一、time
①函数原型:time_t time(time_t *tloc)
②功能:获得1970年1月一日到现在的秒数
③参数:tlooc:存放秒数空间的首地址
④返回值:成功返回秒数,失败返回-1
#include "head.h"
int main(int argc, char const *argv[])
{
time_t t;
t = time(&t);
printf("%ld\n",t);
return 0;
}
输出结果:
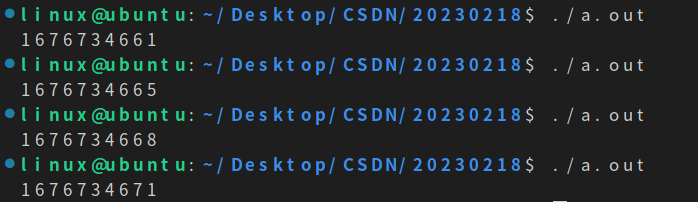
二、localtime
①函数原型:struct tm *localtime(const time_t *timep)
②功能:将time_t类型的时间转换为日历时间
③参数:timep:存放秒数空间的首地址
④返回值:成功返回时间结构体空间首地址,失败返回NULL
struct tm {
int tm_sec; /* Seconds (0-60) */
int tm_min; /* Minutes (0-59) */
int tm_hour; /* Hours (0-23) */
int tm_mday; /* Day of the month (1-31) */
int tm_mon; /* Month (0-11) */
int tm_year; /* Year - 1900 */
int tm_wday; /* Day of the week (0-6, Sunday = 0) */
int tm_yday; /* Day in the year (0-365, 1 Jan = 0) */
int tm_isdst; /* Daylight saving time */
}
#include "head.h"
int main(int argc, char const *argv[])
{
time_t t;
char *pret = NULL;
struct tm *ptm = NULL;
time(&t);
ptm = localtime(&t);
printf("%04d-%02d-%02d %02d:%02d:%02d\n",ptm ->tm_year+1900,ptm->tm_mon+1, ptm->tm_mday, ptm->tm_hour, ptm->tm_min, ptm->tm_sec);
return 0;
}
输出结果:

三、symlink/link
symlink
①函数原型:int symlink(const char *target, const char *linkpath)
②功能:创建一个链接向target文件的软链接文件
#include "head.h"
int main(int argc, char const *argv[])
{
char tmp[4096] = {0};
symlink("file.txt","a.txt");//创建软连接
link("file.txt","b.txt");
readlink("a.txt", tmp, sizeof(tmp));
printf("tmpbuff = %s\n", tmp);
return 0;
}
输出结果:

2.link
①函数原型:int link(const char *oldpath, const char *newpath)
②功能:创建一个链接向oidpath的newpath硬链接文件
#include "head.h"
int main(int argc, char const *argv[])
{
char tmp[4096] = {0};
symlink("file.txt","a.txt");
link("file.txt","b.txt");//创建硬链接
readlink("a.txt", tmp, sizeof(tmp));
printf("tmpbuff = %s\n", tmp);
return 0;
}
输出结果:

3.readlink
①函数原型:ssize_t readlink(const char *pathname, char *buf, size_t bufsiz);
②功能:读取链接文件本身的内容
#include "head.h"
int main(int argc, char const *argv[])
{
char tmp[4096] = {0};
symlink("file.txt","a.txt");
link("file.txt","b.txt");
readlink("a.txt", tmp, sizeof(tmp));//读取链接文件本身的内容(它的链接项)
printf("tmpbuff = %s\n", tmp);//输出所读到的文价本身
return 0;
}
输出结果:

所读取的文件内容为file.txt
四、stat
①函数原型:int stat(const char *pathname, struct stat *statbuf)
②功能:获得pathname对应的文件信息存放入statbuf指向的空间中
③参数:pathname:文件路径 statbuf:存放信息空间首地址
④返回值:成功返回0,失败返回-1
struct stat {
dev_t st_dev; /* ID of device containing file */
ino_t st_ino; /* Inode number */
mode_t st_mode; /* File type and mode */
nlink_t st_nlink; /* Number of hard links */
uid_t st_uid; /* User ID of owner */
gid_t st_gid; /* Group ID of owner */
dev_t st_rdev; /* Device ID (if special file) */
off_t st_size; /* Total size, in bytes */
blksize_t st_blksize; /* Block size for filesystem I/O */
blkcnt_t st_blocks; /* Number of 512B blocks allocated */
/* Since Linux 2.6, the kernel supports nanosecond
precision for the following timestamp fields.
