欢迎来到雲闪世界。面向 AI 学习者的 Python 快速入门(初学者)Python 已成为人工智能和数据科学事实上的编程语言。尽管存在无代码解决方案,但学习如何编码对于构建完全自定义的人工智能项目或产品仍然至关重要。在本文中,我分享了一份使用 Python 进行人工智能开发的初学者快速入门指南。我将介绍基础知识,然后分享一个带有代码的具体示例。
Python是一种编程语言,即一种向计算机发出精确指令来做我们不能或不想做的事情的方式。 在没有现成解决方案的情况下自动执行独特任务时,这非常方便。例如,如果我想自动编写和发送个性化的会议后续信息,我可以编写一个 Python 脚本来执行此操作。 有了 ChatGPT 这样的工具,我们很容易想象未来人们可以用简单的英语描述任何定制任务,计算机就会完成。然而,这样的消费产品目前还不存在。在这样的产品面市之前,了解(至少一点)Python 是非常有价值的。
编码比以往更加简单
虽然目前的人工智能产品(例如 ChatGPT、Claude、Gemini)还没有让编程过时(至少目前如此),但它们让学习编程变得比以往任何时候都更容易。我们现在都有一位能干、耐心的编程助手,随时可以帮助我们学习。 结合“传统”的谷歌搜索所有问题的方法,程序员现在可以更快地行动。例如,我慷慨地使用 ChatGPT 编写示例代码并解释错误消息。这加快了我的进度,并让我在探索新技术堆栈时更有信心。
适用对象
我写这篇文章时考虑到了一类特定的读者:那些试图进入人工智能领域并做过一些编码(例如 JS、HTML/CSS、PHP、Java、SQL、Bash/Powershell、VBA)但对 Python 还不熟悉的人。 我将从 Python 基础知识开始,然后分享一个简单的 AI 项目的示例代码。这并不是对 Python 的全面介绍。相反,它旨在为您提供足够的知识,让您能够快速使用 Python 编写您的第一个 AI 项目。 关于我 — 我是一名数据科学家和自学成才的 Python 程序员(5 年)。虽然我还有很多关于软件开发的知识需要学习,但根据我的个人经验,我在这里介绍了我认为 Python 对于 AI/数据科学项目的基本要求。
安装 Python
许多计算机都预装了 Python。要查看您的计算机是否已安装 Python,请转到终端 (Mac/Linux) 或命令提示符 (Windows),然后输入“python”。
在终端中使用 Python
如果您没有看到这样的屏幕,您可以手动下载 Python(Windows / Mac)。或者,您可以安装Anaconda,这是一个流行的 Python 人工智能和数据科学软件包系统。如果您遇到安装问题,请向您最喜欢的人工智能助手寻求帮助!
Python 运行后,我们现在可以开始编写一些代码了。我建议你在计算机上运行示例。你也可以从GitHub repo下载所有示例代码。
1)数据类型
字符串和数字
数据类型(或简称“类型”)是一种对数据进行分类的方法,以便计算机能够适当、有效地处理数据。
类型由一组可能的值和操作定义。例如,字符串是可以以特定方式操作的任意字符序列(即文本)。在命令行 Python 实例中尝试以下字符串。
"this is a string"
>> 'this is a string'
'so is this:-1*!@&04"(*&^}":>?'
>> 'so is this:-1*!@&04"(*&^}":>?'
"""and
this is
too!!11!"""
>> 'and\n this is\n too!!11!'
