<div>
<img src="mao.jpg" alt="">
</div>
div {
text-align: center;
}
div {
display: flex;
justify-content: center;
}
作为背景图片:
.box {
width: 300px;
height: 200px;
background-color: pink;
background: url(mao.jpg) no-repeat;
background-size: contain;
/\* background-size: cover; 铺满 \*/
}
四、实现一个自适应的正方形
div {
width: 20vw;
height: 20vw;
background-color: pink;
}
div {
width: 20%;
height: 0;
padding-bottom: 20%;
background-color: pink;
}
五、bfc,flex,CSS 选择器
参考:https://www.bilibili.com/video/BV1aZ4y1M7gW
BFC:box formatting context 块级格式化上下文
BFC就是页面上的一个隔离的独立容器,容器里面的子元素不会影响到外面的元素。反之也如此。
常见的定位方案:普通流、浮动、绝对定位
BFC属于普通流,可以看作是元素的属性 ,当这个元素拥有了BFC属性时,就可以看作是隔离的独立容器,容器里面的元素不会在布局上影响到外面的元素
为元素添加BFC:
作用:
1.避免外边距重叠:用两个盒子包含重叠的两个盒子,并设置overflow:hidden
2.清除浮动:给父盒子添加overflow:hidden
3.阻止元素被浮动元素覆盖:给没有浮动元素添加overflow:hidden
CSS选择器:
基础选择器:标签、类、id、通配符
复合选择器:后代、子、并集、伪类
六、回流和重绘
七、行内元素造成的间距怎么解决
八、清除浮动的方法、外边距重叠、外边距塌陷
清除浮动问题:
1.额外标签法:在浮动元素末尾加一个额外的空标签(块级元素),给他设置一个clear:both的样式
2.父级添加overflow属性:给父级盒子添加overflow:hidden
3.父级添加after伪元素
4.父级添加双伪元素
5.给父级定义高度
外边距重叠问题:
1.给下面盒子设置定位absolute/fixed
2.给下面盒子设置浮动
3.下面盒子设置为inline-block
4.给他们嵌套盒子,设置overflow:hidden
外边距塌陷问题:
1.给父盒子设置边框
2.给父盒子设置内边距
3.父盒子overflow:hidden
4.子元素变为inline-block或添加定位和浮动
九、CSS两栏布局
<div class="container">
<div class="left"></div>
<div class="right"></div>
</div>
1.flex:
/\* 1. flex \*/
.container {
display: flex;
height: 200px;
background-color: #ccc;
}
.left {
width: 100px;
background-color: pink;
}
.right {
flex: 1;
background-color: skyblue;
}
2.absolute + margin
/\* 2.absolute + margin \*/
.container {
position: relative;
background-color: #ccc;
}
.left {
position: absolute;
top: 0;
left: 0;
width: 100px;
height: 200px;
background-color: pink;
}
.right {
margin-left: 100px;
height: 200px;
