[[1, 0, 0, 0, 1, 0, 0],
[0, 1, 0, 0, 0, 1, 0],
[0, 0, 1, 0, 0, 0, 1],
[0, 0, 0, 1, 0, 0, 0],
[0, 0, 0, 0, 1, 0, 0],
[0, 0, 0, 0, 0, 1, 0],
[0, 0, 0, 0, 0, 0, 1]])
self.kf.H = np.array(
[[1, 0, 0, 0, 0, 0, 0], [0, 1, 0, 0, 0, 0, 0], [0, 0, 1, 0, 0, 0, 0], [0, 0, 0, 1, 0, 0, 0]])
self.kf.R[2:, 2:] \*= 10.
self.kf.P[4:, 4:] \*= 1000.
self.kf.P \*= 10.
self.kf.Q[-1, -1] \*= 0.01
self.kf.Q[4:, 4:] \*= 0.01
self.kf.x[:4] = convert_bbox_to_z(bbox)
self.time_since_update = 0
self.id = KalmanBoxTracker.count
KalmanBoxTracker.count += 1
self.history = []
self.hits = 0
self.hit_streak = 0
self.age = 0
def update(self, bbox):
self.time_since_update = 0
self.history = []
self.hits += 1
self.hit_streak += 1
self.kf.update(convert_bbox_to_z(bbox))
def predict(self):
if ((self.kf.x[6] + self.kf.x[2]) <= 0):
self.kf.x[6] \*= 0.0
self.kf.predict()
self.age += 1
if (self.time_since_update > 0):
self.hit_streak = 0
self.time_since_update += 1
self.history.append(convert_x_to_bbox(self.kf.x))
return self.history[-1]
def get\_state(self):
return convert_x_to_bbox(self.kf.x)
def associate_detections_to_trackers(detections, trackers, iou_threshold=0.3):
if (len(trackers) == 0):
return np.empty((0, 2), dtype=int), np.arange(len(detections)), np.empty((0, 5), dtype=int)
iou_matrix = iou_batch(detections, trackers)
if min(iou_matrix.shape) > 0:
a = (iou_matrix > iou_threshold).astype(np.int32)
if a.sum(1).max() == 1 and a.sum(0).max() == 1:
matched_indices = np.stack(np.where(a), axis=1)
else:
matched_indices = linear_assignment(-iou_matrix)
else:
matched_indices = np.empty(shape=(0, 2))
unmatched_detections = []
for d, det in enumerate(detections):
if (d not in matched_indices[:, 0]):
unmatched_detections.append(d)
unmatched_trackers = []
for t, trk in enumerate(trackers):
if (t not in matched_indices[:, 1]):
unmatched_trackers.append(t)
matches = []
for m in matched_indices:
if (iou_matrix[m[0], m[1]] < iou_threshold):
unmatched_detections.append(m[0])
unmatched_trackers.append(m[1])
else:
matches.append(m.reshape(1, 2))
if (len(matches) == 0):
matches = np.empty((0, 2), dtype=int)
else:
matches = np.concatenate(matches, axis=0)
return matches, np.array(unmatched_detections), np.array(unmatched_trackers)
class Sort(object):
def __init__(self, max_age=1, min_hits=3, iou_threshold=0.3):
self.max_age = max_age
self.min_hits = min_hits
self.iou_threshold = iou_threshold
self.trackers = []
self.frame_count = 0
def update(self, dets=np.empty((0, 5))):
