响应式核心
ref 定义响应是值类型方法
reactive 引用类型
computed 从现有数据计算新的数据
watch监听数据的变化
watchEffect监听副作用(只要被这个方法引用的数据,发送变化都会触发)
readyonly只读常用与优化
<template>
<view>
<view>setup 语法糖</view>
<view @click="copyn+=1">copyn:{{copyn}}</view>
<view class="title">watch监听</view>
<button size="mini" @click="stop()">停止监听</button>
<view>运费fee:{{fee}}</view>
<button size="mini" @click="fee=fee+5">设置</button>
<view>计算结果dn:{{dn}}</view>
<button @click="n++">{{n}}</button>
<!-- 单击确认 -->
<input
@confirm="addList"
v-model="temp"
placeholder="添加计划"/>
<view>
<view v-for="(item,index) in list" :key="item">
{{item}}
<button size="mini" @click="list.splice(index,1)">x</button>
</view>
</view>
</view>
</template>
<script setup>
// ref 定义响应是值类型方法
// reactive 引用类型
// computed 从现有数据计算新的数据
// watch监听数据的变化
// watchEffect监听副作用(只要被这个方法引用的数据,发送变化都会触发)
// readyonly只读常用与优化
import {ref,reactive,computed,watch,watchEffect,readonly} from 'vue'
// 定义响应式数据n 默认值是8
const n = ref(8);
const copyn = readonly(n);
const list = reactive(uni.getStorageSync("list")||[])
// 定义临时数据
const temp = ref('');
const addList = (e)=>{
console.log(e)
// 在list添加一个元素为当前事件input的值
list.unshift(e.detail.value)
temp.value = '';
}
//计算computed
const dn = computed(()=>n.value*2)
//计算fee
const fee = computed({
get(){ //获取fee的时候执行get
if(n.value==1){return 7}
else{
var total = n.value*5+2;
return total;
}
},
set(v){//设置fee的时候执行 set方法
n.value = Math.floor((v-2)/5)
}
})
// 当list变化时候存储list
// 当组件创建完毕获取list
const stop = watch(list,(val,oldVal)=>{
// uni.setStorageSync("list",list)
})
// 无论是n还是list变化都需要存储n与list
watchEffect(()=>{
uni.setStorageSync("list",list);
uni.setStorageSync("n",n.value)
},{deep:true})
</script>
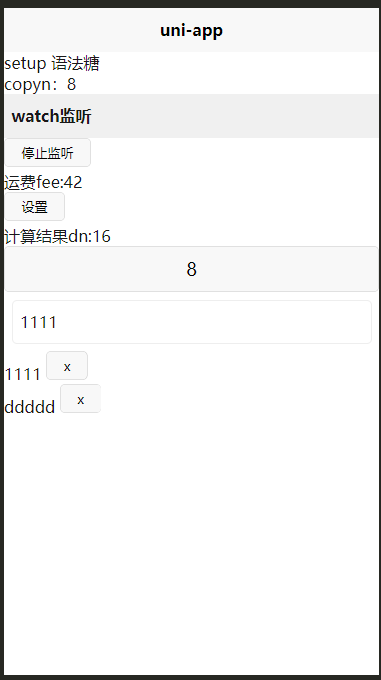
生命周期
beforeCreate 创建前
created 创建后
beforeMount 挂载前
mounted 挂载后
beforeUpdate 更新前
updated 更新后
beforeUnmount 卸载前
unmounted 卸载后
依赖注入
在组合式 API 中使用 provide/inject,两个只能在 setup 期间调用,使用之前,必须从 vue 显示导
入 provide/inject 方法。
provide 函数接收两个参数:
provide( name,value )
name:定义提供 property 的 name 。
value :property 的值。
使用时:
import { provide } from "vue"
export default {
setup(){
provide('info',"值")
}
}
inject 函数有两个参数:
inject(name,default)
name:接收 provide 提供的属性名。
default:设置默认值,可以不写,是可选参数。
使用时:
import { inject } from "vue"
export default {
setup(){
inject('info',"设置默认值")
}
}
完整实例1:provide/inject实例
//父组件代码
<script>
import { provide } from "vue"
export default {
setup(){
provide('info',"值")
}
}
</script>
//子组件 代码
<template>
{{info}}
</template>
<script>
import { inject } from "vue"
export default {
setup(){
const info = inject('info')
return{
info
}
}
}
</script>
4.组件相关
defineProps 定义props
const props = defineProps({
// 长度
"maxLength":{
type:[Number,String],
default:0,
},
// 默认值
"modelValue":{
type:String,
default:"",
},
})
defineEmit
cosnt emits = defineEmit(["update:"update:modelValue"])
emits("update:modelValue",content.value)
5.父子传值和子父传值
父传子:
第一步:Father.vue
<template>
<h2>父组件</h2>
<hr>
<Son :num="num" :arr="array" ></Son>
</template>
<script lang="ts" setup>
import {ref} from 'vue'
import Son from "./Son.vue";
let num = ref(6688)
let array = ref([11, 22, 33, 44])
</script>
第二步:Sun.vue
<template>
<h2>子组件</h2>
{{props.num}}--{{props.arr}}
</template>
<script lang="ts" setup>
let props = defineProps({
num: Number,
arr: {
type: Array,
default: () => [1, 2, 3, 4]
}
})
</script>
子传父:
第一步:Sun.vue
<template>
<h2>子组件</h2>
<button @click="sendMsg">向父组件传递数据</button>
</template>
<script lang="ts" setup>
import {ref} from 'vue'
const emit = defineEmits(["son_sendMsg"]);
const msg = ref("子组件传递给父组件的数据")
function sendMsg() {
emit("son_sendMsg", msg.value)
}
</script>
第二步:Father.vue:
<template>
<h2>父组件</h2>
{{ message }}
<hr>
<Son @son_sendMsg="fun"></Son>
</template>
<script lang="ts" setup>
import {ref} from 'vue'
import Son from "./Son.vue"
let message = ref("")
function fun(msg) {
message.value = msg
}
</script>