网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
将MP4转换为PCM
ffmpeg -i 1.mp4 -vn -ar 44100 -ac 1 -f s16le out.pcm
播放PCM
ffplay -ar 44100 -ac 1 -f s16le -i out.pcm
剪切mp4视频长度
ffmpeg -i ./SRS1.mp4 -vcodec copy -acodec copy -ss 00:10:0 -to 00:20:00 ./1.mp4 -y
音频编码的代码
int flush\_encoder(AVFormatContext \*fmt_ctx, unsigned int stream_index)
{
int ret;
int got_frame;
AVPacket enc_pkt;
if (!(fmt_ctx->streams[stream_index]->codec->codec->capabilities & AV_CODEC_CAP_DELAY))
return 0;
while (1) {
enc_pkt.data = NULL;
enc_pkt.size = 0;
av\_init\_packet(&enc_pkt);
ret = avcodec\_encode\_audio2(fmt_ctx->streams[stream_index]->codec, &enc_pkt,
NULL, &got_frame);
av\_frame\_free(NULL);
if (ret < 0)
break;
if (!got_frame) {
ret = 0;
break;
}
printf("Flush Encoder: Succeed to encode 1 frame!\tsize:%5d\n", enc_pkt.size);
/\* mux encoded frame \*/
ret = av\_write\_frame(fmt_ctx, &enc_pkt);
if (ret < 0)
break;
}
return ret;
}
int testAudio()
{
AVFormatContext\* pFormatCtx;
AVOutputFormat\* fmt;
AVStream\* audio_st;
AVCodecContext\* pCodecCtx;
AVCodec\* pCodec;
uint8\_t\* frame_buf;
AVFrame\* pFrame;
AVPacket pkt;
int got_frame = 0;
int ret = 0;
int size = 0;
FILE \*in_file = NULL; //Raw PCM data
int framenum = 8000; //Audio frame number
const char\* out_file = "test.mp3"; //Output URL
int i;
in_file = fopen("D://out.pcm", "rb");
av\_register\_all();
//Method 1.
pFormatCtx = avformat\_alloc\_context();
fmt = av\_guess\_format(NULL, out_file, NULL);
pFormatCtx->oformat = fmt;
//Method 2.
//avformat\_alloc\_output\_context2(&pFormatCtx, NULL, NULL, out\_file);
//fmt = pFormatCtx->oformat;
//Open output URL
if (avio\_open(&pFormatCtx->pb, out_file, AVIO_FLAG_READ_WRITE) < 0) {
printf("Failed to open output file!\n");
return -1;
}
audio_st = avformat\_new\_stream(pFormatCtx, 0);
if (audio_st == NULL) {
return -1;
}
pCodecCtx = audio_st->codec;
pCodecCtx->codec_id = fmt->audio_codec;
pCodecCtx->codec_type = AVMEDIA_TYPE_AUDIO;
pCodecCtx->sample_fmt = AV_SAMPLE_FMT_S16;
pCodecCtx->sample_rate = 44100;
pCodecCtx->channel_layout = av\_get\_default\_channel\_layout(1);
pCodecCtx->channels = av\_get\_channel\_layout\_nb\_channels(pCodecCtx->channel_layout);
pCodecCtx->bit_rate = 64000;
//pCodecCtx->frame\_size = 1024;
//Show some information
//av\_dump\_format(pFormatCtx, 0, out\_file, 1);
