/\*\* Returns whether the stack is empty. \*/
bool empty() {
return queue1.empty();
}
};
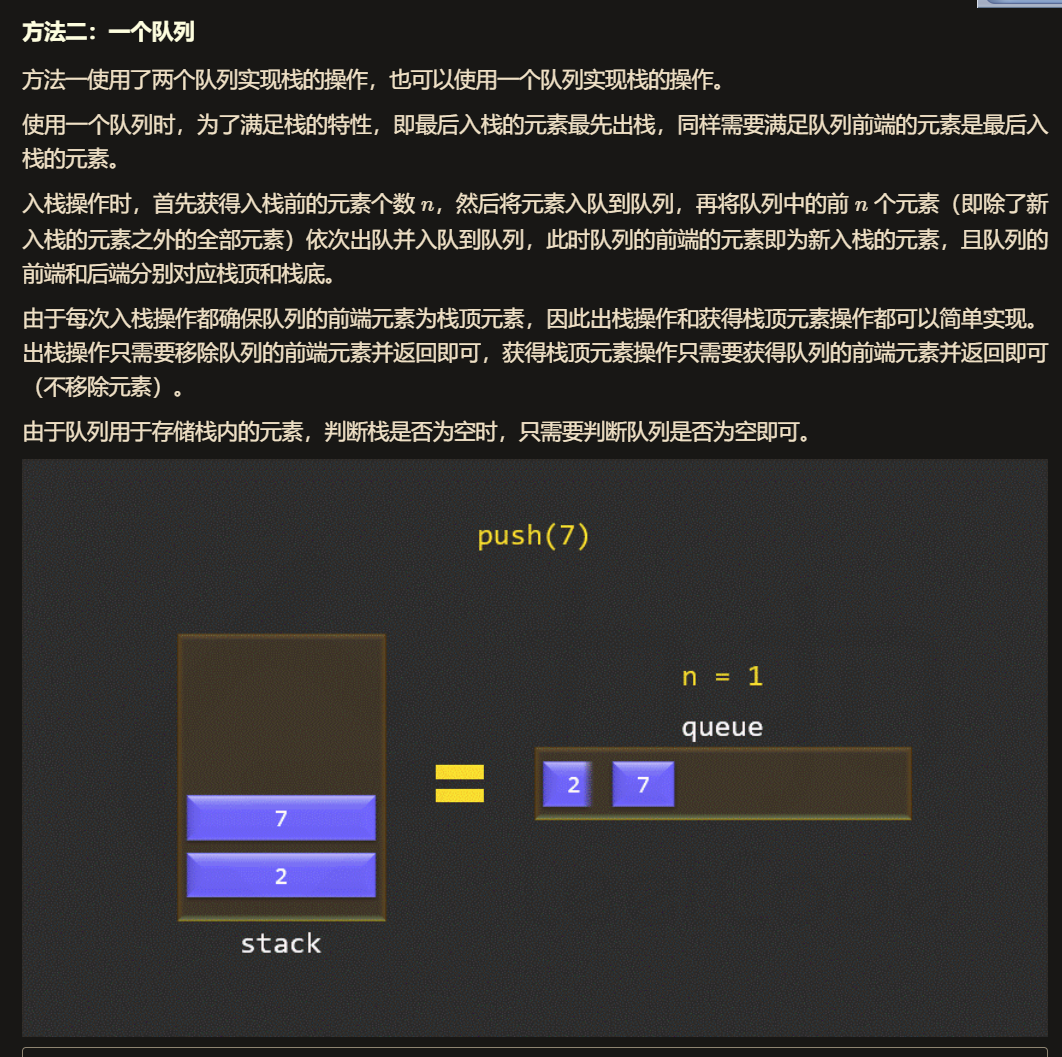
typedef struct tagListNode {
struct tagListNode* next;
int val;
} ListNode;
typedef struct {
ListNode* top;
} MyStack;
MyStack* myStackCreate() {
MyStack* stk = calloc(1, sizeof(MyStack));
return stk;
}
void myStackPush(MyStack* obj, int x) {
ListNode* node = malloc(sizeof(ListNode));
node->val = x;
node->next = obj->top;
obj->top = node;
}
int myStackPop(MyStack* obj) {
ListNode* node = obj->top;
int val = node->val;
obj->top = node->next;
free(node);
return val;
}
int myStackTop(MyStack* obj) {
return obj->top->val;
}
bool myStackEmpty(MyStack* obj) {
return (obj->top == NULL);
}
void myStackFree(MyStack* obj) {
