既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上C C++开发知识点,真正体系化!
由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新
break;
}
else//如果不是雷
{
win++;
//统计mine数组中x,y坐标周围有几个雷
int count = get\_mine\_count(mine, x, y);
show[x][y] = count + '0';//转换成数字字符
DisplayBoard(show, ROW, COL);
}
}
}
else
{
printf("输入的坐标非法,请重新输入\n");
}
}
if (win == row \* col - EASY_COUNT)
{
printf("恭喜你,排雷成功\n");
DisplayBoard(mine, ROW, COL);
}
}
>
> 💎💎💎这里仅仅是简单的把选择的位置点击出来,不含任何技术,如果大家感兴趣的话,可能用递归实现点击实现一大片的功能
> 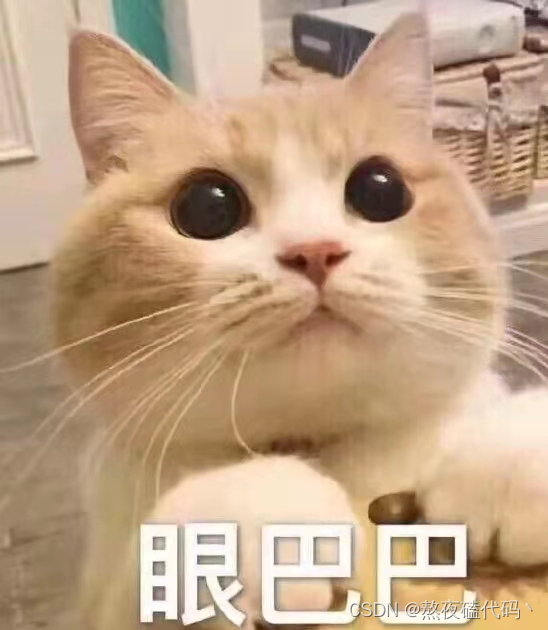
>
>
>
### 5.计算周围雷的个数
int get_mine_count(char board[ROWS][COLS], int x, int y)
{
return (board[x - 1][y] +
board[x - 1][y - 1] +
board[x][y - 1] +
board[x + 1][y - 1] +
board[x + 1][y] +
board[x + 1][y + 1] +
board[x][y + 1] +
board[x - 1][y + 1] - 8 * ‘0’);
}
---
## 三、test.c
#define _CRT_SECURE_NO_WARNINGS 1
#include"game.h"
void menu() {
printf(“********************\n”);
printf(“**** 1.play ******\n”);
printf(“**** 0.exit ******\n”);
printf(“********************\n”);
}
void game() {
//存放雷的信息
char mine[ROWS][COLS] = { 0 };
//存放排查出雷的信息
char show[ROWS][COLS] = { 0 };
//初始化数组内容为指定内容
//mine 数组在没有布置雷的时候都是’0’
InitBoard(mine, ROWS, COLS, ‘0’);
//show 数组在没有布置雷的时候都是’*’
InitBoard(show, ROWS, COLS, ‘*’);
//设置雷
SetMine(mine, ROW, COL);
DisplayBoard(show, ROW, COL);
//排查雷
FindMine(mine, show, ROW, COL);
}
int main() {
//设置随机数的起点
srand((unsigned int)time(NULL));
int input = 0;
do {
menu();
printf(“请选择:>”);
scanf(“%d”, &input);
switch (input) {
case 1:
game();
break;
case 0:
printf(“退出游戏\n”);
break;
default:
printf(“请重新选择\n”);
break;
}
} while (input);
return 0;
}
## 四、game.c
#define _CRT_SECURE_NO_WARNINGS 1
#include"game.h"
void InitBoard(char board[ROWS][COLS], int rows, int cols, char set) {
int x = 0;
int y = 0;
for (x = 0; x < rows; x++) {
for (y = 0; y < cols; y++) {
board[x][y] = set;
}
}
}
void DisplayBoard(char board[ROWS][COLS], int row, int col) {
int i = 0;
int j = 0;
printf(“------扫雷游戏------\n”);
for (j = 0; j <= col; j++) {
printf(“%d “, j);
}
printf(”\n”);
for (i = 1; i <= row; i++) {
printf(“%d “, i);
for (j = 1; j <= col; j++) {
