SpringMVC执行流程图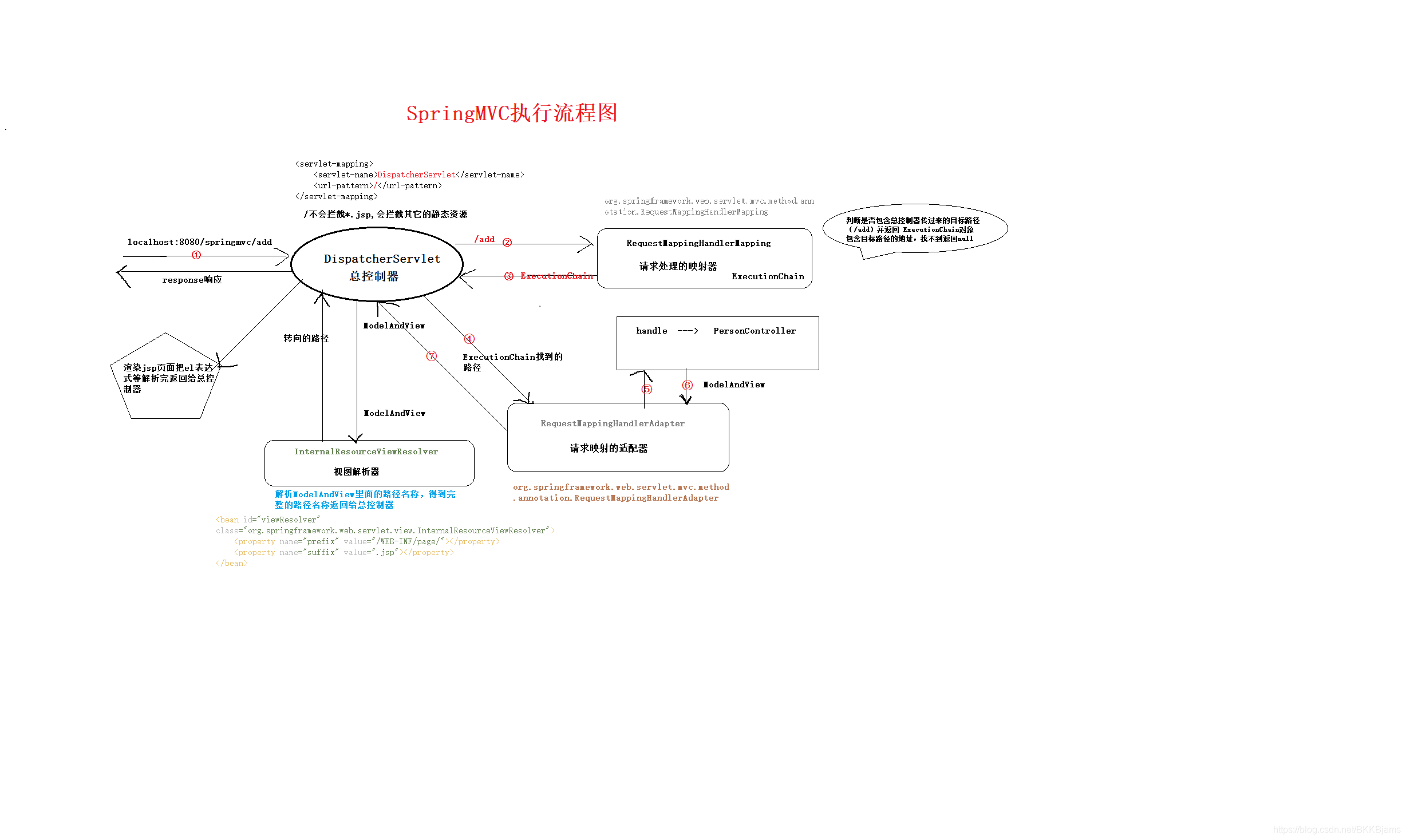
- web.xml
<!--springmvc入口servlet配置-->
<servlet>
<servlet-name>DispatcherServlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:springmvc.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>DispatcherServlet</servlet-name>
<url-pattern>/</url-pattern>
<!--拦截任意路径-->
<!--<url-pattern>/*</url-pattern>-->
<!--不会拦截jsp 会拦截除.jsp以外的所有路径的静态资源-->
<!--走内置的tomcat default-->
<!--<url-pattern>/</url-pattern>-->
<!--拦截匹配后缀的请求 不会拦截静态资源 -->
<!--<url-pattern>*.action</url-pattern>-->
</servlet-mapping>
2.springmvc.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.1.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-4.1.xsd">
<!--开启包扫描-->
<context:component-scan base-package="cn.wzy"></context:component-scan>
<!--静态资源映射-->
<!-- <mvc:resources mapping="/images/**" location="/images/"></mvc:resources>
<mvc:resources mapping="/js/**" location="/js/"></mvc:resources>-->
<!--静态资源交给默认的Servlet的处理 等价于上面的写法 这个相当于一个总的方便 -->
<!--<mvc:default-servlet-handler></mvc:default-servlet-handler>-->
<!--请求映射的处理器-->
<!-- <bean id="handlerMapping"
class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping"></bean>
<!–请求映射的适配器–>
<bean id="handlerAdapter"
class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter"></bean>
-->
<!--注解驱动 包含上面的 请求映射适配器 和请求映射的处理器-->
<mvc:annotation-driven conversion-service="conversionService"></mvc:annotation-driven>
<bean id="conversionService" class="org.springframework.context.support.ConversionServiceFactoryBean">
<property name="converters">
<set>
<bean class="cn.wzy.converter.DateConverter"></bean>
</set>
</property>
</bean>
<!--静态资源交给默认的Servlet的处理 一定要写在注解驱动的下面 不然会报错 -->
<mvc:default-servlet-handler></mvc:default-servlet-handler>
<!--视图解析器-->
<bean id="viewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/page/"></property>
<property name="suffix" value=".jsp"></property>
</bean>
</beans>
- 用fifter过滤器解决中文乱码问题
<!--fifter过滤器解决中文乱码问题-->
<filter>
<filter-name>CharacterEncodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>utf-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>CharacterEncodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
- 前端jsp页面
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<script src="/springmvc001/My97DatePicker/WdatePicker.js"></script>
<body>
<h1>员工注册信息</h1>