while (obj->top != NULL) {
ListNode* node = obj->top;
obj->top = obj->top->next;
free(node);
}
free(obj);
}
class MyStack {
public:
queue q;
/\*\* Initialize your data structure here. \*/
MyStack() {
}
/\*\* Push element x onto stack. \*/
void push(int x) {
int n = q.size();
q.push(x);
for (int i = 0; i < n; i++) {
q.push(q.front());
q.pop();
}
}
/\*\* Removes the element on top of the stack and returns that element. \*/
int pop() {
int r = q.front();
q.pop();
return r;
}
/\*\* Get the top element. \*/
int top() {
int r = q.front();
return r;
}
/\*\* Returns whether the stack is empty. \*/
bool empty() {
return q.empty();
}
};
### [42. 接雨水]( )
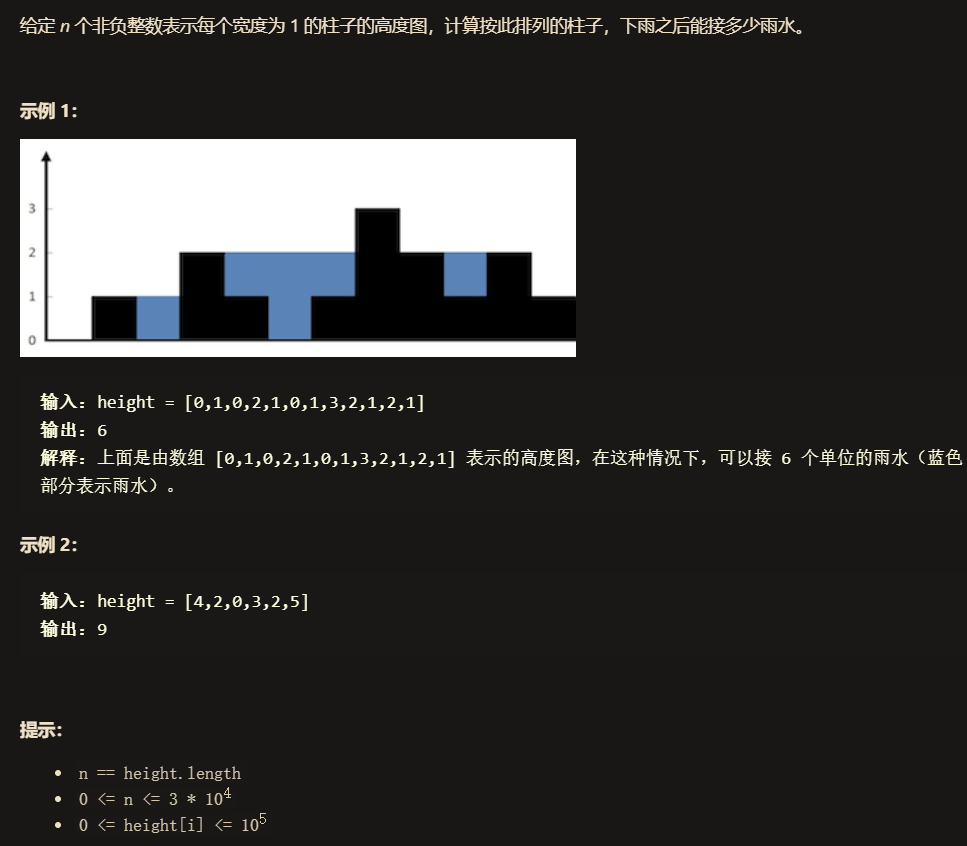
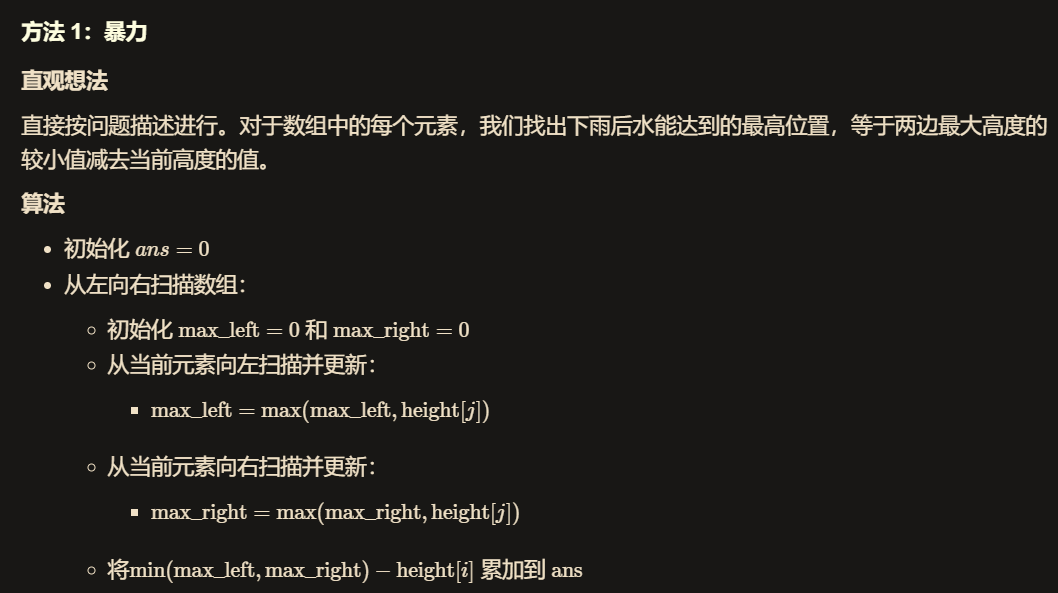