pCodec = avcodec\_find\_encoder(pCodecCtx->codec_id);
if (!pCodec) {
printf("Can not find encoder!\n");
return -1;
}
if (avcodec\_open2(pCodecCtx, pCodec, NULL) < 0) {
printf("Failed to open encoder!\n");
return -1;
}
pFrame = av\_frame\_alloc();
pFrame->nb_samples = pCodecCtx->frame_size;
pFrame->format = pCodecCtx->sample_fmt;
size = av\_samples\_get\_buffer\_size(NULL, pCodecCtx->channels, pCodecCtx->frame_size, pCodecCtx->sample_fmt, 1);
frame_buf = (uint8\_t \*)av\_malloc(size);
avcodec\_fill\_audio\_frame(pFrame, pCodecCtx->channels, pCodecCtx->sample_fmt, (const uint8\_t\*)frame_buf, size, 1);
//Write Header
avformat\_write\_header(pFormatCtx, NULL);
av\_new\_packet(&pkt, size);
for (i = 0; i<framenum; i++) {
//Read PCM
if (fread(frame_buf, 1, size, in_file) <= 0) {
printf("Failed to read raw data! \n");
return -1;
}
else if (feof(in_file)) {
break;
}
pFrame->data[0] = frame_buf; //PCM Data
pFrame->pts = i \* 100;
got_frame = 0;
//Encode
ret = avcodec\_encode\_audio2(pCodecCtx, &pkt, pFrame, &got_frame);
if (ret < 0) {
printf("Failed to encode!\n");
return -1;
}
if (got_frame == 1) {
printf("Succeed to encode 1 frame! \tsize:%5d\n", pkt.size);
pkt.stream_index = audio_st->index;
ret = av\_write\_frame(pFormatCtx, &pkt);
av\_free\_packet(&pkt);
}
}
//Flush Encoder
ret = flush\_encoder(pFormatCtx, 0);
if (ret < 0) {
printf("Flushing encoder failed\n");
return -1;
}
//Write Trailer
av\_write\_trailer(pFormatCtx);
//Clean
if (audio_st) {
avcodec\_close(audio_st->codec);
av\_free(pFrame);
av\_free(frame_buf);
}
avio\_close(pFormatCtx->pb);
avformat\_free\_context(pFormatCtx);
fclose(in_file);
return 0;
}
int main(int argc, char\* argv[])
{
testAudio();
return 0;
}
二、音频重采样转换
首先可以了解到重采样的作用,类似于视频的样式尺寸转换一样,音频主要是采样数nb_samples以及通道数channels和样式等参数的转换。
其实音频重采样主要是应用在只要PCM数据进行编码,但是如MP4编码都所支持的就是AV_SAMPLE_FMT_FLTP浮点数类型那么就可能存在转换。
下面的案例是以Linux下alsa库对pcm音频数据获取之后的格式为S16、双通道、44100的采样率进行编码。
要注意的点是;
1、要根据你对已知数据PCM的样式定义帧以及内存,便于后期将数据赋值给帧。从而达到将数据抽象为帧的关键操作。
定义模板帧
AVFrame \*input_frame = av\_frame\_alloc();
if (!input_frame)
{
ret = AVERROR(ENOMEM);
}
input_frame->nb_samples = 1024;
input_frame->channel_layout = AV_CH_LAYOUT_STEREO;
input_frame->format = AV_SAMPLE_FMT_S16;
input_frame->sample_rate = 44100;
input_frame->channels = 2;
