网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
constructor(a: ClassA) {
this.a = a;
}
}
@Observed
class ClassD {
public c: ClassC;
constructor(c: ClassC) {
this.c = c;
}
}
@Observed
class ClassC extends ClassA {
public k: number;
constructor(k: number) {
// 调用父类方法对k进行处理
super(k);
this.k = k;
}
}
以下组件层次结构呈现的是嵌套类对象的数据结构。
@Component
struct ViewC {
label: string = ‘ViewC1’;
@ObjectLink c: ClassC;
build() {
Row() {
Column() {
Text(ViewC [${this.label}] this.a.c = ${this.c.c}
)
.fontColor(‘#ffffffff’)
.backgroundColor(‘#ff3fc4c4’)
.height(50)
.borderRadius(25)
Button(ViewC: this.c.c add 1
)
.backgroundColor(‘#ff7fcf58’)
.onClick(() => {
this.c.c += 1;
console.log(‘this.c.c:’ + this.c.c)
})
}
.width(300)
}
}
}
@Entry
@Component
struct ViewB {
@State b: ClassB = new ClassB(new ClassA(0));
@State child: ClassD = new ClassD(new ClassC(0));
build() {
Column() {
ViewC({ label: ‘ViewC #3’,
c: this.child.c })
Button(ViewC: this.child.c.c add 10
)
.backgroundColor(‘#ff7fcf58’)
.onClick(() => {
this.child.c.c += 10
console.log(‘this.child.c.c:’ + this.child.c.c)
})
}
}
}
被@Observed装饰的ClassC类,可以观测到继承基类的属性的变化。
ViewB中的事件句柄:
* this.child.c = new ClassA(0) 和this.b = new ClassB(new ClassA(0)): 对@State装饰的变量b和其属性的修改。
* this.child.c.c = … :该变化属于第二层的变化,@State无法观察到第二层的变化,但是ClassA被@Observed装饰,ClassA的属性c的变化可以被@ObjectLink观察到。
ViewC中的事件句柄:
* this.c.c += 1:对@ObjectLink变量a的修改,将触发Button组件的刷新。@ObjectLink和@Prop不同,@ObjectLink不拷贝来自父组件的数据源,而是在本地构建了指向其数据源的引用。
* @ObjectLink变量是只读的,this.a = new ClassA(…)是不允许的,因为一旦赋值操作发生,指向数据源的引用将被重置,同步将被打断。
#### 对象数组
对象数组是一种常用的数据结构。以下示例展示了数组对象的用法。
let NextID: number = 1;
@Observed
class ClassA {
public id: number;
public c: number;
constructor(c: number) {
this.id = NextID++;
this.c = c;
}
}
@Component
struct ViewA {
// 子组件ViewA的@ObjectLink的类型是ClassA
@ObjectLink a: ClassA;
label: string = ‘ViewA1’;
build() {
Row() {
Button(ViewA [${this.label}] this.a.c = ${this.a ? this.a.c : "undefined"}
)
.onClick(() => {
this.a.c += 1;
})
}
}
}
@Entry
@Component
struct ViewB {
// ViewB中有@State装饰的ClassA[]
@State arrA: ClassA[] = [new ClassA(0), new ClassA(0)];
build() {
Column() {
ForEach(this.arrA,
(item: ClassA) => {
ViewA({ label: #${item.id}
, a: item })
},
(item: ClassA): string => item.id.toString()
)
// 使用@State装饰的数组的数组项初始化@ObjectLink,其中数组项是被@Observed装饰的ClassA的实例
ViewA({ label: ViewA this.arrA[first]
, a: this.arrA[0] })
ViewA({ label: ViewA this.arrA[last]
, a: this.arrA[this.arrA.length-1] })
Button(`ViewB: reset array`)
.onClick(() => {
this.arrA = [new ClassA(0), new ClassA(0)];
})
Button(`ViewB: push`)
.onClick(() => {
this.arrA.push(new ClassA(0))
})
Button(`ViewB: shift`)
.onClick(() => {
if (this.arrA.length > 0) {
this.arrA.shift()
} else {