<form action="/springmvc001/register5" method="post">
<table>
<tr>
<td>姓名:</td>
<td><input type="text" name="name"></td>
</tr>
<tr>
<td>姓别:</td>
<td><input type="radio" name="gender" checked="checked" value="man">男
<input type="radio" name="gender" value="female" >女</td>
</tr>
<tr>
<!-- 在实际开发中日期类为了同意格式 提供插件选择 不会让客户直接自己输入-->
<td>出生日期:</td>
<td><input type="text" name="birthday" id="birthday" onfocus="WdatePicker({el:this})" readonly="readonly">
</td>
</tr>
<tr>
<td>年龄:</td>
<td><input type="text" name="age"></td>
</tr>
<tr>
<td>狗名:</td>
<td><input type="text" name="dog.dogName"></td>
</tr>
<tr>
<td>毛色:</td>
<td><input type="text" name="dog.color"></td>
</tr>
<tr>
<td>第一本书书名:</td>
<td><input type="text" name="books[0].bookName"></td>
</tr>
<tr>
<td>第一本书作者:</td>
<td><input type="text" name="books[0].bookAuthor"></td>
</tr>
<tr>
<td>第一本书价格:</td>
<td><input type="text" name="books[0].bookPrice"></td>
</tr>
<tr>
<td>第二本书书名:</td>
<td><input type="text" name="books[1].bookName"></td>
</tr>
<tr>
<td>第二本书作者:</td>
<td><input type="text" name="books[1].bookAuthor"></td>
</tr>
<tr>
<td>第二本书价格:</td>
<td><input type="text" name="books[1].bookPrice"></td>
</tr>
<tr>
<td colspan="2"><input type="submit" value="注册"></td>
</tr>
</table>
</form>
</body>
</html>
- 解决Date日期类与字符串映射不匹配问题
package cn.wzy.converter;
import org.springframework.core.convert.converter.Converter;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
/**
* 日期类转换器
*/
public class DateConverter implements Converter<String, Date> {
@Override
public Date convert(String source) {
try {
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd");
return format.parse(source);
} catch (ParseException e) {
e.printStackTrace();
}
return null;
}
}
- 控制层代码
@RequestMapping("/register5")
public ModelAndView addEmployee5(Employee employee){
System.out.println(employee.getName());
System.out.println(employee.getAge());
System.out.println(employee.getBirthday());
System.out.println(employee.getGender());
System.out.println(employee.getDog().getDogName());
System.out.println(employee.getDog().getColor());
List<Book> bookList = employee.getBooks();
for (Book book : bookList) {
System.out.println(book);
}
ModelAndView modelAndView = new ModelAndView();
modelAndView.setViewName("result");
modelAndView.addObject("message","注册成功");
return modelAndView;
}