For the details before Linux 2.6, see NOTES. */
struct timespec st_atim; /* Time of last access */
struct timespec st_mtim; /* Time of last modification */
struct timespec st_ctim; /* Time of last status change */
#define st_atime st_atim.tv_sec /* Backward compatibility */
#define st_mtime st_mtim.tv_sec
#define st_ctime st_ctim.tv_sec
};
#include "head.h"
int main(int argc, char const *argv[])
{
int ret = 0;
struct stat buf;
struct tm *ptm = NULL;
if (2 != argc)
{
fprintf(stderr, "Usage:./a.out filename\n");
return -1;
}
ret = lstat(argv[1], &buf);
if (-1 == ret)
{
perror("fail to stat");
return -1;
}
if (S_ISREG(buf.st_mode))
{
putchar('-');
}
else if (S_ISDIR(buf.st_mode))
{
putchar('d');
}
else if (S_ISCHR(buf.st_mode))
{
putchar('c');
}
else if (S_ISBLK(buf.st_mode))
{
putchar('b');
}
else if (S_ISFIFO(buf.st_mode))
{
putchar('p');
}
else if (S_ISLNK(buf.st_mode))
{
putchar('l');
}
else if (S_ISSOCK(buf.st_mode))
{
putchar('s');
}
buf.st_mode & S_IRUSR ? putchar('r') : putchar('-');
buf.st_mode & S_IWUSR ? putchar('w') : putchar('-');
buf.st_mode & S_IXUSR ? putchar('x') : putchar('-');
buf.st_mode & S_IRGRP ? putchar('r') : putchar('-');
buf.st_mode & S_IWGRP ? putchar('w') : putchar('-');
buf.st_mode & S_IXGRP ? putchar('x') : putchar('-');
buf.st_mode & S_IROTH ? putchar('r') : putchar('-');
buf.st_mode & S_IWOTH ? putchar('w') : putchar('-');
buf.st_mode & S_IXOTH ? putchar('x') : putchar('-');
printf(" %ld", buf.st_nlink);
printf(" %d", buf.st_uid);
printf(" %d", buf.st_gid);
printf(" %ld", buf.st_size);
ptm = localtime(&buf.st_ctime);
printf(" %02d %02d %02d:%02d", ptm->tm_mon+1, ptm->tm_mday, ptm->tm_hour, ptm->tm_min);
printf(" %s", argv[1]);
printf("\n");
return 0;
}
注意:
主函数传参:
①int main(void)
②void main(void)
③int main(int argc, const char *argv[])
④int main(int argc, const char **argv)
argc:传入参数的个数
argv:指针数组
五、getpwuid/getgrgid
1.getpwuid
①函数原型:struct passwd *getpwuid(uid_t uid)
②功能:根据uid获得用户信息
③参数:uid:用户ID号
④返回值:成功返回用户信息结构体空间首地址,失败返回NULL
struct passwd {
char *pw_name; /* username */
char *pw_passwd; /* user password */
uid_t pw_uid; /* user ID */
gid_t pw_gid; /* group ID */
char *pw_gecos; /* user information */
char *pw_dir; /* home directory */
char *pw_shell; /* shell program */
}
eg:利用IO完成ls的功能
#include "head.h"
int displayfile(char *pfilename)
{
struct stat buf;
int ret = 0;
struct passwd *pwd = NULL;
struct group *grp = NULL;
struct tm *ptm = NULL;
char *pmon[12] = {"Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"};
ret = lstat(pfilename, &buf);
if (-1 == ret)
{
perror("fail to lstat");
return -1;
}
if (S_ISREG(buf.st_mode))
{
putchar('-');
}
else if (S_ISDIR(buf.st_mode))
{
putchar('d');
}
else if (S_ISCHR(buf.st_mode))
{
putchar('c');
}
else if (S_ISBLK(buf.st_mode))
{
putchar('b');
}
else if (S_ISFIFO(buf.st_mode))
{
putchar('p');
}
else if (S_ISLNK(buf.st_mode))
{
putchar('l');
}
else if (S_ISSOCK(buf.st_mode))
{
putchar('s');
}
buf.st_mode & S_IRUSR ? putchar('r') : putchar('-');
buf.st_mode & S_IWUSR ? putchar('w') : putchar('-');
buf.st_mode & S_IXUSR ? putchar('x') : putchar('-');