console.log("length <= 0")
}
})
Button(`ViewB: chg item property in middle`)
.onClick(() => {
this.arrA[Math.floor(this.arrA.length / 2)].c = 10;
})
Button(`ViewB: chg item property in middle`)
.onClick(() => {
this.arrA[Math.floor(this.arrA.length / 2)] = new ClassA(11);
})
}
}
}
* this.arrA[Math.floor(this.arrA.length/2)] = new ClassA(…) :该状态变量的改变触发2次更新:
1. ForEach:数组项的赋值导致ForEach的[itemGenerator](arkts-rendering-control-foreach.md#%E6%8E%A5%E5%8F%A3%E6%8F%8F%E8%BF%B0)被修改,因此数组项被识别为有更改,ForEach的item builder将执行,创建新的ViewA组件实例。
2. ViewA({ label: `ViewA this.arrA[last]`, a: this.arrA[this.arrA.length-1] }):上述更改改变了数组中第二个元素,所以绑定this.arrA[1]的ViewA将被更新。
* this.arrA.push(new ClassA(0)) : 将触发2次不同效果的更新:
1. ForEach:新添加的ClassA对象对于ForEach是未知的 itemGenerator ,ForEach的item builder将执行,创建新的ViewA组件实例。
2. ViewA({ label: `ViewA this.arrA[last]`, a: this.arrA[this.arrA.length-1] }):数组的最后一项有更改,因此引起第二个ViewA的实例的更改。对于ViewA({ label: `ViewA this.arrA[first]`, a: this.arrA[0] }),数组的更改并没有触发一个数组项更改的改变,所以第一个ViewA不会刷新。
* this.arrA[Math.floor(this.arrA.length/2)].c:@State无法观察到第二层的变化,但是ClassA被@Observed装饰,ClassA的属性的变化将被@ObjectLink观察到。
#### 二维数组
使用@Observed观察二维数组的变化。可以声明一个被@Observed装饰的继承Array的子类。
@Observed
class StringArray extends Array {
}
使用new StringArray()来构造StringArray的实例,new运算符使得@Observed生效,@Observed观察到StringArray的属性变化。
声明一个从Array扩展的类class StringArray extends Array<String> {},并创建StringArray的实例。@Observed装饰的类需要使用new运算符来构建class实例。
@Observed
class StringArray extends Array {
}
@Component
struct ItemPage {
@ObjectLink itemArr: StringArray;
build() {
Row() {
Text(‘ItemPage’)
.width(100).height(100)
ForEach(this.itemArr,
(item: string | Resource) => {
Text(item)
.width(100).height(100)
},
(item: string) => item
)
}
}
}
@Entry
@Component
struct IndexPage {
@State arr: Array = [new StringArray(), new StringArray(), new StringArray()];
build() {
Column() {
ItemPage({ itemArr: this.arr[0] })
ItemPage({ itemArr: this.arr[1] })
ItemPage({ itemArr: this.arr[2] })
Divider()
ForEach(this.arr,
(itemArr: StringArray) => {
ItemPage({ itemArr: itemArr })
},
(itemArr: string) => itemArr[0]
)
Divider()
Button('update')
.onClick(() => {
console.error('Update all items in arr');
if ((this.arr[0] as Array<String>)[0] !== undefined) {
// 正常情况下需要有一个真实的ID来与ForEach一起使用,但此处没有
// 因此需要确保推送的字符串是唯一的。
this.arr[0].push(`${this.arr[0].slice(-1).pop()}${this.arr[0].slice(-1).pop()}`);
this.arr[1].push(`${this.arr[1].slice(-1).pop()}${this.arr[1].slice(-1).pop()}`);
this.arr[2].push(`${this.arr[2].slice(-1).pop()}${this.arr[2].slice(-1).pop()}`);
} else {
this.arr[0].push('Hello');
this.arr[1].push('World');
this.arr[2].push('!');
}
})
}
}
}
#### 继承Map类
>
> **说明:**
>
>
> 从API version 11开始,@ObjectLink支持@Observed装饰Map类型和继承Map类的类型。