background-color: skyblue;
}
3.float + margin
/\* 3.float + margin \*/
.container {
background-color: #ccc;
}
.left {
float: left;
width: 100px;
height: 200px;
background-color: pink;
}
.right {
margin-left: 100px;
height: 200px;
background-color: skyblue;
}
4.float + BFC
/\* 4.float + BFC \*/
.container {
background-color: #ccc;
}
.left {
float: left;
height: 200px;
width: 100px;
background-color: pink;
}
.right {
overflow: hidden;
height: 200px;
background-color: skyblue;
}
5.table
/\* 5.table \*/
.container {
display: table;
background-color: #ccc;
width: 100%;
}
.left {
display: table-cell;
height: 200px;
width: 100px;
background-color: pink;
}
.right {
display: table-cell;
height: 200px;
background-color: skyblue;
}
6.grid
/\* 6.grid \*/
.container {
display: grid;
grid-template-rows: 200px;
grid-template-columns: 100px auto;
}
.left {
background-color: pink;
}
.right {
background-color: skyblue;
}
十、CSS三栏布局
<div class="container">
<div class="left"></div>
<div class="main"></div>
<div class="right"></div>
</div>
1.flex
/\* 1.flex \*/
.container {
display: flex;
}
.left {
width: 100px;
height: 200px;
background-color: pink;
}
.main {
flex: 1;
height: 200px;
background-color: #ccc;
}
.right {
width: 100px;
height: 200px;
background-color: skyblue;
}
2.absolute + margin
/\* 2.absolute + margin \*/
.container {
position: relative;
}
.left {
position: absolute;
top: 0;
left: 0;
width: 100px;
height: 200px;
background-color: pink;
}
.main {
height: 200px;
margin-left: 100px;
margin-right: 100px;
background-color: #ccc;
}
.right {
position: absolute;
top: 0;
right: 0;
width: 100px;
height: 200px;
background-color: skyblue;
}
3.float + margin
/\* 3. \*/
.container {}
.left {
float: left;
width: 100px;
height: 200px;
