我们选择上一篇文章版本的示例代码来改造, MQ 我们使用 RabbitMQ 来做示例。
服务端
POM 配置
在 pom.xml 里添加,这 4 个是必须的
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-config-server</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-bus</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-stream-binder-rabbit</artifactId>
</dependency>
配置文件
application.yml 内容如下
spring:
application:
name: config-server
cloud:
config:
server:
git:
uri: https://gitee.com/monkeyshow/yujian2020 # 配置git仓库的地址
search-paths: '4.springcloud/code/spring-cloud-2020/config-repo' # git仓库地址下的相对地址,可以配置多个,用,分割。
# 启用bus
bus:
enabled: true
trace:
enabled: true
server:
port: 12000
eureka:
client:
service-url:
defaultZone: http://localhost:7000/eureka/
management:
endpoints:
web:
exposure:
include: bus-refresh
启动类
加@EnableConfigServer
注解
@SpringBootApplication
@EnableConfigServer
public class ConfigServerApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigServerApplication.class, args);
}
}
客户端
POM 配置
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-bus</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-stream-binder-rabbit</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
配置文件
还是分为两个,分别如下
application.yml
spring:
application:
name: config-client-git
cloud:
bus:
trace:
enabled: true
enabled: true
server:
port: 13000
management:
endpoints:
web:
exposure:
include: refresh
bootstrap.yml
spring:
cloud:
config:
# uri: http://localhost:12000 # 配置中心的具体地址,即 config-server
name: config-client # 对应 {application} 部分
profile: dev # 对应 {profile} 部分
label: master # 对应 {label} 部分,即 Git 的分支。如果配置中心使用的是本地存储,则该参数无用
discovery:
enabled: true
service-id: config-server
eureka:
client:
service-url:
defaultZone: http://localhost:7000/eureka/
Controller
@RestController
@RefreshScope
public class HelloController {
@Value("${hello.yujian}")
private String name;
@GetMapping("/info")
public String hello() {
return name;
}
}
@RefreshScope
必须加,否则客户端会受到服务端的更新消息,但是更新不了,因为不知道更新哪里的。
至于启动主类,用默认生成的不用改,就不贴了。
测试
分别启动 eureka、config-server 和两个 config-client
## 打包
./mvnw clean package -Dmaven.test.skip=true
## 运行两个 client
java -jar target/spring-cloud-config-client-0.0.1-SNAPSHOT.jar --server.port=13000
java -jar target/spring-cloud-config-client-0.0.1-SNAPSHOT.jar --server.port=13001
启动后,RabbitMQ 中会自动创建一个 topic 类型的 Exchange 和两个以springCloudBus.anonymous.
开头的匿名 Queue
我们访问 http://localhost:13000/info 和 http://localhost:13001/info 返回内容的都是dev
。
将 Git 中的配置信息由dev
改为dev bus
,并执行
curl -X POST http://localhost:12000/actuator/bus-refresh/
再次访问 http://localhost:13000/info 和 http://localhost:13001/info 这时返回内容就是修改之后的dev bus
了,说明成功了。
服务端在刷新接口产生的的日志:
## 最后
既已说到spring cloud alibaba,那对于整个微服务架构,如果想要进一步地向上提升自己,到底应该掌握哪些核心技能呢?