>
>
>
在下面的示例中,myMap类型为MyMap<number, string>,点击Button改变myMap的属性,视图会随之刷新。
@Observed
class ClassA {
public a: MyMap<number, string>;
constructor(a: MyMap<number, string>) {
this.a = a;
}
}
@Observed
export class MyMap<K, V> extends Map<K, V> {
public name: string;
constructor(name?: string, args?: [K, V][]) {
super(args);
this.name = name ? name : “My Map”;
}
getName() {
return this.name;
}
}
@Entry
@Component
struct MapSampleNested {
@State message: ClassA = new ClassA(new MyMap(“myMap”, [[0, “a”], [1, “b”], [3, “c”]]));
build() {
Row() {
Column() {
MapSampleNestedChild({ myMap: this.message.a })
}
.width(‘100%’)
}
.height(‘100%’)
}
}
@Component
struct MapSampleNestedChild {
@ObjectLink myMap: MyMap<number, string>
build() {
Row() {
Column() {
ForEach(Array.from(this.myMap.entries()), (item: [number, string]) => {
Text(${item[0]}
).fontSize(30)
Text(${item[1]}
).fontSize(30)
Divider()
})
Button('set new one').onClick(() => {
this.myMap.set(4, "d")
})
Button('clear').onClick(() => {
this.myMap.clear()
})
Button('replace the first one').onClick(() => {
this.myMap.set(0, "aa")
})
Button('delete the first one').onClick(() => {
this.myMap.delete(0)
})
}
.width('100%')
}
.height('100%')
}
}
#### 继承Set类
>
> **说明:**
>
>
> 从API version 11开始,@ObjectLink支持@Observed装饰Set类型和继承Set类的类型。
>
>
>
在下面的示例中,mySet类型为MySet<number>,点击Button改变mySet的属性,视图会随之刷新。
@Observed
class ClassA {
public a: MySet;
constructor(a: MySet) {
this.a = a;
}
}
@Observed
export class MySet extends Set {
public name: string;
constructor(name?: string, args?: T[]) {
super(args);
this.name = name ? name : “My Set”;
}
getName() {
return this.name;
}
}
@Entry
@Component
struct SetSampleNested {
@State message: ClassA = new ClassA(new MySet(“Set”, [0, 1, 2, 3, 4]));
build() {
Row() {
Column() {
SetSampleNestedChild({ mySet: this.message.a })
}
.width(‘100%’)
}
.height(‘100%’)
}
}
@Component
struct SetSampleNestedChild {
@ObjectLink mySet: MySet
build() {
Row() {
Column() {
ForEach(Array.from(this.mySet.entries()), (item: number) => {
Text(${item}
).fontSize(30)
Divider()
})
Button(‘set new one’).onClick(() => {
this.mySet.add(5)
})
Button(‘clear’).onClick(() => {
this.mySet.clear()
})
Button(‘delete the first one’).onClick(() => {
this.mySet.delete(0)
})
}
.width(‘100%’)
}
.height(‘100%’)
}
}
### ObjectLink支持联合类型
@ObjectLink支持@Observed装饰类和undefined或null组成的联合类型,在下面的示例中,count类型为ClassA | ClassB | undefined,点击父组件Page2中的Button改变count的属性或者类型,Child中也会对应刷新。
@Observed
class ClassA {
public a: number;
constructor(a: number) {
this.a = a;
}
}
@Observed
class ClassB {
public b: number;
constructor(b: number) {
this.b = b;
}
}
@Entry
@Component
struct Page2 {
@State count: ClassA | ClassB | undefined = new ClassA(10)