int sizeIN = av\_samples\_get\_buffer\_size(NULL, input_frame->channels, input_frame->nb_samples, AV_SAMPLE_FMT_S16, 1);
uint8\_t \* frame_bufIN = (uint8\_t \*)av\_malloc(sizeIN);
avcodec\_fill\_audio\_frame(input_frame, input_frame->channels, AV_SAMPLE_FMT_S16, (const uint8\_t\*)frame_bufIN, sizeIN, 1);
将PCM数据与重采样输入模板帧关联
int readlen = fread(frame_bufIN, 1, sizeIN, in_file);
2、定义编码器上下文以及重采样的参数要一致,
pCodecCtx = audio_st->codec;
pCodecCtx->codec_id = fmt->audio_codec;
pCodecCtx->codec_type = AVMEDIA_TYPE_AUDIO;
pCodecCtx->sample_fmt = AV_SAMPLE_FMT_FLTP;
pCodecCtx->sample_rate= 44100;
pCodecCtx->channel_layout= AV_CH_LAYOUT_STEREO;
pCodecCtx->channels = 2;
pCodecCtx->bit_rate = 64000;
ret = AudioConvert(input_frame, AV_SAMPLE_FMT_FLTP, 2, 44100, &pOutFrame);
3、注意一些匹配关系
pCodecCtx->channels = 2;
pCodecCtx->channel_layout= AV_CH_LAYOUT_STEREO;匹配的,要么就使用API来指定
pCodecCtx->channel_layout = av\_get\_default\_channel\_layout(1);
pCodecCtx->channels = av\_get\_channel\_layout\_nb\_channels(pCodecCtx->channel_layout);
重采样编码代码
16位 44100采样率 AV_CH_LAYOUT_STEREO
转换为
AV_SAMPLE_FMT_FLTP 44100采样率 AV_CH_LAYOUT_STEREO
#include <stdio.h>
#define \_\_STDC\_CONSTANT\_MACROS
#ifdef \_WIN32
//Windows
extern "C"
{
#include "libavcodec/avcodec.h"
#include "libavformat/avformat.h"
#include "libswresample\swresample.h"
#include "libavutil\opt.h"
};
#else
//Linux...
#ifdef \_\_cplusplus
extern "C"
{
#endif
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#ifdef \_\_cplusplus
};
#endif
#endif
int flush\_encoder1(AVFormatContext \*fmt_ctx,unsigned int stream_index){
int ret;
int got_frame;
AVPacket enc_pkt;
if (!(fmt_ctx->streams[stream_index]->codec->codec->capabilities &
CODEC_CAP_DELAY))
return 0;
while (1) {
enc_pkt.data = NULL;
enc_pkt.size = 0;
av\_init\_packet(&enc_pkt);
ret = avcodec\_encode\_audio2 (fmt_ctx->streams[stream_index]->codec, &enc_pkt,
NULL, &got_frame);
av\_frame\_free(NULL);
if (ret < 0)
break;
if (!got_frame){
ret=0;
break;
}
printf("Flush Encoder: Succeed to encode 1 frame!\tsize:%5d\n",enc_pkt.size);
ret = av\_write\_frame(fmt_ctx, &enc_pkt);
if (ret < 0)
break;
}
return ret;
}
int32\_t AudioConvert(
const AVFrame\* pInFrame, // 输入音频帧
AVSampleFormat eOutSmplFmt, // 输出音频格式
int32\_t nOutChannels, // 输出音频通道数
int32\_t nOutSmplRate, // 输出音频采样率
AVFrame\*\* ppOutFrame) // 输出视频帧
{
struct SwrContext\* pSwrCtx = nullptr;
