代码:
public static List<Integer> spiralOrder(int[][] matrix) { //上 int top = 0; //下 int bottom = matrix.length-1; //左 int left = 0; //右 int right = matrix[0].length-1; // b决定下边界 d决定右边界 List<Integer> order = new ArrayList<>(); //不能跳跃打印,顺序逻辑,一方不满足 直接break if(matrix.length == 1){ for(int i = 0; i < matrix[0].length; i++){ order.add(matrix[0][i]); } return order; } if(matrix[0].length == 1){ for(int i = 0; i < matrix.length; i++){ order.add(matrix[i][0]); } return order; } while (left <= right && top <= bottom) { for (int column = left; column <= right; column++) { order.add(matrix[top][column]); } for (int row = top + 1; row <= bottom; row++) { order.add(matrix[row][right]); } if(left + 1 > right || top + 1 > bottom){ break; } for (int column = right - 1; column > left; column--) { order.add(matrix[bottom][column]); } for (int row = bottom; row > top; row--) { order.add(matrix[row][left]); } left++; right--; top++; bottom--; } return order; }
其关键点在于 if(left + 1 > right || top + 1 > bottom)这句代码 其用来结束两种情况
1.left + 1 > right :此时已经完成了顺时针左到右的打印,所以需要+1,如果此时left+1>right,代表左右之间没有空行了,之间结束循环,返回
类比这种情况,在完成第一次顺时针打印后,left++变成1,right--变成1,但是上下之间还是有空行,所以如果不执行if(left + 1 > right){break;},则最后还会执行自下向上的打印,最后的打印结构会多一个5
2.top + 1 > bottom:这种情况是考虑到上下已经没有行了,但是左右还有行的情况,但这种情况时
类比这种情况,如果不判断top+1 > bottom则结束循环的话,那么在执行第二遍顺时针打印时,发现左右还有空间(left = 1 right = 2)此时还会执行一个自右向左的打印,最终结果会多一个6
矩阵题目:顺时针顺序打印矩阵
最新推荐文章于 2024-11-13 23:40:34 发布
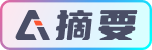