int trap(vector& height)
{
int ans = 0;
int size = height.size();
for (int i = 1; i < size - 1; i++) {
int max_left = 0, max_right = 0;
for (int j = i; j >= 0; j–) { //Search the left part for max bar size
max_left = max(max_left, height[j]);
}
for (int j = i; j < size; j++) { //Search the right part for max bar size
max_right = max(max_right, height[j]);
}
ans += min(max_left, max_right) - height[i];
}
return ans;
}

int trap(vector& height)
{
if (height == null)
return 0;
int ans = 0;
int size = height.size();
vector left_max(size), right_max(size);
left_max[0] = height[0];
for (int i = 1; i < size; i++) {
left_max[i] = max(height[i], left_max[i - 1]);
}
right_max[size - 1] = height[size - 1];
for (int i = size - 2; i >= 0; i–) {
right_max[i] = max(height[i], right_max[i + 1]);
}
for (int i = 1; i < size - 1; i++) {
ans += min(left_max[i], right_max[i]) - height[i];
}
return ans;
}
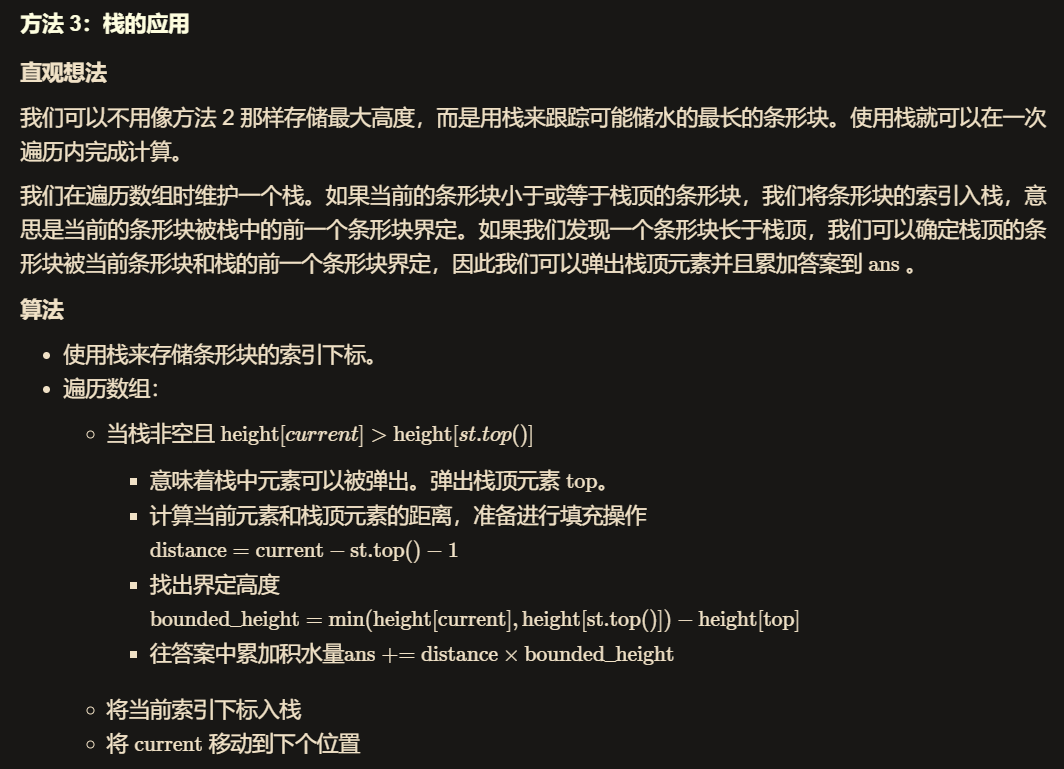
int trap(vector& height)
{
int ans = 0, current = 0;
stack st;
while (current < height.size()) {
while (!st.empty() && height[current] > height[st.top()]) {
int top = st.top();
st.pop();
if (st.empty())
break;
int distance = current - st.top() - 1;
int bounded_height = min(height[current], height[st.top()]) - height[top];
ans += distance * bounded_height;
}
st.push(current++);
}
return ans;
}
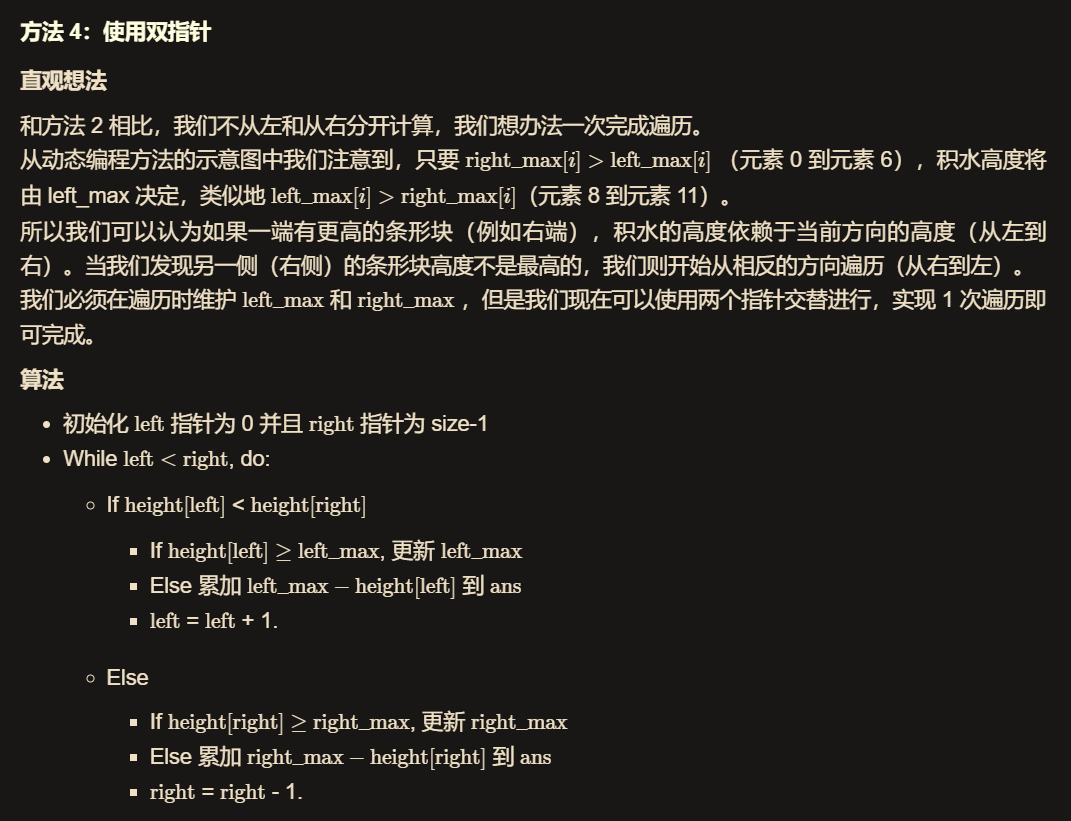
int trap(vector& height)
{
int left = 0, right = height.size() - 1;
int ans = 0;
