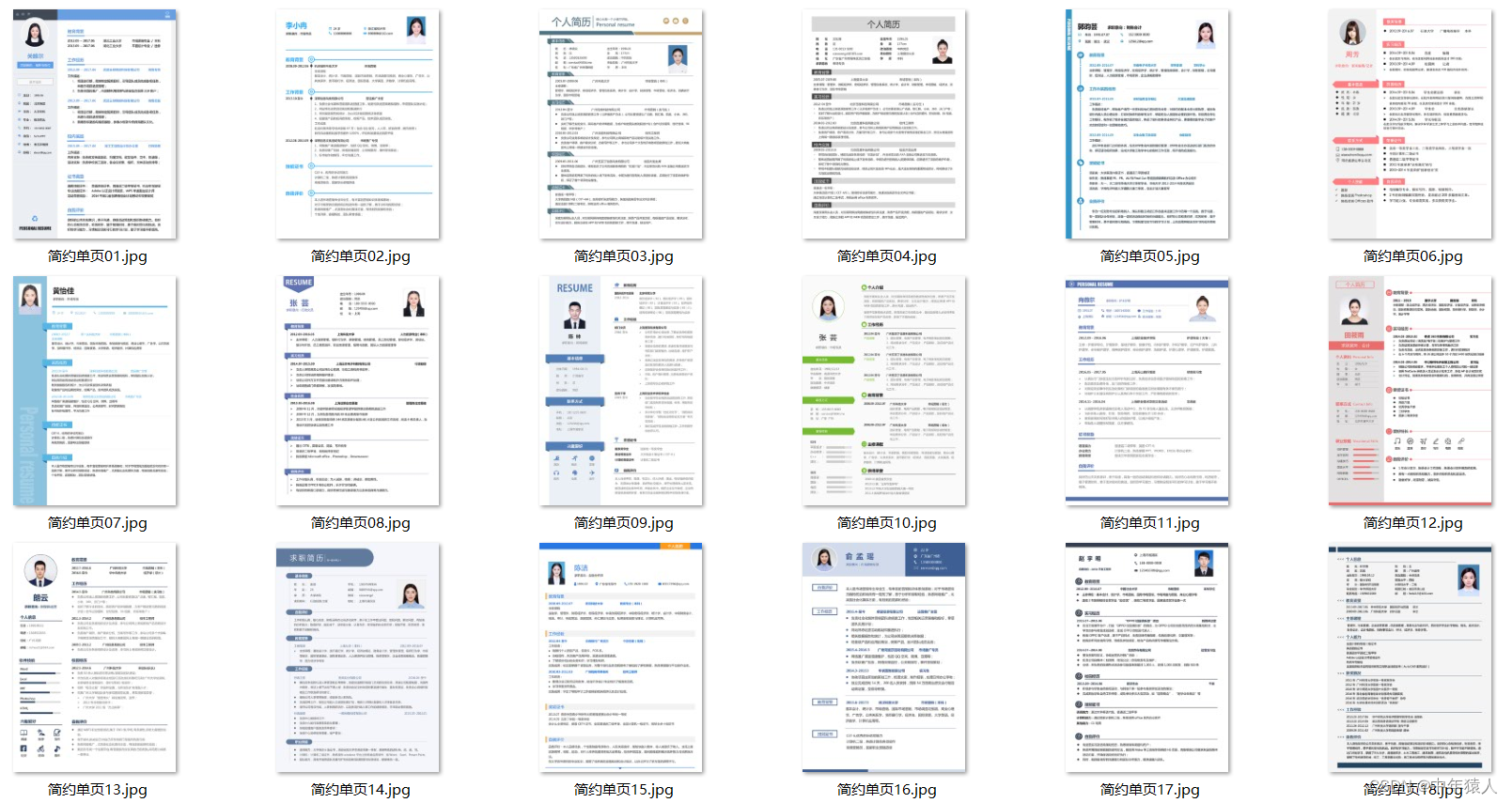
**因篇幅有限,资料较为敏感仅展示部分资料,添加上方即可获取👆**
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化资料的朋友,可以点击这里获取](https://bbs.csdn.net/topics/618540462)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**