printf(”%c “, board[i][j]);
}
printf(”\n”);
}
printf(“------扫雷游戏------\n”);
}
void SetMine(char board[ROWS][COLS], int row, int col)
{
int count = EASY_COUNT;
//1~9
//1~9
while (count)
{
int x = rand() % row + 1;
int y = rand() % col + 1;
if (board[x][y] == '0')
{
board[x][y] = '1';
count--;
}
}
}
int get_mine_count(char board[ROWS][COLS], int x, int y)
{
return (board[x - 1][y] +
board[x - 1][y - 1] +
board[x][y - 1] +
board[x + 1][y - 1] +
board[x + 1][y] +
board[x + 1][y + 1] +
board[x][y + 1] +
board[x - 1][y + 1] - 8 * ‘0’);
}
void FindMine(char mine[ROWS][COLS], char show[ROWS][COLS], int row, int col)
{
int x = 0;
int y = 0;
int win = 0;//找到非雷的个数
while (win < row * col - EASY_COUNT)
{
printf(“请输入要排查的坐标:>”);
scanf(“%d%d”, &x, &y);
if (x >= 1 && x <= row && y >= 1 && y <= col)
{
if (show[x][y] != ‘*’)
{
printf(“该坐标被排查过了,不能重复排查\n”);
}
else
{
//如果是雷
if (mine[x][y] == ‘1’)
{
printf(“很遗憾,你被炸死了\n”);
DisplayBoard(mine, ROW, COL);
break;
}
else//如果不是雷
{
win++;
//统计mine数组中x,y坐标周围有几个雷
int count = get_mine_count(mine, x, y);
show[x][y] = count + ‘0’;//转换成数字字符
DisplayBoard(show, ROW, COL);
}
}
}
else
{
printf(“输入的坐标非法,请重新输入\n”);
}
}
if (win == row * col - EASY_COUNT)
{
printf(“恭喜你,排雷成功\n”);
DisplayBoard(mine, ROW, COL);
}
}
## 五、game.h
#pragma once
#include<stdio.h>
#include<time.h>
#include<stdlib.h>
#define ROW 9
#define COL 9
#define ROWS ROW+2
#define COLS COL+2
#define EASY_COUNT 10
void InitBoard(char board[ROWS][COLS], int rows, int cols, char set);
void SetMine(char board[ROWS][COLS], int row, int col);
void DisplayBoard(char board[ROWS][COLS], int row, int col);
void FindMine(char mine[ROWS][COLS], char show[ROWS][COLS], int row, int col);
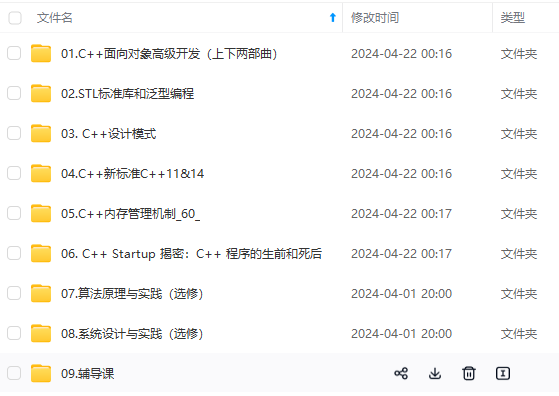
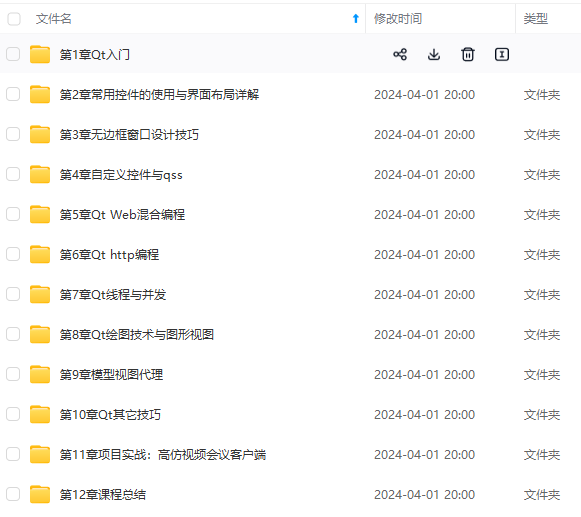
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化的资料的朋友,可以添加戳这里获取](https://bbs.csdn.net/topics/618668825)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**
...(img-klbsYuYp-1715729947359)]
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化的资料的朋友,可以添加戳这里获取](https://bbs.csdn.net/topics/618668825)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**