int left_max = 0, right_max = 0;
while (left < right) {
if (height[left] < height[right]) {
height[left] >= left_max ? (left_max = height[left]) : ans += (left_max - height[left]);
++left;
}
else {
height[right] >= right_max ? (right_max = height[right]) : ans += (right_max - height[right]);
–right;
}
}
return ans;
}
### [剑指 Offer 06. 从尾到头打印链表]( )
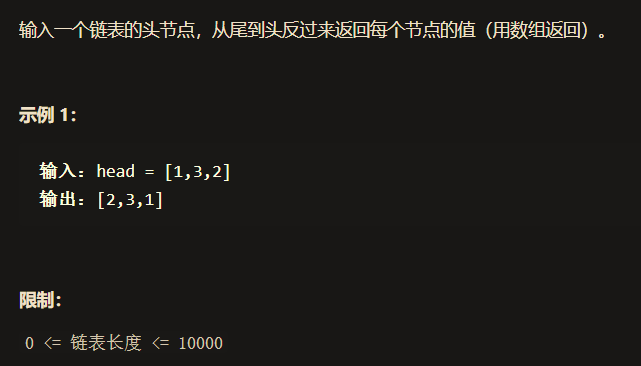
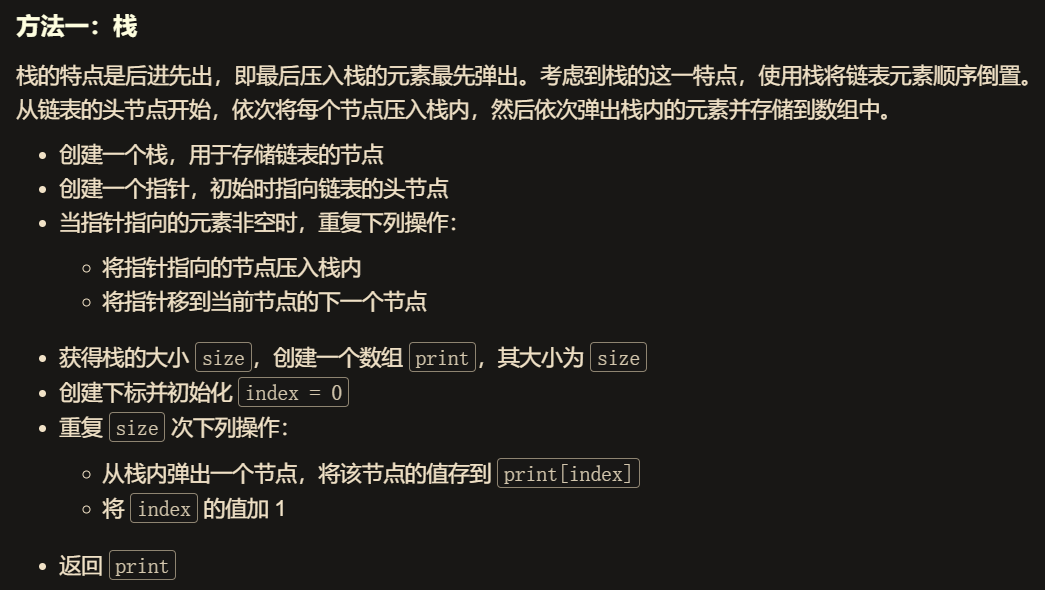
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
vector reversePrint(ListNode* head) {
stack s;
vector res;
ListNode* pre=head;
while(pre){
s.push(pre->val);
pre=pre->next;
}
while(!s.empty()){
res.push_back(s.top());
s.pop();
}
return res;
}
};
### [剑指 Offer 09. 用两个栈实现队列]( )
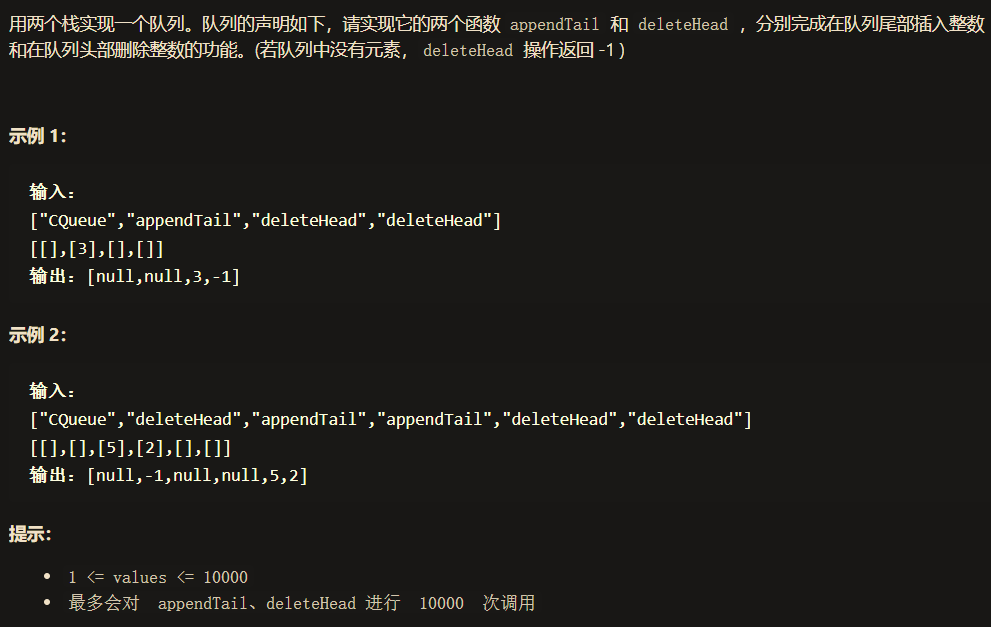