build() {
Column() {
Child({ count: this.count })
Button('change count property')
.onClick(() => {
// 判断count的类型,做属性的更新
if (this.count instanceof ClassA) {
this.count.a += 1
} else if (this.count instanceof ClassB) {
this.count.b += 1
} else {
console.info('count is undefined, cannot change property')
}
})
Button('change count to ClassA')
.onClick(() => {
// 赋值为ClassA的实例
this.count = new ClassA(100)
})
Button('change count to ClassB')
.onClick(() => {
// 赋值为ClassA的实例
this.count = new ClassB(100)
})
Button('change count to undefined')
.onClick(() => {
// 赋值为undefined
this.count = undefined
})
}.width('100%')
}
}
@Component
struct Child {
@ObjectLink count: ClassA | ClassB | undefined
build() {
Column() {
Text(count is instanceof ${this.count instanceof ClassA ? 'ClassA' : this.count instanceof ClassB ? 'ClassB' : 'undefined'}
)
.fontSize(30)
Text(`count's property is ${this.count instanceof ClassA ? this.count.a : this.count?.b}`).fontSize(15)
}.width('100%')
}
}
### 常见问题
#### 在子组件中给@ObjectLink装饰的变量赋值
在子组件中给@ObjectLink装饰的变量赋值是不允许的。
【反例】
@Observed
class ClassA {
public c: number = 0;
constructor(c: number) {
this.c = c;
}
}
@Component
struct ObjectLinkChild {
@ObjectLink testNum: ClassA;
build() {
Text(ObjectLinkChild testNum ${this.testNum.c}
)
.onClick(() => {
// ObjectLink不能被赋值
this.testNum = new ClassA(47);
})
}
}
@Entry
@Component
struct Parent {
@State testNum: ClassA[] = [new ClassA(1)];
build() {
Column() {
Text(Parent testNum ${this.testNum[0].c}
)
.onClick(() => {
this.testNum[0].c += 1;
})
ObjectLinkChild({ testNum: this.testNum[0] })
}
}
}
点击ObjectLinkChild给@ObjectLink装饰的变量赋值:
this.testNum = new ClassA(47);
这是不允许的,对于实现双向数据同步的@ObjectLink,赋值相当于要更新父组件中的数组项或者class的属性,这个对于 TypeScript/JavaScript是不能实现的。框架对于这种行为会发生运行时报错。
【正例】
@Observed
class ClassA {
public c: number = 0;
constructor(c: number) {
this.c = c;
}
}
@Component
struct ObjectLinkChild {
@ObjectLink testNum: ClassA;
build() {
Text(ObjectLinkChild testNum ${this.testNum.c}
)
.onClick(() => {
// 可以对ObjectLink装饰对象的属性赋值
this.testNum.c = 47;
})
}
}
@Entry
@Component
struct Parent {
@State testNum: ClassA[] = [new ClassA(1)];
build() {
Column() {
Text(Parent testNum ${this.testNum[0].c}
)
.onClick(() => {
this.testNum[0].c += 1;
})
ObjectLinkChild({ testNum: this.testNum[0] })
}
}
}
#### 基础嵌套对象属性更改失效
在应用开发中,有很多嵌套对象场景,例如,开发者更新了某个属性,但UI没有进行对应的更新。
每个装饰器都有自己可以观察的能力,并不是所有的改变都可以被观察到,只有可以被观察到的变化才会进行UI更新。@Observed装饰器可以观察到嵌套对象的属性变化,其他装饰器仅能观察到第二层的变化。
【反例】
下面的例子中,一些UI组件并不会更新。
class ClassA {
a: number;
constructor(a: number) {
this.a = a;
}
getA(): number {
return this.a;
}
setA(a: number): void {
this.a = a;
}
}
class ClassC {
c: number;
constructor(c: number) {
this.c = c;
}