self.frame_count += 1
# 根据上一帧航迹的框 预测当前帧的框.
trks = np.zeros((len(self.trackers), 5))
to_del = []
ret = []
for t, trk in enumerate(trks):
pos = self.trackers[t].predict()[0]
trk[:] = [pos[0], pos[1], pos[2], pos[3], 0]
if np.any(np.isnan(pos)):
to_del.append(t)
trks = np.ma.compress_rows(np.ma.masked_invalid(trks))
for t in reversed(to_del):
self.trackers.pop(t)
# 匈牙利匹配 上一帧预测框与当前帧检测框进行 iou 匹配
matched, unmatched_dets, unmatched_trks = associate_detections_to_trackers(dets, trks, self.iou_threshold)
# 如果匹配上 则更新修正当前检测框
for m in matched:
self.trackers[m[1]].update(dets[m[0], :])
# 如果检测框未匹配上,则当作新目标,新起航迹
for i in unmatched_dets:
trk = KalmanBoxTracker(dets[i, :])
self.trackers.append(trk)
i = len(self.trackers)
for trk in reversed(self.trackers):
d = trk.get_state()[0]
if (trk.time_since_update < 1) and (trk.hit_streak >= self.min_hits or self.frame_count <= self.min_hits):
ret.append(np.concatenate((d, [trk.id + 1])).reshape(1, -1))
i -= 1
# 如果超过self.max\_age(3)帧都没有匹配上,则应该去除这个航迹
if (trk.time_since_update > self.max_age):
self.trackers.pop(i)
if (len(ret) > 0):
return np.concatenate(ret)
return np.empty((0, 5))
if name == ‘__main__’:
display, video_save = True, True # 是否show,结果是否存视频
max_age, min_hits, iou_threshold = 3, 3, 0.3 # sort算法参数
colours = 255 * np.random.rand(32, 3) # 随机生产颜色
video = cv2.VideoWriter(“video.mp4”, cv2.VideoWriter_fourcc(‘m’, ‘p’, ‘4’, ‘v’), 10,
(1920, 1080)) if video_save else None
mot_tracker = Sort(max_age=max_age, min_hits=min_hits, iou_threshold=iou_threshold) # 创建sort跟踪器
seq_dets = np.loadtxt(“det.txt”, delimiter=‘,’) # 加载检测txt结果
for frame in range(int(seq_dets[:, 0].max())):
frame += 1 # 从1帧开始
dets = seq_dets[seq_dets[:, 0] == frame, 2:7]
dets[:, 2:4] += dets[:, 0:2] # [x1,y1,w,h] to [x1,y1,x2,y2] 左上角x1,y1,w,h ——>左上角x1,y1,右下角x2,y2