AVFrame\* pOutFrame = nullptr;
// 创建格式转换器,
int64\_t nInChnlLayout = av\_get\_default\_channel\_layout(pInFrame->channels);
int64\_t nOutChnlLayout = (nOutChannels == 1) ? AV_CH_LAYOUT_MONO : AV_CH_LAYOUT_STEREO;
pSwrCtx = swr\_alloc();
if (pSwrCtx == nullptr)
{
return -1;
}
swr\_alloc\_set\_opts(pSwrCtx,
nOutChnlLayout, eOutSmplFmt, nOutSmplRate, nInChnlLayout,
(enum AVSampleFormat)(pInFrame->format), pInFrame->sample_rate,
0, nullptr);
swr\_init(pSwrCtx);
// 计算重采样转换后的样本数量,从而分配缓冲区大小
int64\_t nCvtBufSamples = av\_rescale\_rnd(pInFrame->nb_samples, nOutSmplRate, pInFrame->sample_rate, AV_ROUND_UP);
// 创建输出音频帧
pOutFrame = av\_frame\_alloc();
pOutFrame->format = eOutSmplFmt;
pOutFrame->nb_samples = (int)nCvtBufSamples;
pOutFrame->channel_layout = (uint64\_t)nOutChnlLayout;
int res = av\_frame\_get\_buffer(pOutFrame, 0); // 分配缓冲区
if (res < 0)
{
swr\_free(&pSwrCtx);
av\_frame\_free(&pOutFrame);
return -2;
}
// 进行重采样转换处理,返回转换后的样本数量
int nCvtedSamples = swr\_convert(pSwrCtx,
const\_cast<uint8\_t\*\*>(pOutFrame->data),
(int)nCvtBufSamples,
const\_cast<const uint8\_t\*\*>(pInFrame->data),
pInFrame->nb_samples);
if (nCvtedSamples <= 0)
{
swr\_free(&pSwrCtx);
av\_frame\_free(&pOutFrame);
return -3;
}
pOutFrame->nb_samples = nCvtedSamples;
pOutFrame->pts = pInFrame->pts; // pts等时间戳沿用
pOutFrame->pkt_pts = pInFrame->pkt_pts;
(\*ppOutFrame) = pOutFrame;
swr\_free(&pSwrCtx); // 释放转换器
return 0;
}
int testAudioEncode()
{
AVFormatContext\* pFormatCtx;
AVOutputFormat\* fmt;
AVStream\* audio_st;
AVCodecContext\* pCodecCtx;
AVCodec\* pCodec;
AVPacket pkt;
int got_frame=0;
int ret=0;
int size=0;
FILE \*in_file=NULL; //Raw PCM data
int framenum=1000; //Audio frame number
const char\* out_file = "tdjm.aac"; //Output URL
int i;
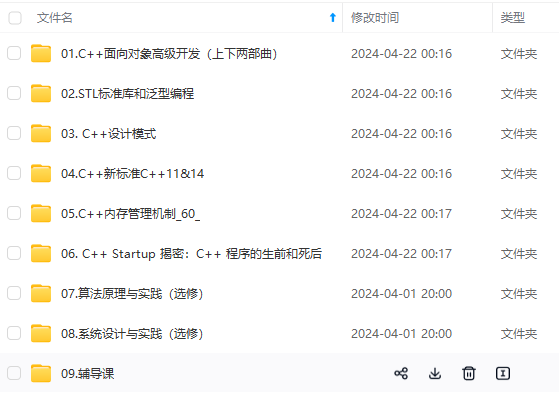
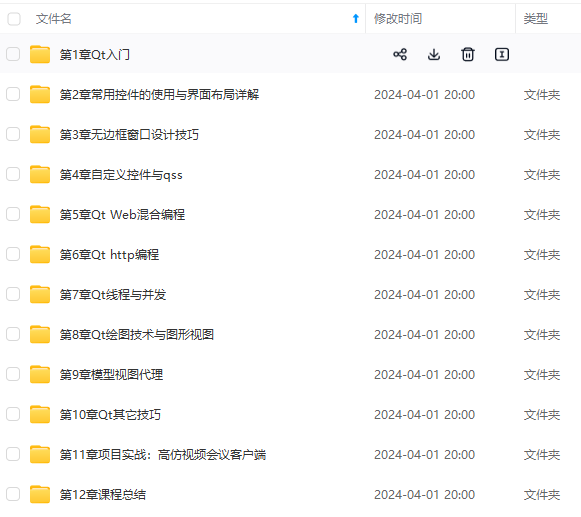
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化的资料的朋友,可以添加戳这里获取](https://bbs.csdn.net/topics/618668825)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**
Audio frame number
const char\* out_file = "tdjm.aac"; //Output URL
int i;
[外链图片转存中...(img-iYjzJLZ6-1715658043583)]
[外链图片转存中...(img-jioFT4rj-1715658043583)]
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化的资料的朋友,可以添加戳这里获取](https://bbs.csdn.net/topics/618668825)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**