getC(): number {
return this.c;
}
setC(c: number): void {
this.c = c;
}
}
class ClassB extends ClassA {
b: number = 47;
c: ClassC;
constructor(a: number, b: number, c: number) {
super(a);
this.b = b;
this.c = new ClassC©;
}
getB(): number {
return this.b;
}
setB(b: number): void {
this.b = b;
}
getC(): number {
return this.c.getC();
}
setC(c: number): void {
return this.c.setC©;
}
}
@Entry
@Component
struct MyView {
@State b: ClassB = new ClassB(10, 20, 30);
build() {
Column({ space: 10 }) {
Text(a: ${this.b.a}
)
Button(“Change ClassA.a”)
.onClick(() => {
this.b.a += 1;
})
Text(`b: ${this.b.b}`)
Button("Change ClassB.b")
.onClick(() => {
this.b.b += 1;
})
Text(`c: ${this.b.c.c}`)
Button("Change ClassB.ClassC.c")
.onClick(() => {
// 点击时上面的Text组件不会刷新
this.b.c.c += 1;
})
}
}
}
* 最后一个Text组件Text(‘c: ${this.b.c.c}’),当点击该组件时UI不会刷新。 因为,@State b : ClassB 只能观察到this.b属性的变化,比如this.b.a, this.b.b 和this.b.c的变化,但是无法观察嵌套在属性中的属性,即this.b.c.c(属性c是内嵌在b中的对象classC的属性)。
* 为了观察到嵌套于内部的ClassC的属性,需要做如下改变:
+ 构造一个子组件,用于单独渲染ClassC的实例。 该子组件可以使用@ObjectLink c : ClassC或@Prop c : ClassC。通常会使用@ObjectLink,除非子组件需要对其ClassC对象进行本地修改。
+ 嵌套的ClassC必须用@Observed装饰。当在ClassB中创建ClassC对象时(本示例中的ClassB(10, 20, 30)),它将被包装在ES6代理中,当ClassC属性更改时(this.b.c.c += 1),该代码将修改通知到@ObjectLink变量。
【正例】
以下示例使用@Observed/@ObjectLink来观察嵌套对象的属性更改。
class ClassA {
a: number;
constructor(a: number) {
this.a = a;
}
getA(): number {
return this.a;
}
setA(a: number): void {
this.a = a;
}
}
@Observed
class ClassC {
c: number;
constructor(c: number) {
this.c = c;
}
getC(): number {
return this.c;
}
setC(c: number): void {
this.c = c;
}
}
class ClassB extends ClassA {
b: number = 47;
c: ClassC;
constructor(a: number, b: number, c: number) {
super(a);
this.b = b;
this.c = new ClassC©;
}
getB(): number {
return this.b;
}
setB(b: number): void {
this.b = b;
}
getC(): number {
return this.c.getC();
}
setC(c: number): void {
return this.c.setC©;
}
}
@Component
struct ViewClassC {
@ObjectLink c: ClassC;
build() {
Column({ space: 10 }) {
Text(c: ${this.c.getC()}
)
Button(“Change C”)
.onClick(() => {
this.c.setC(this.c.getC() + 1);
})
}
}
}
@Entry
@Component
struct MyView {
@State b: ClassB = new ClassB(10, 20, 30);
build() {
Column({ space: 10 }) {
Text(a: ${this.b.a}
)
Button(“Change ClassA.a”)
.onClick(() => {
this.b.a += 1;
})
Text(`b: ${this.b.b}`)
Button("Change ClassB.b")
.onClick(() => {
this.b.b += 1;
})
ViewClassC({ c: this.b.c }) // Text(`c: ${this.b.c.c}`)的替代写法
Button("Change ClassB.ClassC.c")
.onClick(() => {
this.b.c.c += 1;
})
}
}
}
#### 复杂嵌套对象属性更改失效
【反例】
以下示例创建了一个带有@ObjectLink装饰变量的子组件,用于渲染一个含有嵌套属性的ParentCounter,用@Observed装饰嵌套在ParentCounter中的SubCounter。
let nextId = 1;
@Observed
class SubCounter {
counter: number;
