org.springframework.boot
spring-boot-starter-web
org.springframework.boot
spring-boot-starter-actuator
org.springframework.boot
spring-boot-devtools
provided
true
org.projectlombok
lombok
true
org.springframework.boot
spring-boot-starter-test
test
(2):服务消费者在application项目配置文件中开启FeignHystrix功能
server:
port: 8009
spring:
application:
name: cloud-consumer-hystrix-order80
eureka:
client:
register-with-eureka: true # 是否注册到eureka中
fetch-registry: true # 是否获取注册到eureka的服务信息
service-url:
defaultZone: http://eureka7001.com:7001/eureka/ # eureka注册的地址
instance:
prefer-ip-address: true # 鼠标移动上去显示ip地址
instance-id: payment8003 # 服务的名称
#feign:
hystrix:
enabled: true # 开启feign的hystrix功能
(3):创建绑定服务消费者的实现类(服务消费方代码)
package com.springcloud.service;
import org.springframework.stereotype.Component;
/**
- 针对ConsumerService中绑定的服务的方法的服务降级类
*/
// 注入spring容器
@Component
public class ConsumerHystrixService implements ConsumerService {
@Override
public String ok() {
return “ok请求方法失败,进入服务熔断方法!”;
}
@Override
public String timeOut(Integer sleepTime) {
return “timeOut请求方法失败,进入服务熔断方法!”;
}
@Override
public String breakOut() {
return null;
}
}
// 绑定服务提供方的接口
package com.springcloud.service;
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
// 绑定服务名称为CLOUD-PROVIDER-HYSTRIX-PAYMENT8003的服务
@FeignClient(value = “CLOUD-PROVIDER-HYSTRIX-PAYMENT8003”,fallback = ConsumerHystrixService.class)
public interface ConsumerService {
@RequestMapping(“/payment/ok”)
public String ok();
@RequestMapping(“/payment/timeout/{sleepTime}”)
public String timeOut(@PathVariable(“sleepTime”) Integer sleepTime);
@RequestMapping(“/payment/breakout”)
public String breakOut();
}
// 服务消费者的启动类
package com.springcloud;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
import org.springframework.cloud.netflix.hystrix.EnableHystrix;
import org.springframework.cloud.openfeign.EnableFeignClients;
@SpringBootApplication
// 激活Hystrix
@EnableHystrix
// 激活Eureka客户端
@EnableEurekaClient
// 激活OpenFeign
@EnableFeignClients
public class HystrixConsumer80Application {
public static void main(String[] args) {
SpringApplication.run(HystrixConsumer80Application.class,args);
}
}
// 服务访问Controller
package com.springcloud.controller;
import com.springcloud.service.ConsumerService;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@Slf4j
