来自:http://www.dotnetcurry.com/ShowArticle.aspx?ID=454&AspxAutoDetectCookieSupport=1
This article demonstrates how to populate an ASP.NET ListBox using data coming from a JSON Serialized ASP.NET Web Service. This article is a sample chapter from my EBook called
51 Tips, Tricks and Recipes with jQuery and ASP.NET Controls
. The chapter has been modified to publish it as an article.
Note that for demonstration purposes, I have included jQuery code in the same page. Ideally, these resources should be created in separate folders for maintainability.
Let us quickly jump to the solution and see how to populate an ASP.NET ListBox from data coming from a JSON Serialized ASP.NET Web Service
<htmlxmlns="http://www.w3.org/1999/xhtml">
<headid="Head1" runat="server">
<title>Populate a ListBox from JSON Results</title>
<script language="javascript" type="text/javascript"
src="http://code.jquery.com/jquery-latest.js"></script>
<script type="text/javascript">
$(function() {
var firstParam = 'F';
var radBtn = $("table.tbl input:radio");
var lBox = $('select[id$=lb]');
$(radBtn).click(function() {
lBox.empty();
var firstParam = $(':radio:checked').val();
$.ajax({
type:"POST",
url:"Services/EmployeeList.asmx/FetchEmpOnGender",
data:"{empSex:\"" + firstParam + "\"}",
contentType:"application/json; charset=utf-8",
dataType:"json",
success:function(msg) {
var gender = msg.d;
if (gender.length > 0) {
var listItems = [];
for (var key in gender) {
listItems.push('<option value="' +
key +'">' + gender[key].FName
+'</option>');
}
$(lBox).append(listItems.join(''));
}
else {
alert("No records found");
}
},
error:function(XMLHttpRequest, textStatus, errorThrown) {
alert(textStatus);
}
});
});
});
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2>Click on a Radio Button to retrieve 'Gender' based data</h2>
<br /><br />
<asp:RadioButtonListID="rbl" runat="server" class="tbl"
ToolTip="Click on this RadioButton to retrieve data">
<asp:ListItemText="Male" Value="M"></asp:ListItem>
<asp:ListItemText="Female" Value="F"></asp:ListItem>
</asp:RadioButtonList><br/>
<asp:ListBoxID="lb" runat="server"></asp:ListBox>
</div>
</form>
</body>
</html>
In this example, we will see how to consume an ASP.NET Web Service (EmployeeList.asmx) that is JSON Serialized. The data source for this web service is List<Employees> in the Employee.cs class. The class can be downloaded from the
source code
available with this article. Here’s a snapshot of what the class looks like
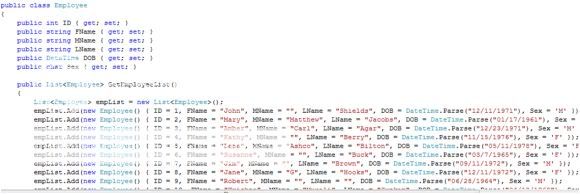
If you look at the EmployeeList.cs file, you will observe that the method has been decorated with the [WebMethod] attribute to allow calls from client script
C#
[WebMethod]
public List<Employee> FetchEmpOnGender(char empSex)
{
var emp = new Employee();
var fetchEmp = emp.GetEmployeeList()
.Where(m => m.Sex == empSex);
return fetchEmp.ToList();
}
VB.NET
<WebMethod> _
PublicFunction FetchEmpOnGender(ByVal empSexAs Char) As List(Of Employee)
Dim emp = New Employee()
Dim fetchEmp = emp.GetEmployeeList().Where(Function(m) m.Sex = empSex)
Return fetchEmp.ToList()
EndFunction
The FetchEmpOnGender() method calls the GetEmployeeList() method on the Employee class which returns a List<Employee>. The UI contains two radio buttons ‘Male’ and ‘Female’ which when selected, passes in the gender information to the web service. Depending on the input received, the web service method filters and returns data for the gender requested.
Note: If a method is not marked with [ScriptMethod] attribute, the method will be called by using the HTTP POST command and the response will be serialized as JSON.
To consume this web service using jQuery$.ajax(), two important points to note is that the request should be a POST request and the request’s content-type must be ‘application/json; charset=utf-8’. The code structure looks similar to the following:
$.ajax({
type:"POST",
url:"Services/EmployeeList.asmx/FetchEmpOnGender",
data:"{empSex:\"" + firstParam + "\"}",
contentType:"application/json; charset=utf-8",
dataType:"json",
success:function(msg) {
},
error:function(XMLHttpRequest, textStatus, errorThrown) {
}
Also observe the way we are passing in the gender parameter using a ‘special formatting’ in the$.ajax() call
data:"{empSex:\"" + firstParam + "\"}"
Once we receive the results, we loop through it and populate an array ‘listItems’ with the FirstName values by passing in a key to the FName value (gender[key].FName))
success:function(msg) {
var gender = msg.d;
if (gender.length > 0) {
var listItems = [];
for (var key in gender) {
listItems.push('<option value="' +
key +'">' + gender[key].FName
+'</option>');
}
The final step is to populate the ListBox using the populated array
$(lBox).append(listItems.join(''));
When you run the application, click on the radio button, to retrieve the FirstName of Employees. Clicking on the ‘Male’ radio button fetches the following results:
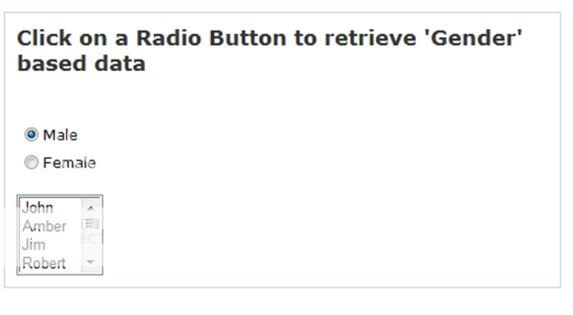
Whereas clicking on the ‘Female’ radio button fetches the following results:
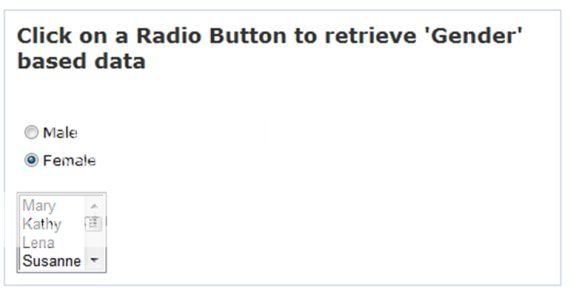
See a
Live Demo
I hope you found this article useful and I thank you for viewing it. This article was taken from my EBook called
51 Tips, Tricks and Recipes with jQuery and ASP.NET Controls
which contains similar recipes that you can use in your applications. The entiresource code of this article can be downloaded over
here
相关下载资源:
https://skydrive.live.com/?cid=2c5f5b0560e374cb&id=2C5F5B0560E374CB!285