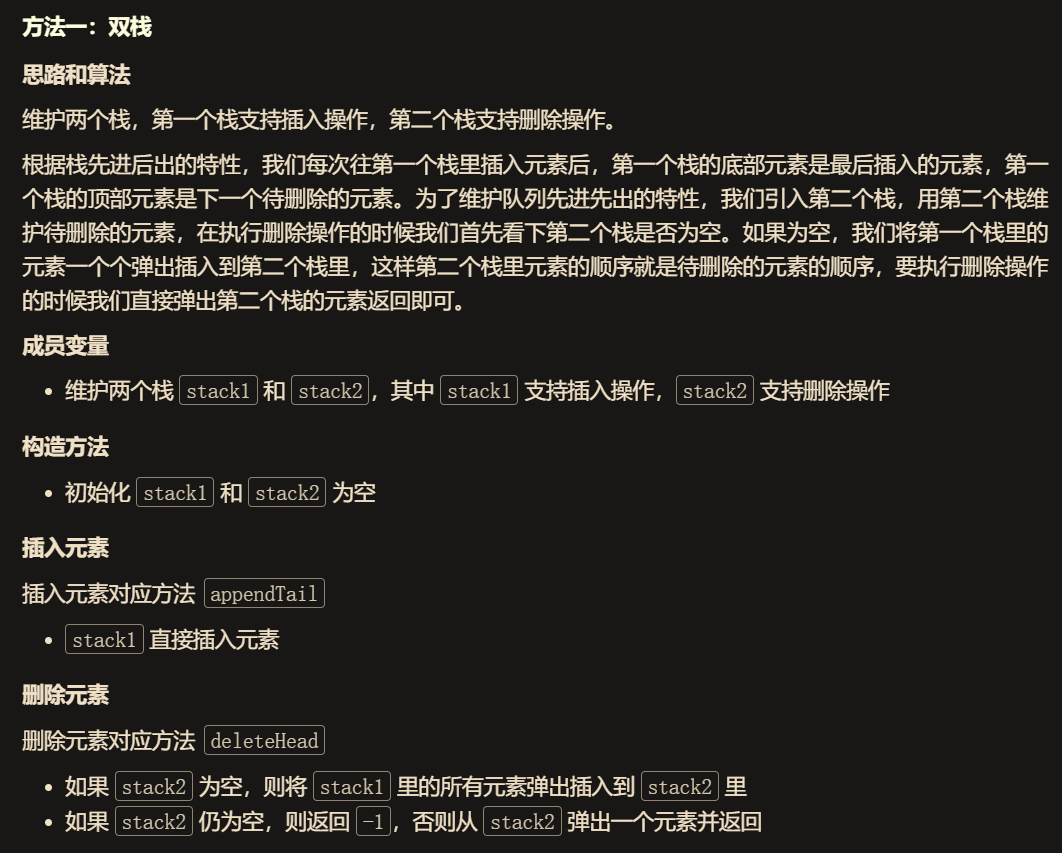
class CQueue {
stack stack1,stack2;
public:
CQueue() {
while (!stack1.empty()) {
stack1.pop();
}
while (!stack2.empty()) {
stack2.pop();
}
}
void appendTail(int value) {
stack1.push(value);
}
int deleteHead() {
// 如果第二个栈为空
if (stack2.empty()) {
while (!stack1.empty()) {
stack2.push(stack1.top());
stack1.pop();
}
}
if (stack2.empty()) {
return -1;
} else {
int deleteItem = stack2.top();
stack2.pop();
return deleteItem;
}
}
};
### [155. 最小栈]( )
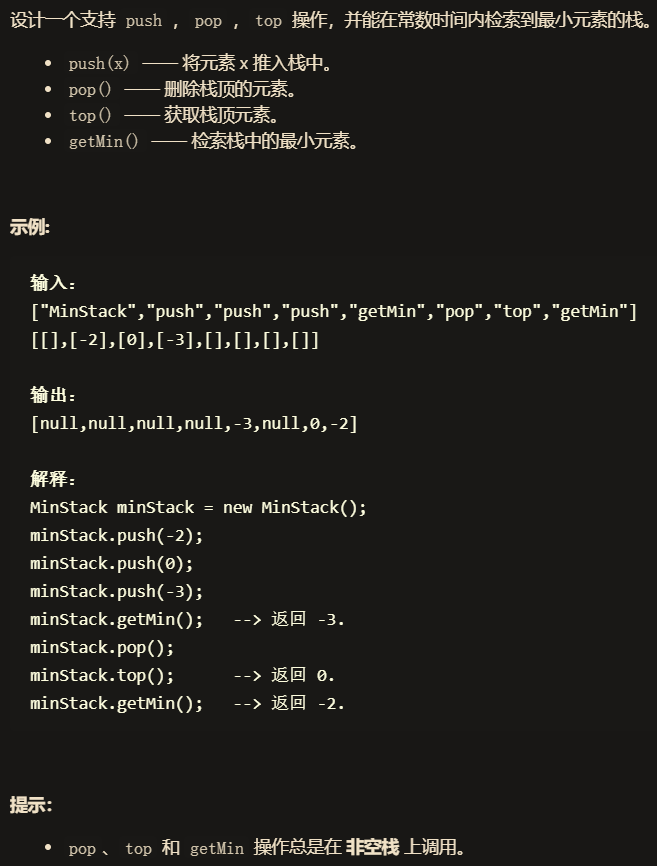
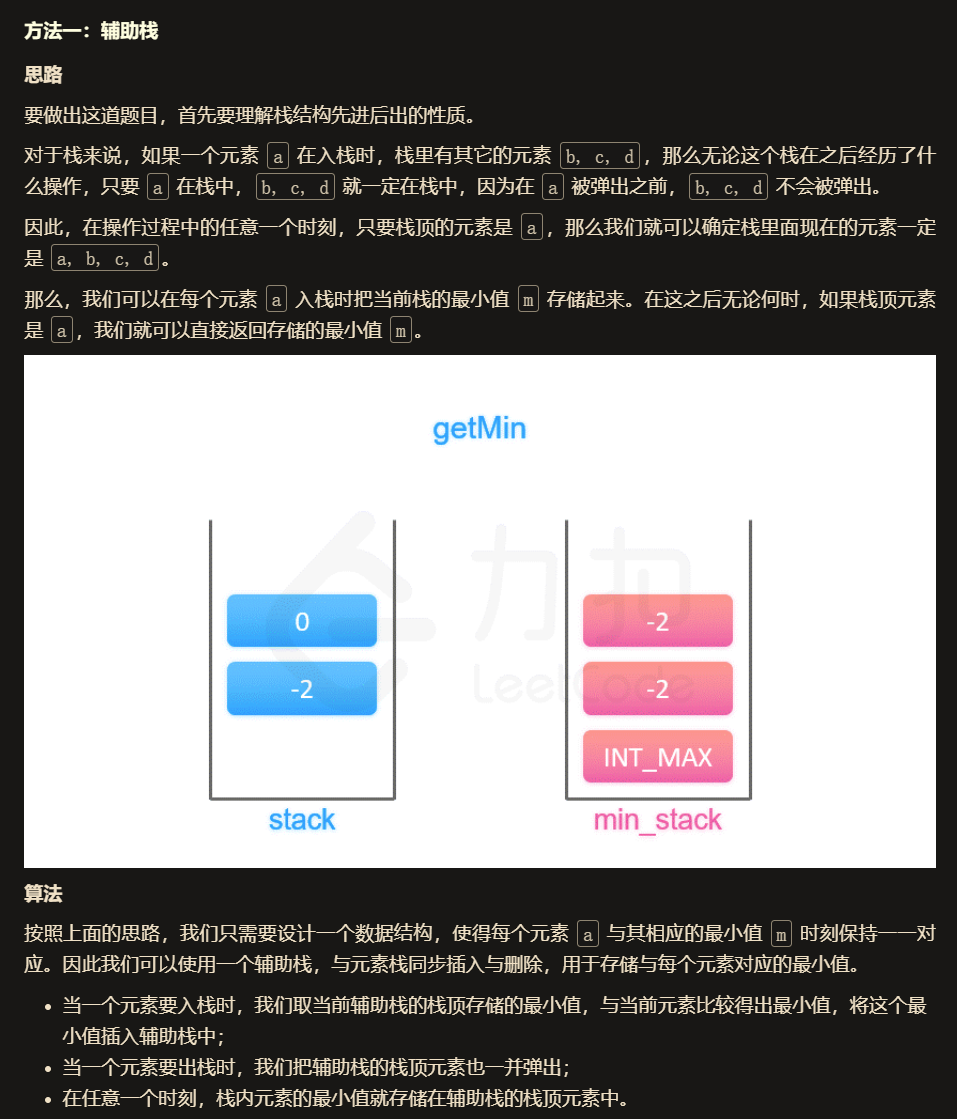
class MinStack {
stack x_stack;
stack min_stack;
public:
MinStack() {
min_stack.push(INT_MAX);
}
void push(int x) {
x_stack.push(x);
min_stack.push(min(min_stack.top(), x));