background-color: pink;
}
.main {
height: 200px;
margin-left: 100px;
margin-right: 100px;
background-color: #ccc;
}
.right {
float: right;
width: 100px;
height: 200px;
background-color: skyblue;
}
4.table
/\* 4.table \*/
.container {
display: table;
width: 100%;
}
.left {
display: table-cell;
width: 100px;
height: 200px;
background-color: pink;
}
.main {
display: table-cell;
height: 200px;
background-color: #ccc;
}
.right {
display: table-cell;
width: 100px;
height: 200px;
background-color: skyblue;
}
5.grid
/\* 5.grid \*/
.container {
display: grid;
grid-template-rows: 200px;
grid-template-columns: 100px auto 100px;
}
.left {
background-color: pink;
}
.main {
background-color: #ccc;
}
.right {
background-color: skyblue;
}
十一、CSS污染怎么解决
1.尽量少用标签选择器
2.使用类选择器时更加具体一些,例如通过后代选择器提高权重(但可能会导致性能问题)
3.把样式写到行内
4.可以给class加一些前缀
。。。
十二、CSS垂直居中方法
1.line-height
2.absolute + top:50% 、margin-top:-子盒子高度一半
3.absolut + top:0、bottom:0、margin-top margin-bottom:auto
4.display:flex + align-items:center
5.display:table-cell + vertical-align:middle
十三、CSS水平居中方法
1.行内元素:text-align:center
2.块级元素:margin-left margin-right:auto
3.absolute + left:50% 、margin-left:-子盒子宽度一半
4.absolut + left:0、right:0、margin-left margin-right:auto
5.display:flex + justify-content:center
十四、css水平垂直居中
1.flex
.father {
width: 200px;
height: 200px;
background-color: pink;
display: flex;
justify-content: center;
align-items: center;
}
.son {
width: 100px;
height: 100px;
background-color: purple;
}
2.flex+auto
.father {
width: 200px;
height: 200px;
background-color: pink;
display: flex;
}
.son {
width: 100px;
height: 100px;
background-color: purple;
margin: auto;
}
3.absolute
.father {
position: relative;
width: 200px;
height: 200px;
background-color: pink;
}
.son {
position: absolute;