mot_tracker.update(dets) # kalman 预测与更新
trackers = mot_tracker.trackers
image_path = os.path.join(“.\img”, ‘%06d.jpg’ % (frame)) # 图片路径
image = cv2.imread(image_path)
for d in trackers:
x1, y1, w, h = d.get_state()[0] # 获取 当前目标框状态
id = d.id
color = colours[int(id) % 32, :]
color = (int(color[0]), int(color[1]), int(color[2]))
cv2.rectangle(image, (int(x1), int(y1)), (int(w), int(h)), color, 3) # 画框
cv2.putText(image, str(int(id)), (int(x1), int(y1) - 10), cv2.FONT_HERSHEY_SIMPLEX, 1,
color, 3) # 画id
if display:
cv2.namedWindow(“show”)
cv2.imshow(“show”, image)
cv2.waitKey(0)
if video_save:
video.write(image)
---
## 算法分析
>
> * 优点:速度,快,很快,非常快。1s可以处理超过1000帧检测结果。
> * 缺点:对于遮挡、以及非线性运动的物体(加减速或者转弯)跟踪效果差
> * 优化方向:优化方式有很多,下次再写博客分享,如果有机会的话[鬼脸.jpg]。
>
>
>
学好 Python 不论是就业还是做副业赚钱都不错,但要学会 Python 还是要有一个学习规划。最后大家分享一份全套的 Python 学习资料,给那些想学习 Python 的小伙伴们一点帮助!
### 一、Python所有方向的学习路线
Python所有方向路线就是把Python常用的技术点做整理,形成各个领域的知识点汇总,它的用处就在于,你可以按照上面的知识点去找对应的学习资源,保证自己学得较为全面。