constructor(c: number) {
this.counter = c;
}
}
@Observed
class ParentCounter {
id: number;
counter: number;
subCounter: SubCounter;
incrCounter() {
this.counter++;
}
incrSubCounter(c: number) {
this.subCounter.counter += c;
}
setSubCounter(c: number): void {
this.subCounter.counter = c;
}
constructor(c: number) {
this.id = nextId++;
this.counter = c;
this.subCounter = new SubCounter©;
}
}
@Component
struct CounterComp {
@ObjectLink value: ParentCounter;
build() {
Column({ space: 10 }) {
Text(${this.value.counter}
)
.fontSize(25)
.onClick(() => {
this.value.incrCounter();
})
Text(${this.value.subCounter.counter}
)
.onClick(() => {
this.value.incrSubCounter(1);
})
Divider().height(2)
}
}
}
@Entry
@Component
struct ParentComp {
@State counter: ParentCounter[] = [new ParentCounter(1), new ParentCounter(2), new ParentCounter(3)];
build() {
Row() {
Column() {
CounterComp({ value: this.counter[0] })
CounterComp({ value: this.counter[1] })
CounterComp({ value: this.counter[2] })
Divider().height(5)
ForEach(this.counter,
(item: ParentCounter) => {
CounterComp({ value: item })
},
(item: ParentCounter) => item.id.toString()
)
Divider().height(5)
// 第一个点击事件
Text(‘Parent: incr counter[0].counter’)
.fontSize(20).height(50)
.onClick(() => {
this.counter[0].incrCounter();
// 每次触发时自增10
this.counter[0].incrSubCounter(10);
})
// 第二个点击事件
Text(‘Parent: set.counter to 10’)
.fontSize(20).height(50)
.onClick(() => {
// 无法将value设置为10,UI不会刷新
this.counter[0].setSubCounter(10);
})
Text(‘Parent: reset entire counter’)
.fontSize(20).height(50)
.onClick(() => {
this.counter = [new ParentCounter(1), new ParentCounter(2), new ParentCounter(3)];
})
}
}
}
}
对于Text(‘Parent: incr counter[0].counter’)的onClick事件,this.counter[0].incrSubCounter(10)调用incrSubCounter方法使SubCounter的counter值增加10,UI同步刷新。
但是,在Text(‘Parent: set.counter to 10’)的onClick中调用this.counter[0].setSubCounter(10),SubCounter的counter值却无法重置为10。
incrSubCounter和setSubCounter都是同一个SubCounter的函数。在第一个点击处理时调用incrSubCounter可以正确更新UI,而第二个点击处理调用setSubCounter时却没有更新UI。实际上incrSubCounter和setSubCounter两个函数都不能触发Text(‘${this.value.subCounter.counter}’)的更新,因为@ObjectLink value : ParentCounter仅能观察其代理ParentCounter的属性,对于this.value.subCounter.counter是SubCounter的属性,无法观察到嵌套类的属性。
但是,第一个click事件调用this.counter[0].incrCounter()将CounterComp自定义组件中@ObjectLink value: ParentCounter标记为已更改。此时触发Text(‘${this.value.subCounter.counter}’)的更新。 如果在第一个点击事件中删除this.counter[0].incrCounter(),也无法更新UI。
【正例】
对于上述问题,为了直接观察SubCounter中的属性,以便this.counter[0].setSubCounter(10)操作有效,可以利用下面的方法:
@ObjectLink value:ParentCounter = new ParentCounter(0);
@ObjectLink subValue:SubCounter = new SubCounter(0);
该方法使得@ObjectLink分别代理了ParentCounter和SubCounter的属性,这样对于这两个类的属性的变化都可以观察到,即都会对UI视图进行刷新。即使删除了上面所说的this.counter[0].incrCounter(),UI也会进行正确的刷新。