"we can even " + "add strings together"
>> 'we can even add strings together'
尽管字符串可以相加(即连接),但它们不能添加到数字数据类型,如int(即整数)或float(即带小数的数字)。如果我们在 Python 中尝试这样做,我们将收到一条错误消息,因为操作仅针对兼容类型定义。
# we can't add strings to other data types (BTW this is how you write comments in Python)
"I am " + 29
>> TypeError: can only concatenate str (not "int") to str
# so we have to write 29 as a string
"I am " + "29"
>> 'I am 29'
列表和词典
除了字符串、整数和浮点数等基本类型之外,Python 还具有用于构建更大数据集合的类型。
列表就是这样一种类型,即值的有序集合。我们可以有字符串列表、数字列表、字符串+数字列表,甚至是列表的列表。
# a list of strings
["a", "b", "c"]
# a list of ints
[1, 2, 3]
# list with a string, int, and float
["a", 2, 3.14]
# a list of lists
[["a", "b"], [1, 2], [1.0, 2.0]]
另一种核心数据类型是字典,它由键值对序列组成,其中键是字符串,值可以是任何数据类型。这是一种表示具有多个属性的数据的好方法。
# a dictionary
{"Name":"Shaw"}
# a dictionary with multiple key-value pairs
{"Name":"Shaw", "Age":29, "Interests":["AI", "Music", "Bread"]}
# a list of dictionaries
[{"Name":"Shaw", "Age":29, "Interests":["AI", "Music", "Bread"]},
{"Name":"Ify", "Age":27, "Interests":["Marketing", "YouTube", "Shopping"]}]
# a nested dictionary
{"User":{"Name":"Shaw", "Age":29, "Interests":["AI", "Music", "Bread"]},
"Last_login":"2024-09-06",
"Membership_Tier":"Free"}
2)变量
到目前为止,我们已经了解了一些基本的 Python 数据类型和操作。然而,我们仍然缺少一个基本功能:变量。
变量提供了底层数据类型实例的抽象表示。例如,我可以创建一个名为 user_name 的变量,它表示一个包含我的名字“Shaw”的字符串。这使我们能够编写不受特定值限制的灵活程序。
# creating a variable and printing it
user_name = "Shaw"
print(user_name)
#>> Shaw
我们可以对其他数据类型(例如整数和列表)执行同样的事情。
# defining more variables and printing them as a formatted string.
user_age = 29
user_interests = ["AI", "Music", "Bread"]
print(f"{user_name} is {user_age} years old. His interests include {user_interests}.")
#>> Shaw is 29 years old. His interests include ['AI', 'Music', 'Bread'].
3)创建脚本
现在我们的示例代码片段越来越长了,让我们看看如何创建我们的第一个脚本。这就是我们如何从命令行编写和执行更复杂的程序。
为此,请在您的计算机上创建一个新文件夹。我将我的文件夹命名为python-quickstart。如果您有喜欢的IDE(例如,集成开发环境),请使用它打开这个新文件夹并创建一个新的 Python 文件,例如,my-script.py。在那里,我们可以编写正式的“Hello, world”程序。
# ceremonial first program
print("Hello, world!")
如果您没有 IDE(不推荐),您可以使用基本的文本编辑器(例如 Apple 的 Text Edit、Windows 的 Notepad)。在这些情况下,您可以打开文本编辑器并使用 .py 扩展名(而不是 .txt)保存新文本文件。 注意:如果您在 Mac 上使用 TextEditor,您可能需要通过“格式”>“制作纯文本”将应用程序置于纯文本模式。
然后,我们可以使用终端(Mac/Linux)或命令提示符(Windows)运行此脚本,方法是导航到包含新 Python 文件的文件夹并运行以下命令。
python my-script.py
恭喜!您已运行第一个 Python 脚本。您可以随意扩展此程序,方法是复制粘贴后面的代码示例并重新运行脚本以查看其输出。
4)循环和条件
Python(或任何其他编程语言)的两个基本功能是循环和条件。
循环允许我们多次运行一段特定的代码。最常用的是for 循环,它在迭代变量时运行相同的代码。
# a simple for loop iterating over a sequence of numbers
for i in range(5):
print(i) # print ith element
# for loop iterating over a list
user_interests = ["AI", "Music", "Bread"]
for interest in user_interests:
print(interest) # print each item in list
# for loop iterating over items in a dictionary
user_dict = {"Name":"Shaw", "Age":29, "Interests":["AI", "Music", "Bread"]}