top: 50%;
left: 50%;
margin-left: -50px;
margin-top: -50px;
或者是:transform: translate(-50%, -50%);
width: 100px;
height: 100px;
background-color: purple;
}
4.absolute+auto
.father {
position: relative;
width: 200px;
height: 200px;
background-color: pink;
}
.son {
position: absolute;
top: 0;
left: 0;
bottom: 0;
right: 0;
margin: auto;
width: 100px;
height: 100px;
background-color: purple;
}
十五、响应式布局实现
针对不同的像素密度,可以使用媒体查询,选择不同精度的图片,以保证图片不失真
适配不同的屏幕大小,使用rem、em、vw、vh等相对单位
十六、选择器优先级
继承/*/子/后代:0
标签/伪元素:1
属性/类/伪类:10
id:100
行内:1000
!important:无穷大
十七、继承与非继承属性
随便记几个
继承:font-family、font-size、line-height、color、visibility、cursor
非继承:display、width、height、vertical-align、定位、background
十八、单行、多行文本溢出隐藏
单行文本溢出
overflow: hidden;
text-overflow: ellipsis;
white-space: nowrap;
多行文本溢出
overflow: hidden;
text-overflow: ellipsis;
display: -webkit-box;
-webkit-box-orient: vertical;
-webkit-line-clamp: 3;
### 最后
整理面试题,不是让大家去只刷面试题,而是熟悉目前实际面试中常见的考察方式和知识点,做到心中有数,也可以用来自查及完善知识体系。
**[开源分享:【大厂前端面试题解析+核心总结学习笔记+真实项目实战+最新讲解视频】](https://bbs.csdn.net/topics/618166371)**
**《前端基础面试题》,《前端校招面试题精编解析大全》,《前端面试题宝典》,《前端面试题:常用算法》**
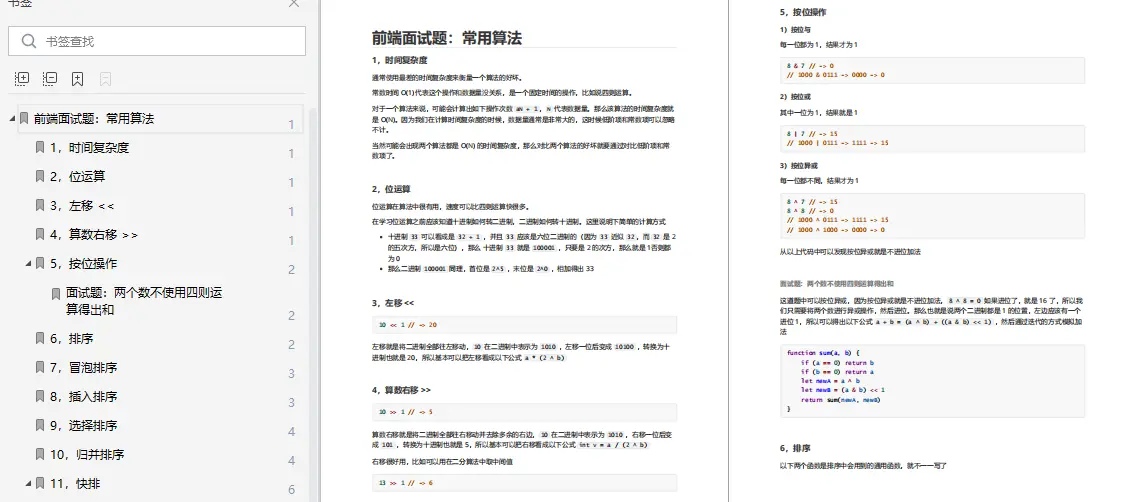
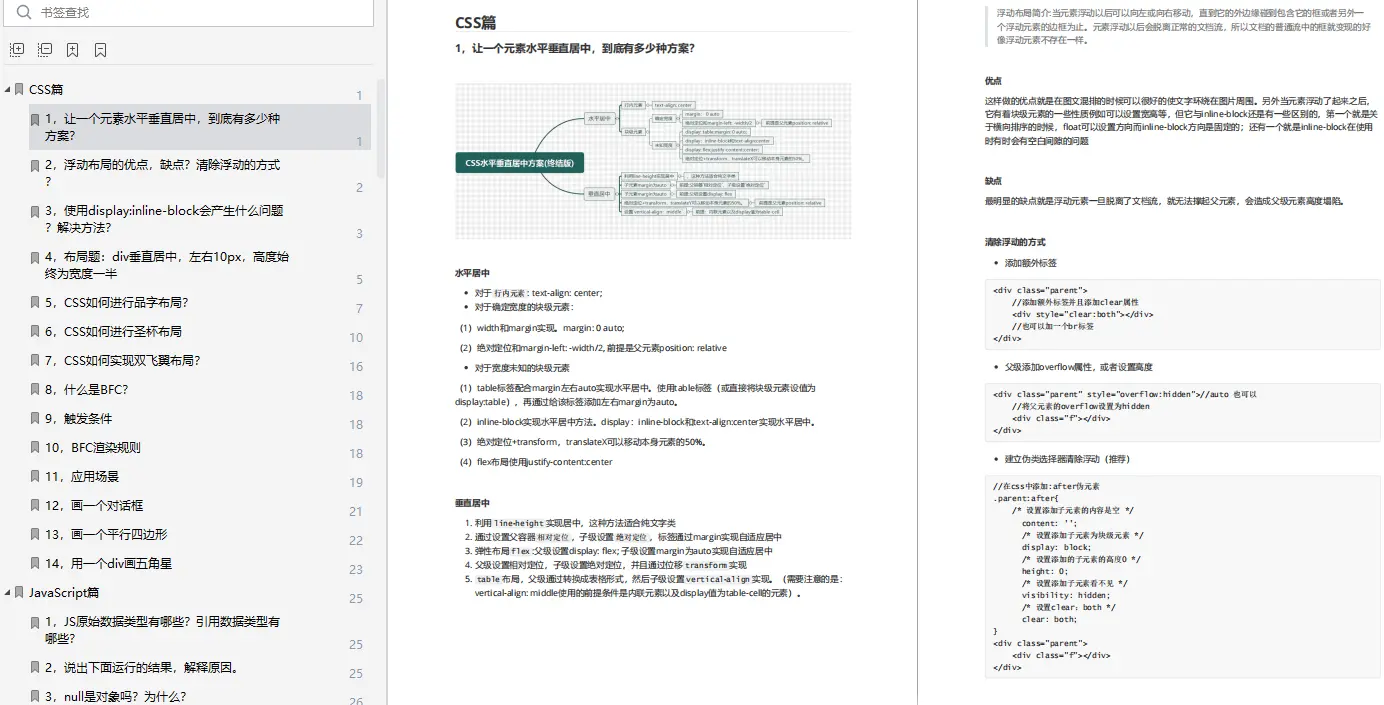
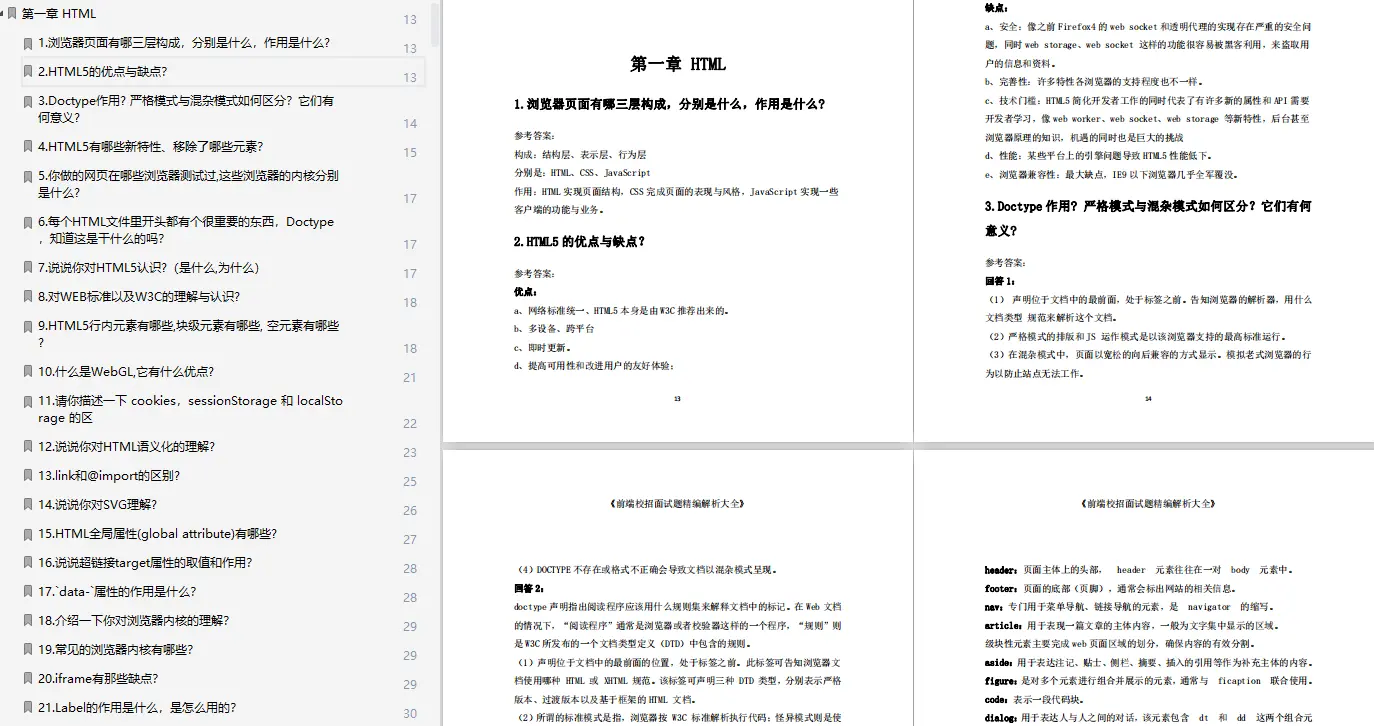
t、vertical-align、定位、background
#### 十八、单行、多行文本溢出隐藏
单行文本溢出
overflow: hidden;
text-overflow: ellipsis;
white-space: nowrap;
多行文本溢出
overflow: hidden;
text-overflow: ellipsis;
display: -webkit-box;
-webkit-box-orient: vertical;
-webkit-line-clamp: 3;
最后
整理面试题,不是让大家去只刷面试题,而是熟悉目前实际面试中常见的考察方式和知识点,做到心中有数,也可以用来自查及完善知识体系。
开源分享:【大厂前端面试题解析+核心总结学习笔记+真实项目实战+最新讲解视频】
《前端基础面试题》,《前端校招面试题精编解析大全》,《前端面试题宝典》,《前端面试题:常用算法》
[外链图片转存中…(img-YxrF7UYv-1714630819477)]
[外链图片转存中…(img-Eo4MrI7y-1714630819478)]
[外链图片转存中…(img-Oxcr3uTj-1714630819479)]