@RestController
public class ConsumerController {
@Autowired
private ConsumerService consumerService;
@RequestMapping(“/consumer/payment/ok”)
public String ok(){
String ok = consumerService.ok();
//int i = 10/0;
log.info(Thread.currentThread().getName()+“,消费端获取到的信息:”+ok);
return ok;
}
@RequestMapping(“/consumer/payment/timeout/{sleepTime}”)
public String timeOut(@PathVariable(“sleepTime”) Integer sleepTime){
String s = consumerService.timeOut(sleepTime);
log.info(Thread.currentThread().getName()+“,消费端获取到的信息:”+s);
return s;
}
@RequestMapping(“/consumer/payment/breakout”)
public String breakOut(){
try{
String s = consumerService.breakOut();
return s;
}catch (Exception e){
log.info(e.getMessage());
}
return “breakOut请求失败”;
}
}
(4):服务提供方代码
// 配置文件
server:
port: 8003
spring:
application:
name: cloud-provider-hystrix-payment8003
eureka:
client:
fetch-registry: true # 从eureka中获取注册的信息
register-with-eureka: true # 注册到eureka中
service-url:
defaultZone: http://eureka7001.com:7001/eureka/ # eureka注册的地址
#defaultZone: http://eureka7001.com:7001/eureka/,http://eureka7002.com:7002/eureka/ # eureka注册的地址
instance:
prefer-ip-address: true # 鼠标移动上去显示ip地址
instance-id: payment8003 # 服务的名称
// pom文件
<?xml version="1.0" encoding="UTF-8"?><project xmlns=“http://maven.apache.org/POM/4.0.0”
xmlns:xsi=“http://www.w3.org/2001/XMLSchema-instance”
xsi:schemaLocation=“http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd”>
spring_parent
com.elvis.springcloud
1.0-SNAPSHOT
4.0.0
cloud-provider-hystrix-payment8003
org.springframework.cloud
spring-cloud-starter-netflix-hystrix
com.netflix.hystrix
hystrix-javanica
org.springframework.cloud
spring-cloud-starter-netflix-eureka-client
org.springframework.boot
spring-boot-starter-web
org.springframework.boot
spring-boot-starter-actuator
org.springframework.boot
spring-boot-devtools
provided
true
org.projectlombok
lombok
true
org.springframework.boot
spring-boot-starter-test
test
// 服务提供者的controller层
package com.springcloud.controller;
import com.netflix.hystrix.contrib.javanica.annotation.DefaultProperties;
import com.netflix.hystrix.contrib.javanica.annotation.HystrixCommand;
import com.netflix.hystrix.contrib.javanica.annotation.HystrixProperty;