for key in user_dict.keys():
print(key, "=", user_dict[key]) # print each key and corresponding value
另一个核心功能是条件,例如 if-else 语句,它使我们能够编写逻辑。例如,我们可能想检查用户是否是成年人或评估他们的智慧。
# check if user is 18 or older
if user_dict["Age"] >= 18:
print("User is an adult")
# check if user is 1000 or older, if not print they have much to learn
if user_dict["Age"] >= 1000:
print("User is wise")
else:
print("User has much to learn")
通常在 for 循环中使用条件来根据特定条件应用不同的操作,例如计算对面包感兴趣的用户数量。
# count the number of users interested in bread
user_list = [{"Name":"Shaw", "Age":29, "Interests":["AI", "Music", "Bread"]},
{"Name":"Ify", "Age":27, "Interests":["Marketing", "YouTube", "Shopping"]}]
count = 0 # intialize count
for user in user_list:
if "Bread" in user["Interests"]:
count = count + 1 # update count
print(count, "user(s) interested in Bread")
5)功能
函数是我们可以对特定数据类型执行的操作。
我们已经看到了一个基本函数print(),它针对任何数据类型进行定义。不过,还有一些其他有用的函数值得了解。
# print(), a function we've used several times already
for key in user_dict.keys():
print(key, ":", user_dict[key])
# type(), getting the data type of a variable
for key in user_dict.keys():
print(key, ":", type(user_dict[key]))
# len(), getting the length of a variable
for key in user_dict.keys():
print(key, ":", len(user_dict[key]))
# TypeError: object of type 'int' has no len()
我们看到,与print()和type()不同,len()并非针对所有数据类型定义,因此当应用于 int 时会引发错误。还有其他几个类似类型的特定函数。
# string methods
# --------------
# make string all lowercase
print(user_dict["Name"].lower())
# make string all uppercase
print(user_dict["Name"].upper())
# split string into list based on a specific character sequence
print(user_dict["Name"].split("ha"))
# replace a character sequence with another
print(user_dict["Name"].replace("w", "whin"))
# list methods
# ------------
# add an element to the end of a list
user_dict["Interests"].append("Entrepreneurship")
print(user_dict["Interests"])
# remove a specific element from a list
user_dict["Interests"].pop(0)
print(user_dict["Interests"])
# insert an element into a specific place in a list
user_dict["Interests"].insert(1, "AI")
print(user_dict["Interests"])
# dict methods
# ------------
# accessing dict keys
print(user_dict.keys())
# accessing dict values
print(user_dict.values())
# accessing dict items
print(user_dict.items())
# removing a key
user_dict.pop("Name")
print(user_dict.items())
# adding a key
user_dict["Name"] = "Shaw"
print(user_dict.items())
虽然核心 Python 函数很有用,但真正的强大之处在于创建用户定义函数来执行自定义操作。此外,自定义函数允许我们编写更简洁的代码。例如,以下是一些重新打包为用户定义函数的先前代码片段。
# define a custom function
def user_description(user_dict):
"""
Function to return a sentence (string) describing input user
"""
return f'{user_dict["Name"]} is {user_dict["Age"]} years old and is interested in {user_dict["Interests"][0]}.'
# print user description
description = user_description(user_dict)
print(description)