该方法可用于实现“两个层级”的观察,即外部对象和内部嵌套对象的观察。但是该方法只能用于@ObjectLink装饰器,无法作用于@Prop(@Prop通过深拷贝传入对象)。详情参考@Prop与@ObjectLink的差异。
let nextId = 1;
@Observed
class SubCounter {
counter: number;
constructor(c: number) {
this.counter = c;
}
}
@Observed
class ParentCounter {
id: number;
counter: number;
subCounter: SubCounter;
incrCounter() {
this.counter++;
}
incrSubCounter(c: number) {
this.subCounter.counter += c;
}
setSubCounter(c: number): void {
this.subCounter.counter = c;
}
constructor(c: number) {
this.id = nextId++;
this.counter = c;
this.subCounter = new SubCounter©;
}
}
@Component
struct CounterComp {
@ObjectLink value: ParentCounter;
build() {
Column({ space: 10 }) {
Text(${this.value.counter}
)
.fontSize(25)
.onClick(() => {
this.value.incrCounter();
})
CounterChild({ subValue: this.value.subCounter })
Divider().height(2)
}
}
}
@Component
struct CounterChild {
@ObjectLink subValue: SubCounter;
build() {
Text(${this.subValue.counter}
)
.onClick(() => {
this.subValue.counter += 1;
})
}
}
@Entry
@Component
struct ParentComp {
@State counter: ParentCounter[] = [new ParentCounter(1), new ParentCounter(2), new ParentCounter(3)];
build() {
Row() {
Column() {
CounterComp({ value: this.counter[0] })
CounterComp({ value: this.counter[1] })
CounterComp({ value: this.counter[2] })
Divider().height(5)
ForEach(this.counter,
(item: ParentCounter) => {
CounterComp({ value: item })
},
(item: ParentCounter) => item.id.toString()
)
Divider().height(5)
Text(‘Parent: reset entire counter’)
.fontSize(20).height(50)
.onClick(() => {
this.counter = [new ParentCounter(1), new ParentCounter(2), new ParentCounter(3)];
})
Text(‘Parent: incr counter[0].counter’)
.fontSize(20).height(50)
.onClick(() => {
this.counter[0].incrCounter();
this.counter[0].incrSubCounter(10);
})
Text(‘Parent: set.counter to 10’)
.fontSize(20).height(50)
.onClick(() => {
this.counter[0].setSubCounter(10);
})
}
}
}
}
#### @Prop与@ObjectLink的差异
在下面的示例代码中,@ObjectLink装饰的变量是对数据源的引用,即在this.value.subValue和this.subValue都是同一个对象的不同引用,所以在点击CounterComp的click handler,改变this.value.subCounter.counter,this.subValue.counter也会改变,对应的组件Text(`this.subValue.counter: ${this.subValue.counter}`)会刷新。
let nextId = 1;
@Observed
class SubCounter {
counter: number;
constructor(c: number) {
this.counter = c;
}
}
@Observed
class ParentCounter {
id: number;
counter: number;
subCounter: SubCounter;
incrCounter() {
this.counter++;
}
incrSubCounter(c: number) {
this.subCounter.counter += c;
}
setSubCounter(c: number): void {
this.subCounter.counter = c;
}
constructor(c: number) {
this.id = nextId++;
深知大多数程序员,想要提升技能,往往是自己摸索成长,但自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上鸿蒙开发知识点,真正体系化!