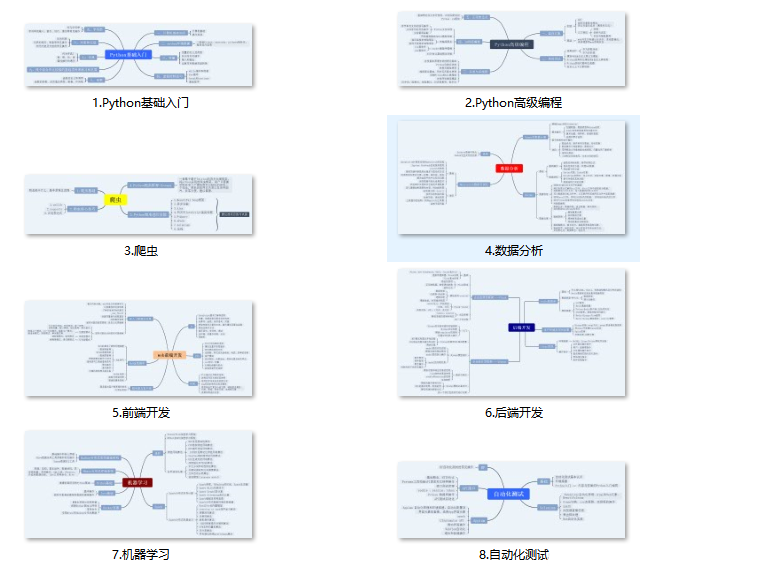
### 二、学习软件
工欲善其事必先利其器。学习Python常用的开发软件都在这里了,给大家节省了很多时间。
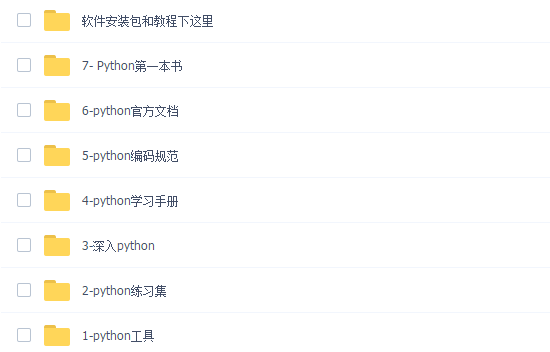
### 三、全套PDF电子书
书籍的好处就在于权威和体系健全,刚开始学习的时候你可以只看视频或者听某个人讲课,但等你学完之后,你觉得你掌握了,这时候建议还是得去看一下书籍,看权威技术书籍也是每个程序员必经之路。
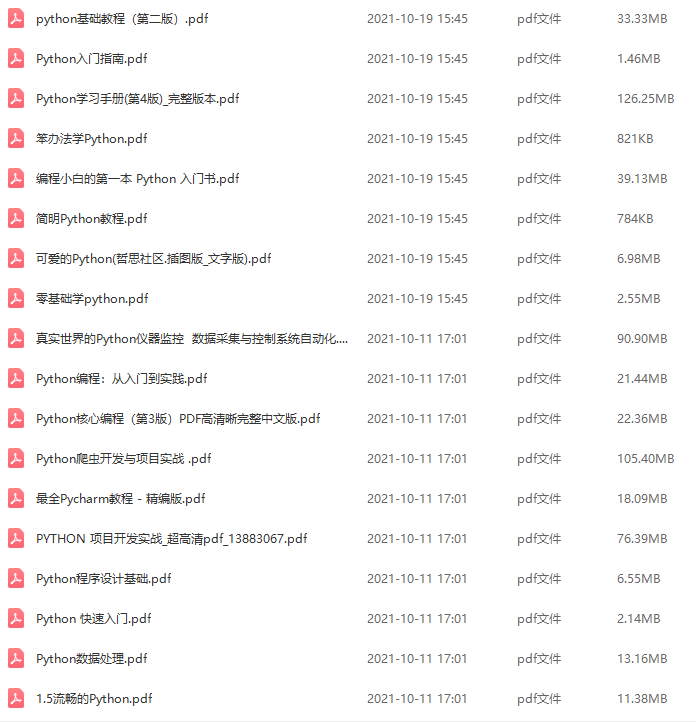
### 四、入门学习视频
我们在看视频学习的时候,不能光动眼动脑不动手,比较科学的学习方法是在理解之后运用它们,这时候练手项目就很适合了。
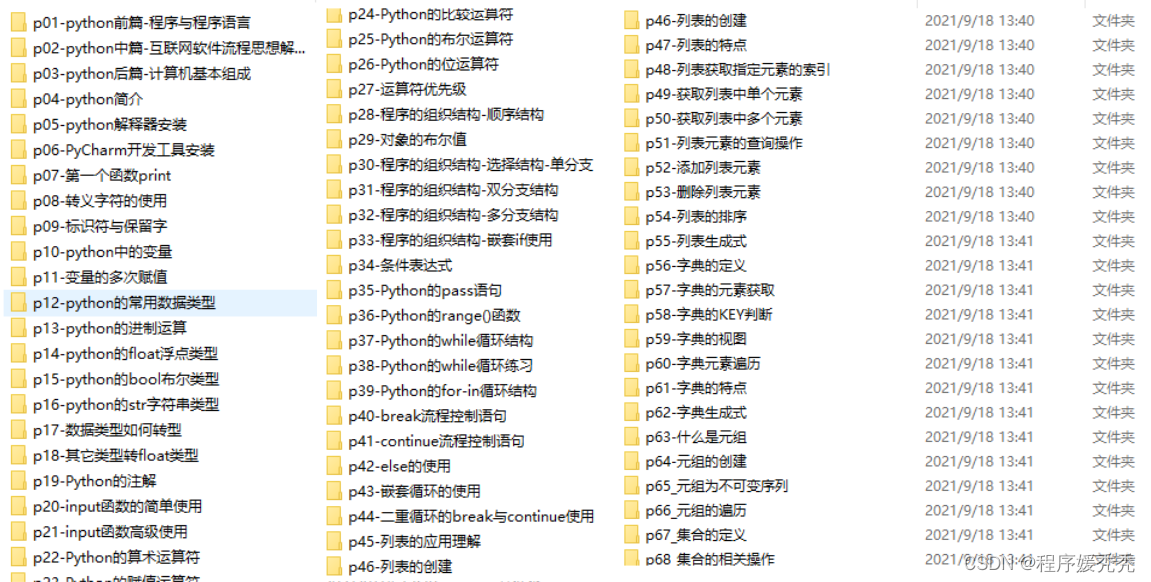
### 五、实战案例
光学理论是没用的,要学会跟着一起敲,要动手实操,才能将自己的所学运用到实际当中去,这时候可以搞点实战案例来学习。
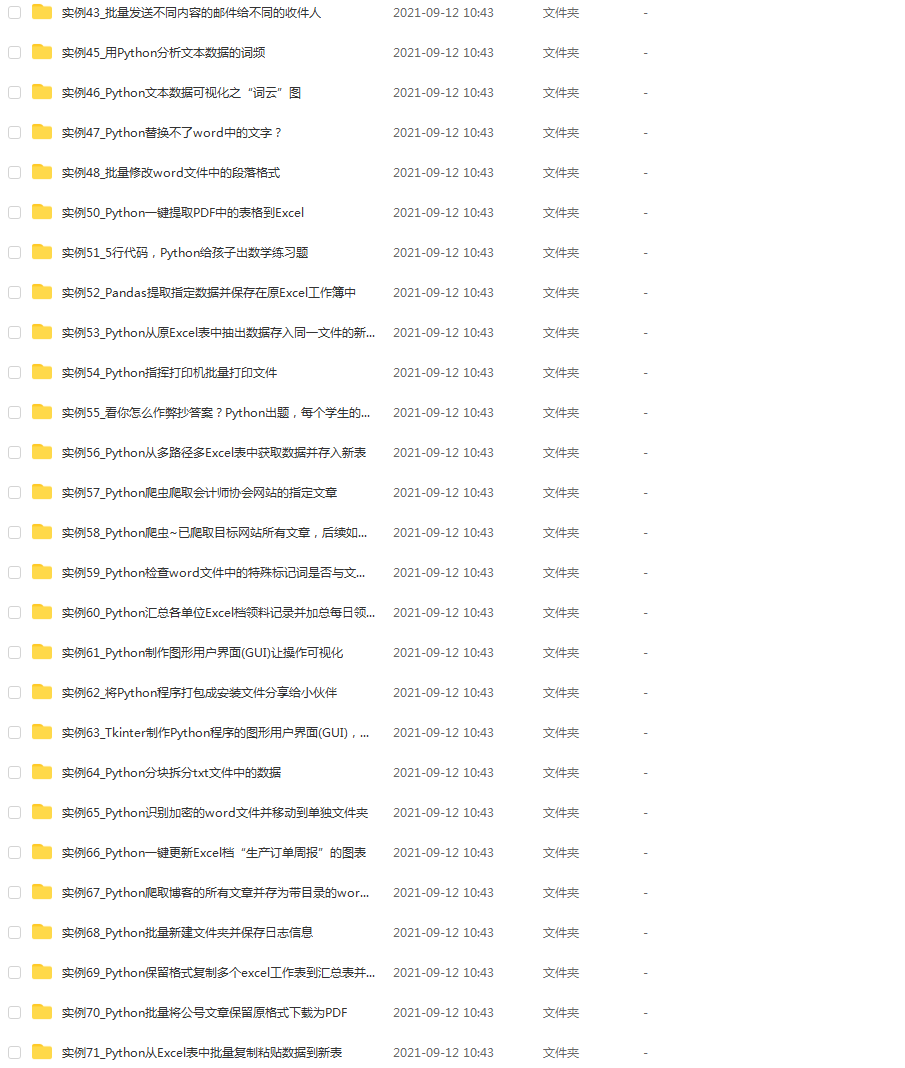
### 六、面试资料
我们学习Python必然是为了找到高薪的工作,下面这些面试题是来自阿里、腾讯、字节等一线互联网大厂最新的面试资料,并且有阿里大佬给出了权威的解答,刷完这一套面试资料相信大家都能找到满意的工作。
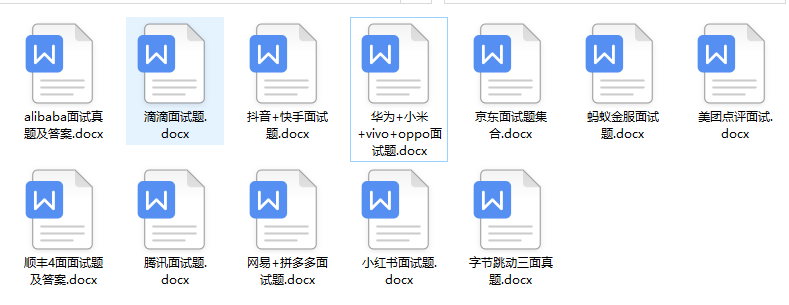
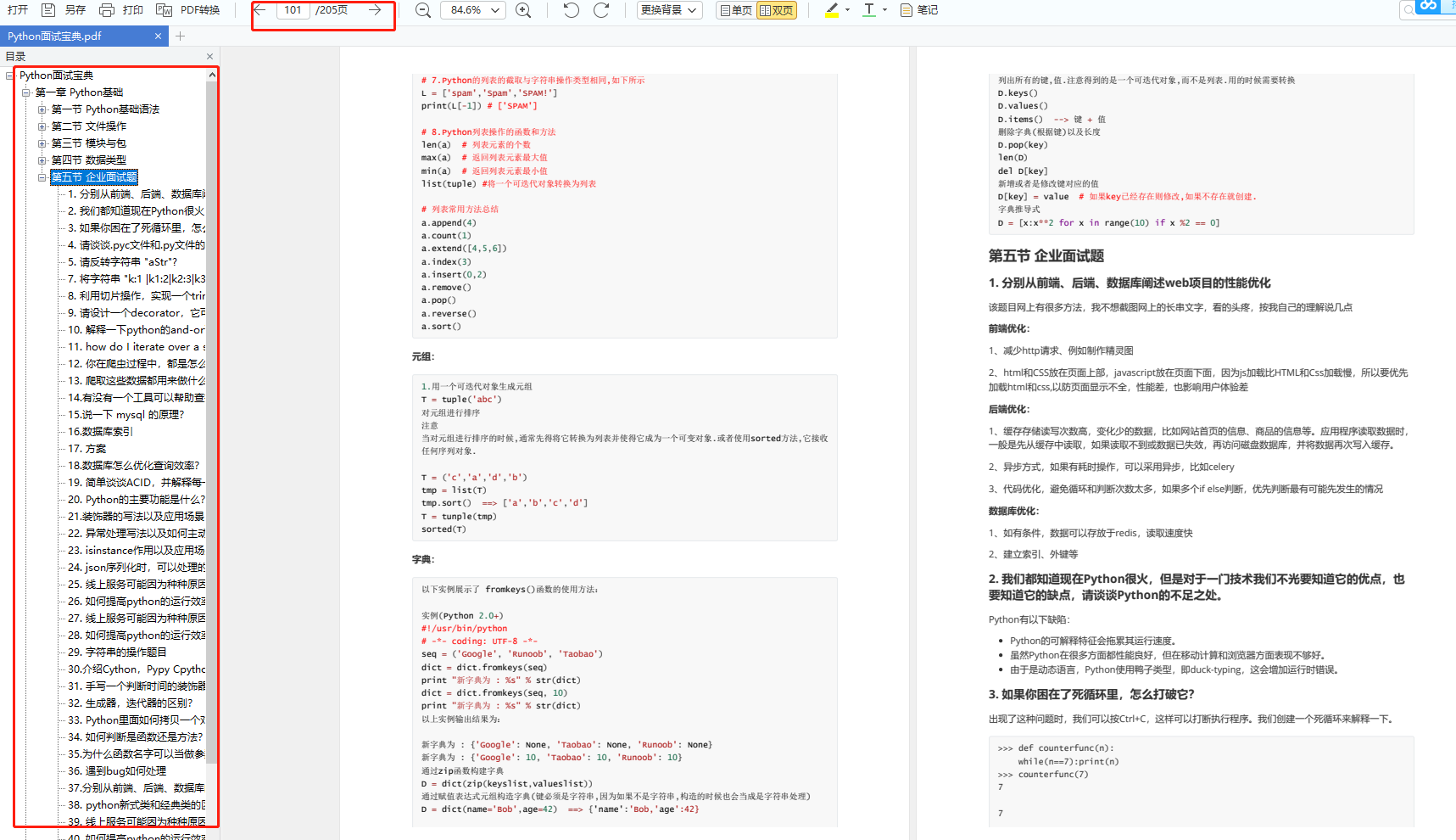
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化学习资料的朋友,可以戳这里无偿获取](https://bbs.csdn.net/topics/618317507)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**