}
void pop() {
x_stack.pop();
min_stack.pop();
}
int top() {
return x_stack.top();
}
int getMin() {
return min_stack.top();
}
};
### [739. 每日温度]( )
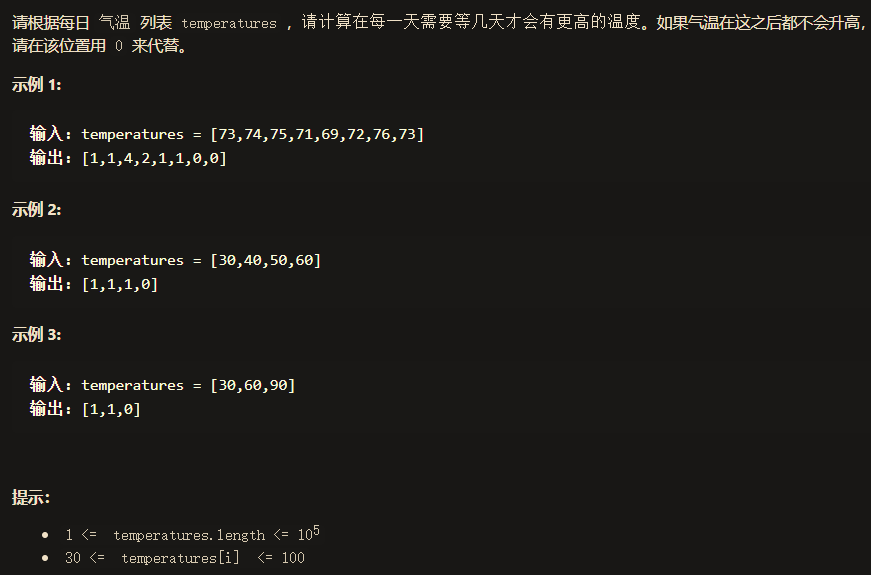
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-EPywWZCg-1631409448387)([外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-JSdOIJAZ-1631410035291)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906131429529.png#pic\_center)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-ueN4ESGE-1631410028435)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906131429529.png#pic\_center)]
[外链图片转存中…(img-YLbHIkti-1631410023714)]
)]
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-4awxo6MT-1631409448387)(https://raw.githubusercontent.com/xkyvvv/blogpic/main/pic1/image-20210906131445488.png)]
class Solution {
public:
vector dailyTemperatures(vector& temperatures) {
int n = temperatures.size();
vector ans(n);
stack s;
for (int i = 0; i < n; ++i) {
while (!s.empty() && temperatures[i] > temperatures[s.top()]) {
int previousIndex = s.top();
ans[previousIndex] = i - previousIndex;
s.pop();
}
s.push(i);
}
return ans;
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上Go语言开发知识点,真正体系化!
ex = s.top();
ans[previousIndex] = i - previousIndex;
s.pop();
}
s.push(i);
}
return ans;
[外链图片转存中…(img-mylvUsQc-1726126044972)]
[外链图片转存中…(img-qkK0gbhP-1726126044973)]
[外链图片转存中…(img-SYswsyyK-1726126044974)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上Go语言开发知识点,真正体系化!