就个人而言,对于整个微服务架构,像RPC、Dubbo、Spring Boot、Spring Cloud Alibaba、Docker、kubernetes、Spring Cloud Netflix、Service Mesh等这些都是最最核心的知识,架构师必经之路!下图,是自绘的微服务架构路线体系大纲,如果有还不知道自己该掌握些啥技术的朋友,可根据小编手绘的大纲进行一个参考。
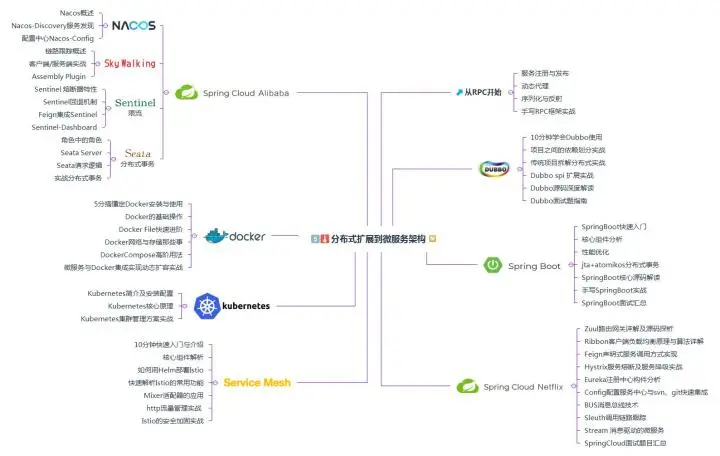
如果觉得图片不够清晰,也可来找小编分享原件的xmind文档!
且除此份微服务体系大纲外,我也有整理与其每个专题核心知识点对应的最强学习笔记:
* 出神入化——SpringCloudAlibaba.pdf
* SpringCloud微服务架构笔记(一).pdf
* SpringCloud微服务架构笔记(二).pdf
* SpringCloud微服务架构笔记(三).pdf
* SpringCloud微服务架构笔记(四).pdf
* Dubbo框架RPC实现原理.pdf
* Dubbo最新全面深度解读.pdf
* Spring Boot学习教程.pdf
* SpringBoo核心宝典.pdf
* 第一本Docker书-完整版.pdf
* 使用SpringCloud和Docker实战微服务.pdf
* K8S(kubernetes)学习指南.pdf
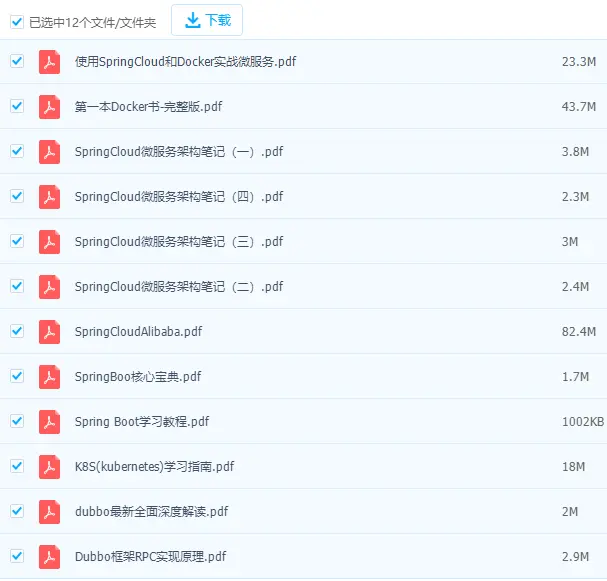
另外,如果不知道从何下手开始学习呢,小编这边也有对每个微服务的核心知识点手绘了其对应的知识架构体系大纲,不过全是导出的xmind文件,全部的源文件也都在此!
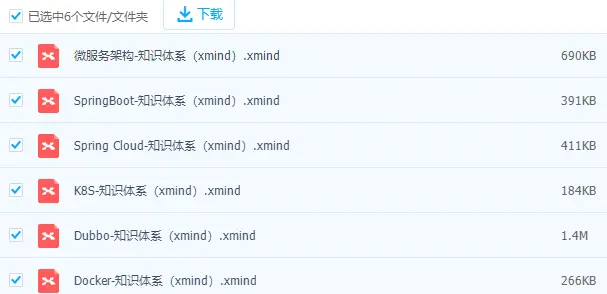
* SpringBoo核心宝典.pdf
* 第一本Docker书-完整版.pdf
* 使用SpringCloud和Docker实战微服务.pdf
* K8S(kubernetes)学习指南.pdf
[外链图片转存中...(img-ewlydcYs-1721837059882)]
另外,如果不知道从何下手开始学习呢,小编这边也有对每个微服务的核心知识点手绘了其对应的知识架构体系大纲,不过全是导出的xmind文件,全部的源文件也都在此!
[外链图片转存中...(img-o6Lc0zWc-1721837059882)]