import com.springcloud.service.PaymentService;
import com.springcloud.service.impl.PaymentServiceImpl;
import javafx.beans.DefaultProperty;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@Slf4j
// 设置全局默认的服务降级响应
@DefaultProperties(defaultFallback = “globalHystrixFallback”)
public class PaymentController {
@Autowired
private PaymentService paymentService;
// 直接反馈信息
@RequestMapping(“/payment/ok”)
// 不指定fallback属性时,默认使用DefaultProperties中指定的降级响应方法
@HystrixCommand
public String ok(){
int age = 10/0;
String ok = paymentService.ok();
return ok;
}
// 请求后睡眠指定时间再反馈
@RequestMapping(“/payment/timeout/{sleepTime}”)
// 注意: fallbackMethod指定的方法的参数列表要跟当前方法的参数列表的参数一样,否则会报错
@HystrixCommand(fallbackMethod = “specialHystrixFallback”,commandProperties = {
// 设置最大的等待时间,超过这个时间获取反馈则认为是超时了
@HystrixProperty(name = “execution.isolation.thread.timeoutInMilliseconds”,value = “3000”)
})
public String timeOut(@PathVariable(“sleepTime”) Integer sleepTime){
String timeOut = paymentService.timeOut(sleepTime);
return timeOut;
}
// 全局通用的Hystrix备选反馈
public String globalHystrixFallback(){
String logs = “全局通用的服务降级备选处理反馈!”;
log.info(logs);
return logs;
}
// 特殊指定的Hystrix备选反馈
public String specialHystrixFallback(Integer abc){
String logs = “特殊指定的服务降级备选处理反馈!”;
log.info(logs);
return logs;
}
@RequestMapping(“/payment/breakout”)
public String breakOut(){
return “测试服务宕机”;
}
}
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
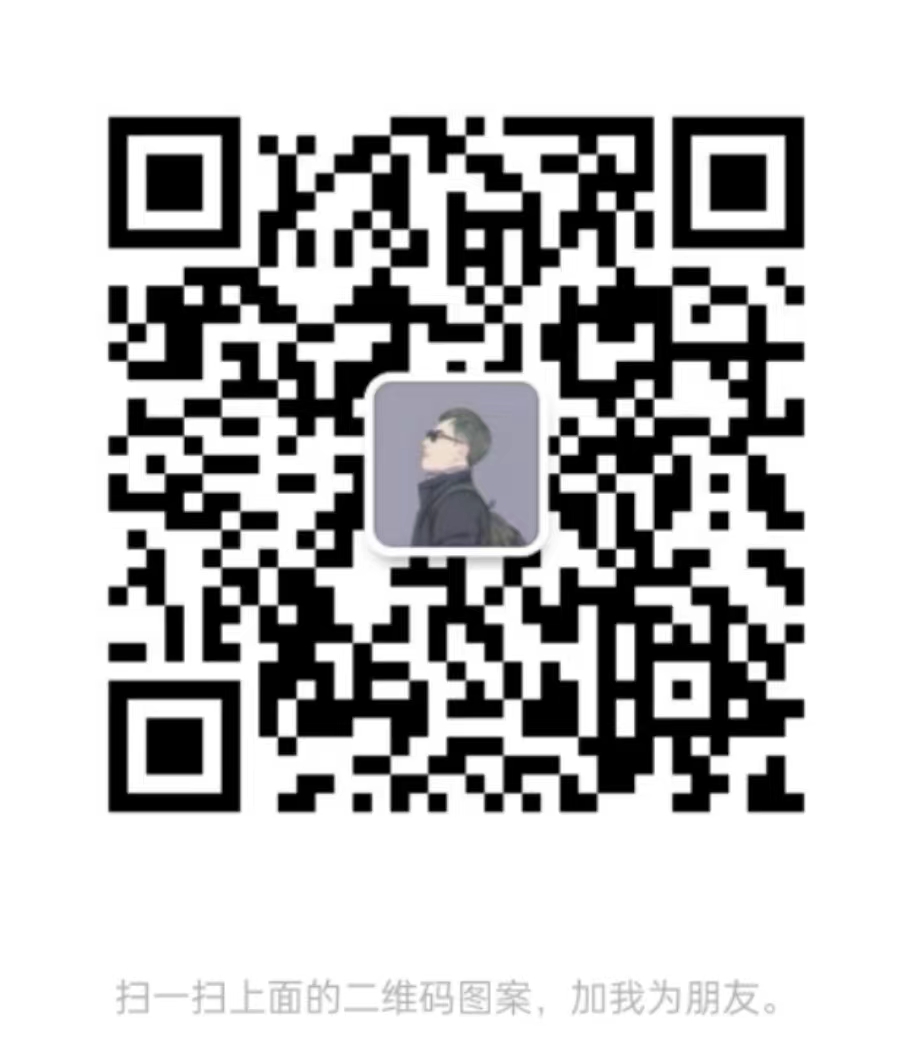
文末
我将这三次阿里面试的题目全部分专题整理出来,并附带上详细的答案解析,生成了一份PDF文档
- 第一个要分享给大家的就是算法和数据结构
- 第二个就是数据库的高频知识点与性能优化
- 第三个则是并发编程(72个知识点学习)
- 最后一个是各大JAVA架构专题的面试点+解析+我的一些学习的书籍资料
还有更多的Redis、MySQL、JVM、Kafka、微服务、Spring全家桶等学习笔记这里就不一一列举出来
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
、源码讲义、实战项目、讲解视频,并且会持续更新!**
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
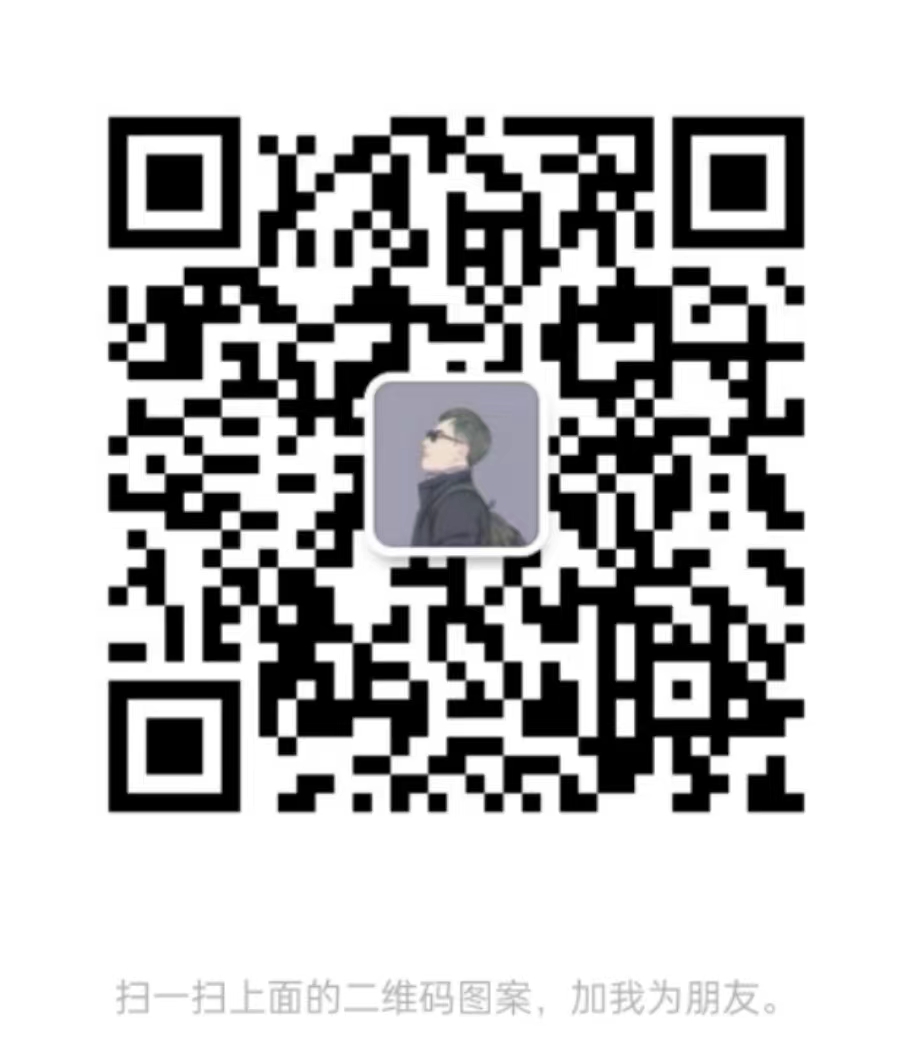
文末
我将这三次阿里面试的题目全部分专题整理出来,并附带上详细的答案解析,生成了一份PDF文档
- 第一个要分享给大家的就是算法和数据结构
[外链图片转存中…(img-sC2xYrOK-1713562407124)]
- 第二个就是数据库的高频知识点与性能优化
[外链图片转存中…(img-JpKgdlCB-1713562407125)]
- 第三个则是并发编程(72个知识点学习)
[外链图片转存中…(img-yk1XZISj-1713562407126)]
- 最后一个是各大JAVA架构专题的面试点+解析+我的一些学习的书籍资料
[外链图片转存中…(img-dzM5pU2P-1713562407127)]
还有更多的Redis、MySQL、JVM、Kafka、微服务、Spring全家桶等学习笔记这里就不一一列举出来
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!