# print description for a new user!
new_user_dict = {"Name":"Ify", "Age":27, "Interests":["Marketing", "YouTube", "Shopping"]}
print(user_description(new_user_dict))
# define another custom function
def interested_user_count(user_list, topic):
"""
Function to count number of users interested in an arbitrary topic
"""
count = 0
for user in user_list:
if topic in user["Interests"]:
count = count + 1
return count
# define user list and topic
user_list = [user_dict, new_user_dict]
topic = "Shopping"
# compute interested user count and print it
count = interested_user_count(user_list, topic)
print(f"{count} user(s) interested in {topic}")
6)库、pip 和 venv
虽然我们可以使用核心 Python 实现任意程序,但对于某些用例来说,这可能非常耗时。Python 的主要优势之一是其活跃的开发者社区和强大的软件包生态系统。几乎所有你想用核心 Python 实现的东西(可能)都已经作为开源库存在了。
我们可以使用Python 的原生包管理器 pip安装此类包。要安装新包,我们从命令行运行 pip 命令。下面介绍如何安装numpy,这是一个实现基本数学对象和运算的基本数据科学库。
pip install numpy
安装numpy后,我们可以将其导入到新的Python脚本中并使用它的一些数据类型和函数。
import numpy as np
# create a "vector"
v = np.array([1, 3, 6])
print(v)
# multiply a "vector"
print(2*v)
# create a matrix
X = np.array([v, 2*v, v/2])
print(X)
# matrix multiplication
print(X*v)
前面的 pip 命令将 numpy 添加到了我们的基础 Python 环境中。或者,创建所谓的虚拟环境是一种最佳实践。这些是Python 库的集合,可轻松用于不同的项目。
以下是创建名为my-env的新虚拟环境的方法。
python -m venv my-env
我的环境 然后,我们就可以激活它。
# mac/linux
source my-env/bin/activate
# windows
.\my-env\Scripts\activate.bat
最后,我们可以使用 pip 安装新的库,例如 numpy。
pip install pip
注意:如果您使用的是 Anaconda,请查看此便捷的备忘单以创建新的 conda 环境。 人工智能和数据科学中还常用其他几个库。以下是对构建人工智能项目的一些有用库的不全面概述。
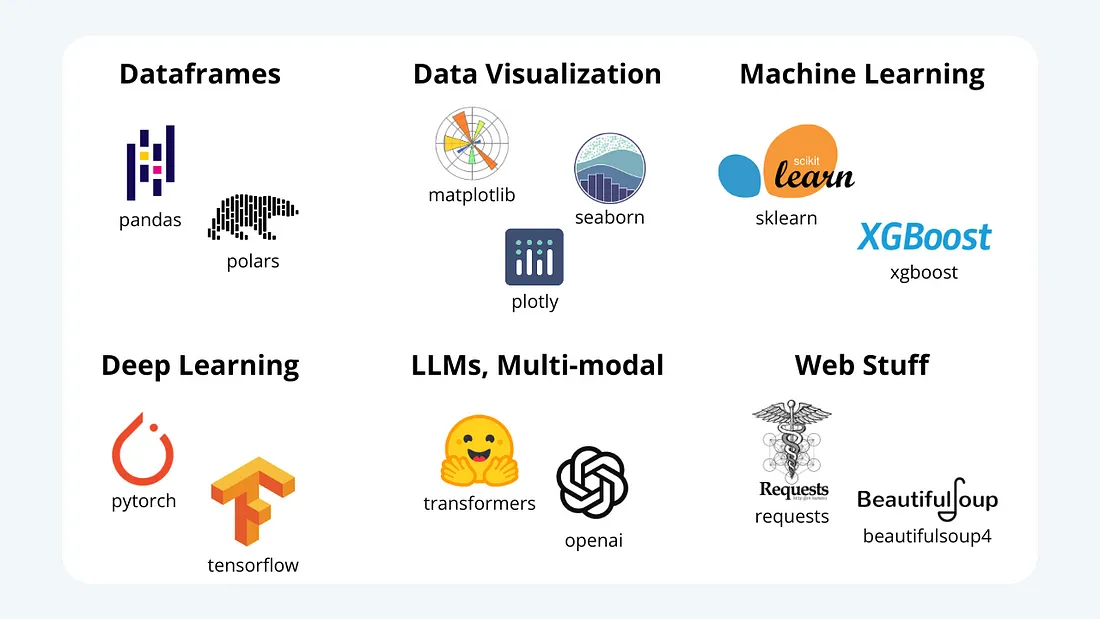
示例代码:从研究论文中提取摘要和关键词
现在我们已经了解了 Python 的基础知识,让我们看看如何使用它来实现一个简单的 AI 项目。在这里,我将使用 OpenAI API 创建一个研究论文摘要器和关键字提取器。
与本指南中的所有其他代码片段一样,示例代码可在GitHub 存储库中找到。
安装依赖项
我们首先安装一些有用的库。您可以使用我们之前创建的相同my-env环境,也可以创建一个新的环境。然后,您可以使用来自 {GitHub repo} 的requirements.txt文件安装所有必需的软件包。
pip 安装 -r 要求.txt txt
这行代码扫描requirements.txt中列出的每个库并安装每个库。
导入
接下来,我们可以创建一个新的 Python 脚本并导入所需的库。
import fitz # PyMuPDF
import openai
import sys
接下来,要使用 OpenAI 的 Python API,我们需要导入 AI 密钥。以下是其中一种方法。
from sk import my_sk
# Set up your OpenAI API key
openai.api_key = my_sk
请注意,sk不是 Python 库。相反,它是一个单独的 Python 脚本,定义了一个变量my_sk,该变量是一个由我的 OpenAI API 密钥组成的字符串,即允许使用 OpenAI API 的唯一(和秘密)令牌。
阅读 PDF
接下来,我们将创建一个函数,根据保存为 .pdf 文件的研究论文的路径,从论文中提取摘要。