buf.st_mode & S_IRGRP ? putchar('r') : putchar('-');
buf.st_mode & S_IWGRP ? putchar('w') : putchar('-');
buf.st_mode & S_IXGRP ? putchar('x') : putchar('-');
buf.st_mode & S_IROTH ? putchar('r') : putchar('-');
buf.st_mode & S_IWOTH ? putchar('w') : putchar('-');
buf.st_mode & S_IXOTH ? putchar('x') : putchar('-');
printf(" %ld", buf.st_nlink);
pwd = getpwuid(buf.st_uid); //获得用户ID Linux
printf(" %s", pwd->pw_name);
grp = getgrgid(buf.st_gid);
printf(" %s", grp->gr_name);
printf(" %5ld", buf.st_size);
ptm = localtime(&buf.st_ctime);
printf(" %s %2d %02d:%02d", pmon[ptm->tm_mon], ptm->tm_mday, ptm->tm_hour, ptm->tm_min);
printf(" %s", pfilename);
printf("\n");
return 0;
}
int MyLsl(void)
{
DIR *dp = NULL;
struct dirent *pp = NULL;
dp = opendir(".");
if (NULL == dp)
{
perror("fail to opendir");
return -1;
}
while (1)
{
pp = readdir(dp);
if (NULL == pp)
{
break;
}
if ('.' == pp->d_name[0])
{
continue;
}
displayfile(pp->d_name);
}
closedir(dp);
return 0;
}
int main(int argc, char const *argv[])
{
MyLsl();
return 0;
}
注意:在头文件中添加#include <pwd.h>
输出结果:
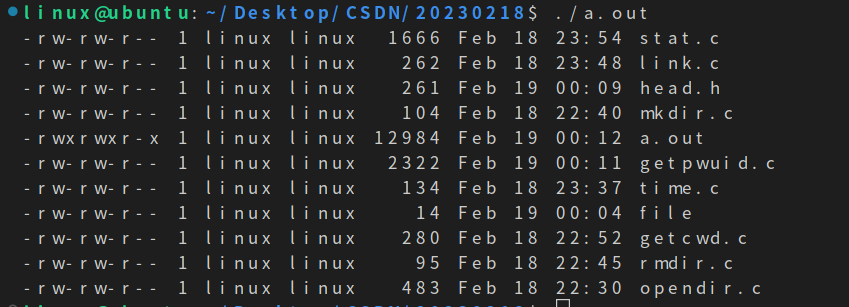
2.getgrgid
①函数原型:struct group *getgrgid(gid_t gid)
②功能:根据组ID获得组信息
③参数:gid:组ID
④返回值:成功返回ID对应的组信息,失败返回NULL
eg:利用IO完成ls的功能
#include "head.h"
int displayfile(char *pfilename)
{
struct stat buf;
int ret = 0;
struct passwd *pwd = NULL;
struct group *grp = NULL;
struct tm *ptm = NULL;
char *pmon[12] = {"Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"};
ret = lstat(pfilename, &buf);
if (-1 == ret)
{
perror("fail to lstat");
return -1;
}
if (S_ISREG(buf.st_mode))
{
putchar('-');
}
else if (S_ISDIR(buf.st_mode))
{
putchar('d');
}
else if (S_ISCHR(buf.st_mode))
{
putchar('c');
}
else if (S_ISBLK(buf.st_mode))
{
putchar('b');
}
else if (S_ISFIFO(buf.st_mode))
{
putchar('p');
}
else if (S_ISLNK(buf.st_mode))
{
putchar('l');
}
else if (S_ISSOCK(buf.st_mode))
{
putchar('s');
}
buf.st_mode & S_IRUSR ? putchar('r') : putchar('-');
buf.st_mode & S_IWUSR ? putchar('w') : putchar('-');
buf.st_mode & S_IXUSR ? putchar('x') : putchar('-');
buf.st_mode & S_IRGRP ? putchar('r') : putchar('-');
buf.st_mode & S_IWGRP ? putchar('w') : putchar('-');
buf.st_mode & S_IXGRP ? putchar('x') : putchar('-');
buf.st_mode & S_IROTH ? putchar('r') : putchar('-');
buf.st_mode & S_IWOTH ? putchar('w') : putchar('-');
buf.st_mode & S_IXOTH ? putchar('x') : putchar('-');
printf(" %ld", buf.st_nlink);
pwd = getpwuid(buf.st_uid);
printf(" %s", pwd->pw_name);
grp = getgrgid(buf.st_gid);//获得组ID信息: Linux
printf(" %s", grp->gr_name);
printf(" %5ld", buf.st_size);
ptm = localtime(&buf.st_ctime);
printf(" %s %2d %02d:%02d", pmon[ptm->tm_mon], ptm->tm_mday, ptm->tm_hour, ptm->tm_min);
printf(" %s", pfilename);
printf("\n");
return 0;
}
int MyLsl(void)
{
DIR *dp = NULL;
struct dirent *pp = NULL;
dp = opendir(".");
if (NULL == dp)
{
perror("fail to opendir");
return -1;
}
while (1)
{
pp = readdir(dp);
if (NULL == pp)
{
break;
}
if ('.' == pp->d_name[0])
{
continue;
}
displayfile(pp->d_name);
}
closedir(dp);
return 0;
}
int main(int argc, char const *argv[])
{
MyLsl();
return 0;
}
注意:在头文件中添加#include #include <grp.h>
输出结果同上