由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新
@ObjectLink的差异
在下面的示例代码中,@ObjectLink装饰的变量是对数据源的引用,即在this.value.subValue和this.subValue都是同一个对象的不同引用,所以在点击CounterComp的click handler,改变this.value.subCounter.counter,this.subValue.counter也会改变,对应的组件Text(this.subValue.counter: ${this.subValue.counter}
)会刷新。
let nextId = 1;
@Observed
class SubCounter {
counter: number;
constructor(c: number) {
this.counter = c;
}
}
@Observed
class ParentCounter {
id: number;
counter: number;
subCounter: SubCounter;
incrCounter() {
this.counter++;
}
incrSubCounter(c: number) {
this.subCounter.counter += c;
}
setSubCounter(c: number): void {
this.subCounter.counter = c;
}
constructor(c: number) {
this.id = nextId++;
**深知大多数程序员,想要提升技能,往往是自己摸索成长,但自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!**
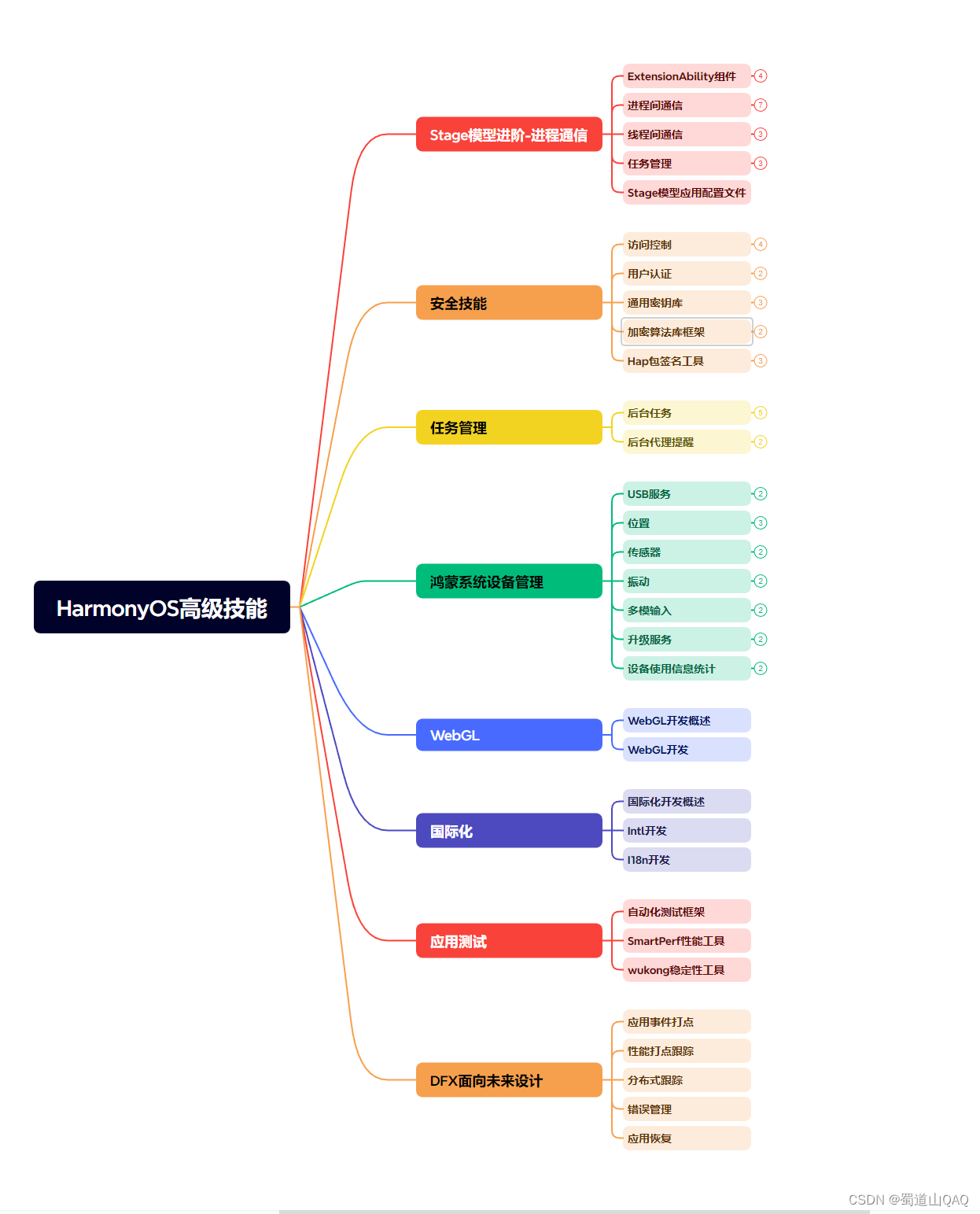
[外链图片转存中...(img-2B3rP7gj-1715813596341)]
[外链图片转存中...(img-ywZOos8O-1715813596341)]
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上鸿蒙开发知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新**
**[需要这份系统化的资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618636735)**