# Function to read the first page of a PDF and extract the abstract
def extract_abstract(pdf_path):
# Open the PDF file and grab text from the 1st page
with fitz.open(pdf_path) as pdf:
first_page = pdf[0]
text = first_page.get_text("text")
# Extract the abstract (assuming the abstract starts with 'Abstract')
# find where abstract starts
start_idx = text.lower().find('abstract')
# end abstract at introduction if it exists on 1st page
if 'introduction' in text.lower():
end_idx = text.lower().find('introduction')
else:
end_idx = None
# extract abstract text
abstract = text[start_idx:end_idx].strip()
# if abstract appears on 1st page return it, if not resturn None
if start_idx != -1:
abstract = text[start_idx:end_idx].strip()
return abstract
else:
return None
用 LLM 进行总结
现在我们有了摘要文本,我们可以使用 LLM 来总结它并生成关键字。在这里,我定义了一个函数来将摘要传递给 OpenAI 的 GPT-4o-mini 模型来执行此操作。
# Function to summarize the abstract and generate keywords using OpenAI API
def summarize_and_generate_keywords(abstract):
# Use OpenAI Chat Completions API to summarize and generate keywords
prompt = f"Summarize the following paper abstract and generate (no more than 5) keywords:\n\n{abstract}"
# make api call
response = openai.chat.completions.create(
model="gpt-4o-mini",
messages=[
{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": prompt}
],
temperature = 0.25
)
# extract response
summary = response.choices[0].message.content
return summary
整合在一起
最后,我们可以使用用户定义的函数为从命令行传递给程序的任何研究论文生成摘要和关键字。
# Get the PDF path from the command-line arguments
pdf_path = sys.argv[1]
# Extract abstract from the PDF
abstract = extract_abstract(pdf_path)
# if abstract exists on first page, print summary.
if abstract:
# Summarize and generate keywords
summary = summarize_and_generate_keywords(abstract)
print(summary)
else:
print("Abstract not found on the first page.")
然后我们可以从命令行执行我们的程序。
python summarize-paper.py "files/attention-is-all-you-need.pdf""files/attention-is-all-you-need.pdf"
成果:
本文介绍了 Transformer,这是一种用于序列
传导任务的新型网络架构,它完全依赖于注意力机制,无需
循环和卷积结构。Transformer
在机器翻译任务中表现出色,在 WMT 2014 英语到德语翻译中获得了 28.4 的 BLEU 分数,在英语到法语翻译任务中获得了 41.8 的
最佳分数,同时在训练时间上也更高效。此外,Transformer 还展示了多功能性,成功应用于具有不同数量训练数据的英语成分解析。**关键词**:Transformer、注意力机制、机器翻译、BLEU 分数、神经网络。
感谢关注雲闪世界。(Aws解决方案架构师vs开发人员&GCP解决方案架构师vs开发人员)